JavaScript はプリミティブ型とオブジェクトを使用した値渡しと参照渡しをどのように処理しますか?
JavaScript 値渡しと参照渡し
JavaScript では、すべての変数が値によって渡されます。つまり、元の値のコピーが返されます。が作成され、関数に渡されます。ただし、値が配列やオブジェクト リテラルなどのオブジェクトである場合、コピーは元のオブジェクトへの参照になります。
関数の引数への影響
- プリミティブ: プリミティブ (数値、文字列など) が関数に渡されるとき、関数内でその値に加えられた変更は、関数のローカル スコープに限定され、関数外の元の変数には影響しません。
- オブジェクト参照: オブジェクト参照が渡されるとき関数に変更を加えると、関数内でオブジェクトのプロパティに加えられた変更は、関数の外であっても元のオブジェクトに反映されます。
への影響関数外の変数
- 参照渡し: オブジェクト参照は参照によって渡されます。つまり、関数内でオブジェクトのプロパティに加えられた変更は、元のオブジェクトのプロパティに影響します。ただし、関数内で参照自体に加えられた変更 (再割り当てなど) は、元のプロパティには影響しません。 variable.
- 値渡し: 値によって渡されるプリミティブ値とオブジェクト参照は、関数の外の元の変数には影響しません。
例
function f(a, b, c) { a = 3; // Reassignment changes the local variable only. b.push("foo"); // Property change affects the original object. c.first = false; // Property change affects the original object. } const x = 4; let y = ["eeny", "miny", "mo"]; let z = { first: true }; f(x, y, z); console.log(x, y, z.first); // Output: 4, ["eeny", "miny", "mo", "foo"], false
上記の例では、 b オブジェクトと c オブジェクトへの変更は次のとおりです。元のオブジェクトに反映されますが、 a の再割り当ては効果がありません。
詳細な例:
function f() { const a = ["1", "2", { foo: "bar" }]; const b = a[1]; // Copy the reference to the original array element a[1] = "4"; // Change the value in the original array console.log(b); // Output: "2" (Original value of the copied reference) }
最初の例では、 a が変更されても、 b は参照のコピーであるため、元の値を保持しています。
function f() { const a = [{ yellow: "blue" }, { red: "cyan" }, { green: "magenta" }]; const b = a[1]; // Copy the reference to the original object a[1].red = "tan"; // Change the property in the original object console.log(b.red); // Output: "tan" (Property change is reflected in both variables) b.red = "black"; // Change the property through the reference console.log(a[1].red); // Output: "black" (Property change is reflected in both variables) }
2 番目の例では、変更が行われています。 a[1].red への変更は、同じオブジェクト参照を共有するため、a と b の両方に影響します。
独立したコピーの作成
オブジェクトの完全に独立したコピーを作成するにはでは、JSON.parse() メソッドと JSON.stringify() メソッドを使用して、それぞれオブジェクトを逆シリアル化およびシリアル化できます。例:
const originalObject = { foo: "bar" }; const independentCopy = JSON.parse(JSON.stringify(originalObject));
以上がJavaScript はプリミティブ型とオブジェクトを使用した値渡しと参照渡しをどのように処理しますか?の詳細内容です。詳細については、PHP 中国語 Web サイトの他の関連記事を参照してください。

ホットAIツール

Undresser.AI Undress
リアルなヌード写真を作成する AI 搭載アプリ

AI Clothes Remover
写真から衣服を削除するオンライン AI ツール。

Undress AI Tool
脱衣画像を無料で

Clothoff.io
AI衣類リムーバー

Video Face Swap
完全無料の AI 顔交換ツールを使用して、あらゆるビデオの顔を簡単に交換できます。

人気の記事

ホットツール

メモ帳++7.3.1
使いやすく無料のコードエディター

SublimeText3 中国語版
中国語版、とても使いやすい

ゼンドスタジオ 13.0.1
強力な PHP 統合開発環境

ドリームウィーバー CS6
ビジュアル Web 開発ツール

SublimeText3 Mac版
神レベルのコード編集ソフト(SublimeText3)

ホットトピック










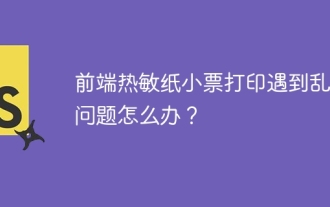
フロントエンドのサーマルペーパーチケット印刷のためのよくある質問とソリューションフロントエンド開発におけるチケット印刷は、一般的な要件です。しかし、多くの開発者が実装しています...
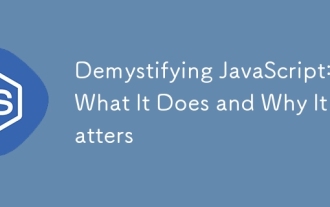
JavaScriptは現代のWeb開発の基礎であり、その主な機能には、イベント駆動型のプログラミング、動的コンテンツ生成、非同期プログラミングが含まれます。 1)イベント駆動型プログラミングにより、Webページはユーザー操作に応じて動的に変更できます。 2)動的コンテンツ生成により、条件に応じてページコンテンツを調整できます。 3)非同期プログラミングにより、ユーザーインターフェイスがブロックされないようにします。 JavaScriptは、Webインタラクション、シングルページアプリケーション、サーバー側の開発で広く使用されており、ユーザーエクスペリエンスとクロスプラットフォーム開発の柔軟性を大幅に改善しています。
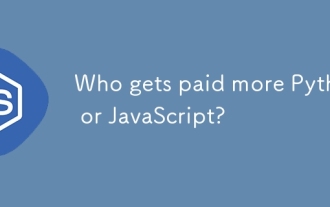
スキルや業界のニーズに応じて、PythonおよびJavaScript開発者には絶対的な給与はありません。 1. Pythonは、データサイエンスと機械学習でさらに支払われる場合があります。 2。JavaScriptは、フロントエンドとフルスタックの開発に大きな需要があり、その給与もかなりです。 3。影響要因には、経験、地理的位置、会社の規模、特定のスキルが含まれます。
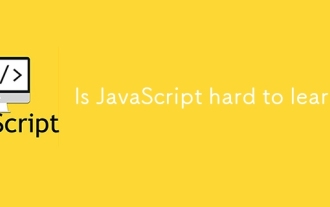
JavaScriptを学ぶことは難しくありませんが、挑戦的です。 1)変数、データ型、関数などの基本概念を理解します。2)非同期プログラミングをマスターし、イベントループを通じて実装します。 3)DOM操作を使用し、非同期リクエストを処理することを約束します。 4)一般的な間違いを避け、デバッグテクニックを使用します。 5)パフォーマンスを最適化し、ベストプラクティスに従ってください。
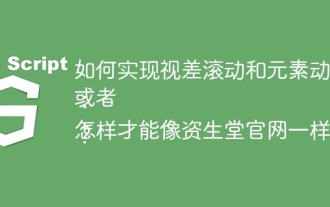
この記事の視差スクロールと要素のアニメーション効果の実現に関する議論では、Shiseidoの公式ウェブサイト(https://www.shisido.co.co.jp/sb/wonderland/)と同様の達成方法について説明します。
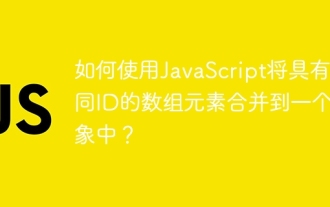
同じIDを持つ配列要素をJavaScriptの1つのオブジェクトにマージする方法は?データを処理するとき、私たちはしばしば同じIDを持つ必要性に遭遇します...
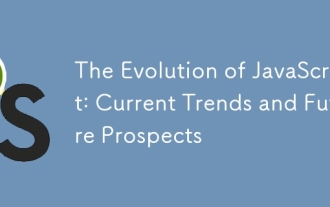
JavaScriptの最新トレンドには、TypeScriptの台頭、最新のフレームワークとライブラリの人気、WebAssemblyの適用が含まれます。将来の見通しは、より強力なタイプシステム、サーバー側のJavaScriptの開発、人工知能と機械学習の拡大、およびIoTおよびEDGEコンピューティングの可能性をカバーしています。
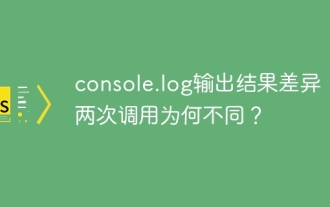
Console.log出力の違いの根本原因に関する詳細な議論。この記事では、Console.log関数の出力結果の違いをコードの一部で分析し、その背後にある理由を説明します。 �...
