Python カメラ SDK の構築とマルチバーコード スキャンへの使用
Windows、Linux、macOS 用の軽量 C カメラ SDK が完成したので、これを他の高級プログラミング言語に統合できます。この記事では、C カメラ ライブラリに基づいて Python カメラ SDK を構築し、それを Dynamsoft Barcode Reader SDK でマルチバーコード スキャンに使用する方法を検討します。
Python マルチバーコード スキャナーのデモ ビデオ
CPython 拡張プロジェクトのスキャフォールディング
CPython 拡張機能は、共有ライブラリ (Windows の DLL、Linux の .so、macOS の .dylib など) です。実行時に Python インタープリターにロードし、その機能を拡張するために使用できます。 Lite カメラ CPython 拡張プロジェクトの構造は次のとおりです:
python-lite-camera │ │── include │ ├── Camera.h │ ├── CameraPreview.h │ ├── stb_image_write.h │── lib │ ├── linux │ │ ├── liblitecam.so │ ├── macos │ │ ├── liblitecam.dylib │ ├── windows │ │ ├── litecam.dll │ │ ├── litecam.lib ├── src │ ├── litecam.cpp │ ├── pycamera.h │ ├── pywindow.h │── litecam │ ├── __init__.py │── setup.py │── MANIFEST.in │── README.md
説明:
- インクルード: C カメラ ライブラリのヘッダー ファイル。
- lib: C カメラ ライブラリの共有ライブラリ。
- src: Python カメラ SDK のソース コード。
- litecam: Python 拡張機能のエントリ ポイント。
- setup.py: ビルド スクリプト。
- MANIFEST.in: Python 以外のファイルを含めるためのマニフェスト ファイル。
- README.md: ドキュメント。
Python 拡張機能用のビルド スクリプト setup.py の作成
次のコンテンツを setup.py に追加します:
from setuptools.command import build_ext from setuptools import setup, Extension import sys import os import io from setuptools.command.install import install import shutil from pathlib import Path lib_dir = '' sources = [ "src/litecam.cpp", ] include_dirs = [os.path.join(os.path.dirname(__file__), "include")] libraries = ['litecam'] extra_compile_args = [] if sys.platform == "linux" or sys.platform == "linux2": lib_dir = 'lib/linux' extra_compile_args = ['-std=c++11'] extra_link_args = ["-Wl,-rpath=$ORIGIN"] elif sys.platform == "darwin": lib_dir = 'lib/macos' extra_compile_args = ['-std=c++11'] extra_link_args = ["-Wl,-rpath,@loader_path"] elif sys.platform == "win32": lib_dir = 'lib/windows' extra_link_args = [] else: raise RuntimeError("Unsupported platform") long_description = io.open("README.md", encoding="utf-8").read() module_litecam = Extension( "litecam", sources=sources, include_dirs=include_dirs, library_dirs=[lib_dir], libraries=libraries, extra_compile_args=extra_compile_args, extra_link_args=extra_link_args, ) def copyfiles(src, dst): if os.path.isdir(src): filelist = os.listdir(src) for file in filelist: libpath = os.path.join(src, file) shutil.copy2(libpath, dst) else: shutil.copy2(src, dst) class CustomBuildExt(build_ext.build_ext): def run(self): build_ext.build_ext.run(self) dst = os.path.join(self.build_lib, "litecam") copyfiles(lib_dir, dst) filelist = os.listdir(self.build_lib) for file in filelist: filePath = os.path.join(self.build_lib, file) if not os.path.isdir(file): copyfiles(filePath, dst) os.remove(filePath) class CustomBuildExtDev(build_ext.build_ext): def run(self): build_ext.build_ext.run(self) dev_folder = os.path.join(Path(__file__).parent, 'litecam') copyfiles(lib_dir, dev_folder) filelist = os.listdir(self.build_lib) for file in filelist: filePath = os.path.join(self.build_lib, file) if not os.path.isdir(file): copyfiles(filePath, dev_folder) class CustomInstall(install): def run(self): install.run(self) setup(name='lite-camera', version='2.0.1', description='LiteCam is a lightweight, cross-platform library for capturing RGB frames from cameras and displaying them. Designed with simplicity and ease of integration in mind, LiteCam supports Windows, Linux and macOS platforms.', long_description=long_description, long_description_content_type="text/markdown", author='yushulx', url='https://github.com/yushulx/python-lite-camera', license='MIT', packages=['litecam'], ext_modules=[module_litecam], classifiers=[ "Development Status :: 5 - Production/Stable", "Environment :: Console", "Intended Audience :: Developers", "Intended Audience :: Education", "Intended Audience :: Information Technology", "Intended Audience :: Science/Research", "License :: OSI Approved :: MIT License", "Operating System :: Microsoft :: Windows", "Operating System :: MacOS", "Operating System :: POSIX :: Linux", "Programming Language :: Python", "Programming Language :: Python :: 3", "Programming Language :: Python :: 3 :: Only", "Programming Language :: Python :: 3.6", "Programming Language :: Python :: 3.7", "Programming Language :: Python :: 3.8", "Programming Language :: Python :: 3.9", "Programming Language :: Python :: 3.10", "Programming Language :: Python :: 3.11", "Programming Language :: Python :: 3.12", "Programming Language :: C++", "Programming Language :: Python :: Implementation :: CPython", "Topic :: Scientific/Engineering", "Topic :: Software Development", ], cmdclass={ 'install': CustomInstall, 'build_ext': CustomBuildExt, 'develop': CustomBuildExtDev}, )
説明:
- lite-camera: Python パッケージの名前。
- ext_modules: CPython 拡張機能のリスト。ソース ファイル、インクルード ディレクトリ、ライブラリ ディレクトリ、ライブラリ、およびさまざまなプラットフォームのコンパイル/リンク フラグを指定します。
- 開発: 開発用のカスタム コマンド。簡単にテストできるように、共有ライブラリを litecam フォルダーにコピーします。
- build_ext: 共有ライブラリを Wheel パッケージにパッケージ化するためのビルド プロセスをカスタマイズします。
C での Python カメラ SDK API の実装
pycamera.h ファイルはカメラからフレームをキャプチャするための PyCamera Python クラスを定義し、pywindow.h ファイルはウィンドウにフレームを表示するための PyWindow Python クラスを定義します。 litecam.cpp ファイルは Python 拡張機能のエントリ ポイントとして機能し、いくつかのグローバル メソッドが定義され、PyCamera クラスと PyWindow クラスが登録されます。
パイカメラ.h
含まれるもの
python-lite-camera │ │── include │ ├── Camera.h │ ├── CameraPreview.h │ ├── stb_image_write.h │── lib │ ├── linux │ │ ├── liblitecam.so │ ├── macos │ │ ├── liblitecam.dylib │ ├── windows │ │ ├── litecam.dll │ │ ├── litecam.lib ├── src │ ├── litecam.cpp │ ├── pycamera.h │ ├── pywindow.h │── litecam │ ├── __init__.py │── setup.py │── MANIFEST.in │── README.md
- Python.h: Python C API を使用するために含まれています。
- structmember.h: オブジェクト メンバーを管理するためのマクロとヘルパーを提供します。
- Camera.h: PyCamera 拡張機能がラップする Camera クラスを定義します。
PyCamera 構造体の定義
from setuptools.command import build_ext from setuptools import setup, Extension import sys import os import io from setuptools.command.install import install import shutil from pathlib import Path lib_dir = '' sources = [ "src/litecam.cpp", ] include_dirs = [os.path.join(os.path.dirname(__file__), "include")] libraries = ['litecam'] extra_compile_args = [] if sys.platform == "linux" or sys.platform == "linux2": lib_dir = 'lib/linux' extra_compile_args = ['-std=c++11'] extra_link_args = ["-Wl,-rpath=$ORIGIN"] elif sys.platform == "darwin": lib_dir = 'lib/macos' extra_compile_args = ['-std=c++11'] extra_link_args = ["-Wl,-rpath,@loader_path"] elif sys.platform == "win32": lib_dir = 'lib/windows' extra_link_args = [] else: raise RuntimeError("Unsupported platform") long_description = io.open("README.md", encoding="utf-8").read() module_litecam = Extension( "litecam", sources=sources, include_dirs=include_dirs, library_dirs=[lib_dir], libraries=libraries, extra_compile_args=extra_compile_args, extra_link_args=extra_link_args, ) def copyfiles(src, dst): if os.path.isdir(src): filelist = os.listdir(src) for file in filelist: libpath = os.path.join(src, file) shutil.copy2(libpath, dst) else: shutil.copy2(src, dst) class CustomBuildExt(build_ext.build_ext): def run(self): build_ext.build_ext.run(self) dst = os.path.join(self.build_lib, "litecam") copyfiles(lib_dir, dst) filelist = os.listdir(self.build_lib) for file in filelist: filePath = os.path.join(self.build_lib, file) if not os.path.isdir(file): copyfiles(filePath, dst) os.remove(filePath) class CustomBuildExtDev(build_ext.build_ext): def run(self): build_ext.build_ext.run(self) dev_folder = os.path.join(Path(__file__).parent, 'litecam') copyfiles(lib_dir, dev_folder) filelist = os.listdir(self.build_lib) for file in filelist: filePath = os.path.join(self.build_lib, file) if not os.path.isdir(file): copyfiles(filePath, dev_folder) class CustomInstall(install): def run(self): install.run(self) setup(name='lite-camera', version='2.0.1', description='LiteCam is a lightweight, cross-platform library for capturing RGB frames from cameras and displaying them. Designed with simplicity and ease of integration in mind, LiteCam supports Windows, Linux and macOS platforms.', long_description=long_description, long_description_content_type="text/markdown", author='yushulx', url='https://github.com/yushulx/python-lite-camera', license='MIT', packages=['litecam'], ext_modules=[module_litecam], classifiers=[ "Development Status :: 5 - Production/Stable", "Environment :: Console", "Intended Audience :: Developers", "Intended Audience :: Education", "Intended Audience :: Information Technology", "Intended Audience :: Science/Research", "License :: OSI Approved :: MIT License", "Operating System :: Microsoft :: Windows", "Operating System :: MacOS", "Operating System :: POSIX :: Linux", "Programming Language :: Python", "Programming Language :: Python :: 3", "Programming Language :: Python :: 3 :: Only", "Programming Language :: Python :: 3.6", "Programming Language :: Python :: 3.7", "Programming Language :: Python :: 3.8", "Programming Language :: Python :: 3.9", "Programming Language :: Python :: 3.10", "Programming Language :: Python :: 3.11", "Programming Language :: Python :: 3.12", "Programming Language :: C++", "Programming Language :: Python :: Implementation :: CPython", "Topic :: Scientific/Engineering", "Topic :: Software Development", ], cmdclass={ 'install': CustomInstall, 'build_ext': CustomBuildExt, 'develop': CustomBuildExtDev}, )
PyCamera 構造体は、C Camera オブジェクトをラップする Python オブジェクトを表します。ハンドラーは、Camera クラスのインスタンスへのポインターです。
メソッド
-
PyCamera_dealloc
#include <Python.h> #include <structmember.h> #include "Camera.h"
ログイン後にコピーログイン後にコピーCamera オブジェクトの割り当てを解除し、関連付けられたメモリを解放します。
-
PyCamera_new
typedef struct { PyObject_HEAD Camera *handler; } PyCamera;
ログイン後にコピーログイン後にコピーPyCamera の新しいインスタンスを作成します。 Python オブジェクトにメモリを割り当て、新しい Camera オブジェクトを作成し、それを self->handler に割り当てます。
-
開く
static int PyCamera_clear(PyCamera *self) { if (self->handler) { delete self->handler; } return 0; } static void PyCamera_dealloc(PyCamera *self) { PyCamera_clear(self); Py_TYPE(self)->tp_free((PyObject *)self); }
ログイン後にコピーログイン後にコピー指定されたインデックスでカメラを開きます。
-
メディアタイプのリスト
static PyObject *PyCamera_new(PyTypeObject *type, PyObject *args, PyObject *kwds) { PyCamera *self; self = (PyCamera *)type->tp_alloc(type, 0); if (self != NULL) { self->handler = new Camera(); } return (PyObject *)self; }
ログイン後にコピーログイン後にコピーサポートされているメディア タイプのリストを返します。 Windows の場合、ワイド文字列を Python Unicode オブジェクトに変換します。
-
リリース
static PyObject *open(PyObject *obj, PyObject *args) { PyCamera *self = (PyCamera *)obj; int index = 0; if (!PyArg_ParseTuple(args, "i", &index)) { return NULL; } bool ret = self->handler->Open(index); return Py_BuildValue("i", ret); }
ログイン後にコピーログイン後にコピーカメラを解放します。
-
setResolution
static PyObject *listMediaTypes(PyObject *obj, PyObject *args) { PyCamera *self = (PyCamera *)obj; std::vector<MediaTypeInfo> mediaTypes = self->handler->ListSupportedMediaTypes(); PyObject *pyList = PyList_New(0); for (size_t i = 0; i < mediaTypes.size(); i++) { MediaTypeInfo &mediaType = mediaTypes[i]; #ifdef _WIN32 PyObject *subtypeName = PyUnicode_FromWideChar(mediaType.subtypeName, wcslen(mediaType.subtypeName)); PyObject *pyMediaType = Py_BuildValue("{s:i,s:i,s:O}", "width", mediaType.width, "height", mediaType.height, "subtypeName", subtypeName); if (subtypeName != NULL) { Py_DECREF(subtypeName); } #else PyObject *pyMediaType = Py_BuildValue("{s:i,s:i,s:s}", "width", mediaType.width, "height", mediaType.height, "subtypeName", mediaType.subtypeName); #endif PyList_Append(pyList, pyMediaType); } return pyList; }
ログイン後にコピーログイン後にコピーカメラの解像度を設定します。
-
キャプチャフレーム
static PyObject *release(PyObject *obj, PyObject *args) { PyCamera *self = (PyCamera *)obj; self->handler->Release(); Py_RETURN_NONE; }
ログイン後にコピーログイン後にコピーカメラからフレームをキャプチャし、RGB データを Python バイト配列として返します。
-
getWidth と getHeight
static PyObject *setResolution(PyObject *obj, PyObject *args) { PyCamera *self = (PyCamera *)obj; int width = 0, height = 0; if (!PyArg_ParseTuple(args, "ii", &width, &height)) { return NULL; } int ret = self->handler->SetResolution(width, height); return Py_BuildValue("i", ret); }
ログイン後にコピーログイン後にコピーキャプチャされたフレームの幅と高さを返します。
インスタンスメソッド
static PyObject *captureFrame(PyObject *obj, PyObject *args) { PyCamera *self = (PyCamera *)obj; FrameData frame = self->handler->CaptureFrame(); if (frame.rgbData) { PyObject *rgbData = PyByteArray_FromStringAndSize((const char *)frame.rgbData, frame.size); PyObject *pyFrame = Py_BuildValue("iiiO", frame.width, frame.height, frame.size, rgbData); ReleaseFrame(frame); return pyFrame; } else { Py_RETURN_NONE; } }
PyCamera Python オブジェクトで使用できるメソッドを定義します。これらのメソッドは、上で定義した対応する C 関数に関連付けられています。
PyCameraType
static PyObject *getWidth(PyObject *obj, PyObject *args) { PyCamera *self = (PyCamera *)obj; int width = self->handler->frameWidth; return Py_BuildValue("i", width); } static PyObject *getHeight(PyObject *obj, PyObject *args) { PyCamera *self = (PyCamera *)obj; int height = self->handler->frameHeight; return Py_BuildValue("i", height); }
メソッド、メモリ割り当て、割り当て解除、その他の動作を含む PyCamera タイプを定義します。
pywindow.h
含まれるもの
static PyMethodDef instance_methods[] = { {"open", open, METH_VARARGS, NULL}, {"listMediaTypes", listMediaTypes, METH_VARARGS, NULL}, {"release", release, METH_VARARGS, NULL}, {"setResolution", setResolution, METH_VARARGS, NULL}, {"captureFrame", captureFrame, METH_VARARGS, NULL}, {"getWidth", getWidth, METH_VARARGS, NULL}, {"getHeight", getHeight, METH_VARARGS, NULL}, {NULL, NULL, 0, NULL}};
- Python.h: Python C API を使用するために必要です。
- structmember.h: オブジェクト メンバーを管理するためのマクロを提供します。
- CameraPreview.h: カメラ プレビューの表示と操作に使用される CameraWindow クラスを定義します。
PyWindow 構造体の定義
static PyTypeObject PyCameraType = { PyVarObject_HEAD_INIT(NULL, 0) "litecam.PyCamera", /* tp_name */ sizeof(PyCamera), /* tp_basicsize */ 0, /* tp_itemsize */ (destructor)PyCamera_dealloc, /* tp_dealloc */ 0, /* tp_print */ 0, /* tp_getattr */ 0, /* tp_setattr */ 0, /* tp_reserved */ 0, /* tp_repr */ 0, /* tp_as_number */ 0, /* tp_as_sequence */ 0, /* tp_as_mapping */ 0, /* tp_hash */ 0, /* tp_call */ 0, /* tp_str */ PyObject_GenericGetAttr, /* tp_getattro */ PyObject_GenericSetAttr, /* tp_setattro */ 0, /* tp_as_buffer */ Py_TPFLAGS_DEFAULT | Py_TPFLAGS_BASETYPE, /*tp_flags*/ "PyCamera", /* tp_doc */ 0, /* tp_traverse */ 0, /* tp_clear */ 0, /* tp_richcompare */ 0, /* tp_weaklistoffset */ 0, /* tp_iter */ 0, /* tp_iternext */ instance_methods, /* tp_methods */ 0, /* tp_members */ 0, /* tp_getset */ 0, /* tp_base */ 0, /* tp_dict */ 0, /* tp_descr_get */ 0, /* tp_descr_set */ 0, /* tp_dictoffset */ 0, /* tp_init */ 0, /* tp_alloc */ PyCamera_new, /* tp_new */ };
C CameraWindow オブジェクトをラップする PyWindow 構造体を定義します。ハンドラー メンバーは、CameraWindow のインスタンスを指します。
PyWindow オブジェクトのメソッド
-
PyWindow_dealloc
#include <Python.h> #include <structmember.h> #include "CameraPreview.h"
ログイン後にコピーCameraWindow オブジェクトの割り当てを解除し、メモリを解放します。
-
PyWindow_new
typedef struct { PyObject_HEAD CameraWindow *handler; } PyWindow;
ログイン後にコピーPyWindow の新しいインスタンスを作成します。 Python オブジェクトにメモリを割り当て、新しい CameraWindow オブジェクトを作成し、それを self->handler に割り当てます。
-
waitKey
python-lite-camera │ │── include │ ├── Camera.h │ ├── CameraPreview.h │ ├── stb_image_write.h │── lib │ ├── linux │ │ ├── liblitecam.so │ ├── macos │ │ ├── liblitecam.dylib │ ├── windows │ │ ├── litecam.dll │ │ ├── litecam.lib ├── src │ ├── litecam.cpp │ ├── pycamera.h │ ├── pywindow.h │── litecam │ ├── __init__.py │── setup.py │── MANIFEST.in │── README.md
ログイン後にコピーログイン後にコピーログイン後にコピーキー押下イベントを待機し、キーが指定された文字と一致する場合は False を返します。 False は、アプリケーションを終了する必要があることを意味します。
-
showFrame
from setuptools.command import build_ext from setuptools import setup, Extension import sys import os import io from setuptools.command.install import install import shutil from pathlib import Path lib_dir = '' sources = [ "src/litecam.cpp", ] include_dirs = [os.path.join(os.path.dirname(__file__), "include")] libraries = ['litecam'] extra_compile_args = [] if sys.platform == "linux" or sys.platform == "linux2": lib_dir = 'lib/linux' extra_compile_args = ['-std=c++11'] extra_link_args = ["-Wl,-rpath=$ORIGIN"] elif sys.platform == "darwin": lib_dir = 'lib/macos' extra_compile_args = ['-std=c++11'] extra_link_args = ["-Wl,-rpath,@loader_path"] elif sys.platform == "win32": lib_dir = 'lib/windows' extra_link_args = [] else: raise RuntimeError("Unsupported platform") long_description = io.open("README.md", encoding="utf-8").read() module_litecam = Extension( "litecam", sources=sources, include_dirs=include_dirs, library_dirs=[lib_dir], libraries=libraries, extra_compile_args=extra_compile_args, extra_link_args=extra_link_args, ) def copyfiles(src, dst): if os.path.isdir(src): filelist = os.listdir(src) for file in filelist: libpath = os.path.join(src, file) shutil.copy2(libpath, dst) else: shutil.copy2(src, dst) class CustomBuildExt(build_ext.build_ext): def run(self): build_ext.build_ext.run(self) dst = os.path.join(self.build_lib, "litecam") copyfiles(lib_dir, dst) filelist = os.listdir(self.build_lib) for file in filelist: filePath = os.path.join(self.build_lib, file) if not os.path.isdir(file): copyfiles(filePath, dst) os.remove(filePath) class CustomBuildExtDev(build_ext.build_ext): def run(self): build_ext.build_ext.run(self) dev_folder = os.path.join(Path(__file__).parent, 'litecam') copyfiles(lib_dir, dev_folder) filelist = os.listdir(self.build_lib) for file in filelist: filePath = os.path.join(self.build_lib, file) if not os.path.isdir(file): copyfiles(filePath, dev_folder) class CustomInstall(install): def run(self): install.run(self) setup(name='lite-camera', version='2.0.1', description='LiteCam is a lightweight, cross-platform library for capturing RGB frames from cameras and displaying them. Designed with simplicity and ease of integration in mind, LiteCam supports Windows, Linux and macOS platforms.', long_description=long_description, long_description_content_type="text/markdown", author='yushulx', url='https://github.com/yushulx/python-lite-camera', license='MIT', packages=['litecam'], ext_modules=[module_litecam], classifiers=[ "Development Status :: 5 - Production/Stable", "Environment :: Console", "Intended Audience :: Developers", "Intended Audience :: Education", "Intended Audience :: Information Technology", "Intended Audience :: Science/Research", "License :: OSI Approved :: MIT License", "Operating System :: Microsoft :: Windows", "Operating System :: MacOS", "Operating System :: POSIX :: Linux", "Programming Language :: Python", "Programming Language :: Python :: 3", "Programming Language :: Python :: 3 :: Only", "Programming Language :: Python :: 3.6", "Programming Language :: Python :: 3.7", "Programming Language :: Python :: 3.8", "Programming Language :: Python :: 3.9", "Programming Language :: Python :: 3.10", "Programming Language :: Python :: 3.11", "Programming Language :: Python :: 3.12", "Programming Language :: C++", "Programming Language :: Python :: Implementation :: CPython", "Topic :: Scientific/Engineering", "Topic :: Software Development", ], cmdclass={ 'install': CustomInstall, 'build_ext': CustomBuildExt, 'develop': CustomBuildExtDev}, )
ログイン後にコピーログイン後にコピーログイン後にコピーウィンドウ内にフレームを表示します。
-
輪郭を描く
#include <Python.h> #include <structmember.h> #include "Camera.h"
ログイン後にコピーログイン後にコピーフレーム上に輪郭 (一連の点) を描画します。
-
描画テキスト
typedef struct { PyObject_HEAD Camera *handler; } PyCamera;
ログイン後にコピーログイン後にコピーフレーム上にテキストを描画します。
window_instance_methods
static int PyCamera_clear(PyCamera *self) { if (self->handler) { delete self->handler; } return 0; } static void PyCamera_dealloc(PyCamera *self) { PyCamera_clear(self); Py_TYPE(self)->tp_free((PyObject *)self); }
PyWindow Python オブジェクトで使用できるメソッドを定義します。
PyWindowType
static PyObject *PyCamera_new(PyTypeObject *type, PyObject *args, PyObject *kwds) { PyCamera *self; self = (PyCamera *)type->tp_alloc(type, 0); if (self != NULL) { self->handler = new Camera(); } return (PyObject *)self; }
メソッド、メモリ割り当て、割り当て解除、その他の動作を含む PyWindow 型を定義します。
ライトカメラ.cpp
static PyObject *open(PyObject *obj, PyObject *args) { PyCamera *self = (PyCamera *)obj; int index = 0; if (!PyArg_ParseTuple(args, "i", &index)) { return NULL; } bool ret = self->handler->Open(index); return Py_BuildValue("i", ret); }
説明:
- getDeviceList: 利用可能なカメラのリストを返します。
- saveJpeg: フレームを JPEG 画像として保存します。
- PyInit_litecam: litecam モジュールを初期化し、PyCamera タイプと PyWindow タイプを登録します。
Python カメラ SDK の構築
-
開発モード
static PyObject *listMediaTypes(PyObject *obj, PyObject *args) { PyCamera *self = (PyCamera *)obj; std::vector<MediaTypeInfo> mediaTypes = self->handler->ListSupportedMediaTypes(); PyObject *pyList = PyList_New(0); for (size_t i = 0; i < mediaTypes.size(); i++) { MediaTypeInfo &mediaType = mediaTypes[i]; #ifdef _WIN32 PyObject *subtypeName = PyUnicode_FromWideChar(mediaType.subtypeName, wcslen(mediaType.subtypeName)); PyObject *pyMediaType = Py_BuildValue("{s:i,s:i,s:O}", "width", mediaType.width, "height", mediaType.height, "subtypeName", subtypeName); if (subtypeName != NULL) { Py_DECREF(subtypeName); } #else PyObject *pyMediaType = Py_BuildValue("{s:i,s:i,s:s}", "width", mediaType.width, "height", mediaType.height, "subtypeName", mediaType.subtypeName); #endif PyList_Append(pyList, pyMediaType); } return pyList; }
ログイン後にコピーログイン後にコピー -
ホイールパッケージ
static PyObject *release(PyObject *obj, PyObject *args) { PyCamera *self = (PyCamera *)obj; self->handler->Release(); Py_RETURN_NONE; }
ログイン後にコピーログイン後にコピー -
ソース配布
static PyObject *setResolution(PyObject *obj, PyObject *args) { PyCamera *self = (PyCamera *)obj; int width = 0, height = 0; if (!PyArg_ParseTuple(args, "ii", &width, &height)) { return NULL; } int ret = self->handler->SetResolution(width, height); return Py_BuildValue("i", ret); }
ログイン後にコピーログイン後にコピー
Python マルチバーコード スキャナーを実装する手順
-
Python カメラ SDK と Dynamsoft Barcode Reader SDK をインストールします:
static PyObject *captureFrame(PyObject *obj, PyObject *args) { PyCamera *self = (PyCamera *)obj; FrameData frame = self->handler->CaptureFrame(); if (frame.rgbData) { PyObject *rgbData = PyByteArray_FromStringAndSize((const char *)frame.rgbData, frame.size); PyObject *pyFrame = Py_BuildValue("iiiO", frame.width, frame.height, frame.size, rgbData); ReleaseFrame(frame); return pyFrame; } else { Py_RETURN_NONE; } }
ログイン後にコピーログイン後にコピー Dynamsoft Barcode Reader の 30 日間の無料試用ライセンスを取得します。
-
マルチバーコードスキャン用の Python スクリプトを作成します:
static PyObject *getWidth(PyObject *obj, PyObject *args) { PyCamera *self = (PyCamera *)obj; int width = self->handler->frameWidth; return Py_BuildValue("i", width); } static PyObject *getHeight(PyObject *obj, PyObject *args) { PyCamera *self = (PyCamera *)obj; int height = self->handler->frameHeight; return Py_BuildValue("i", height); }
ログイン後にコピーログイン後にコピーLICENSE-KEY を Dynamsoft Barcode Reader のライセンス キーに置き換えます。
-
スクリプトを実行します:
static PyMethodDef instance_methods[] = { {"open", open, METH_VARARGS, NULL}, {"listMediaTypes", listMediaTypes, METH_VARARGS, NULL}, {"release", release, METH_VARARGS, NULL}, {"setResolution", setResolution, METH_VARARGS, NULL}, {"captureFrame", captureFrame, METH_VARARGS, NULL}, {"getWidth", getWidth, METH_VARARGS, NULL}, {"getHeight", getHeight, METH_VARARGS, NULL}, {NULL, NULL, 0, NULL}};
ログイン後にコピーログイン後にコピー
ソースコード
https://github.com/yushulx/python-lite-camera
以上がPython カメラ SDK の構築とマルチバーコード スキャンへの使用の詳細内容です。詳細については、PHP 中国語 Web サイトの他の関連記事を参照してください。

ホットAIツール

Undresser.AI Undress
リアルなヌード写真を作成する AI 搭載アプリ

AI Clothes Remover
写真から衣服を削除するオンライン AI ツール。

Undress AI Tool
脱衣画像を無料で

Clothoff.io
AI衣類リムーバー

Video Face Swap
完全無料の AI 顔交換ツールを使用して、あらゆるビデオの顔を簡単に交換できます。

人気の記事

ホットツール

メモ帳++7.3.1
使いやすく無料のコードエディター

SublimeText3 中国語版
中国語版、とても使いやすい

ゼンドスタジオ 13.0.1
強力な PHP 統合開発環境

ドリームウィーバー CS6
ビジュアル Web 開発ツール

SublimeText3 Mac版
神レベルのコード編集ソフト(SublimeText3)

ホットトピック










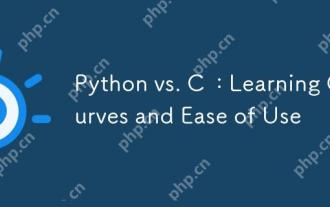
Pythonは学習と使用が簡単ですが、Cはより強力ですが複雑です。 1。Python構文は簡潔で初心者に適しています。動的なタイピングと自動メモリ管理により、使いやすくなりますが、ランタイムエラーを引き起こす可能性があります。 2.Cは、高性能アプリケーションに適した低レベルの制御と高度な機能を提供しますが、学習しきい値が高く、手動メモリとタイプの安全管理が必要です。
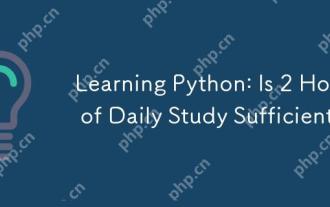
Pythonを1日2時間学ぶだけで十分ですか?それはあなたの目標と学習方法に依存します。 1)明確な学習計画を策定し、2)適切な学習リソースと方法を選択します。3)実践的な実践とレビューとレビューと統合を練習および統合し、統合すると、この期間中にPythonの基本的な知識と高度な機能を徐々に習得できます。
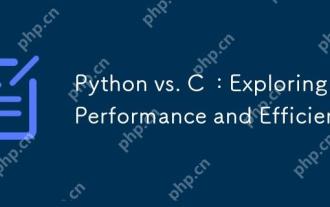
Pythonは開発効率でCよりも優れていますが、Cは実行パフォーマンスが高くなっています。 1。Pythonの簡潔な構文とリッチライブラリは、開発効率を向上させます。 2.Cのコンピレーションタイプの特性とハードウェア制御により、実行パフォーマンスが向上します。選択を行うときは、プロジェクトのニーズに基づいて開発速度と実行効率を比較検討する必要があります。
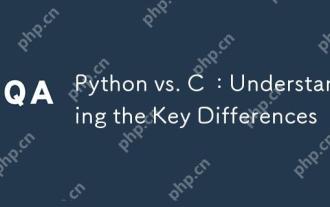
PythonとCにはそれぞれ独自の利点があり、選択はプロジェクトの要件に基づいている必要があります。 1)Pythonは、簡潔な構文と動的タイピングのため、迅速な開発とデータ処理に適しています。 2)Cは、静的なタイピングと手動メモリ管理により、高性能およびシステムプログラミングに適しています。
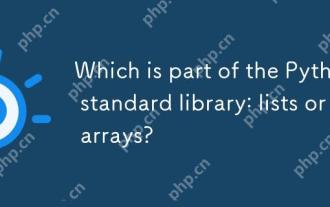
PythonListSarePartOfThestAndardarenot.liestareBuilting-in、versatile、forStoringCollectionsのpythonlistarepart。
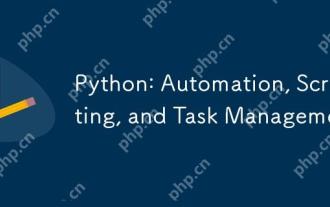
Pythonは、自動化、スクリプト、およびタスク管理に優れています。 1)自動化:OSやShutilなどの標準ライブラリを介してファイルバックアップが実現されます。 2)スクリプトの書き込み:Psutilライブラリを使用してシステムリソースを監視します。 3)タスク管理:スケジュールライブラリを使用してタスクをスケジュールします。 Pythonの使いやすさと豊富なライブラリサポートにより、これらの分野で優先ツールになります。
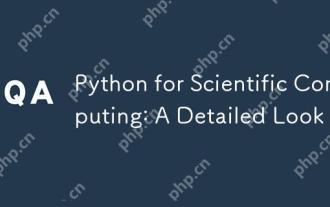
科学コンピューティングにおけるPythonのアプリケーションには、データ分析、機械学習、数値シミュレーション、視覚化が含まれます。 1.numpyは、効率的な多次元配列と数学的関数を提供します。 2。ScipyはNumpy機能を拡張し、最適化と線形代数ツールを提供します。 3. Pandasは、データ処理と分析に使用されます。 4.matplotlibは、さまざまなグラフと視覚的な結果を生成するために使用されます。
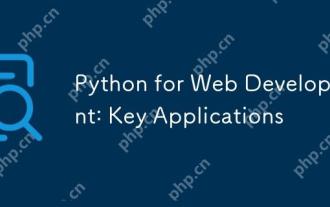
Web開発におけるPythonの主要なアプリケーションには、DjangoおよびFlaskフレームワークの使用、API開発、データ分析と視覚化、機械学習とAI、およびパフォーマンスの最適化が含まれます。 1。DjangoandFlask Framework:Djangoは、複雑な用途の迅速な発展に適しており、Flaskは小規模または高度にカスタマイズされたプロジェクトに適しています。 2。API開発:フラスコまたはdjangorestFrameworkを使用して、Restfulapiを構築します。 3。データ分析と視覚化:Pythonを使用してデータを処理し、Webインターフェイスを介して表示します。 4。機械学習とAI:Pythonは、インテリジェントWebアプリケーションを構築するために使用されます。 5。パフォーマンスの最適化:非同期プログラミング、キャッシュ、コードを通じて最適化
