Javaでファイルを逆の順序で読み取るにはどうすればよいですか?
Java でファイルを逆の順序で読み取る
問題:
ファイルを最後の行から始めて逆の順序で読み取る必要があります。
解決策:
ランダム アクセス ファイルとライン スキャンの使用
BufferedReader を使用してファイルを逆順に読み取るには、次のことができます。 RandomAccessFile を利用してファイル ポインタを操作し、逆の順序で改行をスキャンします。
Java でこのアプローチを実装する方法は次のとおりです:
<code class="java">import java.io.File; import java.io.IOException; import java.util.ArrayList; import java.util.Collections; public class ReverseFileReader { public static void readAndReverse(String filePath) { try { // Create a RandomAccessFile instance for the file RandomAccessFile file = new RandomAccessFile(filePath, "r"); // Calculate the file length long fileLength = file.length(); // Create an ArrayList to store the lines of the file ArrayList<String> lines = new ArrayList<>(); // Set the file pointer to the end of the file file.seek(fileLength); // Scan backwards line by line, starting from the last line while (file.getFilePointer() > 0) { // Find the previous line break and move the file pointer to that position long lineStart = file.getFilePointer(); while (file.getFilePointer() > 0 && file.readByte() != '\n') { file.seek(file.getFilePointer() - 1); } // Read the line and add it to the list file.seek(lineStart); lines.add(file.readLine()); } // Close the file file.close(); // Reverse the order of the lines in the list Collections.reverse(lines); // Print the lines in reverse order for (String line : lines) { System.out.println(line); } } catch (IOException e) { e.printStackTrace(); } } public static void main(String[] args) { // Specify the file path to be read in reverse order String filePath = "/path/to/your/file.txt"; // Read and reverse the file readAndReverse(filePath); } }</code>
実装の詳細:
- RandomAccessFile を使用してファイル ポインタを動的に移動し、ファイルを逆の順序でスキャンします。
- 行を ArrayList に保存して元の順序を保持し、印刷する前にリストを逆にします。
- readLine() メソッドは、ファイル ポインターの位置から行を逆に読み取るために使用されます。
- 逆の順序で改行をスキャンすることで、ファイルを最後から最初まで効果的に読み取ります。
以上がJavaでファイルを逆の順序で読み取るにはどうすればよいですか?の詳細内容です。詳細については、PHP 中国語 Web サイトの他の関連記事を参照してください。

ホットAIツール

Undresser.AI Undress
リアルなヌード写真を作成する AI 搭載アプリ

AI Clothes Remover
写真から衣服を削除するオンライン AI ツール。

Undress AI Tool
脱衣画像を無料で

Clothoff.io
AI衣類リムーバー

Video Face Swap
完全無料の AI 顔交換ツールを使用して、あらゆるビデオの顔を簡単に交換できます。

人気の記事

ホットツール

メモ帳++7.3.1
使いやすく無料のコードエディター

SublimeText3 中国語版
中国語版、とても使いやすい

ゼンドスタジオ 13.0.1
強力な PHP 統合開発環境

ドリームウィーバー CS6
ビジュアル Web 開発ツール

SublimeText3 Mac版
神レベルのコード編集ソフト(SublimeText3)

ホットトピック










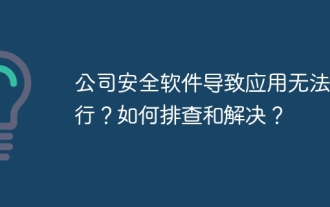
一部のアプリケーションが適切に機能しないようにする会社のセキュリティソフトウェアのトラブルシューティングとソリューション。多くの企業は、内部ネットワークセキュリティを確保するためにセキュリティソフトウェアを展開します。 ...
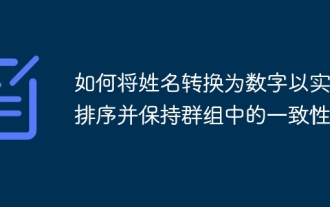
多くのアプリケーションシナリオでソートを実装するために名前を数値に変換するソリューションでは、ユーザーはグループ、特に1つでソートする必要がある場合があります...
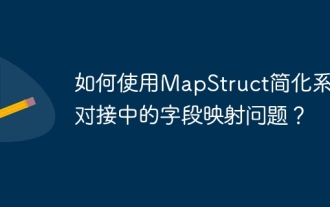
システムドッキングでのフィールドマッピング処理は、システムドッキングを実行する際に難しい問題に遭遇することがよくあります。システムのインターフェイスフィールドを効果的にマッピングする方法A ...
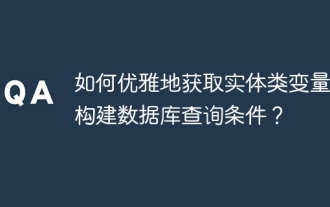
データベース操作にMyBatis-Plusまたはその他のORMフレームワークを使用する場合、エンティティクラスの属性名に基づいてクエリ条件を構築する必要があることがよくあります。あなたが毎回手動で...
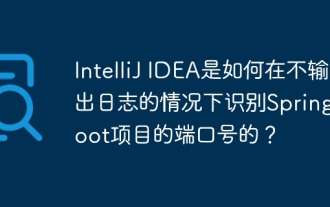
intellijideaultimatiateバージョンを使用してスプリングを開始します...
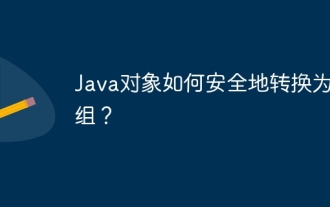
Javaオブジェクトと配列の変換:リスクの詳細な議論と鋳造タイプ変換の正しい方法多くのJava初心者は、オブジェクトのアレイへの変換に遭遇します...
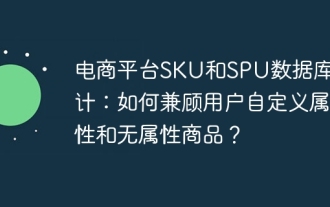
eコマースプラットフォーム上のSKUおよびSPUテーブルの設計の詳細な説明この記事では、eコマースプラットフォームでのSKUとSPUのデータベース設計の問題、特にユーザー定義の販売を扱う方法について説明します。
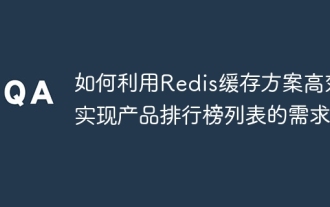
Redisキャッシュソリューションは、製品ランキングリストの要件をどのように実現しますか?開発プロセス中に、多くの場合、ランキングの要件に対処する必要があります。
