JavaScript におけるデバウンスとスロットリング: 関数呼び出しを最適化してパフォーマンスを向上させる
JavaScript におけるデバウンスとスロットリングについて
デバウンスとスロットルは、関数の実行速度を制御するために JavaScript で使用される 2 つの重要なテクニックです。これらの手法は、特にユーザー入力の処理、イベントのスクロール、イベントのサイズ変更などのシナリオで、パフォーマンスを最適化するためによく使用されます。どちらも関数呼び出しの頻度を制限するために使用されますが、動作方法は異なります。
1. デバウンス
デバウンス は、最後のイベントから一定の時間が経過した後にのみ関数が呼び出されるようにします。つまり、ユーザーがテキスト フィールドへの入力やウィンドウのサイズ変更などのアクションの実行を完了するまで、関数の実行が遅延されます。これは、ユーザーが検索バーに入力しているときなど、関数が頻繁に呼び出されないようにする必要があるシナリオで特に役立ちます。
デバウンスの仕組み:
- イベントがトリガーされると、関数呼び出しは指定された時間だけ遅延されます。
- 遅延時間が経過する前にイベントが再度トリガーされると、前の関数呼び出しはキャンセルされ、遅延タイマーがリセットされます。
- この関数は、それ以上のイベントが発生せずに遅延時間が経過した場合にのみ実行されます。
デバウンスの例:
function searchQuery(query) { console.log(`Searching for: ${query}`); } function debounce(func, delay) { let timeout; return function (...args) { clearTimeout(timeout); timeout = setTimeout(() => { func.apply(this, args); }, delay); }; } const debouncedSearch = debounce(searchQuery, 500); // Simulating typing events debouncedSearch("JavaScript"); debouncedSearch("JavaScript debouncing"); debouncedSearch("Debouncing function"); // Only this will be logged after 500ms
この例では:
- debouncedSearch は、別の呼び出しが行われずに 500 ミリ秒が経過した後にのみ searchQuery を呼び出します。
- これにより、文字が入力されるたびに検索関数が呼び出されることがなくなります。
デバウンスの使用例:
- 検索入力: リアルタイムの検索候補を実装する場合。
- ウィンドウのサイズ変更: サイズ変更イベントごとにレイアウトの再計算がトリガーされるのを回避します。
- スクロール イベント: 連続スクロール中に、特に無限スクロール シナリオで関数が起動されないようにします。
2. スロットル
スロットリング では、イベントが何回トリガーされたとしても、関数は指定された間隔ごとに最大 1 回呼び出されます。これは、ユーザーが特定の期間内にウィンドウをスクロールまたはサイズ変更できる回数を制限するなど、関数呼び出しの頻度を制限する場合に便利です。
スロットリングの仕組み:
- この関数は、イベントが初めてトリガーされるとすぐに実行されます。
- その後、イベントがより頻繁にトリガーされる場合でも、イベントは最大でも n ミリ秒ごとに 1 回実行されます。
スロットルの例:
function searchQuery(query) { console.log(`Searching for: ${query}`); } function debounce(func, delay) { let timeout; return function (...args) { clearTimeout(timeout); timeout = setTimeout(() => { func.apply(this, args); }, delay); }; } const debouncedSearch = debounce(searchQuery, 500); // Simulating typing events debouncedSearch("JavaScript"); debouncedSearch("JavaScript debouncing"); debouncedSearch("Debouncing function"); // Only this will be logged after 500ms
この例では:
- throttledScroll は、その間に発生したスクロール イベントの数に関係なく、logScrollEvent が最大で 1 秒に 1 回呼び出されることを保証します。
- 関数は初回のみすぐに実行され、それ以降の呼び出しは間隔を維持するために遅延されます。
スロットルの使用例:
- スクロール イベント: スクロール中に関数が呼び出される頻度を制限します (画像の遅延読み込みなど)。
- サイズ変更イベント: ウィンドウのサイズ変更関数が呼び出される回数を最適化します。
- マウスの動き: マウスの高速移動中の連続実行を防止します。
3. デバウンスとスロットリング: 主な違い
Feature | Debouncing | Throttling |
---|---|---|
Function Execution | Executes after a delay when events stop | Executes at a fixed interval, no matter how many events occur |
Use Case | Ideal for events that occur frequently but should trigger once after some idle time (e.g., input fields, search bars) | Ideal for events that fire continuously (e.g., scroll, resize) but should be limited to a fixed interval |
Example Scenario | Search bar input where suggestions are updated only after the user stops typing for a certain period | Scroll events where a function should only run once every few seconds, even if the user scrolls frequently |
Execution Frequency | Executes only once after the event stops firing | Executes periodically, based on the interval set |
Effectiveness | Prevents unnecessary executions during rapid event firing | Controls the frequency of function executions, even during continuous events |
4. 実践例: デバウンスとスロットリングを一緒に使用する
アプリケーションを最適化するために両方の手法が必要な場合は、デバウンスとスロットルを組み合わせることができます。たとえば、検索クエリをデバウンスしながら、スクロール イベントを抑制したい場合があります。
function searchQuery(query) { console.log(`Searching for: ${query}`); } function debounce(func, delay) { let timeout; return function (...args) { clearTimeout(timeout); timeout = setTimeout(() => { func.apply(this, args); }, delay); }; } const debouncedSearch = debounce(searchQuery, 500); // Simulating typing events debouncedSearch("JavaScript"); debouncedSearch("JavaScript debouncing"); debouncedSearch("Debouncing function"); // Only this will be logged after 500ms
この例では:
- スクロール イベントは、1 秒に 1 回だけトリガーされるように調整されます。
- 検索入力はデバウンスされ、非アクティブ状態が 500 ミリ秒続いた後にのみトリガーされます。
結論
- デバウンスは、イベントがトリガーされずに一定時間が経過した後に関数が実行されることを保証し、検索入力やサイズ変更などのシナリオに最適です。
- スロットリングは、特定の時間枠内で関数を実行できる回数を制限するため、スクロールやウィンドウのサイズ変更などのイベントに役立ちます。
どちらの手法も、特にイベントが急速に発生する場合に、パフォーマンスを最適化し、不必要な実行を防ぐのに役立ちます。
こんにちは、アバイ・シン・カタヤットです!
私はフロントエンドとバックエンドの両方のテクノロジーの専門知識を持つフルスタック開発者です。私はさまざまなプログラミング言語やフレームワークを使用して、効率的でスケーラブルでユーザーフレンドリーなアプリケーションを構築しています。
ビジネス用メールアドレス kaashshorts28@gmail.com までお気軽にご連絡ください。
以上がJavaScript におけるデバウンスとスロットリング: 関数呼び出しを最適化してパフォーマンスを向上させるの詳細内容です。詳細については、PHP 中国語 Web サイトの他の関連記事を参照してください。

ホットAIツール

Undresser.AI Undress
リアルなヌード写真を作成する AI 搭載アプリ

AI Clothes Remover
写真から衣服を削除するオンライン AI ツール。

Undress AI Tool
脱衣画像を無料で

Clothoff.io
AI衣類リムーバー

Video Face Swap
完全無料の AI 顔交換ツールを使用して、あらゆるビデオの顔を簡単に交換できます。

人気の記事

ホットツール

メモ帳++7.3.1
使いやすく無料のコードエディター

SublimeText3 中国語版
中国語版、とても使いやすい

ゼンドスタジオ 13.0.1
強力な PHP 統合開発環境

ドリームウィーバー CS6
ビジュアル Web 開発ツール

SublimeText3 Mac版
神レベルのコード編集ソフト(SublimeText3)

ホットトピック










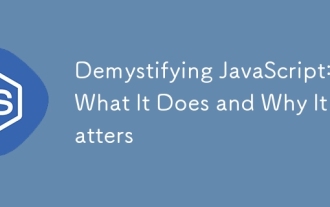
JavaScriptは現代のWeb開発の基礎であり、その主な機能には、イベント駆動型のプログラミング、動的コンテンツ生成、非同期プログラミングが含まれます。 1)イベント駆動型プログラミングにより、Webページはユーザー操作に応じて動的に変更できます。 2)動的コンテンツ生成により、条件に応じてページコンテンツを調整できます。 3)非同期プログラミングにより、ユーザーインターフェイスがブロックされないようにします。 JavaScriptは、Webインタラクション、シングルページアプリケーション、サーバー側の開発で広く使用されており、ユーザーエクスペリエンスとクロスプラットフォーム開発の柔軟性を大幅に改善しています。
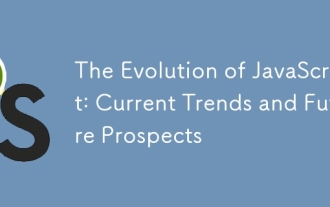
JavaScriptの最新トレンドには、TypeScriptの台頭、最新のフレームワークとライブラリの人気、WebAssemblyの適用が含まれます。将来の見通しは、より強力なタイプシステム、サーバー側のJavaScriptの開発、人工知能と機械学習の拡大、およびIoTおよびEDGEコンピューティングの可能性をカバーしています。
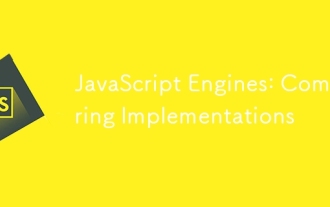
さまざまなJavaScriptエンジンは、各エンジンの実装原則と最適化戦略が異なるため、JavaScriptコードを解析および実行するときに異なる効果をもたらします。 1。語彙分析:ソースコードを語彙ユニットに変換します。 2。文法分析:抽象的な構文ツリーを生成します。 3。最適化とコンパイル:JITコンパイラを介してマシンコードを生成します。 4。実行:マシンコードを実行します。 V8エンジンはインスタントコンピレーションと非表示クラスを通じて最適化され、Spidermonkeyはタイプ推論システムを使用して、同じコードで異なるパフォーマンスパフォーマンスをもたらします。
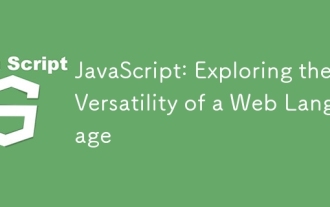
JavaScriptは、現代のWeb開発のコア言語であり、その多様性と柔軟性に広く使用されています。 1)フロントエンド開発:DOM操作と最新のフレームワーク(React、Vue.JS、Angularなど)を通じて、動的なWebページとシングルページアプリケーションを構築します。 2)サーバー側の開発:node.jsは、非ブロッキングI/Oモデルを使用して、高い並行性とリアルタイムアプリケーションを処理します。 3)モバイルおよびデスクトップアプリケーション開発:クロスプラットフォーム開発は、反応および電子を通じて実現され、開発効率を向上させます。
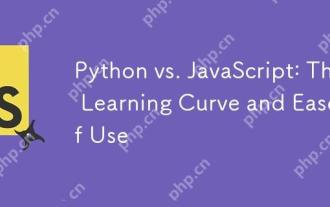
Pythonは、スムーズな学習曲線と簡潔な構文を備えた初心者により適しています。 JavaScriptは、急な学習曲線と柔軟な構文を備えたフロントエンド開発に適しています。 1。Python構文は直感的で、データサイエンスやバックエンド開発に適しています。 2。JavaScriptは柔軟で、フロントエンドおよびサーバー側のプログラミングで広く使用されています。
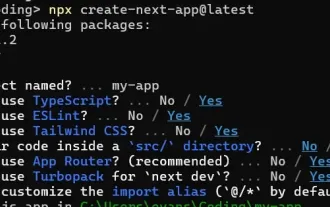
この記事では、許可によって保護されたバックエンドとのフロントエンド統合を示し、next.jsを使用して機能的なedtech SaaSアプリケーションを構築します。 FrontEndはユーザーのアクセス許可を取得してUIの可視性を制御し、APIリクエストがロールベースに付着することを保証します
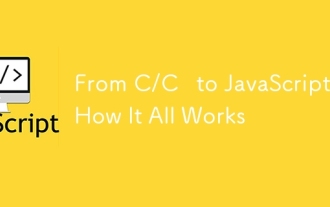
C/CからJavaScriptへのシフトには、動的なタイピング、ゴミ収集、非同期プログラミングへの適応が必要です。 1)C/Cは、手動メモリ管理を必要とする静的に型付けられた言語であり、JavaScriptは動的に型付けされ、ごみ収集が自動的に処理されます。 2)C/Cはマシンコードにコンパイルする必要がありますが、JavaScriptは解釈言語です。 3)JavaScriptは、閉鎖、プロトタイプチェーン、約束などの概念を導入します。これにより、柔軟性と非同期プログラミング機能が向上します。
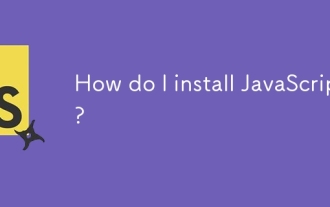
JavaScriptは、最新のブラウザにすでに組み込まれているため、インストールを必要としません。開始するには、テキストエディターとブラウザのみが必要です。 1)ブラウザ環境では、タグを介してHTMLファイルを埋め込んで実行します。 2)node.js環境では、node.jsをダウンロードしてインストールした後、コマンドラインを介してJavaScriptファイルを実行します。
