Python Selenium で複数の要素を確実にロードするにはどうすればよいですか?
Python Selenium: 複数の要素のロードの確認
AJAX 経由で動的にロードされた要素を扱う場合、その外観を確認するのが難しい場合があります。このシナリオを処理するには、Selenium の WebDriverWait とそのさまざまな戦略を利用して、複数の要素の存在を確認します。
すべての要素の可視性:
の可視性を確認するには特定のセレクターに一致するすべての要素については、visibility_of_all_elements_located() 条件を使用できます:
<code class="python">from selenium.webdriver.support.ui import WebDriverWait from selenium.webdriver.common.by import By from selenium.webdriver.support import expected_conditions as EC elements = WebDriverWait(driver, 20).until( EC.visibility_of_all_elements_located( (By.CSS_SELECTOR, "ul.ltr li[id^='t_b_'] > a[id^='t_a_'][href]") ) )</code>
特定の数の要素を待機:
待機する必要がある場合特定の数の要素をロードするには、ExpectedConditions クラスでラムダ関数を使用できます:
<code class="python">WebDriverWait(driver, 20).until( lambda driver: len(driver.find_elements_by_xpath(selector)) > int(myLength) )</code>
子要素の XPath:
子を待機するにはDOM 全体を検索するのではなく、親要素内の要素を検索するには、XPath 式を利用できます:
<code class="python">WebDriverWait(driver, 20).until( EC.presence_of_element_located( (By.XPATH, "//ul[@class='ltr']//li[starts-with(@id, 't_b_')]/a[starts-with(@id, 't_a_')]") ) )</code>
カスタム待機条件:
独自の待機条件を作成したい場合待機状態を回避するには、webdriver.support.ui.ExpectedCondition:
<code class="python">class MoreThanOne(webdriver.support.ui.ExpectedCondition): def __init__(self, selector): self.selector = selector def __call__(self, driver): elements = driver.find_elements_by_css_selector(self.selector) return len(elements) > 1</code>
<code class="python">WebDriverWait(driver, 30).until(MoreThanOne('li'))</code>
DOM での要素損失の防止:
のサブクラスを定義できます。検索操作後に現在の要素を失った場合は、待機を実行する前に変数に保存します。
<code class="python">element = driver.find_element_by_css_selector('ul') WebDriverWait(element, 30).until(MoreThanOne('li'))</code>
結論:
これらの手法は、柔軟で堅牢な方法を提供します。 Selenium で複数の要素がロードされるのを待ちます。要件に応じて、ユースケースに最も適したアプローチを選択してください。
以上がPython Selenium で複数の要素を確実にロードするにはどうすればよいですか?の詳細内容です。詳細については、PHP 中国語 Web サイトの他の関連記事を参照してください。

ホットAIツール

Undresser.AI Undress
リアルなヌード写真を作成する AI 搭載アプリ

AI Clothes Remover
写真から衣服を削除するオンライン AI ツール。

Undress AI Tool
脱衣画像を無料で

Clothoff.io
AI衣類リムーバー

Video Face Swap
完全無料の AI 顔交換ツールを使用して、あらゆるビデオの顔を簡単に交換できます。

人気の記事

ホットツール

メモ帳++7.3.1
使いやすく無料のコードエディター

SublimeText3 中国語版
中国語版、とても使いやすい

ゼンドスタジオ 13.0.1
強力な PHP 統合開発環境

ドリームウィーバー CS6
ビジュアル Web 開発ツール

SublimeText3 Mac版
神レベルのコード編集ソフト(SublimeText3)

ホットトピック










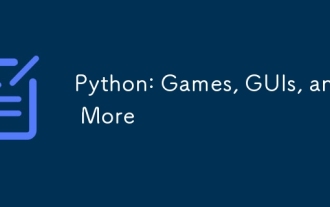
PythonはゲームとGUI開発に優れています。 1)ゲーム開発は、2Dゲームの作成に適した図面、オーディオ、その他の機能を提供し、Pygameを使用します。 2)GUI開発は、TKINTERまたはPYQTを選択できます。 TKINTERはシンプルで使いやすく、PYQTは豊富な機能を備えており、専門能力開発に適しています。
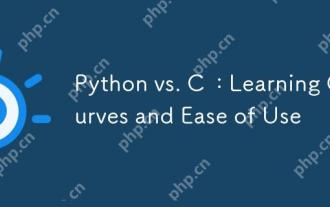
Pythonは学習と使用が簡単ですが、Cはより強力ですが複雑です。 1。Python構文は簡潔で初心者に適しています。動的なタイピングと自動メモリ管理により、使いやすくなりますが、ランタイムエラーを引き起こす可能性があります。 2.Cは、高性能アプリケーションに適した低レベルの制御と高度な機能を提供しますが、学習しきい値が高く、手動メモリとタイプの安全管理が必要です。
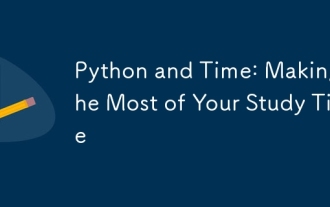
限られた時間でPythonの学習効率を最大化するには、PythonのDateTime、時間、およびスケジュールモジュールを使用できます。 1. DateTimeモジュールは、学習時間を記録および計画するために使用されます。 2。時間モジュールは、勉強と休息の時間を設定するのに役立ちます。 3.スケジュールモジュールは、毎週の学習タスクを自動的に配置します。
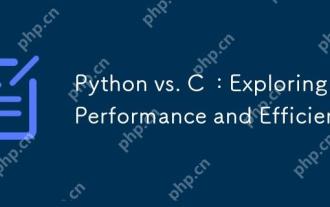
Pythonは開発効率でCよりも優れていますが、Cは実行パフォーマンスが高くなっています。 1。Pythonの簡潔な構文とリッチライブラリは、開発効率を向上させます。 2.Cのコンピレーションタイプの特性とハードウェア制御により、実行パフォーマンスが向上します。選択を行うときは、プロジェクトのニーズに基づいて開発速度と実行効率を比較検討する必要があります。
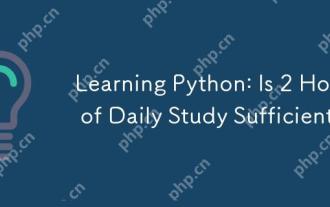
Pythonを1日2時間学ぶだけで十分ですか?それはあなたの目標と学習方法に依存します。 1)明確な学習計画を策定し、2)適切な学習リソースと方法を選択します。3)実践的な実践とレビューとレビューと統合を練習および統合し、統合すると、この期間中にPythonの基本的な知識と高度な機能を徐々に習得できます。
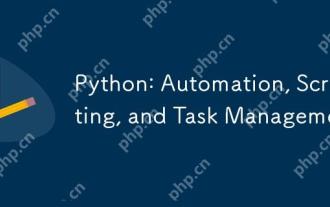
Pythonは、自動化、スクリプト、およびタスク管理に優れています。 1)自動化:OSやShutilなどの標準ライブラリを介してファイルバックアップが実現されます。 2)スクリプトの書き込み:Psutilライブラリを使用してシステムリソースを監視します。 3)タスク管理:スケジュールライブラリを使用してタスクをスケジュールします。 Pythonの使いやすさと豊富なライブラリサポートにより、これらの分野で優先ツールになります。
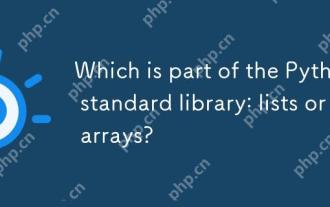
PythonListSarePartOfThestAndardarenot.liestareBuilting-in、versatile、forStoringCollectionsのpythonlistarepart。
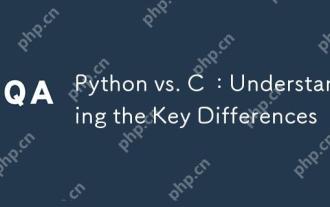
PythonとCにはそれぞれ独自の利点があり、選択はプロジェクトの要件に基づいている必要があります。 1)Pythonは、簡潔な構文と動的タイピングのため、迅速な開発とデータ処理に適しています。 2)Cは、静的なタイピングと手動メモリ管理により、高性能およびシステムプログラミングに適しています。
