React のカスタムフック: コンポーネント間でロジックを再利用する
React のカスタムフック
カスタム フック は、React アプリケーション内の複数のコンポーネント間でステートフル ロジックを再利用できるようにする JavaScript 関数です。カスタム フックは、コンポーネント間で共有できるロジックをカプセル化し、コンポーネントをクリーンに保ち、コードの再利用性を促進するための強力なツールです。
カスタム フックには、React の規則に従って use という接頭辞が付けられ、その中で他のフック (useState、useEffect、useContext など) を使用できます。
カスタムフックを使用する理由
カスタムフックにはいくつかの利点があります:
- コードの再利用性: コンポーネントから再利用可能なロジックを抽出できます。複数のコンポーネント間で共有する必要があるロジックがある場合は、それをカスタム フックに抽出できます。
- 懸念事項の分離: カスタム フックを使用して複雑なロジックをコンポーネントから移動することで、コンポーネントが UI のレンダリングにより集中できるようになり、可読性と保守性が向上します。
- 抽象化: 複雑なロジックを抽象化する方法を提供し、コンポーネントをよりクリーンで理解しやすくします。
カスタムフックを作成する方法
カスタムフックを作成するには、次の手順に従います:
- 関数を作成します: 関数には、再利用するロジックが含まれている必要があります。
- 組み込みフックを使用する: 関数内で、useState、useEffect、またはその他のフックなどの他の React フックを使用して、状態や副作用を管理できます。
- 戻り値: コンポーネントで使用されるカスタム フックから必要な状態、関数、または値を返します。
カスタムフックの基本的な例
マウスの位置を管理するカスタム フックの簡単な例を次に示します。
import { useState, useEffect } from 'react'; // Custom Hook to track mouse position const useMousePosition = () => { const [position, setPosition] = useState({ x: 0, y: 0 }); useEffect(() => { const updatePosition = (event) => { setPosition({ x: event.clientX, y: event.clientY }); }; // Add event listener for mouse movement window.addEventListener('mousemove', updatePosition); // Clean up the event listener return () => { window.removeEventListener('mousemove', updatePosition); }; }, []); return position; }; export default useMousePosition;
説明:
- カスタム フック useMousePosition は、画面上のマウスの位置を追跡します。
- useState を使用してマウス座標 (x および y) の状態を管理します。
- useEffect を使用して、mousemove イベントのイベント リスナーを追加し、コンポーネントがアンマウントされるかエフェクトが再実行されるときにイベント リスナーをクリーンアップします。
- フックはマウスの位置 (x と y) を返します。この位置は、useMousePosition をインポートして呼び出すコンポーネントで使用できます。
コンポーネントでのカスタムフックの使用
これで、任意のコンポーネントでこのカスタム フックを使用して、マウスの位置にアクセスできるようになります。
import { useState, useEffect } from 'react'; // Custom Hook to track mouse position const useMousePosition = () => { const [position, setPosition] = useState({ x: 0, y: 0 }); useEffect(() => { const updatePosition = (event) => { setPosition({ x: event.clientX, y: event.clientY }); }; // Add event listener for mouse movement window.addEventListener('mousemove', updatePosition); // Clean up the event listener return () => { window.removeEventListener('mousemove', updatePosition); }; }, []); return position; }; export default useMousePosition;
説明:
- MouseTracker コンポーネントは、useMousePosition カスタム フックを使用してマウスの位置にアクセスします。
- マウスを移動するたびに位置が更新され、コンポーネントが再レンダリングされて新しい座標が表示されます。
高度な例: フォーム処理用のカスタムフック
フォーム処理など、より複雑なロジック用のカスタム フックを作成できます。
import React from 'react'; import useMousePosition from './useMousePosition'; const MouseTracker = () => { const position = useMousePosition(); // Using the custom hook return ( <div> <h2>Mouse Position:</h2> <p>X: {position.x}, Y: {position.y}</p> </div> ); }; export default MouseTracker;
説明:
- useFormInput フックは初期値を受け取り、入力値と handleChange 関数を返します。
- フックは、フォーム入力状態を管理するために任意のフォーム コンポーネントで使用できます。
コンポーネントでのフォームフックの使用
これで、フォーム コンポーネントで useFormInput を使用できるようになります。
import { useState } from 'react'; // Custom Hook to handle form input const useFormInput = (initialValue) => { const [value, setValue] = useState(initialValue); const handleChange = (event) => { setValue(event.target.value); }; return { value, onChange: handleChange, }; }; export default useFormInput;
説明:
- useFormInput フックは、名前と電子メールの両方の入力の状態と変更イベントを処理するために使用されます。
- handleSubmit 関数は、フォームが送信されたときにフォームの値をログに記録します。
カスタムフックのルール
カスタムフックは React フックと同じルールに従います:
- 最上位レベルでのみフックを呼び出します: 条件付きまたはループ内でフックを呼び出しないでください。
- React 関数からのみフックを呼び出します: カスタム フックは、React 関数コンポーネントまたは他のカスタム フックからのみ呼び出すことができます。
- use で始まる: カスタム フックは、通常の JavaScript 関数と区別するために、 use プレフィックスで始まる必要があります。
副作用のためのカスタムフックの使用
カスタム フックを使用して、データのフェッチなどの副作用を処理することもできます。
import React from 'react'; import useFormInput from './useFormInput'; const MyForm = () => { const nameInput = useFormInput(''); const emailInput = useFormInput(''); const handleSubmit = (event) => { event.preventDefault(); console.log('Name:', nameInput.value); console.log('Email:', emailInput.value); }; return ( <form onSubmit={handleSubmit}> <div> <label>Name:</label> <input type="text" {...nameInput} /> </div> <div> <label>Email:</label> <input type="email" {...emailInput} /> </div> <button type="submit">Submit</button> </form> ); }; export default MyForm;
説明:
- useFetchData は、API からデータをフェッチするカスタム フックです。
- データ、isLoading、およびエラー状態を管理します。
- フックは、API からデータをフェッチする必要があるコンポーネントで再利用できます。
コンポーネントでのデータ取得フックの使用
コンポーネントで useFetchData フックを使用する方法は次のとおりです:
import { useState, useEffect } from 'react'; // Custom Hook to track mouse position const useMousePosition = () => { const [position, setPosition] = useState({ x: 0, y: 0 }); useEffect(() => { const updatePosition = (event) => { setPosition({ x: event.clientX, y: event.clientY }); }; // Add event listener for mouse movement window.addEventListener('mousemove', updatePosition); // Clean up the event listener return () => { window.removeEventListener('mousemove', updatePosition); }; }, []); return position; }; export default useMousePosition;
説明:
- DataComponent は useFetchData カスタム フックを使用して API からデータをフェッチします。
- コンポーネントは、カスタム フックから返された状態に基づいて、フェッチされたデータのロード、エラー、表示を処理します。
カスタムフックの概要
- カスタム フック を使用すると、React アプリケーションでロジックをカプセル化して再利用できます。
- 複雑なロジックを抽象化することで、コンポーネントをクリーンな状態に保つのに役立ちます。
- カスタム フックでは、useState、useEffect などの組み込みフックを使用でき、React フックと同じルールに従います。
- カスタム フックの一般的な使用例には、フォーム入力の管理、データのフェッチ、副作用の処理などが含まれます。
以上がReact のカスタムフック: コンポーネント間でロジックを再利用するの詳細内容です。詳細については、PHP 中国語 Web サイトの他の関連記事を参照してください。

ホットAIツール

Undresser.AI Undress
リアルなヌード写真を作成する AI 搭載アプリ

AI Clothes Remover
写真から衣服を削除するオンライン AI ツール。

Undress AI Tool
脱衣画像を無料で

Clothoff.io
AI衣類リムーバー

Video Face Swap
完全無料の AI 顔交換ツールを使用して、あらゆるビデオの顔を簡単に交換できます。

人気の記事

ホットツール

メモ帳++7.3.1
使いやすく無料のコードエディター

SublimeText3 中国語版
中国語版、とても使いやすい

ゼンドスタジオ 13.0.1
強力な PHP 統合開発環境

ドリームウィーバー CS6
ビジュアル Web 開発ツール

SublimeText3 Mac版
神レベルのコード編集ソフト(SublimeText3)

ホットトピック










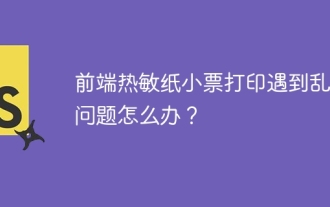
フロントエンドのサーマルペーパーチケット印刷のためのよくある質問とソリューションフロントエンド開発におけるチケット印刷は、一般的な要件です。しかし、多くの開発者が実装しています...
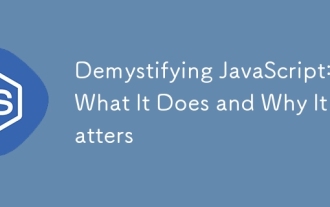
JavaScriptは現代のWeb開発の基礎であり、その主な機能には、イベント駆動型のプログラミング、動的コンテンツ生成、非同期プログラミングが含まれます。 1)イベント駆動型プログラミングにより、Webページはユーザー操作に応じて動的に変更できます。 2)動的コンテンツ生成により、条件に応じてページコンテンツを調整できます。 3)非同期プログラミングにより、ユーザーインターフェイスがブロックされないようにします。 JavaScriptは、Webインタラクション、シングルページアプリケーション、サーバー側の開発で広く使用されており、ユーザーエクスペリエンスとクロスプラットフォーム開発の柔軟性を大幅に改善しています。
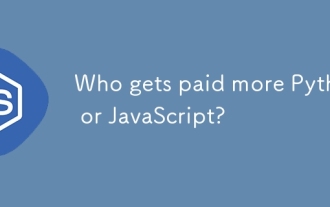
スキルや業界のニーズに応じて、PythonおよびJavaScript開発者には絶対的な給与はありません。 1. Pythonは、データサイエンスと機械学習でさらに支払われる場合があります。 2。JavaScriptは、フロントエンドとフルスタックの開発に大きな需要があり、その給与もかなりです。 3。影響要因には、経験、地理的位置、会社の規模、特定のスキルが含まれます。
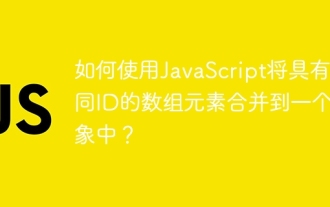
同じIDを持つ配列要素をJavaScriptの1つのオブジェクトにマージする方法は?データを処理するとき、私たちはしばしば同じIDを持つ必要性に遭遇します...
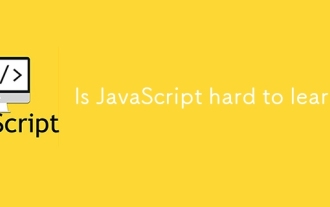
JavaScriptを学ぶことは難しくありませんが、挑戦的です。 1)変数、データ型、関数などの基本概念を理解します。2)非同期プログラミングをマスターし、イベントループを通じて実装します。 3)DOM操作を使用し、非同期リクエストを処理することを約束します。 4)一般的な間違いを避け、デバッグテクニックを使用します。 5)パフォーマンスを最適化し、ベストプラクティスに従ってください。
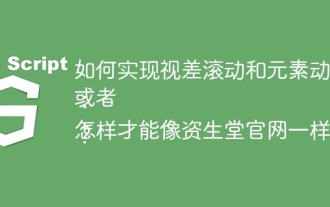
この記事の視差スクロールと要素のアニメーション効果の実現に関する議論では、Shiseidoの公式ウェブサイト(https://www.shisido.co.co.jp/sb/wonderland/)と同様の達成方法について説明します。
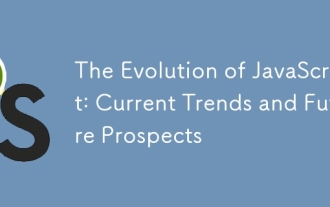
JavaScriptの最新トレンドには、TypeScriptの台頭、最新のフレームワークとライブラリの人気、WebAssemblyの適用が含まれます。将来の見通しは、より強力なタイプシステム、サーバー側のJavaScriptの開発、人工知能と機械学習の拡大、およびIoTおよびEDGEコンピューティングの可能性をカバーしています。
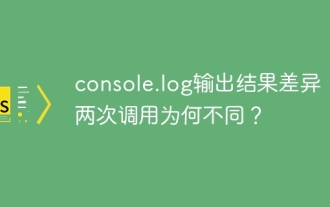
Console.log出力の違いの根本原因に関する詳細な議論。この記事では、Console.log関数の出力結果の違いをコードの一部で分析し、その背後にある理由を説明します。 �...
