ビューの適切な「キャッシュ制御」で Django プロジェクトのセキュリティを強化します
キャッシュにより Django アプリケーションのパフォーマンスが大幅に向上しますが、機密データを保護することが最も重要です。 この記事では、Django ビューでキャッシュ コントロールを効果的に管理し、機密情報がキャッシュされるのを防ぐ方法を説明します。 これは、ログイン画面やユーザー固有の詳細を表示するページなどのページにとって非常に重要です。
キャッシュ制御の重要性
不適切なキャッシュ構成により、機密データがセキュリティ リスクにさらされます。 適切な設定がないと、この情報がユーザーのブラウザまたは中間プロキシに保存され、脆弱性が生じる可能性があります。
Django でのキャッシュ制御の実装
@never_cache
デコレーターは、Django の公式ドキュメントに記載されているように、関数ベースのビューがキャッシュされるのを防ぎます。
from django.views.decorators.cache import never_cache @never_cache def my_secure_view(request): # Secure view logic here return HttpResponse("This page is protected from caching!")
複数のクラスベースのビュー間で再利用性を高めるために、カスタム ミックスインはよりクリーンなソリューションを提供します。
# myproject/views.py from django.contrib.auth.mixins import LoginRequiredMixin from django.utils.decorators import method_decorator from django.views.decorators.cache import never_cache @method_decorator(never_cache, name="dispatch") class PrivateAreaMixin(LoginRequiredMixin): """Extends LoginRequiredMixin with Cache-Control directives."""
このミックスインにより、クラスベースのビューの保護が簡素化されます:
# myapp/views.py from django.views.generic import TemplateView from myproject.views import PrivateAreaMixin class IndexView(PrivateAreaMixin, TemplateView): """Example index view.""" template_name = "index.html"
堅牢なセキュリティのための徹底したテスト
PrivateAreaMixin
の機能を検証するには、包括的なテストが不可欠です。 次の例は、堅牢なテスト スイートを示しています。
# myproject/tests/test_views.py from django.test import TestCase, RequestFactory from django.contrib.auth.models import AnonymousUser from django.contrib.auth import get_user_model from django.http import HttpResponse from django.views import View from myproject.views import PrivateAreaMixin class PrivateAreaMixinTest(TestCase): """Tests the PrivateAreaMixin's Cache-Control implementation.""" factory = RequestFactory() @classmethod def setUpTestData(cls): cls.user = get_user_model().objects.create_user( username="testuser", email="user@test.xyz", password="5tr0ngP4ssW0rd", ) def test_login_required_with_cache_control(self): class AView(PrivateAreaMixin, View): def get(self, request, *args, **kwargs): return HttpResponse() view = AView.as_view() # Test redirection for unauthenticated users request = self.factory.get("/") request.user = AnonymousUser() response = view(request) self.assertEqual(response.status_code, 302) self.assertEqual("/accounts/login/?next=/", response.url) # Test authenticated user and Cache-Control headers request = self.factory.get("/") request.user = self.user response = view(request) self.assertEqual(response.status_code, 200) self.assertIn("Cache-Control", response.headers) self.assertEqual( response.headers["Cache-Control"], "max-age=0, no-cache, no-store, must-revalidate, private", )
ベストプラクティス
@never_cache
と PrivateAreaMixin
のような再利用可能なミックスインを組み合わせると、クリーンで保守可能なコードが得られます。 このアプローチは、厳格なテストと組み合わせることで、機密ビューが安全であり、ベスト プラクティスに準拠していることを保証します。 Django プロジェクトでキャッシュと機密データにどのように対処しますか?
以上がビューの適切な「キャッシュ制御」で Django プロジェクトのセキュリティを強化しますの詳細内容です。詳細については、PHP 中国語 Web サイトの他の関連記事を参照してください。

ホットAIツール

Undresser.AI Undress
リアルなヌード写真を作成する AI 搭載アプリ

AI Clothes Remover
写真から衣服を削除するオンライン AI ツール。

Undress AI Tool
脱衣画像を無料で

Clothoff.io
AI衣類リムーバー

Video Face Swap
完全無料の AI 顔交換ツールを使用して、あらゆるビデオの顔を簡単に交換できます。

人気の記事

ホットツール

メモ帳++7.3.1
使いやすく無料のコードエディター

SublimeText3 中国語版
中国語版、とても使いやすい

ゼンドスタジオ 13.0.1
強力な PHP 統合開発環境

ドリームウィーバー CS6
ビジュアル Web 開発ツール

SublimeText3 Mac版
神レベルのコード編集ソフト(SublimeText3)

ホットトピック










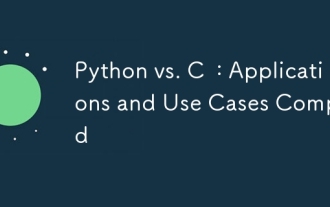
Pythonは、データサイエンス、Web開発、自動化タスクに適していますが、Cはシステムプログラミング、ゲーム開発、組み込みシステムに適しています。 Pythonは、そのシンプルさと強力なエコシステムで知られていますが、Cは高性能および基礎となる制御機能で知られています。
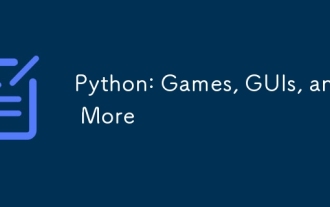
PythonはゲームとGUI開発に優れています。 1)ゲーム開発は、2Dゲームの作成に適した図面、オーディオ、その他の機能を提供し、Pygameを使用します。 2)GUI開発は、TKINTERまたはPYQTを選択できます。 TKINTERはシンプルで使いやすく、PYQTは豊富な機能を備えており、専門能力開発に適しています。
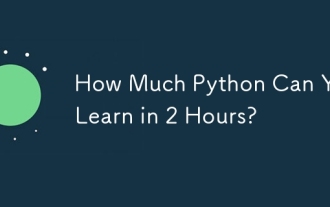
2時間以内にPythonの基本を学ぶことができます。 1。変数とデータ型を学習します。2。ステートメントやループの場合などのマスター制御構造、3。関数の定義と使用を理解します。これらは、簡単なPythonプログラムの作成を開始するのに役立ちます。
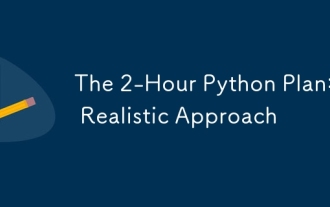
2時間以内にPythonの基本的なプログラミングの概念とスキルを学ぶことができます。 1.変数とデータ型、2。マスターコントロールフロー(条件付きステートメントとループ)、3。機能の定義と使用を理解する4。
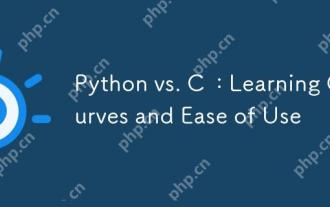
Pythonは学習と使用が簡単ですが、Cはより強力ですが複雑です。 1。Python構文は簡潔で初心者に適しています。動的なタイピングと自動メモリ管理により、使いやすくなりますが、ランタイムエラーを引き起こす可能性があります。 2.Cは、高性能アプリケーションに適した低レベルの制御と高度な機能を提供しますが、学習しきい値が高く、手動メモリとタイプの安全管理が必要です。
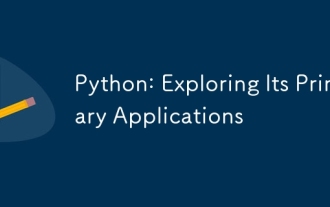
Pythonは、Web開発、データサイエンス、機械学習、自動化、スクリプトの分野で広く使用されています。 1)Web開発では、DjangoおよびFlask Frameworksが開発プロセスを簡素化します。 2)データサイエンスと機械学習の分野では、Numpy、Pandas、Scikit-Learn、Tensorflowライブラリが強力なサポートを提供します。 3)自動化とスクリプトの観点から、Pythonは自動テストやシステム管理などのタスクに適しています。
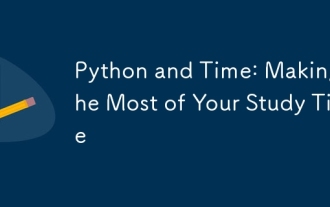
限られた時間でPythonの学習効率を最大化するには、PythonのDateTime、時間、およびスケジュールモジュールを使用できます。 1. DateTimeモジュールは、学習時間を記録および計画するために使用されます。 2。時間モジュールは、勉強と休息の時間を設定するのに役立ちます。 3.スケジュールモジュールは、毎週の学習タスクを自動的に配置します。
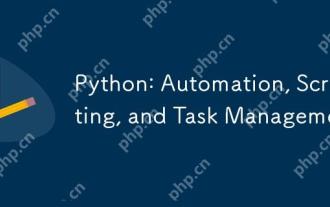
Pythonは、自動化、スクリプト、およびタスク管理に優れています。 1)自動化:OSやShutilなどの標準ライブラリを介してファイルバックアップが実現されます。 2)スクリプトの書き込み:Psutilライブラリを使用してシステムリソースを監視します。 3)タスク管理:スケジュールライブラリを使用してタスクをスケジュールします。 Pythonの使いやすさと豊富なライブラリサポートにより、これらの分野で優先ツールになります。
