Android アニメーション学習 - TweenAnimation_html/css_WEB-ITnose
目次
Android には 3 種類のアニメーションがあります:
- トゥイーン アニメーション: シーン内のオブジェクトに対して画像変換 (移動、拡大縮小、回転) を継続的に実行することによって生成されるアニメーション効果は、グラデーションアニメーションの一種。
- フレームアニメーション: 画面遷移アニメーションの一種で、あらかじめ作成された画像を順番に再生します。
- プロパティ アニメーション: オブジェクトのプロパティを動的に変更することでアニメーション効果を実現するプロパティ アニメーションは、API 11 の新機能です。
このブログは主に Tween アニメーションの基本的な使い方を分析します。
トゥイーン アニメーショントゥイーン アニメーションには 4 つの形式があります:
- アルファ: グラデーション透明アニメーション効果。
- スケール: グラデーションサイズの拡大と縮小のアニメーション効果。
- 翻訳: 画面位置移動アニメーション効果。
- rotate: 画面回転アニメーション効果。
これら 4 つのアニメーション実装メソッドはすべて、Animation クラスとAnimationUtils の連携によって実装されます。アニメーション効果は、res/anim ディレクトリ内の xml ファイルで事前に設定できます。
scale アニメーション? サイズを調整します
scale のパラメータは次のとおりです:
さらに、Animation クラスは、Animation クラスから継承されたすべてのアニメーション (スケール、アルファ、移動、回転) の基本クラスです:
練習せずに話すだけでは十分ではありません。スケール アニメーションの使用を練習するための Anim XML ファイル、レイアウト ファイル、Activity クラスを紹介します。
res/anim/anim_scale.xml ファイルは次のとおりです:
<?xml version="1.0" encoding="utf-8"?><scale xmlns:android="http://schemas.android.com/apk/res/android" android:duration="700" android:fromXScale="0.0" android:fromYScale="0.0" android:pivotX="150" android:pivotY="300" android:toXScale="1.4" android:toYScale="1.4" android:fillAfter="true" android:repeatCount="10" android:repeatMode="reverse"></scale>
res/layout/tween_animation_layout.xml ファイルは次のとおりです:
<?xml version="1.0" encoding="utf-8"?><LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical" > <LinearLayout android:layout_width="match_parent" android:layout_height="wrap_content" android:orientation="horizontal" > <Button android:id="@+id/alpha_button" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_weight="1" android:text="@string/alpha" /> <Button android:id="@+id/scale_button" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_weight="1" android:text="@string/scale" /> <Button android:id="@+id/rotate_button" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_weight="1" android:text="@string/rotate" /> <Button android:id="@+id/trans_button" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_weight="1" android:text="@string/trans" /> </LinearLayout> <ImageView android:id="@+id/anim_image" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_gravity="center_horizontal" android:contentDescription="@null" android:src="@drawable/splash_logo" /></LinearLayout>
アニメーションのデモ実装クラスは次のように実装されます:
import com.example.photocrop.R;import android.app.Activity;import android.os.Bundle;import android.view.View;import android.view.View.OnClickListener;import android.view.animation.Animation;import android.view.animation.AnimationUtils;import android.widget.Button;import android.widget.ImageView;public class TweenAnimationTest extends Activity implements OnClickListener { private Button alphaButton; private Button scaleButton; private Button rotateButton; private Button transButton; private ImageView animImageView; @Override protected void onCreate(Bundle savedInstanceState) { // TODO Auto-generated method stub super.onCreate(savedInstanceState); setContentView(R.layout.tween_animation_layout); initView(); } private void initView() { animImageView = (ImageView) findViewById(R.id.anim_image); alphaButton = (Button) findViewById(R.id.alpha_button); scaleButton = (Button) findViewById(R.id.scale_button); rotateButton = (Button) findViewById(R.id.rotate_button); transButton = (Button) findViewById(R.id.trans_button); alphaButton.setOnClickListener(this); scaleButton.setOnClickListener(this); rotateButton.setOnClickListener(this); transButton.setOnClickListener(this); } @Override public void onClick(View v) { switch (v.getId()) { case R.id.alpha_button: break; case R.id.scale_button: Animation animation = AnimationUtils.loadAnimation(this, R.anim.anim_scale); animImageView.startAnimation(animation); break; case R.id.rotate_button: break; case R.id.trans_button: break; } }}
alpha アニメーションを調整しますか?透明度
alpha パラメータ 以下の通り:
Animation クラスから継承されたプロパティ:
アルファ ボタンをクリックすると、透明アニメーション効果をトリガーします。
res/anim/anim_alpha.xml ファイルは次のとおりです:
<?xml version="1.0" encoding="utf-8"?><alpha xmlns:android="http://schemas.android.com/apk/res/android" android:duration="500" android:fillBefore="true" android:fromAlpha="0.0" android:toAlpha="1.0" ></alpha>
アルファ ボタンのクリックに応答するリスナー イベントは次のとおりです:
case R.id.alpha_button: Animation alphaAnimation = AnimationUtils.loadAnimation(this, R.anim.anim_alpha); animImageView.startAnimation(alphaAnimation); break;
回転アニメーション?
回転パラメータは次のとおりです:android: fromDegrees: 回転を開始する角度位置。正の値は時計回りの角度を表し、負の値は反時計回りの角度を表します。
res/anim/anim_rotate.xml文件如下:
<?xml version="1.0" encoding="utf-8"?><rotate xmlns:android="http://schemas.android.com/apk/res/android" android:duration="1000" android:fillAfter="true" android:fromDegrees="0" android:pivotX="0" android:pivotY="0" android:repeatCount="3" android:toDegrees="810" ></rotate>
响应点击alpha button的listener事件为:
case R.id.rotate_button: Animation rotateAnimation = AnimationUtils.loadAnimation(this, R.anim.anim_rotate); animImageView.startAnimation(rotateAnimation); break;
translate动画?平移
translate参数:
从Animation类继承的属性:
注意:京东splash页面的进度条用的就是translate动画。
res/anim/anim_rotate.xml文件如下:
<?xml version="1.0" encoding="utf-8"?><translate xmlns:android="http://schemas.android.com/apk/res/android" android:duration="2000" android:fillAfter="true" android:fromXDelta="0.0" android:toXDelta="60%p" ></translate>
响应点击alpha button的listener事件为:
case R.id.trans_button: Animation transAnimation = AnimationUtils.loadAnimation(this, R.anim.anim_translate); animImageView.startAnimation(transAnimation); break;

ホットAIツール

Undresser.AI Undress
リアルなヌード写真を作成する AI 搭載アプリ

AI Clothes Remover
写真から衣服を削除するオンライン AI ツール。

Undress AI Tool
脱衣画像を無料で

Clothoff.io
AI衣類リムーバー

AI Hentai Generator
AIヘンタイを無料で生成します。

人気の記事

ホットツール

メモ帳++7.3.1
使いやすく無料のコードエディター

SublimeText3 中国語版
中国語版、とても使いやすい

ゼンドスタジオ 13.0.1
強力な PHP 統合開発環境

ドリームウィーバー CS6
ビジュアル Web 開発ツール

SublimeText3 Mac版
神レベルのコード編集ソフト(SublimeText3)

ホットトピック








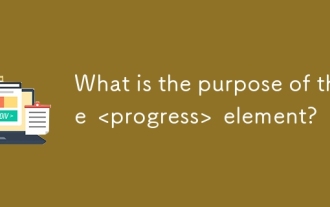
この記事では、HTML&lt; Progress&gt;について説明します。要素、その目的、スタイリング、および&lt; meter&gt;との違い要素。主な焦点は、&lt; Progress&gt;を使用することです。タスクの完了と&lt; Meter&gt; statiの場合
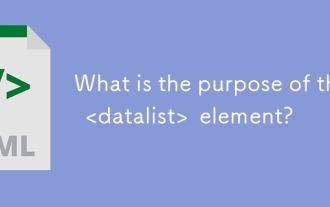
この記事では、HTML&lt; Datalist&GT;について説明します。オートコンプリートの提案を提供し、ユーザーエクスペリエンスの改善、エラーの削減によりフォームを強化する要素。
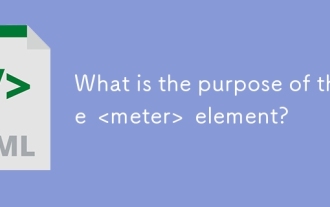
この記事では、html&lt; meter&gt;について説明します。要素は、範囲内でスカラーまたは分数値を表示するために使用され、Web開発におけるその一般的なアプリケーション。それは差別化&lt; Meter&gt; &lt; Progress&gt;およびex
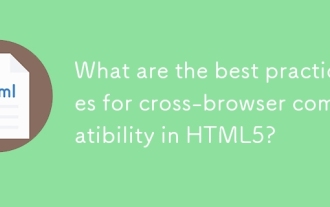
記事では、HTML5クロスブラウザーの互換性を確保するためのベストプラクティスについて説明し、機能検出、プログレッシブエンハンスメント、およびテスト方法に焦点を当てています。
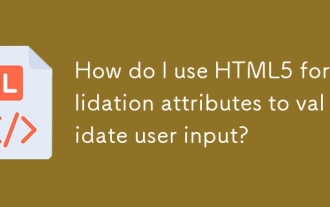
この記事では、ブラウザのユーザー入力を直接検証するために、必要、パターン、MIN、MAX、および長さの制限などのHTML5フォーム検証属性を使用して説明します。
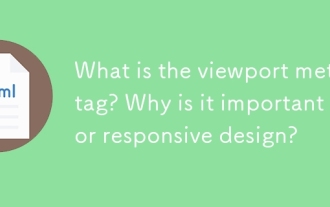
この記事では、モバイルデバイスのレスポンシブWebデザインに不可欠なViewportメタタグについて説明します。適切な使用により、最適なコンテンツのスケーリングとユーザーの相互作用が保証され、誤用が設計とアクセシビリティの問題につながる可能性があることを説明しています。
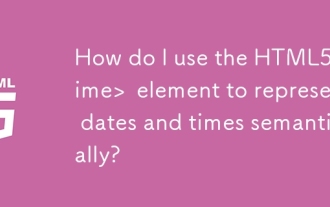
この記事では、html5&lt; time&gt;について説明します。セマンティックデート/時刻表現の要素。 人間の読み取り可能なテキストとともに、マシンの読みやすさ(ISO 8601形式)のDateTime属性の重要性を強調し、Accessibilitを増やします
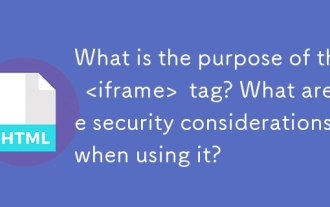
この記事では、&lt; iframe&gt;外部コンテンツをWebページ、その一般的な用途、セキュリティリスク、およびオブジェクトタグやAPIなどの代替案に埋め込む際のタグの目的。
