シンプルで実用的で便利な ajax コメントの完全なコード
简单的ajax评论完整代码
数据库结构CREATE TABLE `comments` (
`id` int(10) unsigned NOT NULL auto_increment,
`name` varchar(128) collate utf8_unicode_ci NOT NULL default '',
`url` varchar(255) collate utf8_unicode_ci NOT NULL default '',
`email` varchar(255) collate utf8_unicode_ci NOT NULL default '',
`body` text collate utf8_unicode_ci NOT NULL,
`dt` timestamp NOT NULL default CURRENT_TIMESTAMP,
PRIMARY KEY (`id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8 COLLATE=utf8_unicode_ci AUTO_INCREMENT=1;
演示
PHP
[php]
// Error reporting:
error_reporting(E_ALL^E_NOTICE);
include "conn.php";
include "comment.class.php";
/* (PS:^_^不错的php学习交流群:276167802,验证:csl,有兴趣的话可以加入进来一起讨论)
/ Select all the comments and populate the $comments array with objects
*/
$comments = array();
$result = mysql_query("SELECT * FROM comments ORDER BY id ASC");
while($row = mysql_fetch_assoc($result))
{
$comments[] = new Comment($row);
}
?>
PHP
[php]
/*
/ Output the comments one by one:
*/
foreach($comments as $c){
echo $c->markup();
}
?>
Add a Comment
submit.php
PHP
[php]
// Error reporting:
error_reporting(E_ALL^E_NOTICE);
include "conn.php";
include "comment.class.php";
/*
/ This array is going to be populated with either
/ the data that was sent to the script, or the
/ error messages.
/*/
$arr = array();
$validates = Comment::validate($arr);
if($validates)
{
/* Everything is OK, insert to database: */
mysql_query(" INSERT INTO comments(name,url,email,body)
VALUES (
'".$arr['name']."',
'".$arr['url']."',
'".$arr['email']."',
'".$arr['body']."'
)");
$arr['dt'] = date('r',time());
$arr['id'] = mysql_insert_id();
/*
/ The data in $arr is escaped for the mysql query,
/ but we need the unescaped variables, so we apply,
/ stripslashes to all the elements in the array:
/*/
$arr = array_map('stripslashes',$arr);
$insertedComment = new Comment($arr);
/* Outputting the markup of the just-inserted comment: */
echo json_encode(array('status'=>1,'html'=>$insertedComment->markup()));
}
else
{
/* Outputtng the error messages */
echo '{"status":0,"errors":'.json_encode($arr).'}';
}
?>
comment.class.php
PHP
[php]
class Comment
{
private $data = array();
public function __construct($row)
{
/*
/ The constructor
*/
$this->data = $row;
}
public function markup()
{
/*
/ This method outputs the XHTML markup of the comment
*/
// Setting up an alias, so we don't have to write $this->data every time:
$d = &$this->data;
$link_open = '';
$link_close = '';
if($d['url']){
// If the person has entered a URL when adding a comment,
// define opening and closing hyperlink tags
$link_open = '';
$link_close = '';
}
// Converting the time to a UNIX timestamp:
$d['dt'] = strtotime($d['dt']);
// Needed for the default gravatar image:
$url = 'http://'.dirname($_SERVER['SERVER_NAME'].$_SERVER["REQUEST_URI"]).'/img/default_avatar.gif';
return '
';
}
パブリック静的関数 validate(&$arr)
{
/*
/ このメソッドは、AJAX 経由で送信されたデータを検証するために使用されます。
/
/ データが有効かどうかに応じて true/false を返し、
に値を入力します。/
でパラメータとして渡される $arr 配列 (上のアンパサンドに注目してください)/ 有効な入力データまたはエラー メッセージ。
*/
$errors = array();
$data = array();
// PHP 5.2.0 で導入された filter_input 関数を使用する
if(!($data['email'] = filter_input(INPUT_POST,'email',FILTER_VALIDATE_EMAIL)))
{
$errors['email'] = '有効な電子メールを入力してください。';
}
if(!($data['url'] = filter_input(INPUT_POST,'url',FILTER_VALIDATE_URL)))
{
// URL フィールドに有効な URL が入力されていない場合、
// URL がまったく入力されなかったかのように動作します:
$url = '';
}
// カスタム コールバック関数でフィルターを使用する:
if(!($data['body'] = filter_input(INPUT_POST,'body',FILTER_CALLBACK,array('options'=>'Comment::validate_text'))))
{
$errors['body'] = 'コメント本文を入力してください。';
}
if(!($data['name'] = filter_input(INPUT_POST,'name',FILTER_CALLBACK,array('options'=>'Comment::validate_text'))))
{
$errors['name'] = '名前を入力してください。';
}
if(!empty($errors)){
// エラーがある場合は、$errors 配列を $arr にコピーします:
$arr = $errors;
false を返します。
}
// データが有効な場合は、すべてのデータをサニタイズして $arr にコピーします:
foreach($data as $k=>$v){
$arr[$k] = mysql_real_escape_string($v);
}
// メールが小文字であることを確認してください:
$arr['email'] = strto lower(trim($arr['email']));
true を返します。
}
プライベート静的関数 validate_text($str)
{
/*
/ このメソッドは FILTER_CALLBACK として内部的に使用されます
*/
if(mb_strlen($str,'utf8')<1)
false を返します。
// すべての HTML 特殊文字 (<、>、"、& .. など) をエンコードし、変換します
//
に改行文字を入力します。タグ:
$str = nl2br(htmlspecialchars($str));
// 残った改行文字を削除します
$str = str_replace(array(chr(10),chr(13)),'',$str);
$str を返す;
}
}
?>
以上は、ajax 認証に関する完全なコードであり、大php の公開者にお役立てください。

ホットAIツール

Undresser.AI Undress
リアルなヌード写真を作成する AI 搭載アプリ

AI Clothes Remover
写真から衣服を削除するオンライン AI ツール。

Undress AI Tool
脱衣画像を無料で

Clothoff.io
AI衣類リムーバー

AI Hentai Generator
AIヘンタイを無料で生成します。

人気の記事

ホットツール

メモ帳++7.3.1
使いやすく無料のコードエディター

SublimeText3 中国語版
中国語版、とても使いやすい

ゼンドスタジオ 13.0.1
強力な PHP 統合開発環境

ドリームウィーバー CS6
ビジュアル Web 開発ツール

SublimeText3 Mac版
神レベルのコード編集ソフト(SublimeText3)

ホットトピック








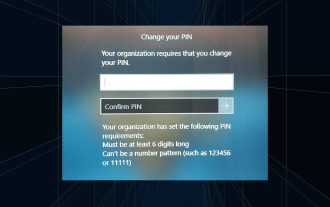
ログイン画面に「組織から PIN の変更を求められています」というメッセージが表示されます。これは、個人のデバイスを制御できる組織ベースのアカウント設定を使用しているコンピューターで PIN の有効期限の制限に達した場合に発生します。ただし、個人アカウントを使用して Windows をセットアップした場合、エラー メッセージは表示されないのが理想的です。常にそうとは限りませんが。エラーが発生したほとんどのユーザーは、個人アカウントを使用して報告します。私の組織が Windows 11 で PIN を変更するように要求するのはなぜですか?アカウントが組織に関連付けられている可能性があるため、主なアプローチはこれを確認することです。ドメイン管理者に問い合わせると解決できます。さらに、ローカル ポリシー設定が間違っていたり、レジストリ キーが間違っていたりすると、エラーが発生する可能性があります。今すぐ
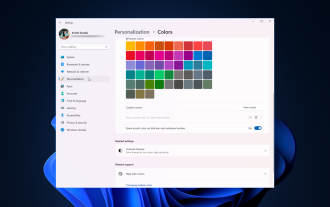
Windows 11 では、新鮮でエレガントなデザインが前面に押し出されており、最新のインターフェイスにより、ウィンドウの境界線などの細部をカスタマイズして変更することができます。このガイドでは、Windows オペレーティング システムで自分のスタイルを反映した環境を作成するのに役立つ手順について説明します。ウィンドウの境界線の設定を変更するにはどうすればよいですか? + を押して設定アプリを開きます。 Windows [個人用設定] に移動し、[色の設定] をクリックします。ウィンドウの境界線の色の変更設定ウィンドウ 11" width="643" height="500" > [タイトル バーとウィンドウの境界線にアクセント カラーを表示する] オプションを見つけて、その横にあるスイッチを切り替えます。 [スタート] メニューとタスク バーにアクセント カラーを表示するにはスタート メニューとタスク バーにテーマの色を表示するには、[スタート メニューとタスク バーにテーマを表示] をオンにします。
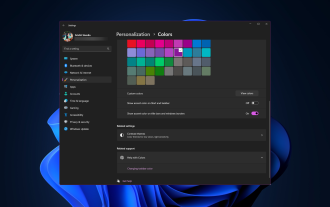
デフォルトでは、Windows 11 のタイトル バーの色は、選択したダーク/ライト テーマによって異なります。ただし、任意の色に変更できます。このガイドでは、デスクトップ エクスペリエンスを変更し、視覚的に魅力的なものにするためにカスタマイズする 3 つの方法について、段階的な手順を説明します。アクティブなウィンドウと非アクティブなウィンドウのタイトル バーの色を変更することはできますか?はい、設定アプリを使用してアクティブなウィンドウのタイトル バーの色を変更したり、レジストリ エディターを使用して非アクティブなウィンドウのタイトル バーの色を変更したりできます。これらの手順を学習するには、次のセクションに進んでください。 Windows 11でタイトルバーの色を変更するにはどうすればよいですか? 1. 設定アプリを使用して + を押して設定ウィンドウを開きます。 Windows「個人用設定」に進み、
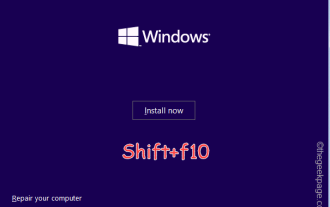
Windows インストーラー ページに「問題が発生しました」というメッセージとともに「OOBELANGUAGE」というメッセージが表示されますか?このようなエラーが原因で Windows のインストールが停止することがあります。 OOBE とは、すぐに使えるエクスペリエンスを意味します。エラー メッセージが示すように、これは OOBE 言語の選択に関連する問題です。心配する必要はありません。OOBE 画面自体から気の利いたレジストリ編集を行うことで、この問題を解決できます。クイックフィックス – 1. OOBE アプリの下部にある [再試行] ボタンをクリックします。これにより、問題が発生することなくプロセスが続行されます。 2. 電源ボタンを使用してシステムを強制的にシャットダウンします。システムの再起動後、OOBE が続行されます。 3. システムをインターネットから切断します。 OOBE のすべての側面をオフライン モードで完了する
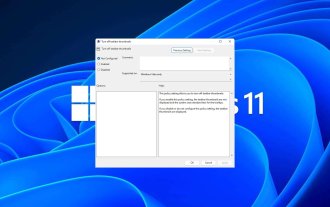
タスクバーのサムネイルは楽しい場合もありますが、気が散ったり煩わしい場合もあります。この領域にマウスを移動する頻度を考えると、重要なウィンドウを誤って閉じてしまったことが何度かある可能性があります。もう 1 つの欠点は、より多くのシステム リソースを使用することです。そのため、リソース効率を高める方法を探している場合は、それを無効にする方法を説明します。ただし、ハードウェアの仕様が対応可能で、プレビューが気に入った場合は、有効にすることができます。 Windows 11でタスクバーのサムネイルプレビューを有効にする方法は? 1. 設定アプリを使用してキーをタップし、[設定] をクリックします。 Windows では、「システム」をクリックし、「バージョン情報」を選択します。 「システムの詳細設定」をクリックします。 [詳細設定] タブに移動し、[パフォーマンス] の下の [設定] を選択します。 「視覚効果」を選択します
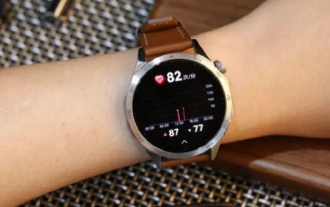
多くのユーザーはスマートウォッチを選ぶときにファーウェイブランドを選択しますが、その中でもファーウェイ GT3pro と GT4 は非常に人気のある選択肢であり、多くのユーザーはファーウェイ GT3pro と GT4 の違いに興味を持っています。 Huawei GT3pro と GT4 の違いは何ですか? 1. 外観 GT4: 46mm と 41mm、材質はガラスミラー + ステンレススチールボディ + 高解像度ファイバーバックシェルです。 GT3pro: 46.6mm および 42.9mm、材質はサファイアガラス + チタンボディ/セラミックボディ + セラミックバックシェルです。 2. 健全な GT4: 最新の Huawei Truseen5.5+ アルゴリズムを使用すると、結果はより正確になります。 GT3pro: ECG 心電図と血管と安全性を追加
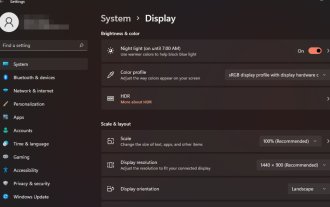
Windows 11 のディスプレイ スケーリングに関しては、好みが人それぞれ異なります。大きなアイコンを好む人もいれば、小さなアイコンを好む人もいます。ただし、適切なスケーリングが重要であることには誰もが同意します。フォントのスケーリングが不十分であったり、画像が過度にスケーリングされたりすると、作業中の生産性が大幅に低下する可能性があるため、システムの機能を最大限に活用するためにカスタマイズする方法を知る必要があります。カスタム ズームの利点: これは、画面上のテキストを読むのが難しい人にとって便利な機能です。一度に画面上でより多くの情報を確認できるようになります。特定のモニターおよびアプリケーションにのみ適用するカスタム拡張プロファイルを作成できます。ローエンド ハードウェアのパフォーマンスの向上に役立ちます。画面上の内容をより詳細に制御できるようになります。 Windows 11の使用方法
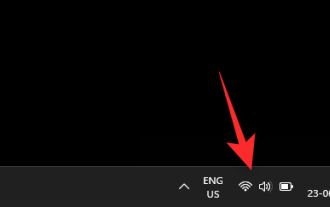
画面の明るさは、最新のコンピューティング デバイスを使用する上で不可欠な部分であり、特に長時間画面を見る場合には重要です。目の疲れを軽減し、可読性を向上させ、コンテンツを簡単かつ効率的に表示するのに役立ちます。ただし、設定によっては、特に新しい UI が変更された Windows 11 では、明るさの管理が難しい場合があります。明るさの調整に問題がある場合は、Windows 11 で明るさを管理するすべての方法を次に示します。 Windows 11で明るさを変更する方法【10の方法を解説】 シングルモニターユーザーは、次の方法でWindows 11の明るさを調整できます。これには、ラップトップだけでなく、単一のモニターを使用するデスクトップ システムも含まれます。はじめましょう。方法 1: アクション センターを使用する アクション センターにアクセスできる

'.$link_open.'
'.$link_close.'
'.$d['body'].'