ソースコードを変更して Python 関数をハッキングする
次の pikoTutorial へようこそ!
実装を手動で変更して関数の動作を変更するのは明らかですが、アプリケーションの実行時に関数の実装を何らかの方法でいじることはできるでしょうか?このプロセスを 3 つのステップに整理してみましょう:
- 実行時に関数のソースコードを取得する
- ソースコードを含む文字列を呼び出し可能なオブジェクトに変換します
- 関数を呼び出す前に関数のソース コードを変更します
実行時に関数のソースコードを取得する
まず、関数のソース コードを取得する方法を学びましょう:
# Import inspect module import inspect # Define some callback function def function(): print('Do something') source_code = inspect.getsource(function) print(source_code)
出力:
def callback(): print('Do something')
ソースコードを含む文字列を呼び出し可能なオブジェクトに変換する
次に、文字列で提供された任意の Python コードを呼び出し可能な Python オブジェクトに変換する方法を見てみましょう。
# Source code that we want to execute source_code = 'print("Hello from the inside of the string!")' # Wrap the source code into a function definition, so that it can be accessed by name function_name = 'print_hello' function_definition = f'def {function_name}():\n {source_code}' namespace = {} # Execute code with a function definition within the given namespace, so that the function definition is created exec(function_definition, namespace) # Retrieve function from the namespace and save to a callable variable print_hello = namespace[function_name] # Call the function print_hello()
出力:
Hello from the inside of the string!
関数を呼び出す前に関数のソース コードを変更する
次に、関数ポインターを入力として受け取り、変更されたソース コードを含む呼び出し可能なオブジェクトを返す関数を実装しましょう。
import inspect def get_hacked_function(function): # Get the source code of the given function original_function_source_code = inspect.getsource(function) # Append a new line to the function source code modified_function_source_code = f'{original_function_source_code} print("You didn\'t expect me here!")' # Call the function within the namespace namespace = {} exec(modified_function_source_code, namespace) # Parse function name by taking everything what's between "def " and "(" at the first line function_name = original_function_source_code.split('(')[0].split()[1] # Retrieve modified function modified_function = namespace[function_name] # Return modified function return modified_function
テストの時間です!
# This is the function passed as an input def original_function(): print("Hello") # Call our hacking function hacked_function = get_hacked_function(original_function) # Call the modified function hacked_function()
出力:
Hello You didn't expect me here!
初心者向けの注意: このような実験は主に教育目的で行われていることに留意してください。 exec() 関数を使用すると、重大なセキュリティ問題が発生する可能性があるため、運用環境での使用はお勧めできません。ソース コードにアクセスできない関数の動作を変更する必要がある場合は、代わりに関数デコレーターの使用を検討してください。 exec() を使用する前に常に注意し、セキュリティへの影響を十分に理解してください。
以上がソースコードを変更して Python 関数をハッキングするの詳細内容です。詳細については、PHP 中国語 Web サイトの他の関連記事を参照してください。

ホットAIツール

Undresser.AI Undress
リアルなヌード写真を作成する AI 搭載アプリ

AI Clothes Remover
写真から衣服を削除するオンライン AI ツール。

Undress AI Tool
脱衣画像を無料で

Clothoff.io
AI衣類リムーバー

Video Face Swap
完全無料の AI 顔交換ツールを使用して、あらゆるビデオの顔を簡単に交換できます。

人気の記事

ホットツール

メモ帳++7.3.1
使いやすく無料のコードエディター

SublimeText3 中国語版
中国語版、とても使いやすい

ゼンドスタジオ 13.0.1
強力な PHP 統合開発環境

ドリームウィーバー CS6
ビジュアル Web 開発ツール

SublimeText3 Mac版
神レベルのコード編集ソフト(SublimeText3)

ホットトピック










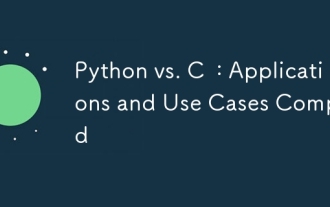
Pythonは、データサイエンス、Web開発、自動化タスクに適していますが、Cはシステムプログラミング、ゲーム開発、組み込みシステムに適しています。 Pythonは、そのシンプルさと強力なエコシステムで知られていますが、Cは高性能および基礎となる制御機能で知られています。
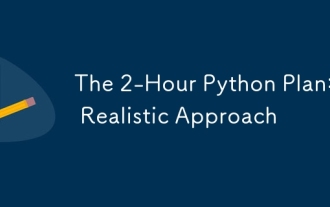
2時間以内にPythonの基本的なプログラミングの概念とスキルを学ぶことができます。 1.変数とデータ型、2。マスターコントロールフロー(条件付きステートメントとループ)、3。機能の定義と使用を理解する4。
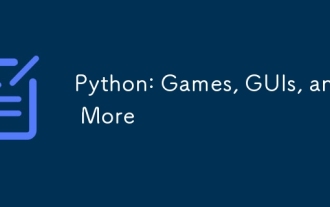
PythonはゲームとGUI開発に優れています。 1)ゲーム開発は、2Dゲームの作成に適した図面、オーディオ、その他の機能を提供し、Pygameを使用します。 2)GUI開発は、TKINTERまたはPYQTを選択できます。 TKINTERはシンプルで使いやすく、PYQTは豊富な機能を備えており、専門能力開発に適しています。
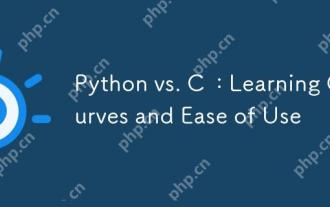
Pythonは学習と使用が簡単ですが、Cはより強力ですが複雑です。 1。Python構文は簡潔で初心者に適しています。動的なタイピングと自動メモリ管理により、使いやすくなりますが、ランタイムエラーを引き起こす可能性があります。 2.Cは、高性能アプリケーションに適した低レベルの制御と高度な機能を提供しますが、学習しきい値が高く、手動メモリとタイプの安全管理が必要です。
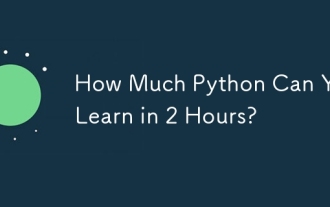
2時間以内にPythonの基本を学ぶことができます。 1。変数とデータ型を学習します。2。ステートメントやループの場合などのマスター制御構造、3。関数の定義と使用を理解します。これらは、簡単なPythonプログラムの作成を開始するのに役立ちます。
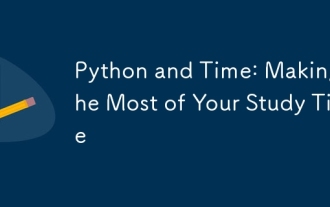
限られた時間でPythonの学習効率を最大化するには、PythonのDateTime、時間、およびスケジュールモジュールを使用できます。 1. DateTimeモジュールは、学習時間を記録および計画するために使用されます。 2。時間モジュールは、勉強と休息の時間を設定するのに役立ちます。 3.スケジュールモジュールは、毎週の学習タスクを自動的に配置します。
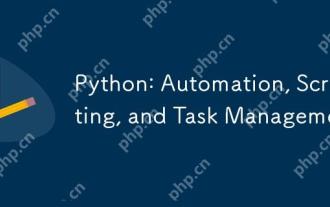
Pythonは、自動化、スクリプト、およびタスク管理に優れています。 1)自動化:OSやShutilなどの標準ライブラリを介してファイルバックアップが実現されます。 2)スクリプトの書き込み:Psutilライブラリを使用してシステムリソースを監視します。 3)タスク管理:スケジュールライブラリを使用してタスクをスケジュールします。 Pythonの使いやすさと豊富なライブラリサポートにより、これらの分野で優先ツールになります。
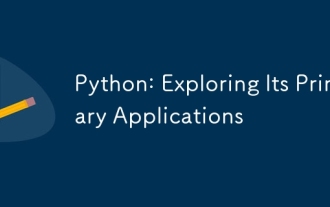
Pythonは、Web開発、データサイエンス、機械学習、自動化、スクリプトの分野で広く使用されています。 1)Web開発では、DjangoおよびFlask Frameworksが開発プロセスを簡素化します。 2)データサイエンスと機械学習の分野では、Numpy、Pandas、Scikit-Learn、Tensorflowライブラリが強力なサポートを提供します。 3)自動化とスクリプトの観点から、Pythonは自動テストやシステム管理などのタスクに適しています。
