C# でクラス属性を動的に取得するにはどうすればよいですか?
クラス属性を動的に取得する
オブジェクト指向プログラミングでは、属性はクラスに付加されたメタデータであり、コード自体を超えた追加情報を提供します。実行時にプロパティを動的に読み取る必要がある場合、その方法は次のとおりです。
ドメイン属性の例
DomainName
属性を含む次のコード スニペットを考えてみましょう:
[DomainName("MyTable")] public class MyClass : DomainBase { }
属性読み取りの汎用メソッド
私たちの目標は、指定されたクラスの DomainName
プロパティを読み取り、その値を返すジェネリック メソッドを作成することです。
string GetDomainName<T>() { var dnAttribute = typeof(T).GetCustomAttributes( typeof(DomainNameAttribute), true ).FirstOrDefault() as DomainNameAttribute; if (dnAttribute != null) { return dnAttribute.Name; } return null; }
string domainNameValue = GetDomainName<MyClass>(); // 返回 "MyTable"
共通属性の読み取り
クラスを使用すると、プロパティ読み取り機能を一般化して、任意のプロパティ タイプで動作させることができます。
AttributeExtensions
public static class AttributeExtensions { public static TValue GetAttributeValue<TAttribute, TValue>( this Type type, Func<TAttribute, TValue> valueSelector) where TAttribute : Attribute { var att = type.GetCustomAttributes( typeof(TAttribute), true ).FirstOrDefault() as TAttribute; if (att != null) { return valueSelector(att); } return default(TValue); } }
以上がC# でクラス属性を動的に取得するにはどうすればよいですか?の詳細内容です。詳細については、PHP 中国語 Web サイトの他の関連記事を参照してください。

ホットAIツール

Undresser.AI Undress
リアルなヌード写真を作成する AI 搭載アプリ

AI Clothes Remover
写真から衣服を削除するオンライン AI ツール。

Undress AI Tool
脱衣画像を無料で

Clothoff.io
AI衣類リムーバー

Video Face Swap
完全無料の AI 顔交換ツールを使用して、あらゆるビデオの顔を簡単に交換できます。

人気の記事

ホットツール

メモ帳++7.3.1
使いやすく無料のコードエディター

SublimeText3 中国語版
中国語版、とても使いやすい

ゼンドスタジオ 13.0.1
強力な PHP 統合開発環境

ドリームウィーバー CS6
ビジュアル Web 開発ツール

SublimeText3 Mac版
神レベルのコード編集ソフト(SublimeText3)

ホットトピック










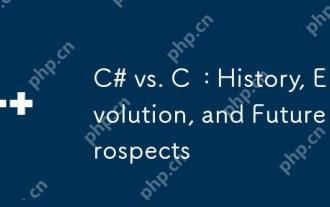
C#とCの歴史と進化はユニークであり、将来の見通しも異なります。 1.Cは、1983年にBjarnestrostrupによって発明され、オブジェクト指向のプログラミングをC言語に導入しました。その進化プロセスには、C 11の自動キーワードとラムダ式の導入など、複数の標準化が含まれます。C20概念とコルーチンの導入、将来のパフォーマンスとシステムレベルのプログラミングに焦点を当てます。 2.C#は2000年にMicrosoftによってリリースされました。CとJavaの利点を組み合わせて、その進化はシンプルさと生産性に焦点を当てています。たとえば、C#2.0はジェネリックを導入し、C#5.0は非同期プログラミングを導入しました。これは、将来の開発者の生産性とクラウドコンピューティングに焦点を当てます。
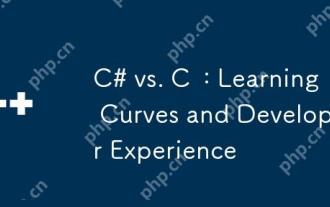
C#とCおよび開発者の経験の学習曲線には大きな違いがあります。 1)C#の学習曲線は比較的フラットであり、迅速な開発およびエンタープライズレベルのアプリケーションに適しています。 2)Cの学習曲線は急勾配であり、高性能および低レベルの制御シナリオに適しています。
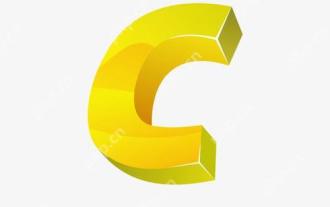
Cでの静的分析の適用には、主にメモリ管理の問題の発見、コードロジックエラーの確認、およびコードセキュリティの改善が含まれます。 1)静的分析では、メモリリーク、ダブルリリース、非初期化ポインターなどの問題を特定できます。 2)未使用の変数、死んだコード、論理的矛盾を検出できます。 3)カバー性などの静的分析ツールは、バッファーオーバーフロー、整数のオーバーフロー、安全でないAPI呼び出しを検出して、コードセキュリティを改善します。
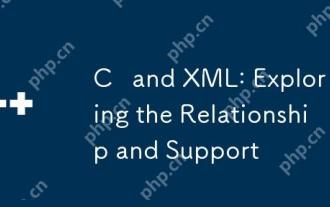
Cは、サードパーティライブラリ(TinyXML、PUGIXML、XERCES-Cなど)を介してXMLと相互作用します。 1)ライブラリを使用してXMLファイルを解析し、それらをC処理可能なデータ構造に変換します。 2)XMLを生成するときは、Cデータ構造をXML形式に変換します。 3)実際のアプリケーションでは、XMLが構成ファイルとデータ交換に使用されることがよくあり、開発効率を向上させます。
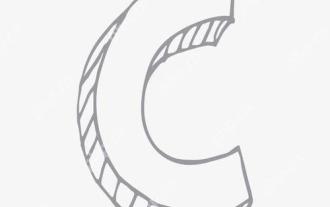
CでChronoライブラリを使用すると、時間と時間の間隔をより正確に制御できます。このライブラリの魅力を探りましょう。 CのChronoライブラリは、時間と時間の間隔に対処するための最新の方法を提供する標準ライブラリの一部です。 Time.HとCtimeに苦しんでいるプログラマーにとって、Chronoは間違いなく恩恵です。コードの読みやすさと保守性を向上させるだけでなく、より高い精度と柔軟性も提供します。基本から始めましょう。 Chronoライブラリには、主に次の重要なコンポーネントが含まれています。STD:: Chrono :: System_Clock:現在の時間を取得するために使用されるシステムクロックを表します。 STD :: Chron
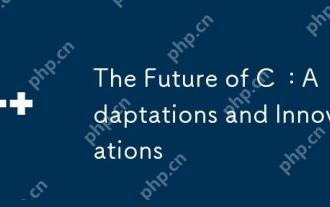
Cの将来は、並列コンピューティング、セキュリティ、モジュール化、AI/機械学習に焦点を当てます。1)並列コンピューティングは、コルーチンなどの機能を介して強化されます。 2)セキュリティは、より厳格なタイプのチェックとメモリ管理メカニズムを通じて改善されます。 3)変調は、コード組織とコンパイルを簡素化します。 4)AIと機械学習は、数値コンピューティングやGPUプログラミングサポートなど、CにComply Coveに適応するように促します。
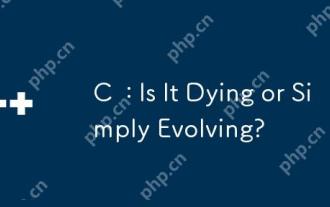
c isnotdying; it'sevolving.1)c relelevantdueToitsversitileSileSixivisityinperformance-criticalApplications.2)thelanguageSlikeModulesandCoroutoUtoimveUsablive.3)despiteChallen
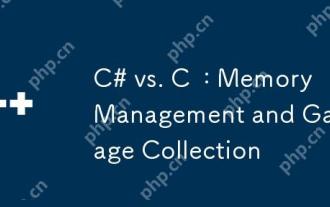
C#は自動ガベージコレクションメカニズムを使用し、Cは手動メモリ管理を使用します。 1。C#のゴミコレクターは、メモリを自動的に管理してメモリの漏れのリスクを減らしますが、パフォーマンスの劣化につながる可能性があります。 2.Cは、微細な管理を必要とするアプリケーションに適した柔軟なメモリ制御を提供しますが、メモリの漏れを避けるためには注意して処理する必要があります。
