


32 ビット コンパイラと 64 ビット コンパイラで「int64_t」、「long int」、および「long long int」の動作が異なるのはなぜですか?
C でのlong long int 対 long int 対 int64_t
次の C プログラムを考えてみましょう。
<code class="cpp">#include <iostream> #include <cstdint> template <typename T> bool is_int64() { return false; } template <> bool is_int64<int64_t>() { return true; } int main() { std::cout << "int:\t" << is_int64<int>() << std::endl; std::cout << "int64_t:\t" << is_int64<int64_t>() << std::endl; std::cout << "long int:\t" << is_int64<long int>() << std::endl; std::cout << "long long int:\t" << is_int64<long long int>() << std::endl; return 0; }</code>
32 ビット GCC コンパイルと 32/64 ビット MSVC コンパイルの両方で、プログラムの出力は次のようになります:
int: 0 int64_t: 1 long int: 0 long long int: 1
ただし、64 ビット GCC コンパイルでは、出力は次のように変わります:
int: 0 int64_t: 1 long int: 1 long long int: 0
この現象は、64 ビット コンパイルでは int64_t がlong long int ではなくlong int として定義されているために発生します。これを修正するには、次のようにプラットフォーム固有のチェックを使用します。
<code class="cpp">#if defined(__GNUC__) && (__WORDSIZE == 64) template <> bool is_int64<long long int>() { return true; } #endif</code>
ただし、この解決策は特定のコンパイラとプラットフォームの組み合わせに依存するため、理想的ではありません。より信頼性の高い方法は、型の等価性をコンパイラに明示的に指定することです。残念ながら、C には、基本データ型間のそのような等価性を定義する方法がありません。代わりに、std::is_same などの型の特性に依存できます。例:
<code class="cpp">// C++11 template <typename T> void same_type(T, T) {} void foo() { long int x; long long int y; same_type(x, y); // Will now compile successfully }</code>
この例では、基礎となる表現に関係なく、x と y が同じ型であるかどうかを判断するために std::is_same が使用されます。
以上が32 ビット コンパイラと 64 ビット コンパイラで「int64_t」、「long int」、および「long long int」の動作が異なるのはなぜですか?の詳細内容です。詳細については、PHP 中国語 Web サイトの他の関連記事を参照してください。

ホットAIツール

Undresser.AI Undress
リアルなヌード写真を作成する AI 搭載アプリ

AI Clothes Remover
写真から衣服を削除するオンライン AI ツール。

Undress AI Tool
脱衣画像を無料で

Clothoff.io
AI衣類リムーバー

Video Face Swap
完全無料の AI 顔交換ツールを使用して、あらゆるビデオの顔を簡単に交換できます。

人気の記事

ホットツール

メモ帳++7.3.1
使いやすく無料のコードエディター

SublimeText3 中国語版
中国語版、とても使いやすい

ゼンドスタジオ 13.0.1
強力な PHP 統合開発環境

ドリームウィーバー CS6
ビジュアル Web 開発ツール

SublimeText3 Mac版
神レベルのコード編集ソフト(SublimeText3)

ホットトピック










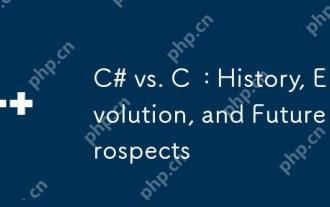
C#とCの歴史と進化はユニークであり、将来の見通しも異なります。 1.Cは、1983年にBjarnestrostrupによって発明され、オブジェクト指向のプログラミングをC言語に導入しました。その進化プロセスには、C 11の自動キーワードとラムダ式の導入など、複数の標準化が含まれます。C20概念とコルーチンの導入、将来のパフォーマンスとシステムレベルのプログラミングに焦点を当てます。 2.C#は2000年にMicrosoftによってリリースされました。CとJavaの利点を組み合わせて、その進化はシンプルさと生産性に焦点を当てています。たとえば、C#2.0はジェネリックを導入し、C#5.0は非同期プログラミングを導入しました。これは、将来の開発者の生産性とクラウドコンピューティングに焦点を当てます。
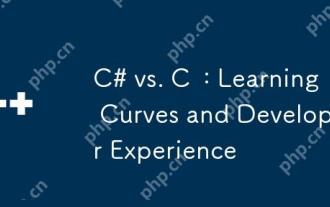
C#とCおよび開発者の経験の学習曲線には大きな違いがあります。 1)C#の学習曲線は比較的フラットであり、迅速な開発およびエンタープライズレベルのアプリケーションに適しています。 2)Cの学習曲線は急勾配であり、高性能および低レベルの制御シナリオに適しています。
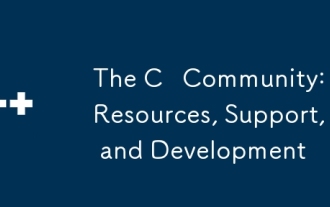
C学習者と開発者は、Stackoverflow、RedditのR/CPPコミュニティ、CourseraおよびEDXコース、Github、Professional Consulting Services、およびCPPCONのオープンソースプロジェクトからリソースとサポートを得ることができます。 1. StackOverFlowは、技術的な質問への回答を提供します。 2。RedditのR/CPPコミュニティが最新ニュースを共有しています。 3。CourseraとEDXは、正式なCコースを提供します。 4. LLVMなどのGitHubでのオープンソースプロジェクトやスキルの向上。 5。JetBrainやPerforceなどの専門的なコンサルティングサービスは、技術サポートを提供します。 6。CPPCONとその他の会議はキャリアを助けます
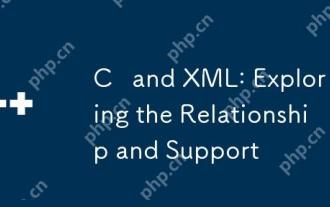
Cは、サードパーティライブラリ(TinyXML、PUGIXML、XERCES-Cなど)を介してXMLと相互作用します。 1)ライブラリを使用してXMLファイルを解析し、それらをC処理可能なデータ構造に変換します。 2)XMLを生成するときは、Cデータ構造をXML形式に変換します。 3)実際のアプリケーションでは、XMLが構成ファイルとデータ交換に使用されることがよくあり、開発効率を向上させます。
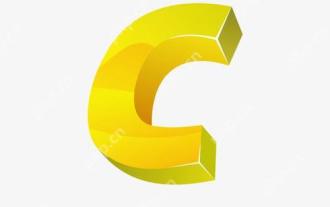
Cでの静的分析の適用には、主にメモリ管理の問題の発見、コードロジックエラーの確認、およびコードセキュリティの改善が含まれます。 1)静的分析では、メモリリーク、ダブルリリース、非初期化ポインターなどの問題を特定できます。 2)未使用の変数、死んだコード、論理的矛盾を検出できます。 3)カバー性などの静的分析ツールは、バッファーオーバーフロー、整数のオーバーフロー、安全でないAPI呼び出しを検出して、コードセキュリティを改善します。
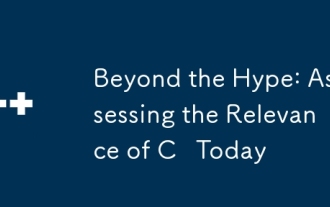
Cは、現代のプログラミングにおいて依然として重要な関連性を持っています。 1)高性能および直接的なハードウェア操作機能により、ゲーム開発、組み込みシステム、高性能コンピューティングの分野で最初の選択肢になります。 2)豊富なプログラミングパラダイムとスマートポインターやテンプレートプログラミングなどの最新の機能は、その柔軟性と効率を向上させます。学習曲線は急ですが、その強力な機能により、今日のプログラミングエコシステムでは依然として重要です。
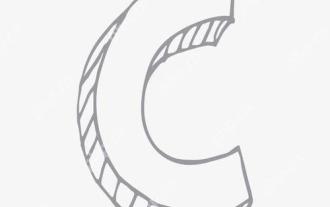
CでChronoライブラリを使用すると、時間と時間の間隔をより正確に制御できます。このライブラリの魅力を探りましょう。 CのChronoライブラリは、時間と時間の間隔に対処するための最新の方法を提供する標準ライブラリの一部です。 Time.HとCtimeに苦しんでいるプログラマーにとって、Chronoは間違いなく恩恵です。コードの読みやすさと保守性を向上させるだけでなく、より高い精度と柔軟性も提供します。基本から始めましょう。 Chronoライブラリには、主に次の重要なコンポーネントが含まれています。STD:: Chrono :: System_Clock:現在の時間を取得するために使用されるシステムクロックを表します。 STD :: Chron
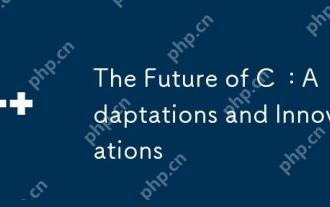
Cの将来は、並列コンピューティング、セキュリティ、モジュール化、AI/機械学習に焦点を当てます。1)並列コンピューティングは、コルーチンなどの機能を介して強化されます。 2)セキュリティは、より厳格なタイプのチェックとメモリ管理メカニズムを通じて改善されます。 3)変調は、コード組織とコンパイルを簡素化します。 4)AIと機械学習は、数値コンピューティングやGPUプログラミングサポートなど、CにComply Coveに適応するように促します。
