Docker を使用してわずか数分で最初の Java アプリを構築してデプロイする
テキストを返し、Docker コンテナを使用してローカル環境のポート 1800 で使用できる単純な Java アプリを 5 分で作成してみましょう (インターネット接続速度によって異なります)。
私のパブリック リポジトリからいつでも完全なソース コードを取得できます:
https://github.com/alexander-uspenskiy/simple-service
依存関係のセットアップ
ステップ 1: 前提条件
- Java 8 をインストールします
- Maven をインストールします
- Docker をインストールします
- VS コード拡張機能をインストールします
Mac のインストール
# Install Homebrew if not present /bin/bash -c "$(curl -fsSL https://raw.githubusercontent.com/Homebrew/install/HEAD/install.sh)" # Install Java 8 brew tap homebrew/cask-versions brew install --cask temurin8 # Install Maven brew install maven # Install Docker Desktop brew install --cask docker # Install VS Code brew install --cask visual-studio-code # Install VS Code Extensions code --install-extension vscjava.vscode-java-pack code --install-extension ms-azuretools.vscode-docker
Windows のインストール
# Install Chocolatey if not present Set-ExecutionPolicy Bypass -Scope Process -Force; [System.Net.ServicePointManager]::SecurityProtocol = [System.Net.ServicePointManager]::SecurityProtocol -bor 3072; iex ((New-Object System.Net.WebClient).DownloadString('https://chocolatey.org/install.ps1')) # Install Java 8 choco install temurin8 # Install Maven choco install maven # Install Docker Desktop choco install docker-desktop # Install VS Code choco install vscode # Install VS Code Extensions code --install-extension vscjava.vscode-java-pack code --install-extension ms-azuretools.vscode-docker
プロジェクトのセットアップ (両方のプラットフォーム)
# Create project structure mkdir -p simple-service cd simple-service
VS コードの設定
{ "java.configuration.runtimes": [ { "name": "JavaSE-1.8", "path": "/Library/Java/JavaVirtualMachines/temurin-8.jdk/Contents/Home", "default": true } ], "java.configuration.updateBuildConfiguration": "automatic", "java.compile.nullAnalysis.mode": "automatic", "maven.executable.path": "/usr/local/bin/mvn" }
インストールの検証
# Verify Java java -version # Verify Maven mvn -version # Verify Docker docker --version
プロジェクトのセットアップ
# Create Maven project mvn archetype:generate \ -DgroupId=com.example \ -DartifactId=simple-service \ -DarchetypeArtifactId=maven-archetype-quickstart \ -DarchetypeVersion=1.4 \ -DinteractiveMode=false
テストアプリの作成
最後の手順を完了すると、事前に構築された構造を持つ simple-service ディレクトリが作成されます。
ステップ 1
- pom.xml ファイルを更新する
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/maven-v4_0_0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.example</groupId> <artifactId>simple-service</artifactId> <packaging>jar</packaging> <version>1.0-SNAPSHOT</version> <name>simple-service</name> <url>http://maven.apache.org</url> <dependencies> <dependency> <groupId>junit</groupId> <artifactId>junit</artifactId> <version>3.8.1</version> <scope>test</scope> </dependency> <dependency> <groupId>org.apache.httpcomponents.client5</groupId> <artifactId>httpclient5</artifactId> <version>5.4</version> </dependency> </dependencies> <properties> <maven.compiler.source>1.8</maven.compiler.source> <maven.compiler.target>1.8</maven.compiler.target> <project.build.sourceEncoding>UTF-8</project.build.sourceEncoding> </properties> <build> <plugins> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-compiler-plugin</artifactId> <version>3.11.0</version> <configuration> <source>1.8</source> <target>1.8</target> </configuration> <executions> <execution> <phase>package</phase> <goals> <goal>shade</goal> </goals> <configuration> <transformers> <transformer implementation="org.apache.maven.plugins.shade.resource.ManifestResourceTransformer"> <mainClass>com.example.App</mainClass> </transformer> </transformers> </configuration> </execution> </executions> </plugin> </plugins> </build> </project>
ステップ 2
App.java にロジックを追加します
package com.example; import com.sun.net.httpserver.HttpServer; import java.net.InetSocketAddress; import java.io.IOException; import java.io.OutputStream; public class App { public static void main(String[] args) throws IOException { HttpServer server = HttpServer.create(new InetSocketAddress(1800), 0); server.createContext("/", (exchange -> { String response = "Hello from Java!"; exchange.sendResponseHeaders(200, response.length()); try (OutputStream os = exchange.getResponseBody()) { os.write(response.getBytes()); } })); server.setExecutor(null); server.start(); System.out.println("Server started on port 1800"); } }
簡単な説明:
-
インポートとセットアップ
- 組み込みの com.sun.net.httpserver パッケージを使用します
- 外部依存関係のないシンプルな HTTP サーバーを作成します
- ポート 1800 で実行
サーバー構成
HttpServer.create()
- 新しいサーバー インスタンスを作成します
InetSocketAddress(1800)
- ポート 1800 にバインドします
- 0 - 接続キューのデフォルトのバックログ値
- リクエストの処理
createContext("/")
- ルート パス "/" へのすべてのリクエストを処理します
- ラムダ式でリクエスト ハンドラーを定義します
- 「Java からこんにちは!」を返します。すべてのリクエスト
-
対応フロー
- レスポンスコード 200 (OK) を設定します
- コンテンツの長さを設定します
- 応答バイトを出力ストリームに書き込みます
- try-with-resources でストリームを自動終了します
サーバー起動
setExecutor(null)
- デフォルトのエグゼキュータを使用します
server.start()
- リクエストのリッスンを開始します
- 確認メッセージを印刷します
ステップ 3
プロジェクトのルートに Dockerfile を作成します:
FROM amazoncorretto:8 WORKDIR /app COPY target/simple-service-1.0-SNAPSHOT.jar app.jar EXPOSE 1800 CMD ["java", "-jar", "app.jar"]
ステップ 4
docker-compose.yml を作成してコンテナをビルドし、ポート 1800 にマップします
services: app: build: . ports: - "1800:1800" restart: unless-stopped
ステップ 5
build.sh を作成します
#!/bin/bash mvn clean package docker compose build docker compose up
ターミナルでこのファイルの実行権限を許可します:
# Install Homebrew if not present /bin/bash -c "$(curl -fsSL https://raw.githubusercontent.com/Homebrew/install/HEAD/install.sh)" # Install Java 8 brew tap homebrew/cask-versions brew install --cask temurin8 # Install Maven brew install maven # Install Docker Desktop brew install --cask docker # Install VS Code brew install --cask visual-studio-code # Install VS Code Extensions code --install-extension vscjava.vscode-java-pack code --install-extension ms-azuretools.vscode-docker
アプリをビルドして実行する
ただ実行するだけです
# Install Chocolatey if not present Set-ExecutionPolicy Bypass -Scope Process -Force; [System.Net.ServicePointManager]::SecurityProtocol = [System.Net.ServicePointManager]::SecurityProtocol -bor 3072; iex ((New-Object System.Net.WebClient).DownloadString('https://chocolatey.org/install.ps1')) # Install Java 8 choco install temurin8 # Install Maven choco install maven # Install Docker Desktop choco install docker-desktop # Install VS Code choco install vscode # Install VS Code Extensions code --install-extension vscjava.vscode-java-pack code --install-extension ms-azuretools.vscode-docker
プロジェクトがビルドされ、イメージが作成され、コンテナが実行されているはずです。
アプリをテストするには、アドレス http://localhost:1800/
でブラウザを開くだけです。コーディングを楽しんでください!
以上がDocker を使用してわずか数分で最初の Java アプリを構築してデプロイするの詳細内容です。詳細については、PHP 中国語 Web サイトの他の関連記事を参照してください。

ホットAIツール

Undresser.AI Undress
リアルなヌード写真を作成する AI 搭載アプリ

AI Clothes Remover
写真から衣服を削除するオンライン AI ツール。

Undress AI Tool
脱衣画像を無料で

Clothoff.io
AI衣類リムーバー

Video Face Swap
完全無料の AI 顔交換ツールを使用して、あらゆるビデオの顔を簡単に交換できます。

人気の記事

ホットツール

メモ帳++7.3.1
使いやすく無料のコードエディター

SublimeText3 中国語版
中国語版、とても使いやすい

ゼンドスタジオ 13.0.1
強力な PHP 統合開発環境

ドリームウィーバー CS6
ビジュアル Web 開発ツール

SublimeText3 Mac版
神レベルのコード編集ソフト(SublimeText3)

ホットトピック










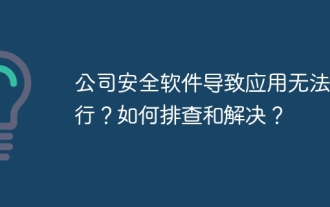
一部のアプリケーションが適切に機能しないようにする会社のセキュリティソフトウェアのトラブルシューティングとソリューション。多くの企業は、内部ネットワークセキュリティを確保するためにセキュリティソフトウェアを展開します。 ...
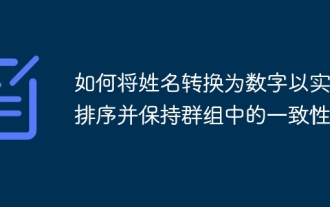
多くのアプリケーションシナリオでソートを実装するために名前を数値に変換するソリューションでは、ユーザーはグループ、特に1つでソートする必要がある場合があります...
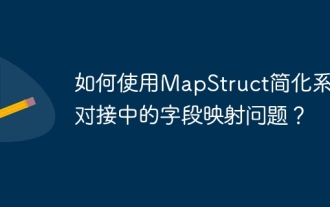
システムドッキングでのフィールドマッピング処理は、システムドッキングを実行する際に難しい問題に遭遇することがよくあります。システムのインターフェイスフィールドを効果的にマッピングする方法A ...
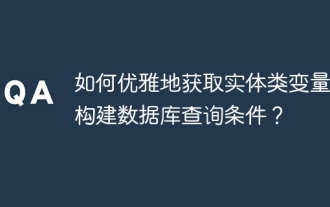
データベース操作にMyBatis-Plusまたはその他のORMフレームワークを使用する場合、エンティティクラスの属性名に基づいてクエリ条件を構築する必要があることがよくあります。あなたが毎回手動で...
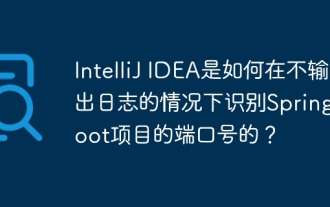
intellijideaultimatiateバージョンを使用してスプリングを開始します...
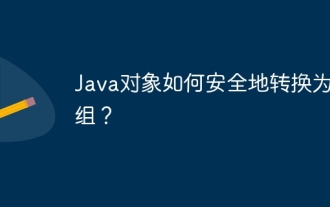
Javaオブジェクトと配列の変換:リスクの詳細な議論と鋳造タイプ変換の正しい方法多くのJava初心者は、オブジェクトのアレイへの変換に遭遇します...
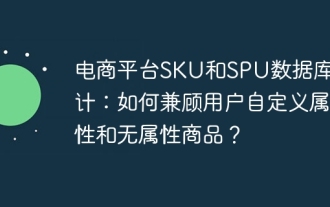
eコマースプラットフォーム上のSKUおよびSPUテーブルの設計の詳細な説明この記事では、eコマースプラットフォームでのSKUとSPUのデータベース設計の問題、特にユーザー定義の販売を扱う方法について説明します。
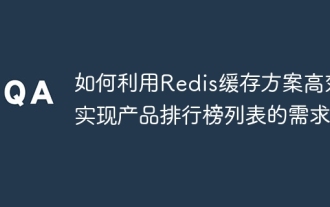
Redisキャッシュソリューションは、製品ランキングリストの要件をどのように実現しますか?開発プロセス中に、多くの場合、ランキングの要件に対処する必要があります。
