Python でメモリ使用量をプロファイリングするにはどうすればよいですか?
Python でのメモリ使用量のプロファイリング
背景:
アルゴリズムとそのパフォーマンスを調査する場合、メモリ効率を高めるためにコードを最適化することが重要になります。これを達成するには、メモリ使用量の監視が不可欠です。
Python メモリ分析:
Python は、ランタイム プロファイリング用の timeit 関数を提供します。ただし、メモリ分析のために、Python 3.4 では、tracemalloc モジュールが導入されています。
tracemalloc の使用:
tracemalloc を使用してメモリ使用量をプロファイリングするには:
import tracemalloc # Start collecting memory usage data tracemalloc.start() # Execute code to analyze memory usage # ... # Take a snapshot of the memory usage data snapshot = tracemalloc.take_snapshot() # Display the top lines with memory consumption display_top(snapshot)
その他アプローチ:
1.バックグラウンド メモリ モニター スレッド:
このアプローチでは、メイン スレッドがコードを実行している間にメモリ使用量を定期的に監視する別のスレッドが作成されます:
import resource import queue from threading import Thread def memory_monitor(command_queue, poll_interval=1): while True: try: command_queue.get(timeout=poll_interval) # Pause the code execution and record the memory usage except Empty: max_rss = resource.getrusage(resource.RUSAGE_SELF).ru_maxrss print('max RSS', max_rss) # Start the memory monitor thread queue = queue.Queue() poll_interval = 0.1 monitor_thread = Thread(target=memory_monitor, args=(queue, poll_interval)) monitor_thread.start()
2。 /proc/self/statm の使用 (Linux のみ):
Linux では、/proc/self/statm ファイルは、次のような詳細なメモリ使用量統計を提供します。
Size Total program size in pages Resident Resident set size in pages Shared Shared pages Text Text (code) pages Lib Shared library pages Data Data/stack pages
以上がPython でメモリ使用量をプロファイリングするにはどうすればよいですか?の詳細内容です。詳細については、PHP 中国語 Web サイトの他の関連記事を参照してください。

ホットAIツール

Undresser.AI Undress
リアルなヌード写真を作成する AI 搭載アプリ

AI Clothes Remover
写真から衣服を削除するオンライン AI ツール。

Undress AI Tool
脱衣画像を無料で

Clothoff.io
AI衣類リムーバー

Video Face Swap
完全無料の AI 顔交換ツールを使用して、あらゆるビデオの顔を簡単に交換できます。

人気の記事

ホットツール

メモ帳++7.3.1
使いやすく無料のコードエディター

SublimeText3 中国語版
中国語版、とても使いやすい

ゼンドスタジオ 13.0.1
強力な PHP 統合開発環境

ドリームウィーバー CS6
ビジュアル Web 開発ツール

SublimeText3 Mac版
神レベルのコード編集ソフト(SublimeText3)

ホットトピック










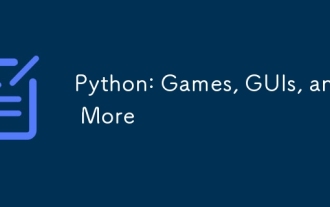
PythonはゲームとGUI開発に優れています。 1)ゲーム開発は、2Dゲームの作成に適した図面、オーディオ、その他の機能を提供し、Pygameを使用します。 2)GUI開発は、TKINTERまたはPYQTを選択できます。 TKINTERはシンプルで使いやすく、PYQTは豊富な機能を備えており、専門能力開発に適しています。
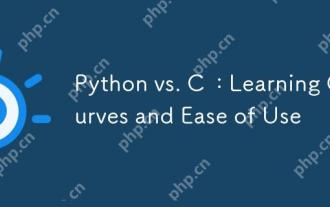
Pythonは学習と使用が簡単ですが、Cはより強力ですが複雑です。 1。Python構文は簡潔で初心者に適しています。動的なタイピングと自動メモリ管理により、使いやすくなりますが、ランタイムエラーを引き起こす可能性があります。 2.Cは、高性能アプリケーションに適した低レベルの制御と高度な機能を提供しますが、学習しきい値が高く、手動メモリとタイプの安全管理が必要です。
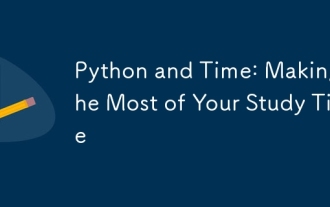
限られた時間でPythonの学習効率を最大化するには、PythonのDateTime、時間、およびスケジュールモジュールを使用できます。 1. DateTimeモジュールは、学習時間を記録および計画するために使用されます。 2。時間モジュールは、勉強と休息の時間を設定するのに役立ちます。 3.スケジュールモジュールは、毎週の学習タスクを自動的に配置します。
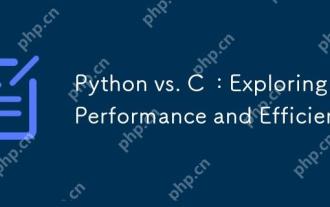
Pythonは開発効率でCよりも優れていますが、Cは実行パフォーマンスが高くなっています。 1。Pythonの簡潔な構文とリッチライブラリは、開発効率を向上させます。 2.Cのコンピレーションタイプの特性とハードウェア制御により、実行パフォーマンスが向上します。選択を行うときは、プロジェクトのニーズに基づいて開発速度と実行効率を比較検討する必要があります。
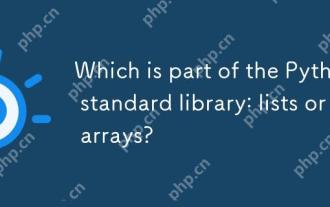
PythonListSarePartOfThestAndardarenot.liestareBuilting-in、versatile、forStoringCollectionsのpythonlistarepart。
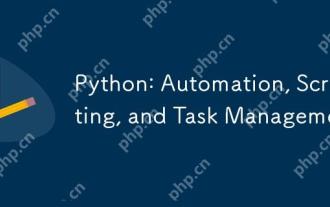
Pythonは、自動化、スクリプト、およびタスク管理に優れています。 1)自動化:OSやShutilなどの標準ライブラリを介してファイルバックアップが実現されます。 2)スクリプトの書き込み:Psutilライブラリを使用してシステムリソースを監視します。 3)タスク管理:スケジュールライブラリを使用してタスクをスケジュールします。 Pythonの使いやすさと豊富なライブラリサポートにより、これらの分野で優先ツールになります。
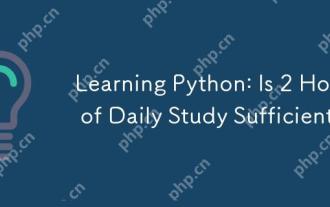
Pythonを1日2時間学ぶだけで十分ですか?それはあなたの目標と学習方法に依存します。 1)明確な学習計画を策定し、2)適切な学習リソースと方法を選択します。3)実践的な実践とレビューとレビューと統合を練習および統合し、統合すると、この期間中にPythonの基本的な知識と高度な機能を徐々に習得できます。
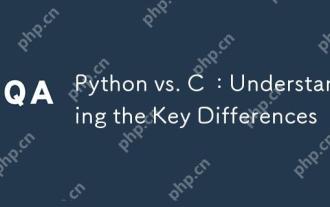
PythonとCにはそれぞれ独自の利点があり、選択はプロジェクトの要件に基づいている必要があります。 1)Pythonは、簡潔な構文と動的タイピングのため、迅速な開発とデータ処理に適しています。 2)Cは、静的なタイピングと手動メモリ管理により、高性能およびシステムプログラミングに適しています。
