IntApp Walls API を使用した Matter チームのメンバーシップの操作
Jan 05, 2025 pm 01:16 PMIntApp Walls API は、倫理の壁を管理し、機密データへのアクセスを安全に制御するための強力なツールです。その運用を活用することで、開発者は問題チームと効率的に対話し、メンバーシップを管理し、機密保持要件の遵守を確保できます。
Intapp Walls API は、Intapp Walls アプリケーションと対話するためのプログラム インターフェイスを提供する SOAP Web サービスです。これは、標準コンポーネント Web サービスとしてデプロイされます。
わかりやすくするために、このドキュメントのサンプル コードでは、エラー チェック、例外処理、ロギング、およびその他の実践を省略しています。これは説明のみを目的としており、必ずしもベスト コーディング プラクティスを反映しているわけではありません。
ここでは 2 つの主要なシナリオについて説明します。
- 案件チームのメンバーシップを取得してリストします。
- 既存の案件チームに新しいメンバーを追加します。
「GetMatterTeamForMatter」、「LoadMatterTeam」、「AddUsersToMatterTeam」などの IntApp Walls API 操作を理解して使用することで、倫理的なウォール管理に関連するタスクを合理化できます。次の例には、コード スニペットとステップバイステップのガイダンスが含まれています。
このドキュメントでは、IntApp Walls API への開発アクセスの構成の詳細については説明しません。ただし、管理ソリューションはローカル ドメインにインストールする必要があり、Web サービスには通常、「APIService.svc」という名前のファイルを介してアクセスできます。このファイルは、Visual Studio でサービス参照として追加する必要があります。
サンプル コードは、次の IntApp Walls API オペレーションを参照します。
GetMatterTeamForMatter: 指定された案件に関連付けられている案件チームの ID を取得します。
LoadMatterTeam: 案件チームのプロパティを読み込みます。
GetDMSUserID: DMS ユーザー ID を取得します。一部の API メソッドでは、ユーザーの DMS ユーザー ID が必要です。たとえば、CreateWall() メソッドでは、ユーザー ID がユーザーのタイムキーパー ID やレコード システム ID ではなく、DMS のユーザー ID である必要があります。このメソッドは、ユーザーの別の既知の ID を指定して DMS ユーザー ID を取得するために使用できます。
LoadMatterTeamMembership: 案件チームのメンバーシップを読み込みます。
GetWarningsIfUserIsIncluded: 指定されたユーザーが特定のクライアントまたは案件へのアクセスを許可された (つまり、含まれている) 場合に生成される警告を取得します。この関数は、倫理の壁の衝突によって生成される可能性のある警告を返します。
AddUsersToMatterTeam: 指定されたロールを持つ既存の案件チームにユーザーを追加します。
例: 案件チームのメンバーシップの取得とリスト
次のコード スニペットでは、IntApp Walls API の「GetMatterTeamForMatter」および「LoadMatterTeam」オペレーションを使用して、案件チーム メンバーのリストを取得し、チーム メンバーシップの詳細をコンソールに書き込みます。
メモ:
• IntApp API を操作するには、通常、特定の権限が必要です。多くの場合、適切な IntApp Walls アクセス権を持つサービス アカウントに付与されます。
• 以下のコード スニペット内の「intapp_web_api」への参照は、Visual Studio で定義されている IntApp API サービス参照の名前を指します。
ステップ 1 一意の IntApp Walls 管理案件チーム ID 番号を取得します。
指定された案件に関連付けられた案件チームの ID を取得します。この案件チーム ID は、案件チームのメンバーシップの詳細を取得するために使用されます。
これを実現するには、「GetMatterTeamForMatter」オペレーションを呼び出します。これには「matterID」パラメータが必要です。 「matterID」は通常、内部で生成された ID であり、「ケース番号」と呼ばれることもあります。この値は、ユーザーまたはプログラマによって独自のタイムキーパー タイプのソースから提供されます。
string matterID = "01234"; // matterID supplied by you string matterTeamID = String.Empty; // the return value // get the walls matter team id // example of matter team id "COOLE-033517" matterTeamID = intapp_web_api.GetMatterTeamForMatter(matterID); public static string GetMatterTeamForMatter(string matterID) { intapp_web_api.Matter matter = new intapp_web_api.Matter(); string matterTeamID = string.Empty; try { intapp_web_api.APIServiceClient intapp_web_api = new intapp_web_api.APIServiceClient(); matterTeamID = intapp_web_api.GetMatterTeamForMatter(matterID); if ((string.IsNullOrEmpty(matterTeamID))) { matterTeamID = "blank"; } } catch (Exception ex) { if (string.IsNullOrEmpty(matterTeamID) || ex.Message == "Error") { matterTeamID = "blank"; } } return matterTeamID; }
ステップ 2 Matter チームの結果をロードします
「LoadMatterTeam」メソッドを定義し、「GetMatterTeamForMatter」メソッドの実行で取得した一意の IntApp Walls 管理案件チーム ID 番号「matterTeamID」変数を使用して、「LoadMatterTeam」メソッドを呼び出して案件チームを取得します。案件チーム内の「UserMemberships」コレクションを反復処理し、ユーザー チーム ID とロールをコンソールに出力します。
public static intapp_web_api.MatterTeam LoadMatterTeam(string matterTeamID) { intapp_web_api.MatterTeam matterTeam = new intapp_web_api.MatterTeam(); try { intapp_web_api.APIServiceClient intapp_web_api = new intapp_web_api.APIServiceClient(); matterTeam = intapp_web_api.LoadMatterTeam(wallscaseteamid); } catch (Exception ex) { throw new Exception(ex.Message.ToString()); } return matterTeam; } MatterTeam the_matter_team_list = LoadMatterTeam(wallscaseteamid); using (APIServiceClient intapp_web_api = new APIServiceClient()) { // iterate through the usermemberships collection in the matterteam foreach (UserMembership user in the_matter_team_list.UserMemberships) { string _userid = user.UserId.ToString(); // get the user id string _therole = user.Role.ToString(); // get the user role // output the user team id and role to the console Console.WriteLine($"user team id: {_userid}"); Console.WriteLine($"user team role: {_therole}"); } }
例: 既存の案件チームのメンバーシップに新しいメンバーを追加する
次のコード スニペットは、案件チーム メンバーのリストを取得する「GetMatterTeamForMatter」および「LoadMatterTeam」操作を基にして、IntApp Walls API を使用して既存のチーム メンバーシップを確認し、まだチームに新しいメンバーを追加する方法を示しています。メンバー。
メモ:
• IntApp API を介して IntApp Walls チームを操作するには、特定の権限が必要ですが、このドキュメントの範囲を超えています。要求者は、IntApp Walls で定義されているように、IntApp Walls の事項管理者ロールに属している必要もあります。
• IntApp API を操作するには、通常、特定の権限が必要です。多くの場合、適切な IntApp Walls アクセス権を持つサービス アカウントに付与されます。
• 以下のコード スニペット内の「intapp_web_api」への参照は、Visual Studio で定義されている IntApp API サービス参照の名前を指します。
ステップ 1: 「GetDMSUserID」オペレーションを使用して、Walls チームに追加するユーザーの「sAMAccountName」を取得します。
「sAMAccountName」 (セキュリティ アカウント マネージャーのアカウント名) は、ドメインへの認証に使用されるユーザーのログオン名を表す Microsoft Active Directory (AD) の属性です。
string theid = "jsmith"; // the sAMAccountName ad account name of user to add string wallsuserid = string.Empty; wallsuserid = intapp_web_api.GetDMSUserID(UserIDSource.WindowsNetworkLogon, $@"YourDomainName\{theid}") // change "YourDomainName" to your domain name // check if wallsuserid contains a value if (string.IsNullOrEmpty(wallsuserid)) { Console.WriteLine("the user you are trying to add to Walls team does not exists in Walls"); return; }
ステップ 2: 物質が壁に存在するかどうかを確認します。
string matterID = "01234"; // matterID supplied by you string matterTeamID = String.Empty; // the return value // get the walls matter team id // example of matter team id "COOLE-033517" matterTeamID = intapp_web_api.GetMatterTeamForMatter(matterID); public static string GetMatterTeamForMatter(string matterID) { intapp_web_api.Matter matter = new intapp_web_api.Matter(); string matterTeamID = string.Empty; try { intapp_web_api.APIServiceClient intapp_web_api = new intapp_web_api.APIServiceClient(); matterTeamID = intapp_web_api.GetMatterTeamForMatter(matterID); if ((string.IsNullOrEmpty(matterTeamID))) { matterTeamID = "blank"; } } catch (Exception ex) { if (string.IsNullOrEmpty(matterTeamID) || ex.Message == "Error") { matterTeamID = "blank"; } } return matterTeamID; }
ステップ 3: 案件が存在する場合、ユーザーはすでにチームメンバーですか?
public static intapp_web_api.MatterTeam LoadMatterTeam(string matterTeamID) { intapp_web_api.MatterTeam matterTeam = new intapp_web_api.MatterTeam(); try { intapp_web_api.APIServiceClient intapp_web_api = new intapp_web_api.APIServiceClient(); matterTeam = intapp_web_api.LoadMatterTeam(wallscaseteamid); } catch (Exception ex) { throw new Exception(ex.Message.ToString()); } return matterTeam; } MatterTeam the_matter_team_list = LoadMatterTeam(wallscaseteamid); using (APIServiceClient intapp_web_api = new APIServiceClient()) { // iterate through the usermemberships collection in the matterteam foreach (UserMembership user in the_matter_team_list.UserMemberships) { string _userid = user.UserId.ToString(); // get the user id string _therole = user.Role.ToString(); // get the user role // output the user team id and role to the console Console.WriteLine($"user team id: {_userid}"); Console.WriteLine($"user team role: {_therole}"); } }
ステップ 4: ユーザーを Matter チームに追加すると、内部競合が発生しますか?
string theid = "jsmith"; // the sAMAccountName ad account name of user to add string wallsuserid = string.Empty; wallsuserid = intapp_web_api.GetDMSUserID(UserIDSource.WindowsNetworkLogon, $@"YourDomainName\{theid}") // change "YourDomainName" to your domain name // check if wallsuserid contains a value if (string.IsNullOrEmpty(wallsuserid)) { Console.WriteLine("the user you are trying to add to Walls team does not exists in Walls"); return; }
ステップ 5: 最後に、ユーザーを Matter チームに追加します。
string matterID = "01234"; // matterID supplied by you try { matterTeamID = intapp_web_api.GetMatterTeamForMatter(matterID); } catch (Exception ex) { if (ex.Message.Contains("The matter") && ex.Message.Contains("does not exist")) { Console.WriteLine("the matter does do not exist"); return; } else { Console.WriteLine(ex.Message); return; } }
結論
IntApp Walls API は、案件チームのメンバーシップを管理し、機密情報を保護するための包括的な一連の操作を提供します。チームの詳細の取得から、競合をチェックしながらの新しいメンバーの追加まで、これらの API 関数により、ワークフローとのシームレスな統合と倫理の壁ポリシーの順守が可能になります。適切に実装すると、案件チームの管理は、データの整合性を維持する合理化された効率的なプロセスになります。
以上がIntApp Walls API を使用した Matter チームのメンバーシップの操作の詳細内容です。詳細については、PHP 中国語 Web サイトの他の関連記事を参照してください。

人気の記事

人気の記事

ホットな記事タグ

メモ帳++7.3.1
使いやすく無料のコードエディター

SublimeText3 中国語版
中国語版、とても使いやすい

ゼンドスタジオ 13.0.1
強力な PHP 統合開発環境

ドリームウィーバー CS6
ビジュアル Web 開発ツール

SublimeText3 Mac版
神レベルのコード編集ソフト(SublimeText3)

ホットトピック










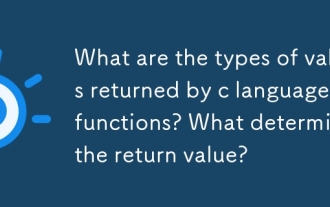
C言語関数によって返される値の種類は何ですか?返品値を決定するものは何ですか?
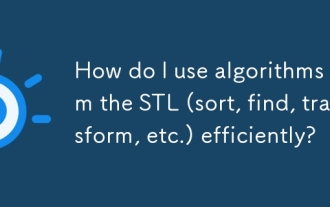
STL(ソート、検索、変換など)のアルゴリズムを効率的に使用するにはどうすればよいですか?
