Qt で QWebPage を使用して複数の URL を効率的に取得するにはどうすればよいですか?
QWebPage を使用して複数の URL を取得する
このシナリオでは、Qt の QWebPage を使用して動的に更新されるページをレンダリングしようとしました。ただし、2 番目のページをレンダリングしようとすると、頻繁にクラッシュが発生しました。
問題分析
問題はアプローチにあります。 URL フェッチごとに新しい QApplication と QWebPage を初期化しています。代わりに、シグナルとカスタム処理を使用して同じインスタンス内の複数の URL を処理し、単一の QApplication と QWebPage を維持することをお勧めします。
提案されたソリューション
WebPage クラス
以下は PyQt5 と PyQt4 の両方のカスタム WebPage クラスです:
PyQt5 WebPage
<code class="python">from PyQt5.QtCore import pyqtSignal, QUrl from PyQt5.QtWidgets import QApplication from PyQt5.QtWebEngineWidgets import QWebEnginePage class WebPage(QWebEnginePage): htmlReady = pyqtSignal(str, str) def __init__(self, verbose=False): super().__init__() self._verbose = verbose self.loadFinished.connect(self.handleLoadFinished) def process(self, urls): self._urls = iter(urls) self.fetchNext() def fetchNext(self): try: url = next(self._urls) except StopIteration: return False else: self.load(QUrl(url)) return True def processCurrentPage(self, html): self.htmlReady.emit(html, self.url().toString()) if not self self.fetchNext(): QApplication.instance().quit() def handleLoadFinished(self): self.toHtml(self.processCurrentPage) def javaScriptConsoleMessage(self, *args, **kwargs): if self._verbose: super().javaScriptConsoleMessage(*args, **kwargs)</code>
PyQt4 WebPage
<code class="python">from PyQt4.QtCore import pyqtSignal, QUrl from PyQt4.QtGui import QApplication from PyQt4.QtWebKit import QWebPage class WebPage(QWebPage): htmlReady = pyqtSignal(str, str) def __init__(self, verbose=False): super(WebPage, self).__init__() self._verbose = verbose self.mainFrame().loadFinished.connect(self.handleLoadFinished) def process(self, urls): self._urls = iter(urls) self.fetchNext() def fetchNext(self): try: url = next(self._urls) except StopIteration: return False else: self.mainFram().load(QUrl(url)) return True def processCurrentPage(self): self.htmlReady.emit(self.mainFrame().toHtml(), self.mainFrame().url().toString()) if not self.fetchNext(): QApplication.instance().quit() def javaScripConsoleMessage(self ,* args, **kwargs): if self._verbose: super(WebPage, self).javaScriptConsoleMessage(*args, **kwargs)</code>
使用法
これらの WebPage クラスの使用方法の例を次に示します。
<code class="python">from PyQt5.QtCore import QUrl from PyQt5.QtWidgets import QApplication # PyQt5 url_list = ['https://example.com', 'https://example2.com'] app = QApplication(sys.argv) webpage = WebPage(verbose=True) webpage.htmlReady.connect(my_html_processor) webpage.process(url_list) sys.exit(app.exec_()) # PyQt4 from PyQt4.QtCore import QUrl from PyQt4.QtGui import QApplication url_list = ['https://example.com', 'https://example2.com'] app = QApplication(sys.argv) webpage = WebPage(verbose=True) webpage.htmlReady.connect(my_html_processor) webpage.process(url_list) sys.exit(app.exec_())</code>
このコードでは、my_html_processor は、処理された HTML を処理するようにカスタマイズできる関数であり、各ページの URL 情報。
このアプローチを実装すると、以前に発生したクラッシュやランダムな動作を防ぐことができ、より安定した効率的な Web スクレイピング ワークフローが実現します。
以上がQt で QWebPage を使用して複数の URL を効率的に取得するにはどうすればよいですか?の詳細内容です。詳細については、PHP 中国語 Web サイトの他の関連記事を参照してください。

ホットAIツール

Undresser.AI Undress
リアルなヌード写真を作成する AI 搭載アプリ

AI Clothes Remover
写真から衣服を削除するオンライン AI ツール。

Undress AI Tool
脱衣画像を無料で

Clothoff.io
AI衣類リムーバー

Video Face Swap
完全無料の AI 顔交換ツールを使用して、あらゆるビデオの顔を簡単に交換できます。

人気の記事

ホットツール

メモ帳++7.3.1
使いやすく無料のコードエディター

SublimeText3 中国語版
中国語版、とても使いやすい

ゼンドスタジオ 13.0.1
強力な PHP 統合開発環境

ドリームウィーバー CS6
ビジュアル Web 開発ツール

SublimeText3 Mac版
神レベルのコード編集ソフト(SublimeText3)

ホットトピック










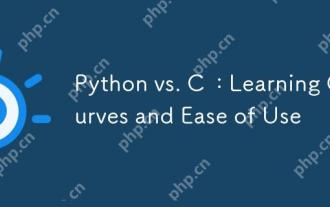
Pythonは学習と使用が簡単ですが、Cはより強力ですが複雑です。 1。Python構文は簡潔で初心者に適しています。動的なタイピングと自動メモリ管理により、使いやすくなりますが、ランタイムエラーを引き起こす可能性があります。 2.Cは、高性能アプリケーションに適した低レベルの制御と高度な機能を提供しますが、学習しきい値が高く、手動メモリとタイプの安全管理が必要です。
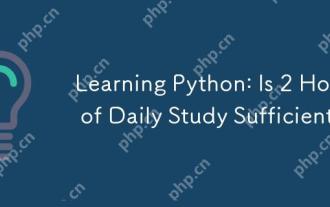
Pythonを1日2時間学ぶだけで十分ですか?それはあなたの目標と学習方法に依存します。 1)明確な学習計画を策定し、2)適切な学習リソースと方法を選択します。3)実践的な実践とレビューとレビューと統合を練習および統合し、統合すると、この期間中にPythonの基本的な知識と高度な機能を徐々に習得できます。
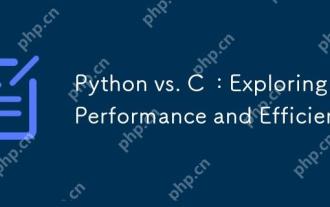
Pythonは開発効率でCよりも優れていますが、Cは実行パフォーマンスが高くなっています。 1。Pythonの簡潔な構文とリッチライブラリは、開発効率を向上させます。 2.Cのコンピレーションタイプの特性とハードウェア制御により、実行パフォーマンスが向上します。選択を行うときは、プロジェクトのニーズに基づいて開発速度と実行効率を比較検討する必要があります。
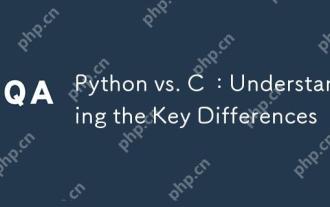
PythonとCにはそれぞれ独自の利点があり、選択はプロジェクトの要件に基づいている必要があります。 1)Pythonは、簡潔な構文と動的タイピングのため、迅速な開発とデータ処理に適しています。 2)Cは、静的なタイピングと手動メモリ管理により、高性能およびシステムプログラミングに適しています。
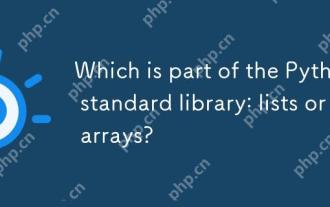
PythonListSarePartOfThestAndardarenot.liestareBuilting-in、versatile、forStoringCollectionsのpythonlistarepart。
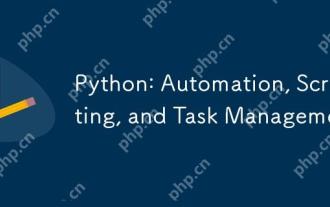
Pythonは、自動化、スクリプト、およびタスク管理に優れています。 1)自動化:OSやShutilなどの標準ライブラリを介してファイルバックアップが実現されます。 2)スクリプトの書き込み:Psutilライブラリを使用してシステムリソースを監視します。 3)タスク管理:スケジュールライブラリを使用してタスクをスケジュールします。 Pythonの使いやすさと豊富なライブラリサポートにより、これらの分野で優先ツールになります。
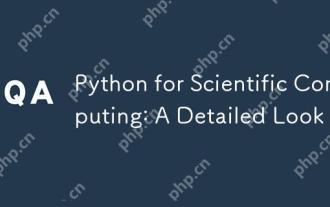
科学コンピューティングにおけるPythonのアプリケーションには、データ分析、機械学習、数値シミュレーション、視覚化が含まれます。 1.numpyは、効率的な多次元配列と数学的関数を提供します。 2。ScipyはNumpy機能を拡張し、最適化と線形代数ツールを提供します。 3. Pandasは、データ処理と分析に使用されます。 4.matplotlibは、さまざまなグラフと視覚的な結果を生成するために使用されます。
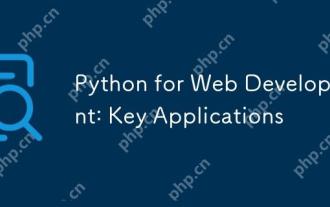
Web開発におけるPythonの主要なアプリケーションには、DjangoおよびFlaskフレームワークの使用、API開発、データ分析と視覚化、機械学習とAI、およびパフォーマンスの最適化が含まれます。 1。DjangoandFlask Framework:Djangoは、複雑な用途の迅速な発展に適しており、Flaskは小規模または高度にカスタマイズされたプロジェクトに適しています。 2。API開発:フラスコまたはdjangorestFrameworkを使用して、Restfulapiを構築します。 3。データ分析と視覚化:Pythonを使用してデータを処理し、Webインターフェイスを介して表示します。 4。機械学習とAI:Pythonは、インテリジェントWebアプリケーションを構築するために使用されます。 5。パフォーマンスの最適化:非同期プログラミング、キャッシュ、コードを通じて最適化
