Python を使用してデータから複数色の線分をプロットする方法
データからの複数色の線分のプロット
データ ポイントを線として視覚化するには、matplotlib を使用できます。ここには、それぞれ緯度と経度の座標を表す 2 つのリスト 'latt' と 'lont' があります。目的は、10 点の各セグメントに固有の色を割り当てて、データ ポイントを結ぶ線をプロットすることです。
アプローチ 1: 個別の線プロット
小規模の場合線分の数に応じて、セグメントごとにさまざまな色で個別の線プロットを作成できます。次のコード例は、このアプローチを示しています。
<code class="python">import numpy as np import matplotlib.pyplot as plt # Assume the list of latitude and longitude is provided # Generate uniqueish colors def uniqueish_color(): return plt.cm.gist_ncar(np.random.random()) # Create a plot fig, ax = plt.subplots() # Iterate through the data in segments of 10 for start, stop in zip(latt[:-1], latt[1:]): # Extract coordinates for each segment x = latt[start:stop] y = lont[start:stop] # Plot each segment with a unique color ax.plot(x, y, color=uniqueish_color()) # Display the plot plt.show()</code>
アプローチ 2: 大規模なデータセットのライン コレクション
膨大な数のライン セグメントを含む大規模なデータセットの場合は、Line を使用します。コレクションにより効率が向上します。以下に例を示します。
<code class="python">import numpy as np import matplotlib.pyplot as plt from matplotlib.collections import LineCollection # Prepare the data as a sequence of line segments segments = np.hstack([latt[:-1], latt[1:]]).reshape(-1, 1, 2) # Create a plot fig, ax = plt.subplots() # Create a LineCollection object coll = LineCollection(segments, cmap=plt.cm.gist_ncar) # Assign random colors to the segments coll.set_array(np.random.random(latt.shape[0])) # Add the LineCollection to the plot ax.add_collection(coll) ax.autoscale_view() # Display the plot plt.show()</code>
結論として、どちらのアプローチでも、データ ポイントのさまざまなセグメントに対してさまざまな色で効果的に線をプロットできます。どちらを選択するかは、プロットする線分の数によって異なります。
以上がPython を使用してデータから複数色の線分をプロットする方法の詳細内容です。詳細については、PHP 中国語 Web サイトの他の関連記事を参照してください。

ホットAIツール

Undresser.AI Undress
リアルなヌード写真を作成する AI 搭載アプリ

AI Clothes Remover
写真から衣服を削除するオンライン AI ツール。

Undress AI Tool
脱衣画像を無料で

Clothoff.io
AI衣類リムーバー

Video Face Swap
完全無料の AI 顔交換ツールを使用して、あらゆるビデオの顔を簡単に交換できます。

人気の記事

ホットツール

メモ帳++7.3.1
使いやすく無料のコードエディター

SublimeText3 中国語版
中国語版、とても使いやすい

ゼンドスタジオ 13.0.1
強力な PHP 統合開発環境

ドリームウィーバー CS6
ビジュアル Web 開発ツール

SublimeText3 Mac版
神レベルのコード編集ソフト(SublimeText3)

ホットトピック










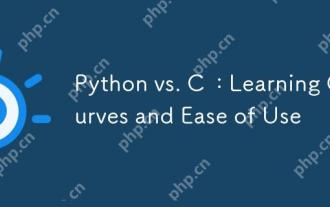
Pythonは学習と使用が簡単ですが、Cはより強力ですが複雑です。 1。Python構文は簡潔で初心者に適しています。動的なタイピングと自動メモリ管理により、使いやすくなりますが、ランタイムエラーを引き起こす可能性があります。 2.Cは、高性能アプリケーションに適した低レベルの制御と高度な機能を提供しますが、学習しきい値が高く、手動メモリとタイプの安全管理が必要です。
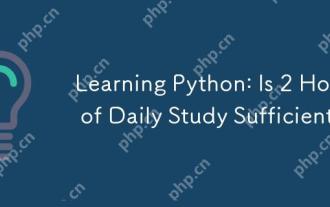
Pythonを1日2時間学ぶだけで十分ですか?それはあなたの目標と学習方法に依存します。 1)明確な学習計画を策定し、2)適切な学習リソースと方法を選択します。3)実践的な実践とレビューとレビューと統合を練習および統合し、統合すると、この期間中にPythonの基本的な知識と高度な機能を徐々に習得できます。
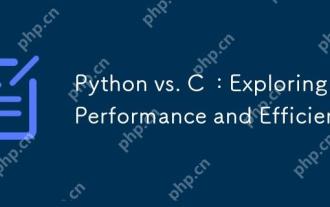
Pythonは開発効率でCよりも優れていますが、Cは実行パフォーマンスが高くなっています。 1。Pythonの簡潔な構文とリッチライブラリは、開発効率を向上させます。 2.Cのコンピレーションタイプの特性とハードウェア制御により、実行パフォーマンスが向上します。選択を行うときは、プロジェクトのニーズに基づいて開発速度と実行効率を比較検討する必要があります。
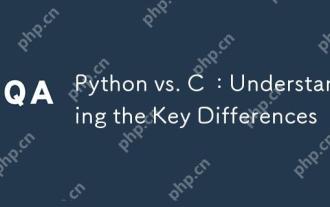
PythonとCにはそれぞれ独自の利点があり、選択はプロジェクトの要件に基づいている必要があります。 1)Pythonは、簡潔な構文と動的タイピングのため、迅速な開発とデータ処理に適しています。 2)Cは、静的なタイピングと手動メモリ管理により、高性能およびシステムプログラミングに適しています。
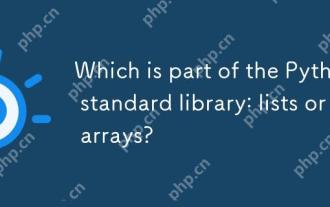
PythonListSarePartOfThestAndardarenot.liestareBuilting-in、versatile、forStoringCollectionsのpythonlistarepart。
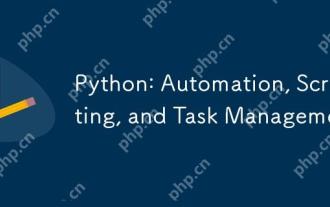
Pythonは、自動化、スクリプト、およびタスク管理に優れています。 1)自動化:OSやShutilなどの標準ライブラリを介してファイルバックアップが実現されます。 2)スクリプトの書き込み:Psutilライブラリを使用してシステムリソースを監視します。 3)タスク管理:スケジュールライブラリを使用してタスクをスケジュールします。 Pythonの使いやすさと豊富なライブラリサポートにより、これらの分野で優先ツールになります。
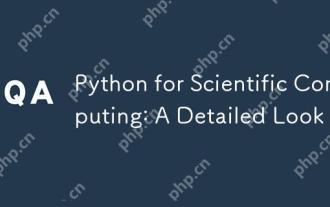
科学コンピューティングにおけるPythonのアプリケーションには、データ分析、機械学習、数値シミュレーション、視覚化が含まれます。 1.numpyは、効率的な多次元配列と数学的関数を提供します。 2。ScipyはNumpy機能を拡張し、最適化と線形代数ツールを提供します。 3. Pandasは、データ処理と分析に使用されます。 4.matplotlibは、さまざまなグラフと視覚的な結果を生成するために使用されます。
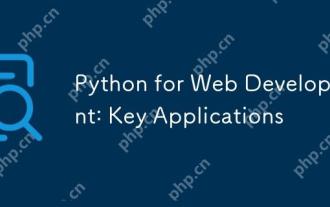
Web開発におけるPythonの主要なアプリケーションには、DjangoおよびFlaskフレームワークの使用、API開発、データ分析と視覚化、機械学習とAI、およびパフォーマンスの最適化が含まれます。 1。DjangoandFlask Framework:Djangoは、複雑な用途の迅速な発展に適しており、Flaskは小規模または高度にカスタマイズされたプロジェクトに適しています。 2。API開発:フラスコまたはdjangorestFrameworkを使用して、Restfulapiを構築します。 3。データ分析と視覚化:Pythonを使用してデータを処理し、Webインターフェイスを介して表示します。 4。機械学習とAI:Pythonは、インテリジェントWebアプリケーションを構築するために使用されます。 5。パフォーマンスの最適化:非同期プログラミング、キャッシュ、コードを通じて最適化
