JavaScript 配列メソッド: コードのパフォーマンスと可読性を向上させる
はじめに: 効率的な配列操作の力
配列は JavaScript データ処理のバックボーンです。このガイドでは、配列の操作方法を変革し、より速く、よりクリーンで、より効率的なコードを記述する方法を示します。
1. 適切な反復方法の選択
性能比較
const numbers = Array.from({ length: 10000 }, (_, i) => i); // ?️ Fastest: Traditional For Loop console.time('For Loop'); for (let i = 0; i < numbers.length; i++) { // Process numbers[i] } console.timeEnd('For Loop'); // ? Good Performance: forEach console.time('forEach'); numbers.forEach(num => { // Process number }); console.timeEnd('forEach'); // ? Slowest: for...of console.time('for...of'); for (const num of numbers) { // Process number } console.timeEnd('for...of'); // ?️ Special Case: for...in console.time('for...in'); for (const index in numbers) { // Process numbers[index] } console.timeEnd('for...in');
プロのヒント: 異なるループをいつ使用するか
- For ループ: 大規模な配列の場合は最速
- forEach: クリーンで、簡単な操作で読みやすい
- for...of: 休憩/継続する必要がある場合に最適です
- for...in: 主にオブジェクトのプロパティ に使用する場合は慎重に使用してください。
ボーナス: for...in ループについて理解する
// Demonstrating for...in Behavior const problemArray = [1, 2, 3]; problemArray.customProperty = 'Danger!'; console.log('for...in Iteration:'); for (const index in problemArray) { console.log(problemArray[index]); // Logs: 1, 2, 3, and 'Danger!' } // Safe Object Iteration const user = { name: 'Alice', age: 30 }; console.log('Safe Object Iteration:'); for (const key in user) { if (user.hasOwnProperty(key)) { console.log(`${key}: ${user[key]}`); } }
2. 配列の変換: マップと従来のループ
非効率的なアプローチ
// ❌ Slow and Verbose let doubledNumbers = []; for (let i = 0; i < numbers.length; i++) { doubledNumbers.push(numbers[i] * 2); }
最適化されたアプローチ
// ✅ Efficient and Readable const doubledNumbers = numbers.map(num => num * 2);
3. データのフィルタリング: スマートかつ高速
実際のフィルタリングの例
const products = [ { name: 'Laptop', price: 1200, inStock: true }, { name: 'Phone', price: 800, inStock: false }, { name: 'Tablet', price: 500, inStock: true } ]; // Multiple Filter Conditions const affordableAndAvailableProducts = products .filter(product => product.price < 1000) .filter(product => product.inStock);
最適化されたフィルタリング技術
// ? More Efficient Single-Pass Filtering const affordableProducts = products.filter(product => product.price < 1000 && product.inStock );
4. 配列の削減: 単に合計するだけではない
複雑なデータの集約
const transactions = [ { category: 'Food', amount: 50 }, { category: 'Transport', amount: 30 }, { category: 'Food', amount: 40 } ]; // Group and Calculate Spending const categorySummary = transactions.reduce((acc, transaction) => { // Initialize category if not exists acc[transaction.category] = (acc[transaction.category] || 0) + transaction.amount; return acc; }, {}); // Result: { Food: 90, Transport: 30 }
5. よくあるパフォーマンスの落とし穴の回避
メモリ効率の高い配列のクリア
// ✅ Best Way to Clear an Array let myArray = [1, 2, 3, 4, 5]; myArray.length = 0; // Fastest method // ❌ Less Efficient Methods // myArray = []; // Creates new array // myArray.splice(0, myArray.length); // More overhead
6. スプレッド演算子: 強力かつ効率的
セーフアレイのコピー
// Create Shallow Copy const originalArray = [1, 2, 3]; const arrayCopy = [...originalArray]; // Combining Arrays const combinedArray = [...originalArray, ...anotherArray];
7. 機能構成: メソッドの連鎖
強力なデータ変換
const users = [ { name: 'Alice', age: 25, active: true }, { name: 'Bob', age: 30, active: false }, { name: 'Charlie', age: 35, active: true } ]; const processedUsers = users .filter(user => user.active) .map(user => ({ ...user, seniorStatus: user.age >= 30 })) .sort((a, b) => b.age - a.age);
パフォーマンス測定のヒント
シンプルなパフォーマンス追跡
function measurePerformance(fn, label = 'Operation') { const start = performance.now(); fn(); const end = performance.now(); console.log(`${label} took ${end - start} milliseconds`); } // Usage measurePerformance(() => { // Your array operation here }, 'Array Transformation');
ベストプラクティスのチェックリスト
- 適切な反復方法を使用する
- 不変の変換を好む
- 可読性を高めるためのチェーンメソッド
- ネストされたループを避ける
- 負荷の高い計算にはメモ化を使用する
- パフォーマンスのプロファイルと測定
避けるべきよくある間違い
- 不要なコピーの作成
- 不要な場合の配列の変更
- 複雑な変換を多用する
- 小規模な操作ではパフォーマンスを無視します
結論: アレイ最適化の旅
配列メソッドをマスターすることについては次のとおりです。
- パフォーマンスへの影響を理解する
- きれいで読みやすいコードを書く
- 各タスクに適切な方法を選択する
行動喚起
- これらのテクニックを練習してください
- コードをプロファイルする
- 常にパフォーマンスの向上を目指します
ボーナスチャレンジ
マップ、フィルター、リデュースのみを使用して、複雑なデータセットを効率的に変換するデータ処理パイプラインを実装します。
学習リソース
- MDN ウェブ ドキュメント
- パフォーマンス.now()
- 関数型プログラミングのチュートリアル GFG
この投稿についてのコメントをぜひ共有してください....
LinkedIn に接続しましょう
以上がJavaScript 配列メソッド: コードのパフォーマンスと可読性を向上させるの詳細内容です。詳細については、PHP 中国語 Web サイトの他の関連記事を参照してください。

ホットAIツール

Undresser.AI Undress
リアルなヌード写真を作成する AI 搭載アプリ

AI Clothes Remover
写真から衣服を削除するオンライン AI ツール。

Undress AI Tool
脱衣画像を無料で

Clothoff.io
AI衣類リムーバー

AI Hentai Generator
AIヘンタイを無料で生成します。

人気の記事

ホットツール

メモ帳++7.3.1
使いやすく無料のコードエディター

SublimeText3 中国語版
中国語版、とても使いやすい

ゼンドスタジオ 13.0.1
強力な PHP 統合開発環境

ドリームウィーバー CS6
ビジュアル Web 開発ツール

SublimeText3 Mac版
神レベルのコード編集ソフト(SublimeText3)

ホットトピック








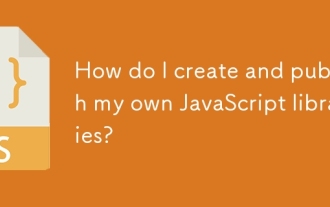
記事では、JavaScriptライブラリの作成、公開、および維持について説明し、計画、開発、テスト、ドキュメント、およびプロモーション戦略に焦点を当てています。
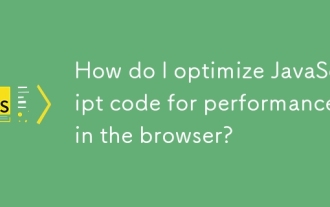
この記事では、ブラウザでJavaScriptのパフォーマンスを最適化するための戦略について説明し、実行時間の短縮、ページの負荷速度への影響を最小限に抑えることに焦点を当てています。
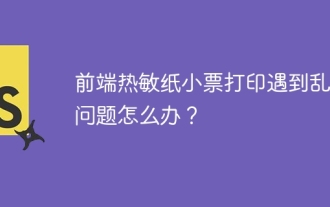
フロントエンドのサーマルペーパーチケット印刷のためのよくある質問とソリューションフロントエンド開発におけるチケット印刷は、一般的な要件です。しかし、多くの開発者が実装しています...
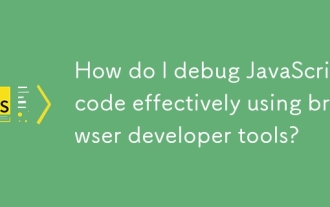
この記事では、ブラウザ開発者ツールを使用した効果的なJavaScriptデバッグについて説明し、ブレークポイントの設定、コンソールの使用、パフォーマンスの分析に焦点を当てています。
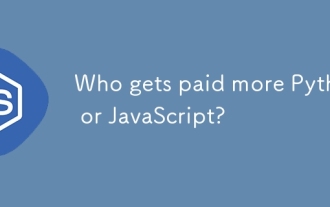
スキルや業界のニーズに応じて、PythonおよびJavaScript開発者には絶対的な給与はありません。 1. Pythonは、データサイエンスと機械学習でさらに支払われる場合があります。 2。JavaScriptは、フロントエンドとフルスタックの開発に大きな需要があり、その給与もかなりです。 3。影響要因には、経験、地理的位置、会社の規模、特定のスキルが含まれます。
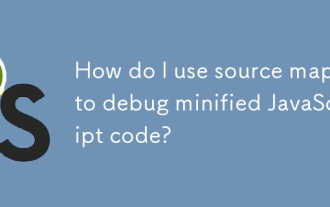
この記事では、ソースマップを使用して、元のコードにマッピングすることにより、Minified JavaScriptをデバッグする方法について説明します。ソースマップの有効化、ブレークポイントの設定、Chrome DevtoolsやWebpackなどのツールの使用について説明します。
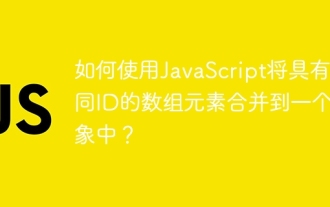
同じIDを持つ配列要素をJavaScriptの1つのオブジェクトにマージする方法は?データを処理するとき、私たちはしばしば同じIDを持つ必要性に遭遇します...
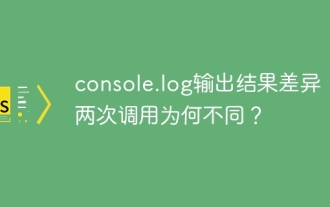
Console.log出力の違いの根本原因に関する詳細な議論。この記事では、Console.log関数の出力結果の違いをコードの一部で分析し、その背後にある理由を説明します。 �...
