libsodium を使用して PHP 文字列を安全に暗号化および復号化する方法
PHP 文字列を暗号化および復号化する方法
暗号化について議論するときは、暗号化と認証を区別することが重要です。通常は、暗号化とメッセージ認証コード (MAC) を組み合わせた認証付き暗号化が推奨されます。セキュリティを確保するために、独自の暗号化の実装を避け、代わりに暗号化の専門家によって開発およびレビューされた十分に確立されたライブラリに依存することを強くお勧めします。
libsodium を使用した暗号化手順:
- CTR モードで AES を使用してメッセージを暗号化するか、認証を処理する GCM を使用します。
- HMAC-SHA-256 を使用して暗号文を認証し、MAC が IV と暗号文の両方をカバーしていることを確認します。
libsodium を使用した復号化手順:
- の MAC を確認します。受信した MAC に対する暗号文。検証が失敗し、データの破損が示された場合は中止します。
- 認証された暗号化方式を使用してメッセージを復号化します。
追加の考慮事項:
- 暗号化の前に圧縮することは避けてください。
- 適切な文字列操作を使用してください。拡張文字セットを処理する関数。
- 暗号的に安全な擬似乱数生成器 (CSPRNG) を使用して IV を安全に生成します。
- 脆弱性を防ぐために、MAC の前に常に暗号化を実行します。
- 注意してください特定のエンコーディングで発生する可能性のある情報漏洩の問題メソッド。
libsodium を使用したコード例:
<?php declare(strict_types=1); /** * Encrypt a message * * @param string $message - message to encrypt * @param string $key - encryption key * @return string * @throws RangeException */ function safeEncrypt(string $message, string $key): string { if (mb_strlen($key, '8bit') !== SODIUM_CRYPTO_SECRETBOX_KEYBYTES) { throw new RangeException('Key is not the correct size (must be 32 bytes).'); } $nonce = random_bytes(SODIUM_CRYPTO_SECRETBOX_NONCEBYTES); $cipher = base64_encode( $nonce. sodium_crypto_secretbox( $message, $nonce, $key ) ); sodium_memzero($message); sodium_memzero($key); return $cipher; } /** * Decrypt a message * * @param string $encrypted - message encrypted with safeEncrypt() * @param string $key - encryption key * @return string * @throws Exception */ function safeDecrypt(string $encrypted, string $key): string { $decoded = base64_decode($encrypted); $nonce = mb_substr($decoded, 0, SODIUM_CRYPTO_SECRETBOX_NONCEBYTES, '8bit'); $ciphertext = mb_substr($decoded, SODIUM_CRYPTO_SECRETBOX_NONCEBYTES, null, '8bit'); $plain = sodium_crypto_secretbox_open( $ciphertext, $nonce, $key ); if (!is_string($plain)) { throw new Exception('Invalid MAC'); } sodium_memzero($ciphertext); sodium_memzero($key); return $plain; } ?>
以上がlibsodium を使用して PHP 文字列を安全に暗号化および復号化する方法の詳細内容です。詳細については、PHP 中国語 Web サイトの他の関連記事を参照してください。

ホットAIツール

Undresser.AI Undress
リアルなヌード写真を作成する AI 搭載アプリ

AI Clothes Remover
写真から衣服を削除するオンライン AI ツール。

Undress AI Tool
脱衣画像を無料で

Clothoff.io
AI衣類リムーバー

AI Hentai Generator
AIヘンタイを無料で生成します。

人気の記事

ホットツール

メモ帳++7.3.1
使いやすく無料のコードエディター

SublimeText3 中国語版
中国語版、とても使いやすい

ゼンドスタジオ 13.0.1
強力な PHP 統合開発環境

ドリームウィーバー CS6
ビジュアル Web 開発ツール

SublimeText3 Mac版
神レベルのコード編集ソフト(SublimeText3)

ホットトピック








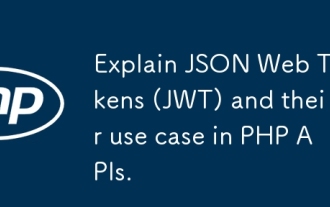
JWTは、JSONに基づくオープン標準であり、主にアイデンティティ認証と情報交換のために、当事者間で情報を安全に送信するために使用されます。 1。JWTは、ヘッダー、ペイロード、署名の3つの部分で構成されています。 2。JWTの実用的な原則には、JWTの生成、JWTの検証、ペイロードの解析という3つのステップが含まれます。 3. PHPでの認証にJWTを使用する場合、JWTを生成および検証でき、ユーザーの役割と許可情報を高度な使用に含めることができます。 4.一般的なエラーには、署名検証障害、トークンの有効期限、およびペイロードが大きくなります。デバッグスキルには、デバッグツールの使用とロギングが含まれます。 5.パフォーマンスの最適化とベストプラクティスには、適切な署名アルゴリズムの使用、有効期間を合理的に設定することが含まれます。
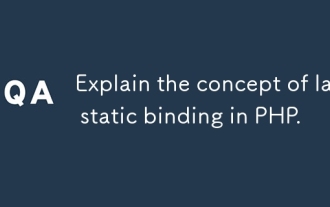
記事では、PHP 5.3で導入されたPHPの後期静的結合(LSB)について説明し、より柔軟な継承を求める静的メソッドコールのランタイム解像度を可能にします。 LSBの実用的なアプリケーションと潜在的なパフォーマ
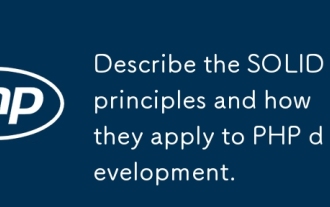
PHP開発における固体原理の適用には、次のものが含まれます。1。単一責任原則(SRP):各クラスは1つの機能のみを担当します。 2。オープンおよびクローズ原理(OCP):変更は、変更ではなく拡張によって達成されます。 3。Lischの代替原則(LSP):サブクラスは、プログラムの精度に影響を与えることなく、基本クラスを置き換えることができます。 4。インターフェイス分離原理(ISP):依存関係や未使用の方法を避けるために、細粒インターフェイスを使用します。 5。依存関係の反転原理(DIP):高レベルのモジュールと低レベルのモジュールは抽象化に依存し、依存関係噴射を通じて実装されます。
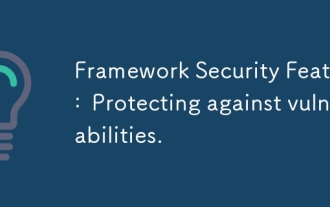
記事では、入力検証、認証、定期的な更新など、脆弱性から保護するためのフレームワークの重要なセキュリティ機能について説明します。
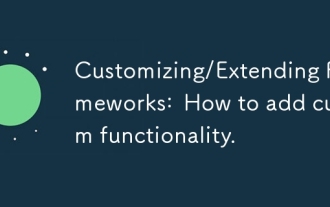
この記事では、フレームワークにカスタム機能を追加し、アーキテクチャの理解、拡張ポイントの識別、統合とデバッグのベストプラクティスに焦点を当てています。
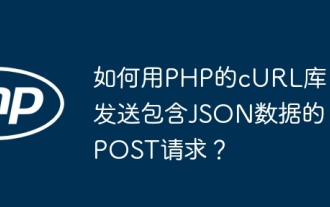
PHP開発でPHPのCurlライブラリを使用してJSONデータを送信すると、外部APIと対話する必要があることがよくあります。一般的な方法の1つは、Curlライブラリを使用して投稿を送信することです。
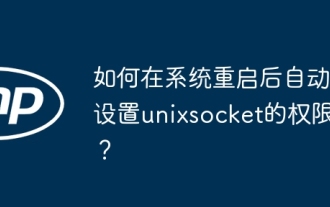
システムが再起動した後、UnixSocketの権限を自動的に設定する方法。システムが再起動するたびに、UnixSocketの許可を変更するために次のコマンドを実行する必要があります:sudo ...
