JavaScript での文字列補間。
Introduction to Template Literals.
String manipulation is a very common task in programming, especially when building interactive web applications. If you've ever spent time working with JavaScript, then you've likely had to put some variables into strings.
In older versions of JavaScript, this meant using the + operator to join strings together through a process called string concatenation. However, with the introduction of template literals in the JavaScript ES6(2015) update. We now have a cleaner way to insert variables into strings, called string interpolation.
What are Template Literals?
Template literals allow us to manipulate strings easier. They are enclosed in backticks (`) rather than (') or ("), and they support string interpolation by using the (${}) syntax to place variables, or function calls directly into a string.
Here’s an example of how template literals simplify string interpolation.
const name = "John" const age = 24 // Old method using concatenation const greeting = "Hello, " + name + "! You are " + age + " years old." // New method using template literals const greetingWithTemplateLiteral = `Hello, ${name}! You are ${age} years old.` console.log(greetingWithTemplateLiteral) // Outputs: Hello, John! You are 24 years old.
Benefits of Using Template Literals
1. Improved Readability
When using string concatenation, it was easy to get lost in a bunch of + signs, especially when working with longer strings. Template literals avoid this by letting you write strings in a way that is easier to follow.
const product = "laptop" const price = 1400 console.log(`The price of the ${product} is $${price}`) // Outputs: The price of the laptop is $1400
2. Multi-line Strings
Before template literals, you had to use escape characters like \n to make multi-line strings. Now you can write them inside backticks(`).
// Old method const multiLineString1 = "This is the first line" + "\n" + "This is the second line" + "\n" + "This is the third line" // New method const multiLineString2 = `This is the first line This is the second line This is the third line` console.log(multiLineString1) console.log(multiLineString2) /* Both output: This is the first line This is the second line This is the third line */
3. Expression Evaluation
You can also perform calculations, call functions, or manipulate data inside strings.
const a = 1 const b = 10 console.log(`The sum of a and b is ${a + b}`) // Outputs: The sum of a and b is 11 const upperCaseName = (name) => name.toUpperCase() console.log(`Your name in uppercase is ${upperCaseName("John")}`) // Outputs: Your name in uppercase is JOHN
Common Use Cases in JavaScript
1. HTML Generation
Instead of building HTML strings with concatenation, you can put variables directly into the string with interpolation.
const name = "John" const htmlContent = ` <h1>Hello, ${name}!</h1> <p>Welcome to the site.</p> `
2. Logging Messages
You can also insert variables directly into log messages without the need for concatenation.
const user = "John" const action = "logged in" console.log(`User ${user} just ${action}`) // Outputs: User John just logged in
3. Creating URLs
Template literals make it easier to construct URLs as well.
const userId = 123 const apiUrl = `https://api.example.com/user/${userId}/details` console.log(apiUrl) // Outputs: https://api.example.com/user/123/details
4. Conditional Logic
Another great use case is Conditional logic. With template literals you can give strings simple conditions using the ternary operator (? :), which is a shorthand for an if-else condition.
Logical operators like && (and) or || (or) can also be used to add conditional parts to a string. This removes the need for extra if-else statements or the need for concatenation.
const isMember = true console.log(`User is ${isMember ? 'a member' : 'not a member'}`) // Outputs: User is a member
You can also add more complex expressions inside template literals.
/* In this example, the condition age >= 18 is evaluated the result is either “an adult” or “a minor” based on the value of age*/ const age = 24 const message = `You are ${age >= 18 ? 'an adult' : 'a minor'}` console.log(message) // Outputs: You are an adult /*In this, if isLoggedIn is true and username exists username is displayed or else, it defaults to “Guest” */ const isLoggedIn = true const username = "John" const greeting = `Welcome ${isLoggedIn && username ? username : 'Guest'}` console.log(greeting) // Outputs: Welcome John
Conclusion
Template literals in JavaScript offer a cleaner, and more efficient way to handle string interpolation. Between building web content, logging messages, or creating more readable code, this method provides the flexibility you need.
Next time your juggling with variables and strings, try using template literals. You'll quickly see why it's my go-to method for working with JavaScript.
Resources
- MDN Web Docs - Template Literals
- GitHub - Phase 1 Review Strings Lab
- W3 Schools - JavaScript Template Strings
以上がJavaScript での文字列補間。の詳細内容です。詳細については、PHP 中国語 Web サイトの他の関連記事を参照してください。

ホットAIツール

Undresser.AI Undress
リアルなヌード写真を作成する AI 搭載アプリ

AI Clothes Remover
写真から衣服を削除するオンライン AI ツール。

Undress AI Tool
脱衣画像を無料で

Clothoff.io
AI衣類リムーバー

Video Face Swap
完全無料の AI 顔交換ツールを使用して、あらゆるビデオの顔を簡単に交換できます。

人気の記事

ホットツール

メモ帳++7.3.1
使いやすく無料のコードエディター

SublimeText3 中国語版
中国語版、とても使いやすい

ゼンドスタジオ 13.0.1
強力な PHP 統合開発環境

ドリームウィーバー CS6
ビジュアル Web 開発ツール

SublimeText3 Mac版
神レベルのコード編集ソフト(SublimeText3)

ホットトピック










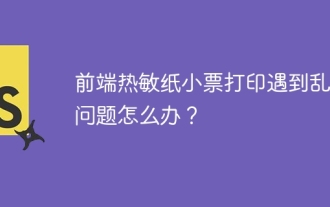
フロントエンドのサーマルペーパーチケット印刷のためのよくある質問とソリューションフロントエンド開発におけるチケット印刷は、一般的な要件です。しかし、多くの開発者が実装しています...
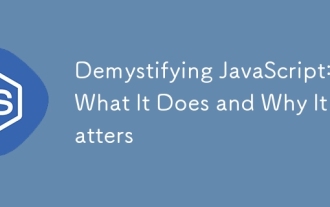
JavaScriptは現代のWeb開発の基礎であり、その主な機能には、イベント駆動型のプログラミング、動的コンテンツ生成、非同期プログラミングが含まれます。 1)イベント駆動型プログラミングにより、Webページはユーザー操作に応じて動的に変更できます。 2)動的コンテンツ生成により、条件に応じてページコンテンツを調整できます。 3)非同期プログラミングにより、ユーザーインターフェイスがブロックされないようにします。 JavaScriptは、Webインタラクション、シングルページアプリケーション、サーバー側の開発で広く使用されており、ユーザーエクスペリエンスとクロスプラットフォーム開発の柔軟性を大幅に改善しています。
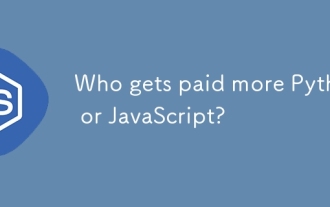
スキルや業界のニーズに応じて、PythonおよびJavaScript開発者には絶対的な給与はありません。 1. Pythonは、データサイエンスと機械学習でさらに支払われる場合があります。 2。JavaScriptは、フロントエンドとフルスタックの開発に大きな需要があり、その給与もかなりです。 3。影響要因には、経験、地理的位置、会社の規模、特定のスキルが含まれます。
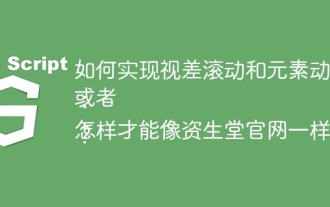
この記事の視差スクロールと要素のアニメーション効果の実現に関する議論では、Shiseidoの公式ウェブサイト(https://www.shisido.co.co.jp/sb/wonderland/)と同様の達成方法について説明します。
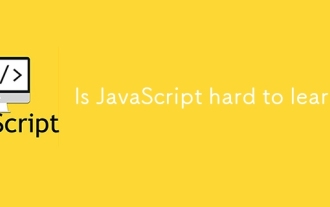
JavaScriptを学ぶことは難しくありませんが、挑戦的です。 1)変数、データ型、関数などの基本概念を理解します。2)非同期プログラミングをマスターし、イベントループを通じて実装します。 3)DOM操作を使用し、非同期リクエストを処理することを約束します。 4)一般的な間違いを避け、デバッグテクニックを使用します。 5)パフォーマンスを最適化し、ベストプラクティスに従ってください。
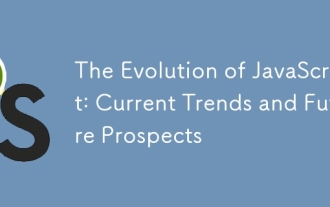
JavaScriptの最新トレンドには、TypeScriptの台頭、最新のフレームワークとライブラリの人気、WebAssemblyの適用が含まれます。将来の見通しは、より強力なタイプシステム、サーバー側のJavaScriptの開発、人工知能と機械学習の拡大、およびIoTおよびEDGEコンピューティングの可能性をカバーしています。
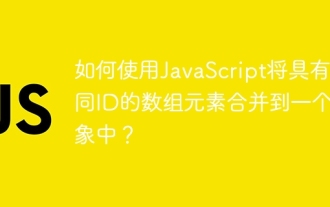
同じIDを持つ配列要素をJavaScriptの1つのオブジェクトにマージする方法は?データを処理するとき、私たちはしばしば同じIDを持つ必要性に遭遇します...
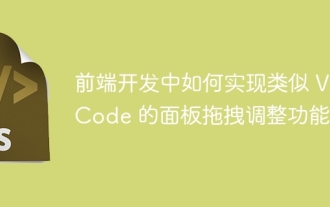
フロントエンドのVSCodeと同様に、パネルドラッグアンドドロップ調整機能の実装を調べます。フロントエンド開発では、VSCODEと同様のVSCODEを実装する方法...
