Python ボットを構築してソーシャル メディア エンゲージメントを自動化する
著者: Trix Cyrus
ウェイマップ侵入テストツール: ここをクリック
TrixSec Github: ここをクリック
完全なマルチソーシャルメディアボットスクリプト
このスクリプトは、Twitter、Facebook、Instagram でのエンゲージメントを自動化するための基本構造を作成する方法を示します。 Facebook と Instagram の場合、リクエスト ライブラリを使用して API を呼び出す必要があります。
注: Facebook と Instagram には自動化に関する厳格なルールがあり、特定のアクションについては承認プロセスを経る必要がある場合があります。
import tweepy import requests import schedule import time # Twitter API credentials twitter_api_key = 'YOUR_TWITTER_API_KEY' twitter_api_secret_key = 'YOUR_TWITTER_API_SECRET_KEY' twitter_access_token = 'YOUR_TWITTER_ACCESS_TOKEN' twitter_access_token_secret = 'YOUR_TWITTER_ACCESS_TOKEN_SECRET' # Facebook API credentials facebook_access_token = 'YOUR_FACEBOOK_ACCESS_TOKEN' facebook_page_id = 'YOUR_FACEBOOK_PAGE_ID' # Instagram API credentials (using Graph API) instagram_access_token = 'YOUR_INSTAGRAM_ACCESS_TOKEN' instagram_business_account_id = 'YOUR_INSTAGRAM_BUSINESS_ACCOUNT_ID' # Authenticate to Twitter twitter_auth = tweepy.OAuth1UserHandler(twitter_api_key, twitter_api_secret_key, twitter_access_token, twitter_access_token_secret) twitter_api = tweepy.API(twitter_auth) # Function to post a tweet def post_tweet(status): try: twitter_api.update_status(status) print("Tweet posted successfully!") except Exception as e: print(f"An error occurred while posting tweet: {e}") # Function to like tweets based on a keyword def like_tweets(keyword, count=5): try: tweets = twitter_api.search(q=keyword, count=count) for tweet in tweets: twitter_api.create_favorite(tweet.id) print(f"Liked tweet by @{tweet.user.screen_name}: {tweet.text}") except Exception as e: print(f"An error occurred while liking tweets: {e}") # Function to post a Facebook update def post_facebook_update(message): try: url = f"https://graph.facebook.com/{facebook_page_id}/feed" payload = { 'message': message, 'access_token': facebook_access_token } response = requests.post(url, data=payload) if response.status_code == 200: print("Facebook post created successfully!") else: print(f"Failed to post on Facebook: {response.text}") except Exception as e: print(f"An error occurred while posting on Facebook: {e}") # Function to post an Instagram update (a photo in this example) def post_instagram_photo(image_url, caption): try: url = f"https://graph.facebook.com/v12.0/{instagram_business_account_id}/media" payload = { 'image_url': image_url, 'caption': caption, 'access_token': instagram_access_token } response = requests.post(url, data=payload) media_id = response.json().get('id') # Publish the media if media_id: publish_url = f"https://graph.facebook.com/v12.0/{instagram_business_account_id}/media_publish" publish_payload = { 'creation_id': media_id, 'access_token': instagram_access_token } publish_response = requests.post(publish_url, data=publish_payload) if publish_response.status_code == 200: print("Instagram post created successfully!") else: print(f"Failed to publish Instagram post: {publish_response.text}") else: print(f"Failed to create Instagram media: {response.text}") except Exception as e: print(f"An error occurred while posting on Instagram: {e}") # Function to perform all actions def run_bot(): # Customize your status and keywords post_tweet("Automated tweet from my Python bot!") like_tweets("Python programming", 5) post_facebook_update("Automated update on Facebook!") post_instagram_photo("YOUR_IMAGE_URL", "Automated Instagram post!") # Schedule the bot to run every hour schedule.every().hour.do(run_bot) print("Multi-social media bot is running...") # Keep the script running while True: schedule.run_pending() time.sleep(1)
このスクリプトの使用方法
必要なライブラリをインストールします: tweepy と request がインストールされていることを確認してください。 pip:
を使用してインストールできます。
pip install tweepy requests schedule
API 認証情報の設定: プレースホルダーを Twitter、Facebook、Instagram の実際の API 認証情報に置き換えます。
アクションをカスタマイズする: post_tweet と post_facebook_update のテキストを変更し、post_instagram_photo の YOUR_IMAGE_URL を投稿する有効な画像 URL に置き換えることができます。
スクリプトの実行: 次のコマンドを実行してスクリプトを実行します:
python your_script_name.py
ボットを監視する: ボットは無期限に実行され、指定されたアクションを 1 時間ごとに実行します。プロセスを中断することで停止できます (Ctrl C など)。
重要な考慮事項
API の制限: 各ソーシャル メディア プラットフォームには独自のレート制限と制限があるため、各 API のドキュメントを必ず確認してください。
倫理的使用: スパム送信やプラットフォーム ガイドラインへの違反を避けるために、ボットがユーザーとどのようにやり取りするかに注意してください。
テスト: ボットを広く展開する前に、制御された環境でボットをテストします。
~TrixSec
以上がPython ボットを構築してソーシャル メディア エンゲージメントを自動化するの詳細内容です。詳細については、PHP 中国語 Web サイトの他の関連記事を参照してください。

ホットAIツール

Undresser.AI Undress
リアルなヌード写真を作成する AI 搭載アプリ

AI Clothes Remover
写真から衣服を削除するオンライン AI ツール。

Undress AI Tool
脱衣画像を無料で

Clothoff.io
AI衣類リムーバー

Video Face Swap
完全無料の AI 顔交換ツールを使用して、あらゆるビデオの顔を簡単に交換できます。

人気の記事

ホットツール

メモ帳++7.3.1
使いやすく無料のコードエディター

SublimeText3 中国語版
中国語版、とても使いやすい

ゼンドスタジオ 13.0.1
強力な PHP 統合開発環境

ドリームウィーバー CS6
ビジュアル Web 開発ツール

SublimeText3 Mac版
神レベルのコード編集ソフト(SublimeText3)

ホットトピック










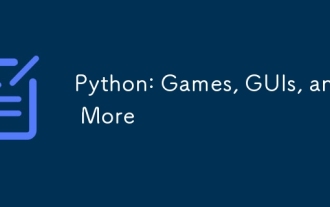
PythonはゲームとGUI開発に優れています。 1)ゲーム開発は、2Dゲームの作成に適した図面、オーディオ、その他の機能を提供し、Pygameを使用します。 2)GUI開発は、TKINTERまたはPYQTを選択できます。 TKINTERはシンプルで使いやすく、PYQTは豊富な機能を備えており、専門能力開発に適しています。
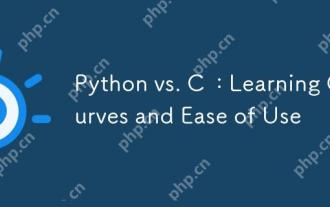
Pythonは学習と使用が簡単ですが、Cはより強力ですが複雑です。 1。Python構文は簡潔で初心者に適しています。動的なタイピングと自動メモリ管理により、使いやすくなりますが、ランタイムエラーを引き起こす可能性があります。 2.Cは、高性能アプリケーションに適した低レベルの制御と高度な機能を提供しますが、学習しきい値が高く、手動メモリとタイプの安全管理が必要です。
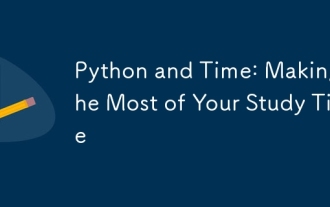
限られた時間でPythonの学習効率を最大化するには、PythonのDateTime、時間、およびスケジュールモジュールを使用できます。 1. DateTimeモジュールは、学習時間を記録および計画するために使用されます。 2。時間モジュールは、勉強と休息の時間を設定するのに役立ちます。 3.スケジュールモジュールは、毎週の学習タスクを自動的に配置します。
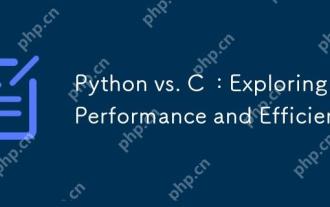
Pythonは開発効率でCよりも優れていますが、Cは実行パフォーマンスが高くなっています。 1。Pythonの簡潔な構文とリッチライブラリは、開発効率を向上させます。 2.Cのコンピレーションタイプの特性とハードウェア制御により、実行パフォーマンスが向上します。選択を行うときは、プロジェクトのニーズに基づいて開発速度と実行効率を比較検討する必要があります。
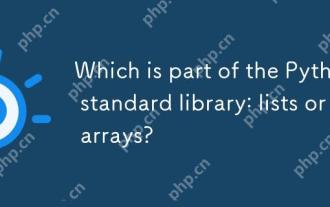
PythonListSarePartOfThestAndardarenot.liestareBuilting-in、versatile、forStoringCollectionsのpythonlistarepart。
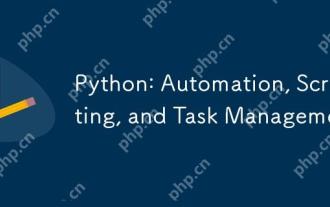
Pythonは、自動化、スクリプト、およびタスク管理に優れています。 1)自動化:OSやShutilなどの標準ライブラリを介してファイルバックアップが実現されます。 2)スクリプトの書き込み:Psutilライブラリを使用してシステムリソースを監視します。 3)タスク管理:スケジュールライブラリを使用してタスクをスケジュールします。 Pythonの使いやすさと豊富なライブラリサポートにより、これらの分野で優先ツールになります。
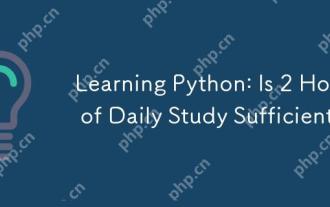
Pythonを1日2時間学ぶだけで十分ですか?それはあなたの目標と学習方法に依存します。 1)明確な学習計画を策定し、2)適切な学習リソースと方法を選択します。3)実践的な実践とレビューとレビューと統合を練習および統合し、統合すると、この期間中にPythonの基本的な知識と高度な機能を徐々に習得できます。
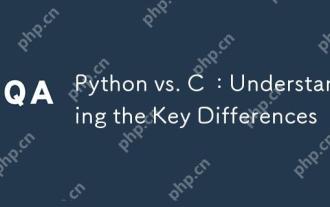
PythonとCにはそれぞれ独自の利点があり、選択はプロジェクトの要件に基づいている必要があります。 1)Pythonは、簡潔な構文と動的タイピングのため、迅速な開発とデータ処理に適しています。 2)Cは、静的なタイピングと手動メモリ管理により、高性能およびシステムプログラミングに適しています。
