最小の驚きの原則 (POLA)
主要な概念
1. 一貫したメソッドの命名
// Bad - Inconsistent naming class UserManager { public function getUser($id) { /* ... */ } public function fetchRole($id) { /* ... */ } public function retrievePermissions($id) { /* ... */ } } // Good - Consistent naming pattern class UserManager { public function getUser($id) { /* ... */ } public function getRole($id) { /* ... */ } public function getPermissions($id) { /* ... */ } }
メソッドに名前を付けるときは、一貫性が非常に重要です。悪い例では、同様の操作に 3 つの異なる動詞 (get、fetch、retrieve) を使用しています。そのため、開発者は同じ種類のアクションに対して異なる用語を覚えておく必要があります。良い例では、すべてのメソッドで一貫して「get」を使用しているため、API がより予測しやすく、覚えやすくなっています。開発者は、データにアクセスする必要がある場合、「get」で始まるメソッドを探す必要があることが直感的にわかります。
2. 期待される戻り値の型
// Bad - Unexpected return types class FileHandler { public function readFile($path) { if (!file_exists($path)) { return false; // Unexpected boolean } return file_get_contents($path); } } // Good - Consistent return types with exceptions class FileHandler { public function readFile($path): string { if (!file_exists($path)) { throw new FileNotFoundException("File not found: {$path}"); } return file_get_contents($path); } }
悪い例では戻り値の型が混在しており、文字列 (ファイルの内容) を返す場合もあれば、ブール値 (false) を返す場合もあります。これにより、戻り値の処理方法が不確実になります。良い例では、文字列の戻り値の型を宣言し、エラーの場合に例外を使用することで型の安全性を確保しています。これは PHP の組み込み動作と一致し、エラー処理がより明示的かつ予測可能になります。
3. 予測可能なパラメータの順序
// Bad - Inconsistent parameter order class OrderProcessor { public function createOrder($items, $userId, $date) { /* ... */ } public function updateOrder($date, $orderId, $items) { /* ... */ } } // Good - Consistent parameter order class OrderProcessor { public function createOrder($userId, $items, $date) { /* ... */ } public function updateOrder($orderId, $items, $date) { /* ... */ } }
パラメータの順序は、同様のメソッド間で一貫している必要があります。悪い例では、同様のパラメータが異なる位置に配置されており、API が混乱しています。良い例では、論理的な順序が維持されています。最初に識別子 (userId/orderId)、次にメイン データ (項目)、そして最後にオプション/メタデータ パラメーター (日付) が続きます。このパターンは、PHP フレームワークの一般的な規則と一致し、API をより直感的にします。
4. 明確なメソッドの動作
// Bad - Ambiguous behavior class Cart { public function add($product) { // Sometimes creates new item, sometimes updates quantity // Unpredictable! } } // Good - Clear, single responsibility class Cart { public function addNewItem($product) { /* ... */ } public function updateQuantity($productId, $quantity) { /* ... */ } }
メソッドには明確な単一の責任がある必要があります。悪い例の「add」メソッドはあいまいです。新しい項目を追加するか、既存の項目を更新する可能性があります。良い例では、これを明確な名前と目的を持つ 2 つの異なるメソッドに分割しています。これにより、コードの動作が予測可能になり、単一責任原則 (SRP) に従います。
5. 一貫したエラー処理
class PaymentProcessor { public function processPayment(float $amount): PaymentResult { try { // Process payment return new PaymentResult(true, 'Payment successful'); } catch (PaymentException $e) { // Always handle errors the same way throw new PaymentFailedException($e->getMessage()); } } }
この例は、例外による一貫したエラー処理を示しています。何か問題が発生した場合、別のタイプを返したりエラー コードを使用したりする代わりに、常に特定の例外タイプ (PaymentFailedException) をスローします。これにより、アプリケーション全体でのエラー処理の予測可能なパターンが作成されます。このメソッドは、成功した場合に専用の PaymentResult オブジェクトも使用し、型の一貫性を維持します。
これらの各プラクティスは、PHP 開発の一般的なパターンと規則に基づいて開発者が期待する方法で動作するため、より保守しやすく、理解しやすく、バグが発生しにくいコードに貢献します。
以上が最小の驚きの原則 (POLA)の詳細内容です。詳細については、PHP 中国語 Web サイトの他の関連記事を参照してください。

ホットAIツール

Undresser.AI Undress
リアルなヌード写真を作成する AI 搭載アプリ

AI Clothes Remover
写真から衣服を削除するオンライン AI ツール。

Undress AI Tool
脱衣画像を無料で

Clothoff.io
AI衣類リムーバー

Video Face Swap
完全無料の AI 顔交換ツールを使用して、あらゆるビデオの顔を簡単に交換できます。

人気の記事

ホットツール

メモ帳++7.3.1
使いやすく無料のコードエディター

SublimeText3 中国語版
中国語版、とても使いやすい

ゼンドスタジオ 13.0.1
強力な PHP 統合開発環境

ドリームウィーバー CS6
ビジュアル Web 開発ツール

SublimeText3 Mac版
神レベルのコード編集ソフト(SublimeText3)

ホットトピック










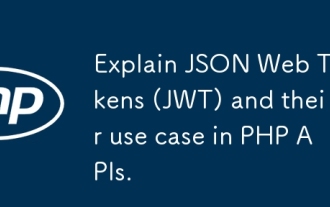
JWTは、JSONに基づくオープン標準であり、主にアイデンティティ認証と情報交換のために、当事者間で情報を安全に送信するために使用されます。 1。JWTは、ヘッダー、ペイロード、署名の3つの部分で構成されています。 2。JWTの実用的な原則には、JWTの生成、JWTの検証、ペイロードの解析という3つのステップが含まれます。 3. PHPでの認証にJWTを使用する場合、JWTを生成および検証でき、ユーザーの役割と許可情報を高度な使用に含めることができます。 4.一般的なエラーには、署名検証障害、トークンの有効期限、およびペイロードが大きくなります。デバッグスキルには、デバッグツールの使用とロギングが含まれます。 5.パフォーマンスの最適化とベストプラクティスには、適切な署名アルゴリズムの使用、有効期間を合理的に設定することが含まれます。

php8.1の列挙関数は、指定された定数を定義することにより、コードの明確さとタイプの安全性を高めます。 1)列挙は、整数、文字列、またはオブジェクトであり、コードの読みやすさとタイプの安全性を向上させることができます。 2)列挙はクラスに基づいており、トラバーサルや反射などのオブジェクト指向の機能をサポートします。 3)列挙を比較と割り当てに使用して、タイプの安全性を確保できます。 4)列挙は、複雑なロジックを実装するためのメソッドの追加をサポートします。 5)厳密なタイプのチェックとエラー処理は、一般的なエラーを回避できます。 6)列挙は魔法の価値を低下させ、保守性を向上させますが、パフォーマンスの最適化に注意してください。
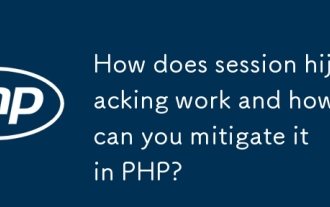
セッションハイジャックは、次の手順で達成できます。1。セッションIDを取得します。2。セッションIDを使用します。3。セッションをアクティブに保ちます。 PHPでのセッションハイジャックを防ぐための方法には次のものが含まれます。1。セッション_regenerate_id()関数を使用して、セッションIDを再生します。2。データベースを介してストアセッションデータを3。
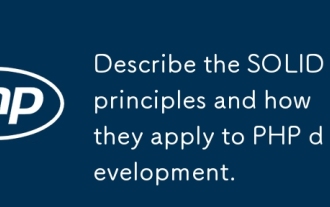
PHP開発における固体原理の適用には、次のものが含まれます。1。単一責任原則(SRP):各クラスは1つの機能のみを担当します。 2。オープンおよびクローズ原理(OCP):変更は、変更ではなく拡張によって達成されます。 3。Lischの代替原則(LSP):サブクラスは、プログラムの精度に影響を与えることなく、基本クラスを置き換えることができます。 4。インターフェイス分離原理(ISP):依存関係や未使用の方法を避けるために、細粒インターフェイスを使用します。 5。依存関係の反転原理(DIP):高レベルのモジュールと低レベルのモジュールは抽象化に依存し、依存関係噴射を通じて実装されます。
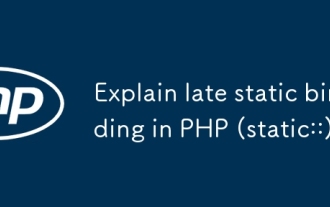
静的結合(静的::) PHPで後期静的結合(LSB)を実装し、クラスを定義するのではなく、静的コンテキストで呼び出しクラスを参照できるようにします。 1)解析プロセスは実行時に実行されます。2)継承関係のコールクラスを検索します。3)パフォーマンスオーバーヘッドをもたらす可能性があります。
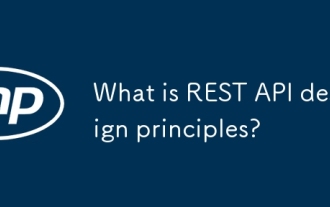
Restapiの設計原則には、リソース定義、URI設計、HTTPメソッドの使用、ステータスコードの使用、バージョンコントロール、およびHATEOASが含まれます。 1。リソースは名詞で表され、階層で維持される必要があります。 2。HTTPメソッドは、GETを使用してリソースを取得するなど、セマンティクスに準拠する必要があります。 3.ステータスコードは、404など、リソースが存在しないことを意味します。 4。バージョン制御は、URIまたはヘッダーを介して実装できます。 5。それに応じてリンクを介してhateoasブーツクライアント操作をブーツします。
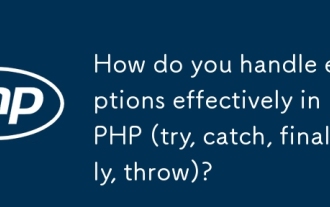
PHPでは、Try、Catch、最後にキーワードをスローすることにより、例外処理が達成されます。 1)TRYブロックは、例外をスローする可能性のあるコードを囲みます。 2)キャッチブロックは例外を処理します。 3)最後にブロックは、コードが常に実行されることを保証します。 4)スローは、例外を手動でスローするために使用されます。これらのメカニズムは、コードの堅牢性と保守性を向上させるのに役立ちます。
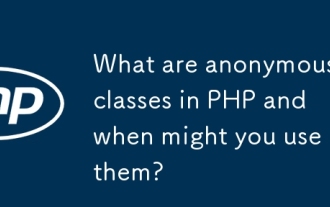
PHPの匿名クラスの主な機能は、1回限りのオブジェクトを作成することです。 1.匿名クラスでは、名前のないクラスをコードで直接定義することができます。これは、一時的な要件に適しています。 2。クラスを継承したり、インターフェイスを実装して柔軟性を高めることができます。 3.使用時にパフォーマンスとコードの読みやすさに注意し、同じ匿名のクラスを繰り返し定義しないようにします。
