Turtle Graphics で複数のキー押下イベントをバインドするにはどうすればよいですか?
Turtle Graphics での複数のキー押下イベントのバインド
複数のキー押下からのユーザー入力を調整することは、ゲームを含む多くの対話型アプリケーションにおいて重要です。 Turtle グラフィックスでは、特定のキーの組み合わせに基づいてドットを接続することが一般的な要件です。
複数のキーの押下をまとめて効率的に処理するには、次のアプローチが広く使用されています。
Using win.onkeypress( )
Turtle の win.onkeypress() メソッドは、キー押下イベントを特定の関数にバインドする簡単な方法を提供します。個々のキーごとにイベント リスナーを登録することで、対応するキーが押されたときに必要なアクションを実行できます。
たとえば、キーの組み合わせに基づいてタートルをさまざまな方向に移動させるシナリオを考えてみましょう。 「上」、「下」、「左」、および「右」キーのイベント リスナーを登録し、それぞれの動きを処理する個別の関数を定義できます。
<code class="python">import turtle flynn = turtle.Turtle() win = turtle.Screen() win.listen() def Up(): flynn.setheading(90) flynn.forward(25) def Down(): flynn.setheading(270) flynn.forward(20) def Left(): flynn.setheading(180) flynn.forward(20) def Right(): flynn.setheading(0) flynn.forward(20) win.onkeypress(Up, "Up") win.onkeypress(Down, "Down") win.onkeypress(Left, "Left") win.onkeypress(Right, "Right")</code>
このコードでは、ユーザーがキーを押したときに「上」キーを押すと、タートルは北方向に 25 ユニット移動します。同様に、タートルは「下」、「左」、および「右」キーの押下に応答します。
組み合わせの処理
上記の解決策は単一のキー押下イベントをカバーしていますが、組み合わせを処理するには、 「上」キーと「右」キーを同時に押すなど、より高度なアプローチを採用する必要があります。
組み合わせイベント専用の win.onkeypress() メソッドは、タイミングの問題や不一致を引き起こす可能性があります。代わりに、タイマー メカニズムを利用して、キー押下の組み合わせの発生を定期的にチェックできます。
<code class="python">from turtle import Turtle, Screen win = Screen() flynn = Turtle('turtle') def process_events(): events = tuple(sorted(key_events)) if events and events in key_event_handlers: (key_event_handlers[events])() key_events.clear() win.ontimer(process_events, 200) def Up(): key_events.add('UP') def Down(): key_events.add('DOWN') def Left(): key_events.add('LEFT') def Right(): key_events.add('RIGHT') def move_up(): flynn.setheading(90) flynn.forward(25) def move_down(): flynn.setheading(270) flynn.forward(20) def move_left(): flynn.setheading(180) flynn.forward(20) def move_right(): flynn.setheading(0) flynn.forward(20) def move_up_right(): flynn.setheading(45) flynn.forward(20) def move_down_right(): flynn.setheading(-45) flynn.forward(20) def move_up_left(): flynn.setheading(135) flynn.forward(20) def move_down_left(): flynn.setheading(225) flynn.forward(20) key_event_handlers = { \ ('UP',): move_up, \ ('DOWN',): move_down, \ ('LEFT',): move_left, \ ('RIGHT',): move_right, \ ('RIGHT', 'UP'): move_up_right, \ ('DOWN', 'RIGHT'): move_down_right, \ ('LEFT', 'UP'): move_up_left, \ ('DOWN', 'LEFT'): move_down_left, \ } key_events = set() win.onkey(Up, "Up") win.onkey(Down, "Down") win.onkey(Left, "Left") win.onkey(Right, "Right") win.listen() process_events() win.mainloop()</code>
このアプローチでは、key_events セット内のキー イベントのリストが維持され、タイマー関数が実行されるたびに、 、key_event_handlers ディクショナリに組み合わせが存在するかどうかを確認します。組み合わせが見つかった場合、対応するハンドラー関数が実行され、タートルが目的のアクションを実行します。
以上がTurtle Graphics で複数のキー押下イベントをバインドするにはどうすればよいですか?の詳細内容です。詳細については、PHP 中国語 Web サイトの他の関連記事を参照してください。

ホットAIツール

Undresser.AI Undress
リアルなヌード写真を作成する AI 搭載アプリ

AI Clothes Remover
写真から衣服を削除するオンライン AI ツール。

Undress AI Tool
脱衣画像を無料で

Clothoff.io
AI衣類リムーバー

Video Face Swap
完全無料の AI 顔交換ツールを使用して、あらゆるビデオの顔を簡単に交換できます。

人気の記事

ホットツール

メモ帳++7.3.1
使いやすく無料のコードエディター

SublimeText3 中国語版
中国語版、とても使いやすい

ゼンドスタジオ 13.0.1
強力な PHP 統合開発環境

ドリームウィーバー CS6
ビジュアル Web 開発ツール

SublimeText3 Mac版
神レベルのコード編集ソフト(SublimeText3)

ホットトピック










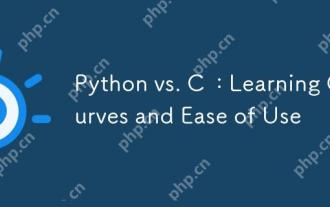
Pythonは学習と使用が簡単ですが、Cはより強力ですが複雑です。 1。Python構文は簡潔で初心者に適しています。動的なタイピングと自動メモリ管理により、使いやすくなりますが、ランタイムエラーを引き起こす可能性があります。 2.Cは、高性能アプリケーションに適した低レベルの制御と高度な機能を提供しますが、学習しきい値が高く、手動メモリとタイプの安全管理が必要です。
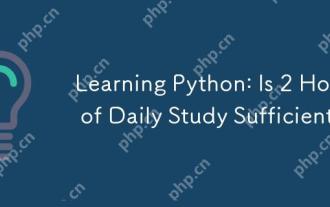
Pythonを1日2時間学ぶだけで十分ですか?それはあなたの目標と学習方法に依存します。 1)明確な学習計画を策定し、2)適切な学習リソースと方法を選択します。3)実践的な実践とレビューとレビューと統合を練習および統合し、統合すると、この期間中にPythonの基本的な知識と高度な機能を徐々に習得できます。
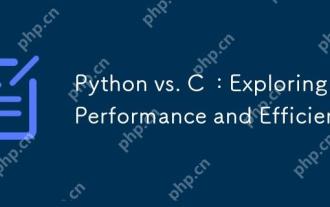
Pythonは開発効率でCよりも優れていますが、Cは実行パフォーマンスが高くなっています。 1。Pythonの簡潔な構文とリッチライブラリは、開発効率を向上させます。 2.Cのコンピレーションタイプの特性とハードウェア制御により、実行パフォーマンスが向上します。選択を行うときは、プロジェクトのニーズに基づいて開発速度と実行効率を比較検討する必要があります。
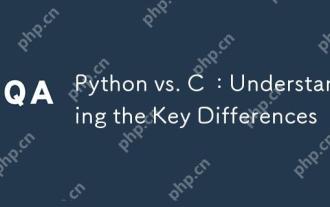
PythonとCにはそれぞれ独自の利点があり、選択はプロジェクトの要件に基づいている必要があります。 1)Pythonは、簡潔な構文と動的タイピングのため、迅速な開発とデータ処理に適しています。 2)Cは、静的なタイピングと手動メモリ管理により、高性能およびシステムプログラミングに適しています。
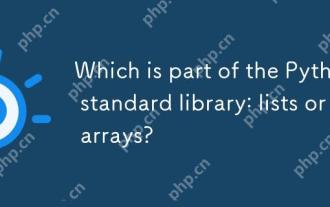
PythonListSarePartOfThestAndardarenot.liestareBuilting-in、versatile、forStoringCollectionsのpythonlistarepart。
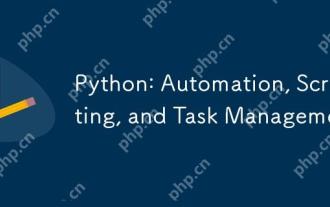
Pythonは、自動化、スクリプト、およびタスク管理に優れています。 1)自動化:OSやShutilなどの標準ライブラリを介してファイルバックアップが実現されます。 2)スクリプトの書き込み:Psutilライブラリを使用してシステムリソースを監視します。 3)タスク管理:スケジュールライブラリを使用してタスクをスケジュールします。 Pythonの使いやすさと豊富なライブラリサポートにより、これらの分野で優先ツールになります。
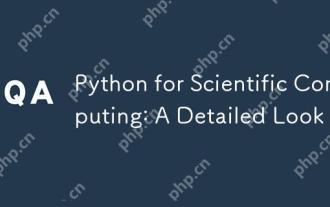
科学コンピューティングにおけるPythonのアプリケーションには、データ分析、機械学習、数値シミュレーション、視覚化が含まれます。 1.numpyは、効率的な多次元配列と数学的関数を提供します。 2。ScipyはNumpy機能を拡張し、最適化と線形代数ツールを提供します。 3. Pandasは、データ処理と分析に使用されます。 4.matplotlibは、さまざまなグラフと視覚的な結果を生成するために使用されます。
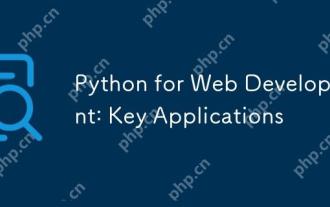
Web開発におけるPythonの主要なアプリケーションには、DjangoおよびFlaskフレームワークの使用、API開発、データ分析と視覚化、機械学習とAI、およびパフォーマンスの最適化が含まれます。 1。DjangoandFlask Framework:Djangoは、複雑な用途の迅速な発展に適しており、Flaskは小規模または高度にカスタマイズされたプロジェクトに適しています。 2。API開発:フラスコまたはdjangorestFrameworkを使用して、Restfulapiを構築します。 3。データ分析と視覚化:Pythonを使用してデータを処理し、Webインターフェイスを介して表示します。 4。機械学習とAI:Pythonは、インテリジェントWebアプリケーションを構築するために使用されます。 5。パフォーマンスの最適化:非同期プログラミング、キャッシュ、コードを通じて最適化
