JSオブジェクト指向プログラミング_JavaScriptスキルを詳しく解説
JavaScript はプロトタイプに基づいており、クラスの概念がないため (ES6 にはクラスの概念があります。今は説明しません)、アクセスできるのはオブジェクトだけであり、すべてが真のオブジェクトです
したがって、オブジェクトについて話すとき、多くの学生は型付きオブジェクトとオブジェクト自体の概念を混同します。理解を容易にするために、以下の用語ではオブジェクトについては言及しません。 🎜>
方法 1
クラス (関数シミュレーション)
function Person(name,id){ //实例变量可以被继承 this.name = name; //私有变量无法被继承 var id = id; //私有函数无法被继承 function speak(){ alert("person1"); } } //静态变量,无法被继承 Person.age = 18; //公有函数可以被继承 Person.prototype.say = function(){ alert("person2"); }
function Person(name,id){ //实例变量可以被继承 this.name = name; //私有变量无法被继承 var id = id; //私有函数无法被继承 function speak(){ alert("person1"); } } //静态变量,无法被继承 Person.age = 18; //公有静态成员可被继承 Person.prototype.sex = "男"; //公有静态函数可以被继承 Person.prototype.say = function(){ alert("person2"); } //创建子类 function Student(){ } //继承person Student.prototype = new Person("iwen",1); //修改因继承导致的constructor变化 Student.prototype.constructor = Student; var s = new Student(); alert(s.name);//iwen alert(s instanceof Person);//true s.say();//person2
function Person(name,id){ //实例变量可以被继承 this.name = name; //私有变量无法被继承 var id = id; //私有函数无法被继承 function speak(){ alert("person1"); } } //静态变量,无法被继承 Person.age = 18; //公有静态成员可被继承 Person.prototype.sex = "男"; //公有静态函数可以被继承 Person.prototype.say = function(){ alert("person2"); } //创建子类 function Student(){ } //继承person Student.prototype = new Person("iwen",1); //修改因继承导致的constructor变化 Student.prototype.constructor = Student; //保存父类的引用 var superPerson = Student.prototype.say; //复写父类的方法 Student.prototype.say = function(){ //调用父类的方法 superPerson.call(this); alert("Student"); } //创建实例测试 var s = new Student(); alert(s instanceof Person);//true s.say();//person2 student
function extend(Child, Parent) { var F = function(){}; F.prototype = Parent.prototype; Child.prototype = new F(); Child.prototype.constructor = Child; Child.uber = Parent.prototype; }
方法 2
コピーと同じで、継承したいオブジェクトは定義された _this オブジェクトを介して引き継がれます。この場合、_this を渡すことで継承が実現できます。これは、上記のよりも理解しやすいです。
//创建父类 function Person(name,id){ //创建一个对象来承载父类所有公有东西 //也就是说_this承载的对象才会被传递给子类 var _this = {}; _this.name = name; //这样的是不会传递下去的 this.id = id; //承载方法 _this.say = function(){ alert("Person"); } //返回_this对象 return _this; } //子类 function Student(){ //获取person的_this对象,从而模仿继承 var _this = Person("iwne",1); //保存父类的_this引用 var superPerson = _this.say; //复写父类的方法 _this.say = function(){ //执行父类的say superPerson.call(_this); alert("Student"); } return _this; } var s = new Student(); s.say();

ホットAIツール

Undresser.AI Undress
リアルなヌード写真を作成する AI 搭載アプリ

AI Clothes Remover
写真から衣服を削除するオンライン AI ツール。

Undress AI Tool
脱衣画像を無料で

Clothoff.io
AI衣類リムーバー

Video Face Swap
完全無料の AI 顔交換ツールを使用して、あらゆるビデオの顔を簡単に交換できます。

人気の記事

ホットツール

メモ帳++7.3.1
使いやすく無料のコードエディター

SublimeText3 中国語版
中国語版、とても使いやすい

ゼンドスタジオ 13.0.1
強力な PHP 統合開発環境

ドリームウィーバー CS6
ビジュアル Web 開発ツール

SublimeText3 Mac版
神レベルのコード編集ソフト(SublimeText3)

ホットトピック








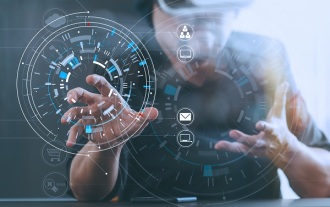
顔の検出および認識テクノロジーは、すでに比較的成熟しており、広く使用されているテクノロジーです。現在、最も広く使用されているインターネット アプリケーション言語は JS ですが、Web フロントエンドでの顔検出と認識の実装には、バックエンドの顔認識と比較して利点と欠点があります。利点としては、ネットワーク インタラクションの削減とリアルタイム認識により、ユーザーの待ち時間が大幅に短縮され、ユーザー エクスペリエンスが向上することが挙げられます。欠点としては、モデル サイズによって制限されるため、精度も制限されることが挙げられます。 js を使用して Web 上に顔検出を実装するにはどうすればよいですか? Web 上で顔認識を実装するには、JavaScript、HTML、CSS、WebRTC など、関連するプログラミング言語とテクノロジに精通している必要があります。同時に、関連するコンピューター ビジョンと人工知能テクノロジーを習得する必要もあります。 Web 側の設計により、次の点に注意してください。
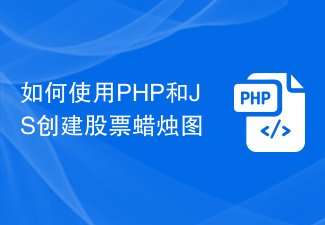
PHP と JS を使用して株のローソク足チャートを作成する方法。株のローソク足チャートは、株式市場で一般的なテクニカル分析グラフィックです。始値、終値、最高値、株価などのデータを描画することで、投資家が株式をより直観的に理解するのに役立ちます。株価の最低価格、価格変動。この記事では、PHP と JS を使用して株価のローソク足チャートを作成する方法を、具体的なコード例とともに説明します。 1. 準備 開始する前に、次の環境を準備する必要があります。 1. PHP を実行するサーバー 2. HTML5 および Canvas をサポートするブラウザー 3
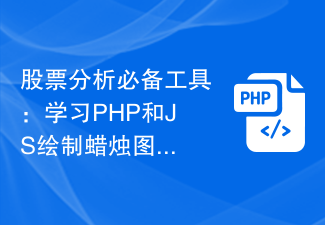
株式分析に必須のツール: PHP および JS でローソク足チャートを描画する手順を学びます。特定のコード例が必要です。インターネットとテクノロジーの急速な発展に伴い、株式取引は多くの投資家にとって重要な方法の 1 つになりました。株価分析は投資家の意思決定の重要な部分であり、ローソク足チャートはテクニカル分析で広く使用されています。 PHP と JS を使用してローソク足チャートを描画する方法を学ぶと、投資家がより適切な意思決定を行うのに役立つ、より直感的な情報が得られます。ローソク足チャートとは、株価をローソク足の形で表示するテクニカルチャートです。株価を示しています
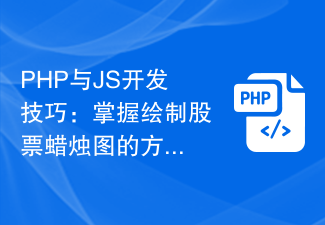
インターネット金融の急速な発展に伴い、株式投資を選択する人がますます増えています。株式取引では、ローソク足チャートは一般的に使用されるテクニカル分析手法であり、株価の変化傾向を示し、投資家がより正確な意思決定を行うのに役立ちます。この記事では、PHP と JS の開発スキルを紹介し、株価ローソク足チャートの描画方法を読者に理解してもらい、具体的なコード例を示します。 1. 株のローソク足チャートを理解する 株のローソク足チャートの描き方を紹介する前に、まずローソク足チャートとは何かを理解する必要があります。ローソク足チャートは日本人が開発した
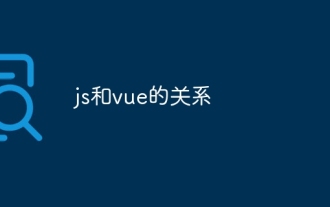
js と vue の関係: 1. Web 開発の基礎としての JS、2. フロントエンド フレームワークとしての Vue.js の台頭、3. JS と Vue の補完関係、4. JS と Vue の実用化ビュー。
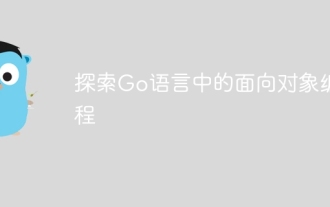
Go 言語は、型定義とメソッドの関連付けを通じてオブジェクト指向プログラミングをサポートします。従来の継承はサポートされていませんが、合成を通じて実装されます。インターフェイスは型間の一貫性を提供し、抽象メソッドを定義できるようにします。実際の事例では、顧客操作の作成、取得、更新、削除など、OOP を使用して顧客情報を管理する方法を示します。
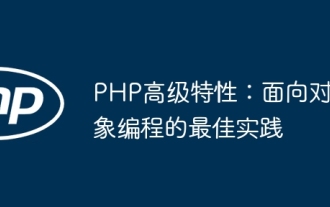
PHP における OOP のベスト プラクティスには、命名規則、インターフェイスと抽象クラス、継承とポリモーフィズム、依存関係の注入が含まれます。実際のケースには、ウェアハウス モードを使用してデータを管理する場合や、ストラテジー モードを使用して並べ替えを実装する場合などがあります。

Go 言語は、オブジェクト指向プログラミング、構造体によるオブジェクトの定義、ポインター レシーバーを使用したメソッドの定義、インターフェイスによるポリモーフィズムの実装をサポートしています。オブジェクト指向の機能は、Go 言語でのコードの再利用、保守性、カプセル化を提供しますが、クラスや継承、メソッド シグネチャ キャストといった従来の概念が欠如しているなどの制限もあります。
