過剰な弾丸の発射を防ぐためのクールダウンメカニズムを実装するにはどうすればよいですか?
複数の弾丸の同時発射を防ぐ方法
元のコードでは、pygame が原因で複数の弾丸が同時に発射されていました。 .key.get_pressed() 関数は、キーが押されている限り True を返します。この動作は、プレイヤーの動きを制御するのには適していますが、キーを押すたびに 1 つの弾丸だけを発射したい場合の弾丸の発射には適していません。
この問題を解決するには、pygame.KEYDOWN を利用できます。このイベントは、キーが最初に押されたときに 1 回だけ発生します。 pygame.key.get_pressed() の代わりにこのイベントを使用することで、スペースバーが押されたときに弾丸が 1 つだけ発射されるようにすることができます。
さらに、弾丸の連射を防ぐための簡単なクールダウン メカニズムを実装できます。射撃。これは、次の弾丸を発射できる最も早い時間を表す next_bullet_threshold という変数を導入することで実現できます。弾丸が発射されるたびに、 next_bullet_threshold を現在時刻に必要なクールダウン期間を加えた時刻に更新します。現在の時間が next_bullet_threshold より大きいかどうかを確認することで、目的のクールダウン期間外に弾丸が発射されるのを防ぐことができます。
実装されたクールダウン メカニズムを使用して変更されたコードは次のとおりです:
<code class="python">import pygame pygame.init() red = 255,0,0 blue = 0,0,255 black = 0,0,0 screenWidth = 800 screenHeight = 600 gameDisplay = pygame.display.set_mode((screenWidth,screenHeight)) ## screen width and height pygame.display.set_caption('JUST SOME BLOCKS') ## set my title of the window clock = pygame.time.Clock() class player(): ## has all of my attributes for player 1 def __init__(self,x,y,width,height): self.x = x self.y = y self.height = height self.width = width self.vel = 5 self.left = False self.right = False self.up = False self.down = False class projectile(): ## projectile attributes def __init__(self,x,y,radius,colour,facing): self.x = x self.y = y self.radius = radius self.facing = facing self.colour = colour self.vel = 8 * facing # speed of bullet * the direction (-1 or 1) def draw(self,gameDisplay): pygame.draw.circle(gameDisplay, self.colour , (self.x,self.y),self.radius) ## put a 1 after that to make it so the circle is just an outline def redrawGameWindow(): for bullet in bullets: ## draw bullets bullet.draw(gameDisplay) pygame.display.update() #mainloop player1 = player(300,410,50,70) # moves the stuff from the class (when variables are user use player1.var) bullets = [] run = True next_bullet_threshold = 0 # Initialize the cooldown threshold bullet_cooldown = 500 # Set the desired cooldown period in milliseconds while run == True: clock.tick(27) for event in pygame.event.get(): if event.type == pygame.QUIT: run = False for bullet in bullets: if bullet.x < screenWidth and bullet.x > 0 and bullet.y < screenHeight and bullet.y > 0: ## makes sure bullet does not go off screen bullet.x += bullet.vel else: bullets.pop(bullets.index(bullet)) keys = pygame.key.get_pressed() ## check if a key has been pressed ## red player movement if keys[pygame.K_w] and player1.y > player1.vel: ## check if that key has been pressed down (this will check for w) and checks for boundry player1.y -= player1.vel ## move the shape in a direction player1.up = True player1.down = False if keys[pygame.K_a] and player1.x > player1.vel: ### this is for a player1.x -= player1.vel player1.left = True player1.right = False if keys[pygame.K_s] and player1.y < screenHeight - player1.height - player1.vel: ## this is for s player1.y += player1.vel player1.down = True player1.up = False if keys[pygame.K_d] and player1.x < screenWidth - player1.width - player1.vel: ## this is for d player1.x += player1.vel player1.right = True player1.left = False if keys[pygame.K_SPACE]: # shooting with the space bar current_time = pygame.time.get_ticks() if current_time >= next_bullet_threshold: # Check the cooldown timer if player1.left == True: ## handles the direction of the bullet facing = -1 else: facing = 1 if len(bullets) < 5: ## max amounts of bullets on screen bullets.append(projectile(player1.x + player1.width //2 ,player1.y + player1.height//2,6,black,facing)) ##just like calling upon a function next_bullet_threshold = current_time + bullet_cooldown # Update cooldown timer ## level gameDisplay.fill((0,255,0)) ### will stop the shape from spreading around and will have a background pygame.draw.rect(gameDisplay,(red),(player1.x,player1.y,player1.width,player1.height)) ## draw player pygame.display.update() redrawGameWindow() pygame.quit()</code>
以上が過剰な弾丸の発射を防ぐためのクールダウンメカニズムを実装するにはどうすればよいですか?の詳細内容です。詳細については、PHP 中国語 Web サイトの他の関連記事を参照してください。

ホットAIツール

Undresser.AI Undress
リアルなヌード写真を作成する AI 搭載アプリ

AI Clothes Remover
写真から衣服を削除するオンライン AI ツール。

Undress AI Tool
脱衣画像を無料で

Clothoff.io
AI衣類リムーバー

Video Face Swap
完全無料の AI 顔交換ツールを使用して、あらゆるビデオの顔を簡単に交換できます。

人気の記事

ホットツール

メモ帳++7.3.1
使いやすく無料のコードエディター

SublimeText3 中国語版
中国語版、とても使いやすい

ゼンドスタジオ 13.0.1
強力な PHP 統合開発環境

ドリームウィーバー CS6
ビジュアル Web 開発ツール

SublimeText3 Mac版
神レベルのコード編集ソフト(SublimeText3)

ホットトピック










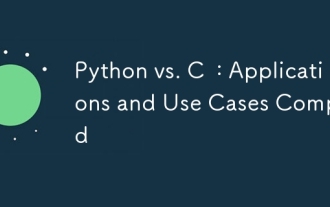
Pythonは、データサイエンス、Web開発、自動化タスクに適していますが、Cはシステムプログラミング、ゲーム開発、組み込みシステムに適しています。 Pythonは、そのシンプルさと強力なエコシステムで知られていますが、Cは高性能および基礎となる制御機能で知られています。
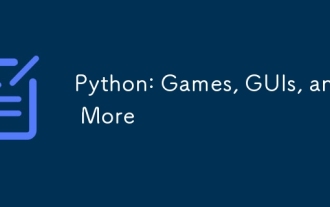
PythonはゲームとGUI開発に優れています。 1)ゲーム開発は、2Dゲームの作成に適した図面、オーディオ、その他の機能を提供し、Pygameを使用します。 2)GUI開発は、TKINTERまたはPYQTを選択できます。 TKINTERはシンプルで使いやすく、PYQTは豊富な機能を備えており、専門能力開発に適しています。
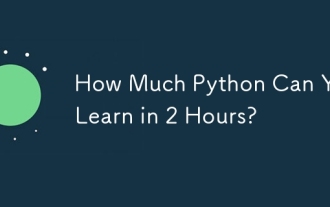
2時間以内にPythonの基本を学ぶことができます。 1。変数とデータ型を学習します。2。ステートメントやループの場合などのマスター制御構造、3。関数の定義と使用を理解します。これらは、簡単なPythonプログラムの作成を開始するのに役立ちます。
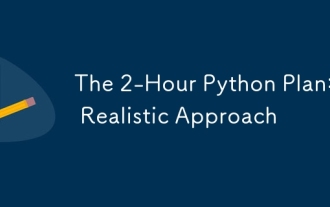
2時間以内にPythonの基本的なプログラミングの概念とスキルを学ぶことができます。 1.変数とデータ型、2。マスターコントロールフロー(条件付きステートメントとループ)、3。機能の定義と使用を理解する4。
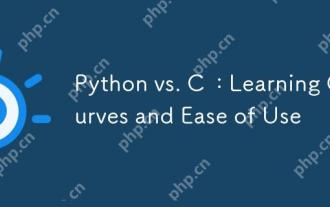
Pythonは学習と使用が簡単ですが、Cはより強力ですが複雑です。 1。Python構文は簡潔で初心者に適しています。動的なタイピングと自動メモリ管理により、使いやすくなりますが、ランタイムエラーを引き起こす可能性があります。 2.Cは、高性能アプリケーションに適した低レベルの制御と高度な機能を提供しますが、学習しきい値が高く、手動メモリとタイプの安全管理が必要です。
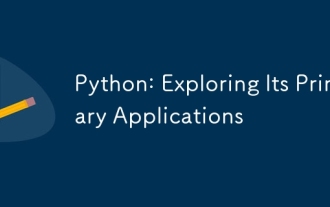
Pythonは、Web開発、データサイエンス、機械学習、自動化、スクリプトの分野で広く使用されています。 1)Web開発では、DjangoおよびFlask Frameworksが開発プロセスを簡素化します。 2)データサイエンスと機械学習の分野では、Numpy、Pandas、Scikit-Learn、Tensorflowライブラリが強力なサポートを提供します。 3)自動化とスクリプトの観点から、Pythonは自動テストやシステム管理などのタスクに適しています。
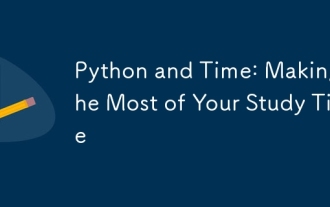
限られた時間でPythonの学習効率を最大化するには、PythonのDateTime、時間、およびスケジュールモジュールを使用できます。 1. DateTimeモジュールは、学習時間を記録および計画するために使用されます。 2。時間モジュールは、勉強と休息の時間を設定するのに役立ちます。 3.スケジュールモジュールは、毎週の学習タスクを自動的に配置します。
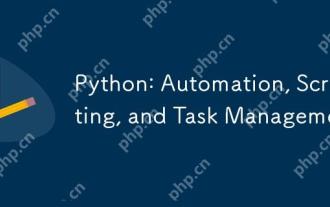
Pythonは、自動化、スクリプト、およびタスク管理に優れています。 1)自動化:OSやShutilなどの標準ライブラリを介してファイルバックアップが実現されます。 2)スクリプトの書き込み:Psutilライブラリを使用してシステムリソースを監視します。 3)タスク管理:スケジュールライブラリを使用してタスクをスケジュールします。 Pythonの使いやすさと豊富なライブラリサポートにより、これらの分野で優先ツールになります。
