グラディエーター製品 ダイナミック パーティクルとインタラクティブ アニメーションを備えたグラディエーターをテーマにした製品ショーケース
はじめに
このチュートリアルでは、アニメーション化された製品カード、ダイナミックなホバー効果、クリックインタラクション、各アイテムに命を吹き込む光るパーティクル効果を備えた、超プレミアムな 3D グラディエーターをテーマにした製品ショーケースを作成します。没入型のユーザー エクスペリエンスを実現するように設計されたこのショーケースは、3D 変換、輝くアニメーション、脈動するハイライトを組み合わせて、各製品にユニークでインタラクティブな感触を与えます。このデザインは、プレイヤーが古代の戦いと戦略のスリルを体験するインタラクティブ ゲームである Gladiators Battle からインスピレーションを得ています。
これに沿って独自のインタラクティブな製品ショーケースを作成し、HTML、CSS、JavaScript を使用して見事なビジュアルとダイナミックなアニメーションを作成する方法を学びましょう。
ステップ 1: HTML 構造のセットアップ
各製品カードは、バッジ、アイコン、統計情報などのインタラクティブな要素を備えた、盾や剣などの剣闘士をテーマにしたアイテムを表しています。
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>3D Gladiator Product Showcase</title> <link rel="stylesheet" href="styles.css"> <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/6.0.0-beta3/css/all.min.css"> </head> <body> <div> <p>Key HTML Elements<br> Badge: Labels each item with statuses like "New" or "Popular."<br> Product Image: Displays the item with a glowing effect and 3D depth.<br> Stats: Uses progress bars to display item attributes like defense or attack.<br> Icons: Interactive icons at the bottom of each card provide quick access to favorite actions.<br> Step 2: Designing with CSS<br> Basic Styles and Background<br> The background uses a radial gradient to create a dramatic look, while each product card is styled with gradients, shadows, and smooth transitions.<br> </p> <pre class="brush:php;toolbar:false">body { display: flex; align-items: center; justify-content: center; min-height: 100vh; margin: 0; background: radial-gradient(circle at center, #1b1b2f, #090909); font-family: Arial, sans-serif; overflow: hidden; color: #fff; } .product-showcase { display: flex; gap: 40px; perspective: 1200px; }
製品カードのスタイル
各カードは 3D の外観でデザインされており、インタラクティブ性を高めるホバー効果が含まれています。 :hover エフェクトは微妙な回転と輝きを提供し、高級感を高めます。
.product-card { position: relative; width: 270px; height: 420px; padding: 25px; background: linear-gradient(145deg, #2a2a2a, #3c3c3c); border-radius: 20px; box-shadow: 0 20px 40px rgba(0, 0, 0, 0.7), 0 0 20px rgba(255, 215, 0, 0.5); display: flex; flex-direction: column; align-items: center; justify-content: center; transform-style: preserve-3d; transition: transform 0.5s, box-shadow 0.5s, background 0.5s; cursor: pointer; overflow: hidden; } .product-card:hover { transform: scale(1.1) rotateY(10deg); box-shadow: 0 30px 60px rgba(255, 215, 0, 0.8), 0 0 30px rgba(255, 255, 0, 0.7); }
統計と進行状況バー
プログレスバーを使用して防御力や耐久性などの属性を表示し、ショーケースにユニークな視覚要素を追加します。
.stats { width: 100%; margin-top: 15px; } .stat-bar { display: flex; align-items: center; justify-content: space-between; margin-bottom: 10px; color: #ffd700; font-size: 14px; font-weight: bold; } .progress { width: 60%; height: 8px; background: rgba(255, 255, 255, 0.2); border-radius: 5px; } .progress-bar { height: 100%; background: linear-gradient(90deg, #ffcc00, #f9844a); }
パーティクルエフェクトの追加
動いたり色が変わったりするパーティクルを追加することで没入感が高まります。これらの粒子は脈動して光る効果を与えることができます。
.particle { position: absolute; width: 4px; height: 4px; border-radius: 50%; background: rgba(255, 215, 0, 0.9); box-shadow: 0 0 10px rgba(255, 215, 0, 0.5), 0 0 20px rgba(255, 255, 0, 0.3); animation: particleAnimation 3s ease-in-out infinite, particleMove 4s linear infinite; }
ステップ 3: JavaScript のインタラクティブ性を追加する
JavaScript は、ホバー アニメーション、クリック イベント、および光るパーティクル エフェクトを管理します。
ホバーアニメーションとクリックアニメーションを追加する
マウスの動きでカードの回転と拡大縮小をアニメーション化し、クリックでズームを切り替えます。
const cards = document.querySelectorAll('.product-card'); cards.forEach((card) => { let isClicked = false; card.addEventListener('mousemove', (e) => { if (!isClicked) { const { width, height } = card.getBoundingClientRect(); const offsetX = e.clientX - card.offsetLeft - width / 2; const offsetY = e.clientY - card.offsetTop - height / 2; const rotationX = (offsetY / height) * -25; const rotationY = (offsetX / width) * 25; card.style.transform = `rotateY(${rotationY}deg) rotateX(${rotationX}deg) scale(1.05)`; } }); card.addEventListener('mouseleave', () => { if (!isClicked) { card.style.transform = 'rotateY(0deg) rotateX(0deg) scale(1)'; } }); card.addEventListener('click', () => { isClicked = !isClicked; card.style.transform = isClicked ? 'scale(1.2) rotateY(0deg) rotateX(0deg)' : 'rotateY(0deg) rotateX(0deg) scale(1)'; }); });
光るパーティクルを追加する
雰囲気を高めるために、各商品カードの周りでランダムに動くパーティクルを作成します。
function addParticleEffect() { const particle = document.createElement('div'); particle.classList.add('particle'); particle.style.left = `${Math.random() * 100}%`; particle.style.top = `${Math.random() * 100}%`; particle.style.animationDuration = `${2 + Math.random() * 3}s`; document.body.appendChild(particle); setTimeout(() => particle.remove(), 5000); } setInterval(() => { cards.forEach(() => addParticleEffect()); }, 1000);
結論
ダイナミックなアニメーションとパーティクル効果を備えた 3D 剣闘士をテーマにした製品ショーケースを構築すると、ユーザーを魅了する魅力的でインタラクティブなエクスペリエンスが生まれます。視覚的なスタイル設定のための CSS とインタラクティブ性のための JavaScript を組み合わせることで、製品ディスプレイやゲーム関連サイトに最適な高品質で没入型のコンポーネントを作成しました。剣闘士の戦いからインスピレーションを得たこのショーケースは、現代の Web デザインと歴史的テーマを組み合わせる力を強調しています。
?さらに発見:
剣闘士の戦いを探索する: https://gladiatorsbattle.com で古代の戦士と戦略的なゲームプレイの世界に飛び込みましょう。
GitHub: https://github.com/HanGPIErr で他のプロジェクトをご覧ください。
LinkedIn: https://www.linkedin.com/in/pierre-romain-lopez/ でプロジェクトの最新情報を入手してください。
Twitter: https://x.com/GladiatorsBT で設計と開発の洞察を参照してください。
魅力的でインタラクティブなコンポーネントの作成に関するさらなるチュートリアルにご期待ください!
以上がグラディエーター製品 ダイナミック パーティクルとインタラクティブ アニメーションを備えたグラディエーターをテーマにした製品ショーケースの詳細内容です。詳細については、PHP 中国語 Web サイトの他の関連記事を参照してください。

ホットAIツール

Undresser.AI Undress
リアルなヌード写真を作成する AI 搭載アプリ

AI Clothes Remover
写真から衣服を削除するオンライン AI ツール。

Undress AI Tool
脱衣画像を無料で

Clothoff.io
AI衣類リムーバー

Video Face Swap
完全無料の AI 顔交換ツールを使用して、あらゆるビデオの顔を簡単に交換できます。

人気の記事

ホットツール

メモ帳++7.3.1
使いやすく無料のコードエディター

SublimeText3 中国語版
中国語版、とても使いやすい

ゼンドスタジオ 13.0.1
強力な PHP 統合開発環境

ドリームウィーバー CS6
ビジュアル Web 開発ツール

SublimeText3 Mac版
神レベルのコード編集ソフト(SublimeText3)

ホットトピック










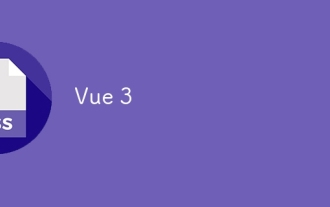
それは&#039; Vueチームにそれを成し遂げてくれておめでとうございます。それは大規模な努力であり、長い時間がかかったことを知っています。すべての新しいドキュメントも同様です。
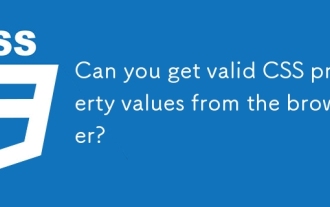
私はこの非常に正当な質問で誰かに書いてもらいました。 Leaは、ブラウザから有効なCSSプロパティ自体を取得する方法についてブログを書いています。それはこのようなものです。
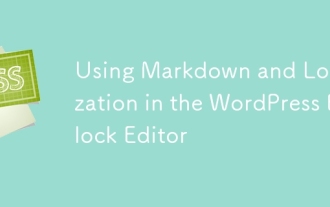
WordPressエディターでユーザーに直接ドキュメントを表示する必要がある場合、それを行うための最良の方法は何ですか?
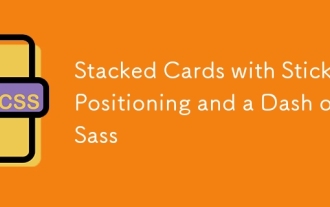
先日、Corey Ginnivanのウェブサイトから、この特に素敵なビットを見つけました。そこでは、スクロール中にカードのコレクションが互いに積み重ねられていました。
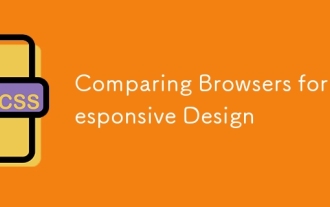
これらのデスクトップアプリがいくつかあり、目標があなたのサイトをさまざまな次元ですべて同時に表示しています。たとえば、書くことができます
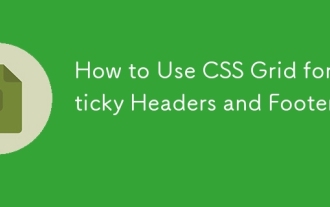
CSS Gridは、レイアウトをこれまで以上に簡単にするように設計されたプロパティのコレクションです。何でもするように、少し学習曲線がありますが、グリッドは
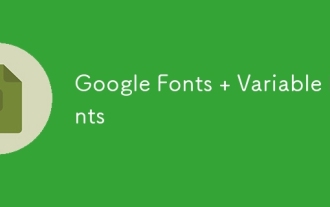
Google Fontsが新しいデザイン(ツイート)を展開したようです。最後の大きな再設計と比較して、これははるかに反復的です。違いをほとんど伝えることができません
