不明なキーを使用してネストされた JSON をアンマーシャリングするにはどうすればよいですか?
不明なキーを含むネストされた JSON を解明する
JSON の複雑さを解明する
未知のキーと複雑なネスト構造を含む JSON データに遭遇することは、気の遠くなるような作業になる可能性があります。次の JSON 形式を考えてみましょう:
{ "message": { "Server1.example.com": [ { "application": "Apache", "host": { "name": "/^Server-[13456]/" }, "owner": "User1", "project": "Web", "subowner": "User2" } ], "Server2.example.com": [ { "application": "Mysql", "host": { "name": "/^Server[23456]/" }, "owner": "User2", "project": "DB", "subowner": "User3" } ] }, "response_ms": 659, "success": true }
この例に示すように、サーバー名 (Server[0-9].example.com) は事前に決定されておらず、変更される可能性があります。さらに、サーバー名に続くフィールドには明示的なキーがありません。
ソリューションの構造
このデータを効果的に取得するには、map[string]ServerStruct 構造を使用できます。
type YourStruct struct { Success bool ResponseMS int Servers map[string]*ServerStruct } type ServerStruct struct { Application string Owner string [...] }
これらの構造を組み込むことで、マップへの不明なサーバー名の割り当てが可能になります。
JSON の秘密を明らかにする
JSON のアンマーシャリングをさらに効率的に行うには、必要な JSON タグを追加することを検討してください。
import "encoding/json" // YourStruct contains the JSON structure with success, response time, and a map of servers type YourStruct struct { Success bool ResponseMS int `json:"response_ms"` Servers map[string]*ServerStruct `json:"message"` } // ServerStruct holds server information, including application, owner, etc. type ServerStruct struct { Application string `json:"application"` Owner string `json:"owner"` [...] } // UnmarshalJSON is a custom unmarshaller that handles nesting and unknown keys func (s *YourStruct) UnmarshalJSON(data []byte) error { type YourStructHelper struct { Success bool ResponseMS int `json:"response_ms"` Servers map[string]ServerStruct `json:"message"` } var helper YourStructHelper if err := json.Unmarshal(data, &helper); err != nil { return err } s.Success = helper.Success s.ResponseMS = helper.ResponseMS s.Servers = make(map[string]*ServerStruct) for k, v := range helper.Servers { s.Servers[k] = &v // Explicitly allocate memory for each server } return nil }
これらの調整により、提供された JSON をカスタム構造体に効果的にアンマーシャリングし、簡単なデータ操作への道を開くことができます。
以上が不明なキーを使用してネストされた JSON をアンマーシャリングするにはどうすればよいですか?の詳細内容です。詳細については、PHP 中国語 Web サイトの他の関連記事を参照してください。

ホットAIツール

Undresser.AI Undress
リアルなヌード写真を作成する AI 搭載アプリ

AI Clothes Remover
写真から衣服を削除するオンライン AI ツール。

Undress AI Tool
脱衣画像を無料で

Clothoff.io
AI衣類リムーバー

Video Face Swap
完全無料の AI 顔交換ツールを使用して、あらゆるビデオの顔を簡単に交換できます。

人気の記事

ホットツール

メモ帳++7.3.1
使いやすく無料のコードエディター

SublimeText3 中国語版
中国語版、とても使いやすい

ゼンドスタジオ 13.0.1
強力な PHP 統合開発環境

ドリームウィーバー CS6
ビジュアル Web 開発ツール

SublimeText3 Mac版
神レベルのコード編集ソフト(SublimeText3)

ホットトピック










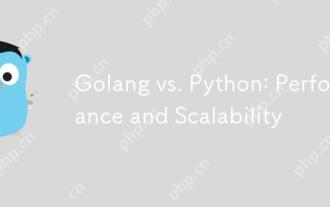
Golangは、パフォーマンスとスケーラビリティの点でPythonよりも優れています。 1)Golangのコンピレーションタイプの特性と効率的な並行性モデルにより、高い並行性シナリオでうまく機能します。 2)Pythonは解釈された言語として、ゆっくりと実行されますが、Cythonなどのツールを介してパフォーマンスを最適化できます。
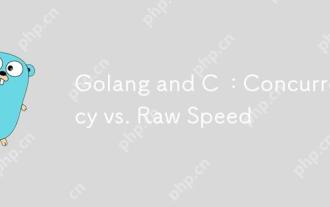
Golangは並行性がCよりも優れていますが、Cは生の速度ではGolangよりも優れています。 1)Golangは、GoroutineとChannelを通じて効率的な並行性を達成します。これは、多数の同時タスクの処理に適しています。 2)Cコンパイラの最適化と標準ライブラリを介して、極端な最適化を必要とするアプリケーションに適したハードウェアに近い高性能を提供します。
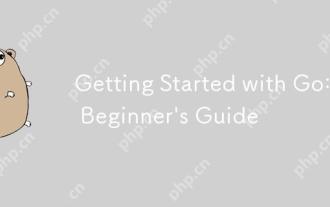
goisidealforforbeginnersandsutable forcloudnetworkservicesduetoitssimplicity、andconcurrencyfeatures.1)installgofromtheofficialwebsiteandverify with'goversion'.2)
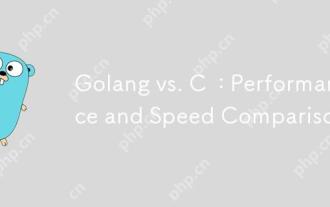
Golangは迅速な発展と同時シナリオに適しており、Cは極端なパフォーマンスと低レベルの制御が必要なシナリオに適しています。 1)Golangは、ごみ収集と並行機関のメカニズムを通じてパフォーマンスを向上させ、高配列Webサービス開発に適しています。 2)Cは、手動のメモリ管理とコンパイラの最適化を通じて究極のパフォーマンスを実現し、埋め込みシステム開発に適しています。
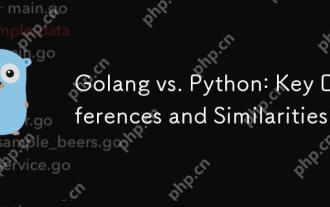
GolangとPythonにはそれぞれ独自の利点があります。Golangは高性能と同時プログラミングに適していますが、PythonはデータサイエンスとWeb開発に適しています。 Golangは同時性モデルと効率的なパフォーマンスで知られていますが、Pythonは簡潔な構文とリッチライブラリエコシステムで知られています。
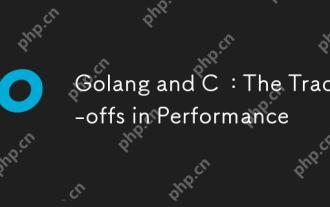
GolangとCのパフォーマンスの違いは、主にメモリ管理、コンピレーションの最適化、ランタイム効率に反映されています。 1)Golangのゴミ収集メカニズムは便利ですが、パフォーマンスに影響を与える可能性があります。
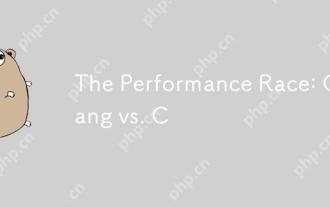
GolangとCにはそれぞれパフォーマンス競争において独自の利点があります。1)Golangは、高い並行性と迅速な発展に適しており、2)Cはより高いパフォーマンスと微細な制御を提供します。選択は、プロジェクトの要件とチームテクノロジースタックに基づいている必要があります。
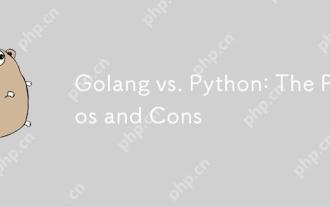
GolangisidealforBuildingsCalables Systemsduetoitsefficiency andConcurrency、Whilepythonexcelsinquickscriptinganddataanalysisduetoitssimplicityand vastecosystem.golang'ssignencouragesclean、readisinediteNeditinesinedinediseNabletinedinedinedisedisedioncourase
