C ラムダでムーブ キャプチャを実装するにはどうすればよいですか?
ラムダでのムーブ キャプチャ
質問:
また、ムーブ キャプチャを実装するにはどうすればよいですか? C 11 ラムダでは右辺値参照として知られていますか?例:
std::unique_ptr<int> myPointer(new int); std::function<void(void)> example = [std::move(myPointer)] { *myPointer = 4; };
答え:
C 14 の一般化ラムダキャプチャ
C 14 では、一般化ラムダキャプチャでは移動キャプチャが可能になります。このコードは現在有効です:
using namespace std; auto u = make_unique<some_type>(some, parameters); go.run([u = move(u)] { do_something_with(u); });
オブジェクトをラムダから別の関数に移動するには、ラムダを可変にします:
go.run([u = move(u)] mutable { do_something_with(std::move(u)); });
C 11 での移動キャプチャの回避策
ヘルパー関数 make_rref を使用すると、移動キャプチャを容易にできます。その実装は次のとおりです:
#include <cassert> #include <memory> #include <utility> template <typename T> struct rref_impl { rref_impl() = delete; rref_impl(T&& x) : x{std::move(x)} {} rref_impl(rref_impl& other) : x{std::move(other.x)}, isCopied{true} { assert(other.isCopied == false); } rref_impl(rref_impl&& other) : x{std::move(other.x)}, isCopied{std::move(other.isCopied)} { } rref_impl& operator=(rref_impl other) = delete; T& operator&&() { return std::move(x); } private: T x; bool isCopied = false; }; template<typename T> rref_impl<T> make_rref(T&& x) { return rref_impl<T>{std::move(x)}; }
make_rref のテスト ケース:
int main() { std::unique_ptr<int> p{new int(0)}; auto rref = make_rref(std::move(p)); auto lambda = [rref]() mutable -> std::unique_ptr<int> { return rref.move(); }; assert(lambda()); assert(!lambda()); }
C 11 での一般化ラムダ キャプチャのエミュレーション
別の回避策はcapture()によって提供されますfunction:
#include <cassert> #include <memory> int main() { std::unique_ptr<int> p{new int(0)}; auto lambda = capture(std::move(p), [](std::unique_ptr<int>& p) { return std::move(p); }); assert(lambda()); assert(!lambda()); }
キャプチャは次のように実装されます:
#include <utility> template <typename T, typename F> class capture_impl { T x; F f; public: capture_impl(T&& x, F&& f) : x{std::forward<T>(x)}, f{std::forward<F>(f)} {} template <typename ...Ts> auto operator()(Ts&& ...args) -> decltype(f(x, std::forward<Ts>(args)...)) { return f(x, std::forward<Ts>(args)...); } template <typename ...Ts> auto operator()(Ts&& ...args) const -> decltype(f(x, std::forward<Ts>(args)...)) { return f(x, std::forward<Ts>(args)...); } }; template <typename T, typename F> capture_impl<T, F> capture(T&& x, F&& f) { return capture_impl<T, F>( std::forward<T>(x), std::forward<F>(f)); }
このソリューションは、キャプチャされた型がコピー可能でない場合にラムダをコピーすることを防ぎ、実行時エラーを回避します。
以上がC ラムダでムーブ キャプチャを実装するにはどうすればよいですか?の詳細内容です。詳細については、PHP 中国語 Web サイトの他の関連記事を参照してください。

ホットAIツール

Undresser.AI Undress
リアルなヌード写真を作成する AI 搭載アプリ

AI Clothes Remover
写真から衣服を削除するオンライン AI ツール。

Undress AI Tool
脱衣画像を無料で

Clothoff.io
AI衣類リムーバー

Video Face Swap
完全無料の AI 顔交換ツールを使用して、あらゆるビデオの顔を簡単に交換できます。

人気の記事

ホットツール

メモ帳++7.3.1
使いやすく無料のコードエディター

SublimeText3 中国語版
中国語版、とても使いやすい

ゼンドスタジオ 13.0.1
強力な PHP 統合開発環境

ドリームウィーバー CS6
ビジュアル Web 開発ツール

SublimeText3 Mac版
神レベルのコード編集ソフト(SublimeText3)

ホットトピック










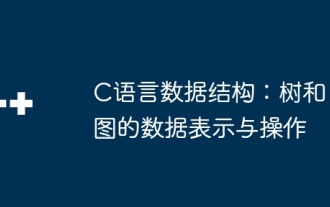
C言語データ構造:ツリーとグラフのデータ表現は、ノードからなる階層データ構造です。各ノードには、データ要素と子ノードへのポインターが含まれています。バイナリツリーは特別なタイプの木です。各ノードには、最大2つの子ノードがあります。データは、structreenode {intdata; structreenode*left; structreenode*右;}を表します。操作は、ツリートラバーサルツリー(前向き、順序、および後期)を作成します。検索ツリー挿入ノード削除ノードグラフは、要素が頂点であるデータ構造のコレクションであり、近隣を表す右または未照明のデータを持つエッジを介して接続できます。
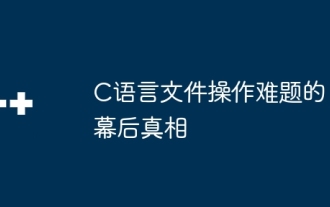
ファイルの操作の問題に関する真実:ファイルの開きが失敗しました:不十分な権限、間違ったパス、およびファイルが占有されます。データの書き込みが失敗しました:バッファーがいっぱいで、ファイルは書き込みできず、ディスクスペースが不十分です。その他のFAQ:遅いファイルトラバーサル、誤ったテキストファイルエンコード、およびバイナリファイルの読み取りエラー。
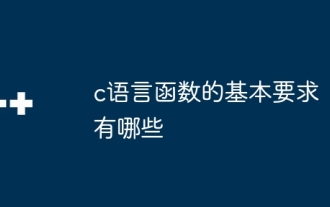
C言語関数は、コードモジュール化とプログラム構築の基礎です。それらは、宣言(関数ヘッダー)と定義(関数体)で構成されています。 C言語は値を使用してパラメーターをデフォルトで渡しますが、外部変数はアドレスパスを使用して変更することもできます。関数は返品値を持つか、または持たない場合があり、返品値のタイプは宣言と一致する必要があります。機能の命名は、ラクダを使用するか、命名法を強調して、明確で理解しやすい必要があります。単一の責任の原則に従い、機能をシンプルに保ち、メンテナビリティと読みやすさを向上させます。
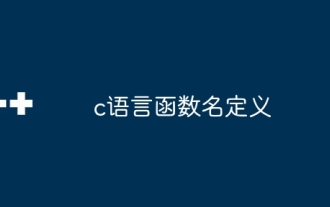
C言語関数名の定義には、以下が含まれます。関数名は、キーワードとの競合を避けるために、明確で簡潔で統一されている必要があります。関数名にはスコープがあり、宣言後に使用できます。関数ポインターにより、関数を引数として渡すか、割り当てます。一般的なエラーには、競合の命名、パラメータータイプの不一致、および未宣言の関数が含まれます。パフォーマンスの最適化は、機能の設計と実装に焦点を当てていますが、明確で読みやすいコードが重要です。
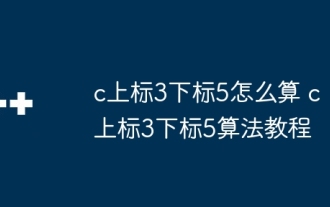
C35の計算は、本質的に組み合わせ数学であり、5つの要素のうち3つから選択された組み合わせの数を表します。計算式はC53 = 5です! /(3! * 2!)。これは、ループで直接計算して効率を向上させ、オーバーフローを避けることができます。さらに、組み合わせの性質を理解し、効率的な計算方法をマスターすることは、確率統計、暗号化、アルゴリズム設計などの分野で多くの問題を解決するために重要です。
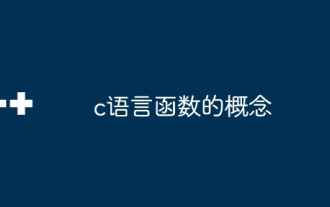
C言語関数は再利用可能なコードブロックです。彼らは入力を受け取り、操作を実行し、結果を返すことができます。これにより、再利用性が改善され、複雑さが軽減されます。関数の内部メカニズムには、パラメーターの渡し、関数の実行、および戻り値が含まれます。プロセス全体には、関数インラインなどの最適化が含まれます。単一の責任、少数のパラメーター、命名仕様、エラー処理の原則に従って、優れた関数が書かれています。関数と組み合わせたポインターは、外部変数値の変更など、より強力な関数を実現できます。関数ポインターは機能をパラメーターまたはストアアドレスとして渡し、機能への動的呼び出しを実装するために使用されます。機能機能とテクニックを理解することは、効率的で保守可能で、理解しやすいCプログラムを書くための鍵です。
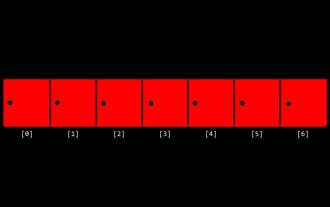
アルゴリズムは、問題を解決するための一連の指示であり、その実行速度とメモリの使用量はさまざまです。プログラミングでは、多くのアルゴリズムがデータ検索とソートに基づいています。この記事では、いくつかのデータ取得およびソートアルゴリズムを紹介します。線形検索では、配列[20,500,10,5,100,1,50]があることを前提としており、数50を見つける必要があります。線形検索アルゴリズムは、ターゲット値が見つかるまで、または完全な配列が見られるまで配列の各要素を1つずつチェックします。アルゴリズムのフローチャートは次のとおりです。線形検索の擬似コードは次のとおりです。各要素を確認します:ターゲット値が見つかった場合:return true return false c言語実装:#include#includeintmain(void){i
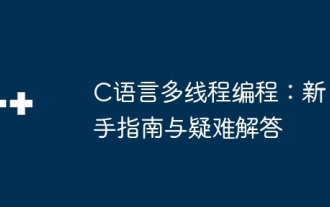
C言語マルチスレッドプログラミングガイド:スレッドの作成:pthread_create()関数を使用して、スレッドID、プロパティ、およびスレッド関数を指定します。スレッドの同期:ミューテックス、セマフォ、および条件付き変数を介したデータ競争を防ぎます。実用的なケース:マルチスレッドを使用してフィボナッチ数を計算し、複数のスレッドにタスクを割り当て、結果を同期させます。トラブルシューティング:プログラムのクラッシュ、スレッドの停止応答、パフォーマンスボトルネックなどの問題を解決します。
