goroutine を利用しながら URL のリストを処理するときに、同時実行する Go ルーチンの数を制限するにはどうすればよいでしょうか?
Oct 31, 2024 am 11:31 AM同時 Go ルーチン数の制限
問題:
URL のリストを処理しようとしていますゴルーチンを同時に使用しますが、同時に実行されるゴルーチンの最大数は事前に定義されています。この例では、URL が 30 個あるにもかかわらず、並列化を同時に 10 個のゴルーチンに制限したいとします。
解決策:
この問題を解決する鍵は、コードのアーキテクチャ。 URL ごとに個別の goroutine を生成する代わりに、共有チャネルから URL を消費する限られた数のワーカー goroutine を生成します。このチャネルのバッファリングされた性質により同時実行性が調整されます。
コードの変更:
このアプローチを組み込んだコードの更新バージョンは次のとおりです:
<code class="go">package main import ( "flag" "fmt" "os" "sync" "time" ) func main() { parallel := flag.Int("parallel", 10, "max parallel requests allowed") flag.Parse() urls := flag.Args() // Create a buffered channel to buffer URLs urlsChan := make(chan string, *parallel) // Create a separate goroutine to feed URLs into the channel go func() { for _, u := range urls { urlsChan <- u } // Close the channel to indicate that there are no more URLs to process close(urlsChan) }() var wg sync.WaitGroup client := rest.Client{} results := make(chan string) // Start the specified number of worker goroutines for i := 0; i < *parallel; i++ { wg.Add(1) go func() { defer wg.Done() // Continuously retrieve URLs from the channel until it is closed for url := range urlsChan { worker(url, client, results) } }() } // Launch a separate goroutine to close the results channel when all workers are finished go func() { // Wait for all workers to finish processing URLs wg.Wait() // Close the results channel to signal that there are no more results close(results) }() // Read results from the channel until it is closed for res := range results { fmt.Println(res) } os.Exit(0) }</code>
この改訂されたコードでは、
- 処理対象の URL を保持するためにバッファリングされたチャネル urlsChan が作成されます。バッファ サイズは *Parallel に設定され、チャネルに同時にアクセスできるゴルーチンの数を効果的に制限します。
- 別のゴルーチンは、urlsChan チャネルに URL を設定する専用です。
- ワーカー ゴルーチンurlsChan チャネルが閉じられるまで継続的に URL を消費します。
- すべてのワーカーがタスクを完了すると、結果チャネルを閉じるために別の goroutine が使用されます。
この変更されたアーキテクチャを活用することにより、を使用すると、指定した並列処理制限に基づいて、同時に実行するゴルーチンの数を効果的に調整できます。
以上がgoroutine を利用しながら URL のリストを処理するときに、同時実行する Go ルーチンの数を制限するにはどうすればよいでしょうか?の詳細内容です。詳細については、PHP 中国語 Web サイトの他の関連記事を参照してください。

人気の記事

人気の記事

ホットな記事タグ

メモ帳++7.3.1
使いやすく無料のコードエディター

SublimeText3 中国語版
中国語版、とても使いやすい

ゼンドスタジオ 13.0.1
強力な PHP 統合開発環境

ドリームウィーバー CS6
ビジュアル Web 開発ツール

SublimeText3 Mac版
神レベルのコード編集ソフト(SublimeText3)

ホットトピック










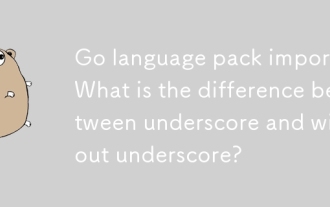
Go Language Packのインポート:アンダースコアとアンダースコアなしの違いは何ですか?
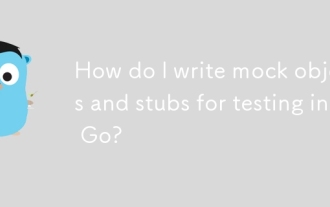
GOでテスト用のモックオブジェクトとスタブを書くにはどうすればよいですか?
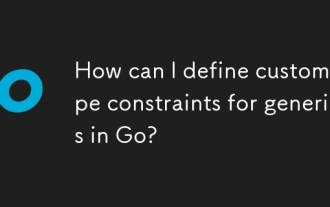
GOのジェネリックのカスタムタイプ制約を定義するにはどうすればよいですか?
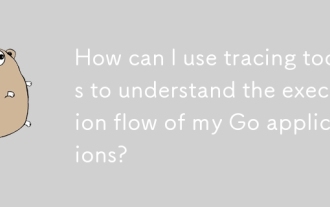
トレースツールを使用して、GOアプリケーションの実行フローを理解するにはどうすればよいですか?
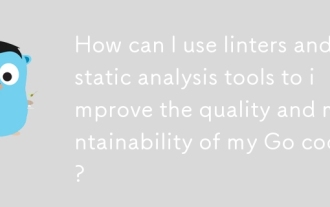
Linterと静的分析ツールを使用して、GOコードの品質と保守性を向上させるにはどうすればよいですか?
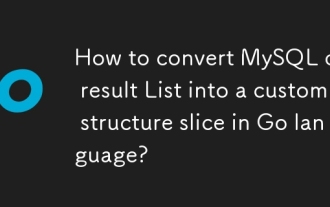
MySQLクエリ結果リストをGO言語のカスタム構造スライスに変換する方法は?
