猶予付きアサート: AssertJ を使用したよりクリーンなコードのためのカスタム ソフト アサーション
導入
ソフト アサーションが何なのかわからない場合は、「ソフト アサーション – 単体テストと統合テストにソフト アサーションを使用する必要がある理由」をお読みください。
この記事は、Assert J を使用してカスタム アサーションを作成する方法を説明する「Assert with Grace: Custom Assertions for Cleaner Code」の続きです。ここでは、そのアプローチを拡張して、カスタム アサーションに加えてソフト アサーション アプローチを使用する方法を学びます。
AssertJ を使用したカスタム ソフト アサーション
AssertJ の Assertions クラスまたはカスタム クラスを使用して、ハード アサーションを作成できます。ソフト アサーションの利点をすべて得るには、次のことを行う必要があります。
- カスタム アサーションを実装する
- カスタム ソフト アサーション クラスを作成し、AssertJ から AbstractSoftAssertion を拡張します
カスタム アサーション
カスタム アサーションの作成方法については、「猶予付きアサート: よりクリーンなコードのためのカスタム アサーション」の記事で学習しました。次のようになります:
public class SimulationAssert extends AbstractAssert<SimulationAssert, Simulation> { protected SimulationAssert(Simulation actual) { super(actual, SimulationAssert.class); } public static SimulationAssert assertThat(Simulation actual) { return new SimulationAssert(actual); } public SimulationAssert hasValidInstallments() { isNotNull(); if (actual.getInstallments() < 2 || actual.getInstallments() >= 48) { failWithMessage("Installments must be must be equal or greater than 2 and equal or less than 48"); } return this; } public SimulationAssert hasValidAmount() { isNotNull(); var minimum = new BigDecimal("1.000"); var maximum = new BigDecimal("40.000"); if (actual.getAmount().compareTo(minimum) < 0 || actual.getAmount().compareTo(maximum) > 0) { failWithMessage("Amount must be equal or greater than $ 1.000 or equal or less than than $ 40.000"); } return this; } }
カスタム アサーションを使用すると、テストでの読みやすさが向上するだけでなく、有効な値をテストする責任がカスタム アサーションに送信されます。
class SimulationsCustomAssertionTest { @Test void simulationErrorAssertion() { var simulation = Simulation.builder().name("John").cpf("9582728395").email("john@gmail.com") .amount(new BigDecimal("1.500")).installments(5).insurance(false).build(); SimulationAssert.assertThat(simulation).hasValidInstallments(); SimulationAssert.assertThat(simulation).hasValidAmount(); } }
カスタム アサーションを用意したら、カスタム ソフト アサーションを実装します。
カスタム ソフト アサーションを作成する
カスタム ソフト アサーションを作成する簡単なプロセスがあり、前提条件としてカスタム アサーションを実装する必要があります。前の記事を考慮すると、カスタム アサーションとして SimulationAssert クラスがあり、カスタム ソフト アサーションとして SimulationSoftAssert を作成します。手順は次のとおりです:
- AbstractSoftAssertions クラスを拡張します
- 次のコマンドを使用して、assertThat() メソッドを作成します。
- メソッドはカスタム アサーション クラスとしてオブジェクトを返します
- アサーションの主題へのパラメータ
- メソッドは、パラメータがカスタム アサーション クラスとアサーションのサブジェクトであるメソッド プロキシを返します
- 次のコマンドを使用して、assertSoftly() メソッドを作成します。
- カスタム ソフト アサート クラスのコンシューマとしてのパラメータ
- パラメータがカスタム ソフト アサーション クラスとメソッド パラメータであるため、SoftAssertionsProvider.assertSoftly() メソッドを使用します
手順は複雑に見えますが、実際には次のようになります。
public class SimulationSoftAssert extends AbstractSoftAssertions { public SimulationAssert assertThat(Simulation actual) { return proxy(SimulationAssert.class, Simulation.class, actual); } public static void assertSoftly(Consumer<SimulationSoftAssert> softly) { SoftAssertionsProvider.assertSoftly(SimulationSoftAssert.class, softly); } }
カスタム ソフト アサーションの使用
AssertJ SoftAssertion クラスはソフト アサーションを担当します。これは、シミュレーション コンテキストに適用できる例です:
AssertJ SoftAssertion クラスはソフト アサーションを担当します。これは、シミュレーション コンテキストに適用できる例です:
public class SimulationAssert extends AbstractAssert<SimulationAssert, Simulation> { protected SimulationAssert(Simulation actual) { super(actual, SimulationAssert.class); } public static SimulationAssert assertThat(Simulation actual) { return new SimulationAssert(actual); } public SimulationAssert hasValidInstallments() { isNotNull(); if (actual.getInstallments() < 2 || actual.getInstallments() >= 48) { failWithMessage("Installments must be must be equal or greater than 2 and equal or less than 48"); } return this; } public SimulationAssert hasValidAmount() { isNotNull(); var minimum = new BigDecimal("1.000"); var maximum = new BigDecimal("40.000"); if (actual.getAmount().compareTo(minimum) < 0 || actual.getAmount().compareTo(maximum) > 0) { failWithMessage("Amount must be equal or greater than $ 1.000 or equal or less than than $ 40.000"); } return this; } }
これを使用する場合の「問題」は、作成したカスタム アサーションを使用できないことです。上記の例では、SoftAssertions クラスはカスタム アサーションにアクセスできないため、isEqualTo() を使用して分割払いと金額のアサーションを確認できます。
カスタム ソフト アサーション クラスを作成することで、この問題を解決しました。したがって、SoftAssertions クラスを使用する代わりに、カスタム クラス SimulationSoftAssert.
を使用します。
class SimulationsCustomAssertionTest { @Test void simulationErrorAssertion() { var simulation = Simulation.builder().name("John").cpf("9582728395").email("john@gmail.com") .amount(new BigDecimal("1.500")).installments(5).insurance(false).build(); SimulationAssert.assertThat(simulation).hasValidInstallments(); SimulationAssert.assertThat(simulation).hasValidAmount(); } }
SimulationSoftAssert.assertSoftly() は、アサーション中のエラーやその他のアクティビティを管理できるようにすべての内部メソッドを呼び出すソフト アサーションのプロバイダーです。使用中のassertThat()は、assertSoftly()内で、ソフト・アサートとアサーションのサブジェクトの間のproxy()によってカスタム・アサーションにアクセスできるカスタムのものになります。
このアプローチを使用すると、カスタム アサーションを実装することにより、ソフト アサーションでカスタム アサーションを利用できるようになります。
終わり
以上です!
完全に実装され、実際に動作する例は、credit-api プロジェクトにあります。ここでは、次の内容を確認できます。
- SimulationAssert クラス
- SimulationsCustomAssertionTest クラスでのテストの使用法
以上が猶予付きアサート: AssertJ を使用したよりクリーンなコードのためのカスタム ソフト アサーションの詳細内容です。詳細については、PHP 中国語 Web サイトの他の関連記事を参照してください。

ホットAIツール

Undresser.AI Undress
リアルなヌード写真を作成する AI 搭載アプリ

AI Clothes Remover
写真から衣服を削除するオンライン AI ツール。

Undress AI Tool
脱衣画像を無料で

Clothoff.io
AI衣類リムーバー

Video Face Swap
完全無料の AI 顔交換ツールを使用して、あらゆるビデオの顔を簡単に交換できます。

人気の記事

ホットツール

メモ帳++7.3.1
使いやすく無料のコードエディター

SublimeText3 中国語版
中国語版、とても使いやすい

ゼンドスタジオ 13.0.1
強力な PHP 統合開発環境

ドリームウィーバー CS6
ビジュアル Web 開発ツール

SublimeText3 Mac版
神レベルのコード編集ソフト(SublimeText3)

ホットトピック










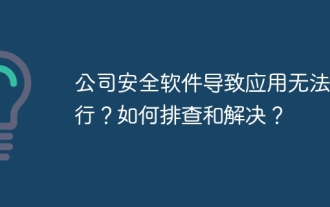
一部のアプリケーションが適切に機能しないようにする会社のセキュリティソフトウェアのトラブルシューティングとソリューション。多くの企業は、内部ネットワークセキュリティを確保するためにセキュリティソフトウェアを展開します。 ...
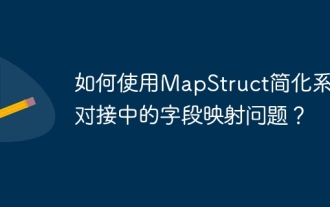
システムドッキングでのフィールドマッピング処理は、システムドッキングを実行する際に難しい問題に遭遇することがよくあります。システムのインターフェイスフィールドを効果的にマッピングする方法A ...
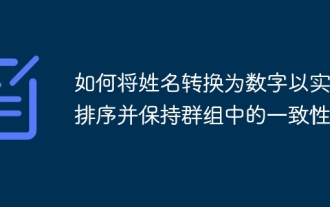
多くのアプリケーションシナリオでソートを実装するために名前を数値に変換するソリューションでは、ユーザーはグループ、特に1つでソートする必要がある場合があります...
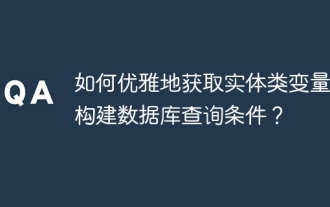
データベース操作にMyBatis-Plusまたはその他のORMフレームワークを使用する場合、エンティティクラスの属性名に基づいてクエリ条件を構築する必要があることがよくあります。あなたが毎回手動で...
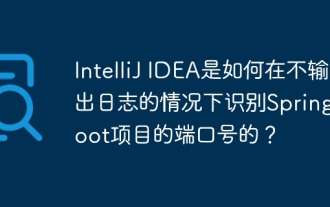
intellijideaultimatiateバージョンを使用してスプリングを開始します...
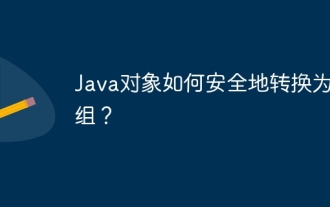
Javaオブジェクトと配列の変換:リスクの詳細な議論と鋳造タイプ変換の正しい方法多くのJava初心者は、オブジェクトのアレイへの変換に遭遇します...
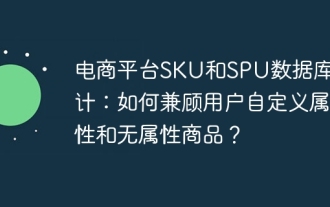
eコマースプラットフォーム上のSKUおよびSPUテーブルの設計の詳細な説明この記事では、eコマースプラットフォームでのSKUとSPUのデータベース設計の問題、特にユーザー定義の販売を扱う方法について説明します。
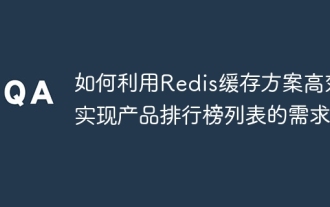
Redisキャッシュソリューションは、製品ランキングリストの要件をどのように実現しますか?開発プロセス中に、多くの場合、ランキングの要件に対処する必要があります。
