スレッドが完了するのを待っている間に Tkinter GUI がフリーズするのを防ぐにはどうすればよいですか?
スレッドの完了を待機中に Tkinter GUI がフリーズ/ハングする
tkinter GUI のボタンが押されると、多くの場合、インターフェイスがフリーズします。スレッドを使用しているにもかかわらず、問題は解決しません。 Python クックブックのアドバイスを活用して、非同期タスクの実行中に GUI の応答性を維持するための解決策を次に示します。
メイン スレッドのブロックを避ける
GUI フリーズの背後にある主な原因は、join( ) バックグラウンド スレッド上で。これを防ぐためのより良いアプローチは、「ポーリング」メカニズムを実装することです。
ポーリング メカニズム
Tkinter の汎用 after() メソッドを使用すると、一定の間隔でキューを継続的に監視できます。これにより、スレッドの完了を待機している間、GUI の応答性を維持できます。
実装例
次のコードは、このアプローチの例です。
<code class="python">import Tkinter as tk import threading import queue # Create a queue for communication queue = queue.Queue() # Define the GUI portion class GuiPart(object): def __init__(self, master, queue): # Set up the GUI tk.Button(master, text='Done', command=self.end_command).pack() def processIncoming(self): while not queue.empty(): # Handle incoming messages here def end_command(self): # Perform necessary cleanup and exit # Define the threaded client class ThreadedClient(object): def __init__(self, master): # Set up GUI and thread self.gui = GuiPart(master, queue) self.thread = threading.Thread(target=self.worker_thread) self.thread.start() # Start periodic checking of the queue self.periodic_call() def periodic_call(self): self.gui.processIncoming() master.after(200, self.periodic_call) def worker_thread(self): # Perform asynchronous I/O tasks here, adding messages to the queue # Main program root = tk.Tk() client = ThreadedClient(root) root.mainloop()</code>
この例では、 GUI とバックグラウンド スレッドはキューを通じて処理されるため、GUI をフリーズさせることなく非同期実行が可能になります。
以上がスレッドが完了するのを待っている間に Tkinter GUI がフリーズするのを防ぐにはどうすればよいですか?の詳細内容です。詳細については、PHP 中国語 Web サイトの他の関連記事を参照してください。

ホットAIツール

Undresser.AI Undress
リアルなヌード写真を作成する AI 搭載アプリ

AI Clothes Remover
写真から衣服を削除するオンライン AI ツール。

Undress AI Tool
脱衣画像を無料で

Clothoff.io
AI衣類リムーバー

Video Face Swap
完全無料の AI 顔交換ツールを使用して、あらゆるビデオの顔を簡単に交換できます。

人気の記事

ホットツール

メモ帳++7.3.1
使いやすく無料のコードエディター

SublimeText3 中国語版
中国語版、とても使いやすい

ゼンドスタジオ 13.0.1
強力な PHP 統合開発環境

ドリームウィーバー CS6
ビジュアル Web 開発ツール

SublimeText3 Mac版
神レベルのコード編集ソフト(SublimeText3)

ホットトピック










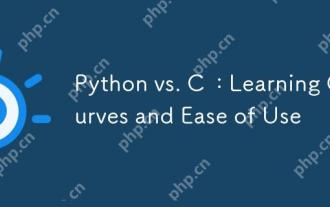
Pythonは学習と使用が簡単ですが、Cはより強力ですが複雑です。 1。Python構文は簡潔で初心者に適しています。動的なタイピングと自動メモリ管理により、使いやすくなりますが、ランタイムエラーを引き起こす可能性があります。 2.Cは、高性能アプリケーションに適した低レベルの制御と高度な機能を提供しますが、学習しきい値が高く、手動メモリとタイプの安全管理が必要です。
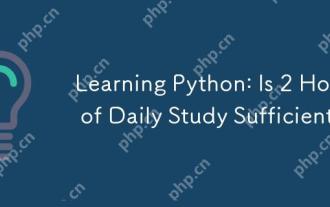
Pythonを1日2時間学ぶだけで十分ですか?それはあなたの目標と学習方法に依存します。 1)明確な学習計画を策定し、2)適切な学習リソースと方法を選択します。3)実践的な実践とレビューとレビューと統合を練習および統合し、統合すると、この期間中にPythonの基本的な知識と高度な機能を徐々に習得できます。
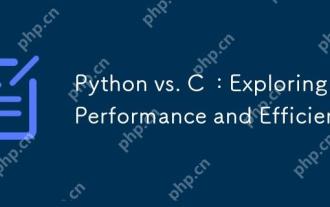
Pythonは開発効率でCよりも優れていますが、Cは実行パフォーマンスが高くなっています。 1。Pythonの簡潔な構文とリッチライブラリは、開発効率を向上させます。 2.Cのコンピレーションタイプの特性とハードウェア制御により、実行パフォーマンスが向上します。選択を行うときは、プロジェクトのニーズに基づいて開発速度と実行効率を比較検討する必要があります。
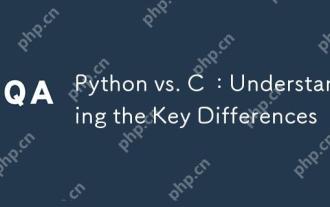
PythonとCにはそれぞれ独自の利点があり、選択はプロジェクトの要件に基づいている必要があります。 1)Pythonは、簡潔な構文と動的タイピングのため、迅速な開発とデータ処理に適しています。 2)Cは、静的なタイピングと手動メモリ管理により、高性能およびシステムプログラミングに適しています。
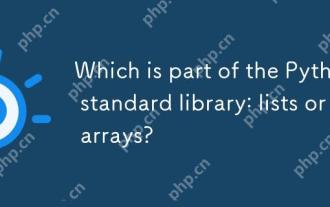
PythonListSarePartOfThestAndardarenot.liestareBuilting-in、versatile、forStoringCollectionsのpythonlistarepart。
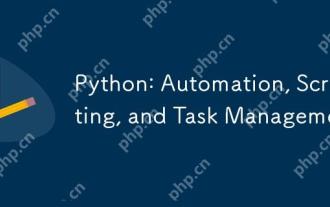
Pythonは、自動化、スクリプト、およびタスク管理に優れています。 1)自動化:OSやShutilなどの標準ライブラリを介してファイルバックアップが実現されます。 2)スクリプトの書き込み:Psutilライブラリを使用してシステムリソースを監視します。 3)タスク管理:スケジュールライブラリを使用してタスクをスケジュールします。 Pythonの使いやすさと豊富なライブラリサポートにより、これらの分野で優先ツールになります。
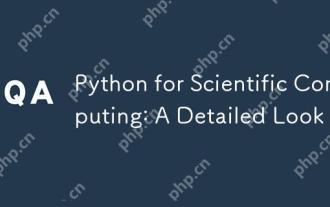
科学コンピューティングにおけるPythonのアプリケーションには、データ分析、機械学習、数値シミュレーション、視覚化が含まれます。 1.numpyは、効率的な多次元配列と数学的関数を提供します。 2。ScipyはNumpy機能を拡張し、最適化と線形代数ツールを提供します。 3. Pandasは、データ処理と分析に使用されます。 4.matplotlibは、さまざまなグラフと視覚的な結果を生成するために使用されます。
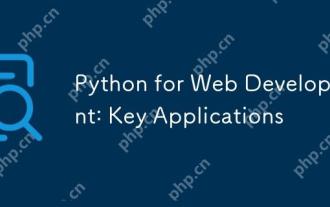
Web開発におけるPythonの主要なアプリケーションには、DjangoおよびFlaskフレームワークの使用、API開発、データ分析と視覚化、機械学習とAI、およびパフォーマンスの最適化が含まれます。 1。DjangoandFlask Framework:Djangoは、複雑な用途の迅速な発展に適しており、Flaskは小規模または高度にカスタマイズされたプロジェクトに適しています。 2。API開発:フラスコまたはdjangorestFrameworkを使用して、Restfulapiを構築します。 3。データ分析と視覚化:Pythonを使用してデータを処理し、Webインターフェイスを介して表示します。 4。機械学習とAI:Pythonは、インテリジェントWebアプリケーションを構築するために使用されます。 5。パフォーマンスの最適化:非同期プログラミング、キャッシュ、コードを通じて最適化
