アセンブリでの strlen と strcmp の再作成: ステップバイステップ ガイド
アセンブリで低レベル関数を記述するのは難しそうに思えるかもしれませんが、内部でどのように機能するかについて理解を深めるには優れた方法です。このブログでは、2 つの一般的な C 標準ライブラリ関数 strlen と strcmp をアセンブリ言語で再作成し、C プログラムからそれらを呼び出す方法を学びます。
このガイドは初心者向けなので、アセンブリ プログラミングが初めてでも心配する必要はありません。飛び込んでみましょう! ?
目次
- アセンブリと C 統合の概要
- strlen と strcmp とは何ですか?
- 環境のセットアップ
- アセンブリでの strlen の記述
- アセンブリでの strcmp の記述
- アセンブリと C の統合
- プログラムのコンパイルと実行
- 期待される出力
- 結論
- Twitter でつながる
1.アセンブリと C 統合の概要
アセンブリ言語は、マシンコードに近い非常に低レベルで動作します。 C のような高級言語と組み合わせると、両方の長所を活用できます。
- 高レベルの可読性。
- 低レベルの制御と最適化。
このガイドでは、アセンブリで 2 つの関数 (my_strlen と my_strcmp) を作成し、C から呼び出してこの統合を示します。
2. strlen と strcmp とは何ですか?
- strlen: この関数は、ヌル終了文字列 ( で終わる文字列) の長さを計算します。
-
strcmp: この関数は 2 つの文字列を 1 文字ずつ比較します。返される内容は次のとおりです。
- 文字列が同一の場合は 0。
- 最初の文字列が小さい場合は負の値。
- 最初の文字列の方が大きい場合は正の値。
アセンブリでの動作を再現します。
3.環境のセットアップ
必要なツール:
- NASM (ネットワイド アセンブラー): アセンブリ コードをアセンブルします。
- GCC (GNU Compiler Collection): C およびアセンブリ コードをコンパイルおよびリンクします。
ツールのインストール (Linux):
次のコマンドを実行します:
sudo apt update sudo apt install nasm gcc
4.アセンブリでの strlen の記述
strlen のロジック:
- カウンターを 0 に初期化して開始します。
- ヌル終端文字 ( ) が見つかるまで、文字列の各文字を読み取ります。
- カウンターを長さとして返します。
my_strlen のアセンブリ コード:
sudo apt update sudo apt install nasm gcc
-
使用されるレジスタ:
- RDI: 入力文字列を指します。
- RAX: 文字列長を格納します (出力)。
5.アセンブリでの strcmp の記述
strcmp のロジック:
- 2 つの文字列の文字を比較します。
- 文字が異なるか、null ターミネータ ( ) に達すると停止します。
- 戻る:
- 文字列が等しい場合は 0。
- ASCII 値が異なる場合の違い。
my_strcmp のアセンブリ コード:
section .text global my_strlen my_strlen: xor rax, rax ; Set RAX (length) to 0 .next_char: cmp byte [rdi + rax], 0 ; Compare current byte with 0 je .done ; If 0, jump to done inc rax ; Increment RAX jmp .next_char ; Repeat .done: ret ; Return length in RAX
-
使用されるレジスタ:
- RDI および RSI: 入力文字列へのポインター。
- RAX:結果(出力)を格納します。
6.アセンブリと C の統合
これらのアセンブリ関数を呼び出す C プログラムを書いてみましょう。
C コード:
section .text global my_strcmp my_strcmp: xor rax, rax ; Set RAX (result) to 0 .next_char: mov al, [rdi] ; Load byte from first string cmp al, [rsi] ; Compare with second string jne .diff ; If not equal, jump to diff test al, al ; Check if we’ve hit <pre class="brush:php;toolbar:false">#include <stdio.h> #include <stddef.h> // Declare the assembly functions extern size_t my_strlen(const char *str); extern int my_strcmp(const char *s1, const char *s2); int main() { // Test my_strlen const char *msg = "Hello, Assembly!"; size_t len = my_strlen(msg); printf("Length of '%s': %zu\n", msg, len); // Test my_strcmp const char *str1 = "Hello"; const char *str2 = "Hello"; const char *str3 = "World"; int result1 = my_strcmp(str1, str2); int result2 = my_strcmp(str1, str3); printf("Comparing '%s' and '%s': %d\n", str1, str2, result1); printf("Comparing '%s' and '%s': %d\n", str1, str3, result2); return 0; }
nasm -f elf64 functions.asm -o functions.o gcc main.c functions.o -o main ./main
7.プログラムのコンパイルと実行
- アセンブリ コードをfunctions.asmに保存します。
- C コードを main.c に保存します。
- それらをコンパイルしてリンクします。
Length of 'Hello, Assembly!': 17 Comparing 'Hello' and 'Hello': 0 Comparing 'Hello' and 'World': -15
8.期待される出力
9.結論
アセンブリで strlen と strcmp を記述することで、以下についてより深く理解できます。
- 低レベルのメモリ操作。
- マシンレベルで文字列が処理される方法。
- アセンブリを C と統合して、両方の言語の長所を活用します。
アセンブリで再作成したい他の C 標準ライブラリ関数は何ですか?以下のコメント欄でお知らせください。
10. Twitter でつながる
このガイドは気に入りましたか? Twitter でご意見やご質問を共有してください。一緒に接続して、より低レベルのプログラミングを探索しましょう。 ?
以上がアセンブリでの strlen と strcmp の再作成: ステップバイステップ ガイドの詳細内容です。詳細については、PHP 中国語 Web サイトの他の関連記事を参照してください。

ホットAIツール

Undresser.AI Undress
リアルなヌード写真を作成する AI 搭載アプリ

AI Clothes Remover
写真から衣服を削除するオンライン AI ツール。

Undress AI Tool
脱衣画像を無料で

Clothoff.io
AI衣類リムーバー

Video Face Swap
完全無料の AI 顔交換ツールを使用して、あらゆるビデオの顔を簡単に交換できます。

人気の記事

ホットツール

メモ帳++7.3.1
使いやすく無料のコードエディター

SublimeText3 中国語版
中国語版、とても使いやすい

ゼンドスタジオ 13.0.1
強力な PHP 統合開発環境

ドリームウィーバー CS6
ビジュアル Web 開発ツール

SublimeText3 Mac版
神レベルのコード編集ソフト(SublimeText3)

ホットトピック










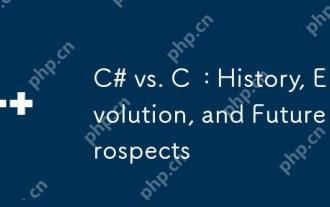
C#とCの歴史と進化はユニークであり、将来の見通しも異なります。 1.Cは、1983年にBjarnestrostrupによって発明され、オブジェクト指向のプログラミングをC言語に導入しました。その進化プロセスには、C 11の自動キーワードとラムダ式の導入など、複数の標準化が含まれます。C20概念とコルーチンの導入、将来のパフォーマンスとシステムレベルのプログラミングに焦点を当てます。 2.C#は2000年にMicrosoftによってリリースされました。CとJavaの利点を組み合わせて、その進化はシンプルさと生産性に焦点を当てています。たとえば、C#2.0はジェネリックを導入し、C#5.0は非同期プログラミングを導入しました。これは、将来の開発者の生産性とクラウドコンピューティングに焦点を当てます。
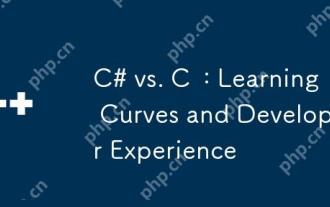
C#とCおよび開発者の経験の学習曲線には大きな違いがあります。 1)C#の学習曲線は比較的フラットであり、迅速な開発およびエンタープライズレベルのアプリケーションに適しています。 2)Cの学習曲線は急勾配であり、高性能および低レベルの制御シナリオに適しています。
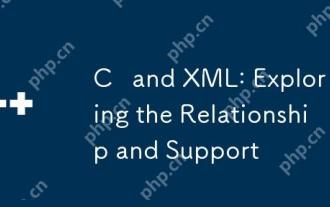
Cは、サードパーティライブラリ(TinyXML、PUGIXML、XERCES-Cなど)を介してXMLと相互作用します。 1)ライブラリを使用してXMLファイルを解析し、それらをC処理可能なデータ構造に変換します。 2)XMLを生成するときは、Cデータ構造をXML形式に変換します。 3)実際のアプリケーションでは、XMLが構成ファイルとデータ交換に使用されることがよくあり、開発効率を向上させます。
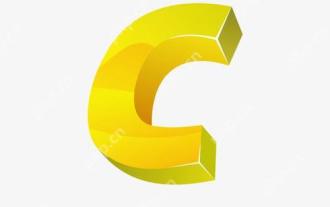
Cでの静的分析の適用には、主にメモリ管理の問題の発見、コードロジックエラーの確認、およびコードセキュリティの改善が含まれます。 1)静的分析では、メモリリーク、ダブルリリース、非初期化ポインターなどの問題を特定できます。 2)未使用の変数、死んだコード、論理的矛盾を検出できます。 3)カバー性などの静的分析ツールは、バッファーオーバーフロー、整数のオーバーフロー、安全でないAPI呼び出しを検出して、コードセキュリティを改善します。
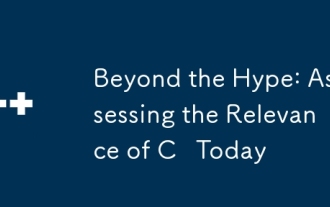
Cは、現代のプログラミングにおいて依然として重要な関連性を持っています。 1)高性能および直接的なハードウェア操作機能により、ゲーム開発、組み込みシステム、高性能コンピューティングの分野で最初の選択肢になります。 2)豊富なプログラミングパラダイムとスマートポインターやテンプレートプログラミングなどの最新の機能は、その柔軟性と効率を向上させます。学習曲線は急ですが、その強力な機能により、今日のプログラミングエコシステムでは依然として重要です。
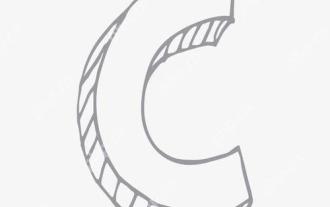
CでChronoライブラリを使用すると、時間と時間の間隔をより正確に制御できます。このライブラリの魅力を探りましょう。 CのChronoライブラリは、時間と時間の間隔に対処するための最新の方法を提供する標準ライブラリの一部です。 Time.HとCtimeに苦しんでいるプログラマーにとって、Chronoは間違いなく恩恵です。コードの読みやすさと保守性を向上させるだけでなく、より高い精度と柔軟性も提供します。基本から始めましょう。 Chronoライブラリには、主に次の重要なコンポーネントが含まれています。STD:: Chrono :: System_Clock:現在の時間を取得するために使用されるシステムクロックを表します。 STD :: Chron
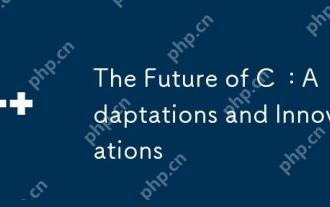
Cの将来は、並列コンピューティング、セキュリティ、モジュール化、AI/機械学習に焦点を当てます。1)並列コンピューティングは、コルーチンなどの機能を介して強化されます。 2)セキュリティは、より厳格なタイプのチェックとメモリ管理メカニズムを通じて改善されます。 3)変調は、コード組織とコンパイルを簡素化します。 4)AIと機械学習は、数値コンピューティングやGPUプログラミングサポートなど、CにComply Coveに適応するように促します。
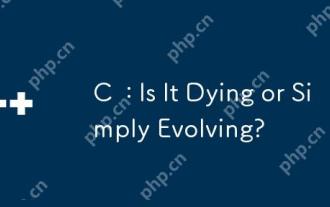
c isnotdying; it'sevolving.1)c relelevantdueToitsversitileSileSixivisityinperformance-criticalApplications.2)thelanguageSlikeModulesandCoroutoUtoimveUsablive.3)despiteChallen
