JavaScript学習ノートを整理するためのリファレンスタイプ_JavaScriptスキル
Reference types are very important in JavaScript. A reference type is a data structure used to organize data and functionality together. It describes the properties and methods of a type of object. Object is a basic type, Array is an array type, Date is a date type, RegExp is a regular expression type, etc.
Embrace JavaScript
The once unknown JavaScript has doubled in value with the popularity of AJAX. Now JavaScript is no longer just a dispensable auxiliary tool in WEB development. There is even a position dedicated to it "JavaScript Engineer". Then Even if you are just a WEB backend development programmer, you must know JavaScript. At least in some relevant job requirements, you can see the words "familiarity with JavaScript is preferred". I even want to tell you that you will be able to develop desktop software with JavaScript, thanks to another development model of Adobe AIR, which is to develop AIR with HTML+CSS+JavaScript.
1.Object type
1. Create:
var dog = new Object();
Commonly used to store and transmit data. For example, storage:
var person = new Object(); person.name = "Nicholas"; person.age = 29;
The second way to create: (When creating, the attribute name can also be in string format, that is, you can add quotes to the attribute name.)
var person = { name : "Nicholas", age : 29 };
2. Get the attribute value: person["name"]; or: person.name;
2.Array type
The same array can store any type of data (a hodgepodge).
1. The array can be dynamically adjusted (add one more data, it will grow in length by itself, it is not dead.).
2. Create:
var stars=new Array();//方式1 var stars=new Array(20);//方式2 var stars=new Array("周杰伦","林俊杰","孙燕姿");//方式3 var stars=Array(20);//方式4 var stars=["周杰伦","孙燕姿","林俊杰"];//方式6
3. Dynamic adjustment example:
var stars=["周杰伦","林俊杰","孙燕姿"]; stars[1]="JJ";//动态改变(把林俊杰变为JJ) stars[3]="皮裤汪";//动态增长(加了一个长度) stars.length=1;//动态强制缩减(林俊杰、孙燕姿、皮裤汪强制移除,长度变为1)
4. Detect array: Array.isArray(value);
5. Use join() to convert the array into a delimited string:
var stars = ["周杰伦", "王尼玛", "张全蛋"]; alert(stars .join(",")); //周杰伦,王尼玛,张全蛋 alert(stars .join("-")); //周杰伦-王尼玛-张全蛋
6. You can use arrays like stacks (pop() out, push() in).
7. Arrays can be used like queues. (Combining shift() and push()):
var stars = new Array(); //create an array var count = colors.push("周杰伦", "王尼玛"); //push two items alert(count); //2 count = stars .push("张全蛋"); //push another item on alert(count); //3 var item = colors.shift(); //get the first item alert(item); //周杰伦 alert(colors.length); //2 /**所谓栈变队列,其实就是把栈颠倒过来再拉取*/
8. Sort.
1.reverse()Reverse the order of the array; (return the sorted array)
2.sort()Sort from small to large. But it is sorted by strings, not by numbers: (returns the sorted array).
var values = [, , , , ]; values.sort(); alert(values); //,,,,
To sort the way you expect, you can add a comparison function as a parameter to sort():
function compare(value, value) { if (value < value) { return -; } else if (value > value) { return ; } else { return ; } } var values = [, , , , ]; values.sort(compare); alert(values); //,,,,
Simplified version of the comparison function (sort only cares whether it returns a positive number, a negative number or 0):
function compare(value1,value2){ return value2 - value1; }
9. Operations on arrays: joining, slicing, splicing.
1. Connection : use concat, memory: concat-->concatenate: connection, chain.
Example:
var stars = ["周杰伦", "王尼玛", "张全蛋"]; var stars = stars .concat("太子妃", ["花千骨", "梅长苏"]); alert(stars); //周杰伦,王尼玛,张全蛋 alert(stars); //周杰伦,王尼玛,张全蛋,太子妃,花千骨,梅长苏
2. Slice . Use slice, memory: slice translation: slice. Example:
var stars = ["梅长苏", "誉王", "靖王", "霓凰", "飞流"]; var stars= stars.slice(); var stars= stars.slice(,); alert(stars); //誉王,靖王,霓凰,飞流(从第一个位置开始切) alert(stars); //誉王,靖王,霓凰(从第个位置切到第个位置,表示半封闭,不包含)
3. Splicing. splice. Powerful. Can be deleted, inserted, replaced.
1.Delete any number of items: For example: splice(0,2), delete the 0th and 1st items (semi-closed interval) (return the deleted items).
2.Insert any number of items at the specified position: for example: splice(2,0,"Jay Chou","王尼马"), insert Jay Chou and Wang NIMA starting from the 2nd position Two items.
3.Insert any number of items at the specified position and delete any number of items at the same time. For example: splice(2,1,"Jay Chou","Wang Nima"), delete one item from the second position, and then start inserting two items, Jay Chou and Wang Nima.
10. Position method: indexOf, lastIndexOf;
11. Iterative method: Divided into: passing only if all are qualified, passing if any one is qualified, filtering some residue, one-to-one mapping, iterative query, and reduction.
1. Pass only if all are qualified:
var numbers = [1,2,3,4,5,4,3,2,1]; var everyResult = numbers.every(function(item, index, array){ return (item > 2); }); alert(everyResult); //false
In the above example, true will be returned only if each item is greater than 2.
2. Pass if you pass any one:
var numbers = [1,2,3,4,5,4,3,2,1]; var someResult = numbers.some(function(item, index, array){ return (item > 2); }); alert(someResult); //true
In the above example, if one is greater than 2, it will return true.
3. Filter part of the residue:
var numbers = [1,2,3,4,5,4,3,2,1]; var filterResult = numbers.filter(function(item, index, array){ return (item > 2); }); alert(filterResult); //[3,4,5,4,3]
In the above example, all values greater than 2 are filtered out.
4. One-to-one mapping:
var numbers = [1,2,3,4,5,4,3,2,1]; var mapResult = numbers.map(function(item, index, array){ return item * 2; }); alert(mapResult); //[2,4,6,8,10,8,6,4,2]
In the above example, multiply each term by 2.
5. Iteration: use for-each.
6. Reduce: reduce.
var values = [1,2,3,4,5]; var sum = values.reduce(function(prev, cur, index, array){ return prev + cur; }); alert(sum);//15
累加求和返回,5项缩为1项。
3.RegExp类型
1.var expression=/ pattern / flags;
2.flags分三种:g(global全局模式,应用于所有字符串)、i(case-insensive,忽略字母大小写)、m(multiline,多行模式,一行检验完了接着下一行。)。举例:
/**匹配字符串中所有'at'的实例*/ var pattern1=/at/g; /**匹配第一个'bat'或'cat',不分大小写*/ var pattern2 =/[bc]at/i; /**匹配所有以'at'结尾的3个字符组合,不分大小写*/ var pattern3=/.at/gi;
3.模式中所有的元字符必须转义,元字符:( { [ \ ^ $ | ) ? * + . ] }
4.Function类型
1.每个函数都是Function类型的实例,而且与其他引用类型一样,都有属性和方法。
2.函数的两种定义方法:
方法1:
function sum(a,b){ return a + b; }
方法2:
var sum=function(a,b){ return a + b; }
3.函数没有重载。
5.Boolean、Number、String:基本包装类型
var a="Jay Chou is a superstar"; var b=a.substring(0,8);
上例中,a是基本类型,但是a可以调用substring方法,是因为,后台自动完成a的包装操作,创建String类型的一个实例。Boolean,Number也类似。
6.单体内置对象,不需要实例化,直接使用,如:Math,Global。
1.所有全局作用域中定义的函数、变量,都是Global对象的方法,比如:parseInt,isNaN等。
2.eval()方法也是Global对象的方法,它负责解析javascript。
3.Math对象是保存数学公式和相关信息的。它有很多方法, 如:min求最小值,max求最大值,ceil()向上取整,floor向下取整,round四舍五入,random取随机数。
ps:引用类型理解:变量的交换等于把现有一间店的钥匙(变量引用地址)复制一把给了另外一个老板,此时两个老板同时管理一间店,两个老板的行为都有可能对一间店的运营造成影响。
引用类型例子
function chainStore() { var store1=['Nike China']; var store2=store1; alert(store2[0]); //Nike China store1[0]='Nike U.S.A.'; alert(store2[0]); //Nike U.S.A. } chainStore(); //在上面的代码中,store2只进行了一次赋值,理论上它的值已定,但后面通过改写store1的值,发现store2的值也发生了改变,这正是引用类型的特征,也是我们要注意的地方

ホットAIツール

Undresser.AI Undress
リアルなヌード写真を作成する AI 搭載アプリ

AI Clothes Remover
写真から衣服を削除するオンライン AI ツール。

Undress AI Tool
脱衣画像を無料で

Clothoff.io
AI衣類リムーバー

AI Hentai Generator
AIヘンタイを無料で生成します。

人気の記事

ホットツール

メモ帳++7.3.1
使いやすく無料のコードエディター

SublimeText3 中国語版
中国語版、とても使いやすい

ゼンドスタジオ 13.0.1
強力な PHP 統合開発環境

ドリームウィーバー CS6
ビジュアル Web 開発ツール

SublimeText3 Mac版
神レベルのコード編集ソフト(SublimeText3)

ホットトピック










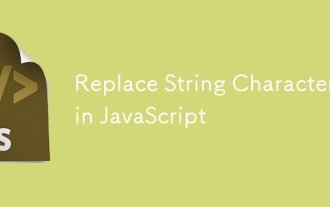
JavaScript文字列置換法とFAQの詳細な説明 この記事では、javaScriptの文字列文字を置き換える2つの方法について説明します:内部JavaScriptコードとWebページの内部HTML。 JavaScriptコード内の文字列を交換します 最も直接的な方法は、置換()メソッドを使用することです。 str = str.replace( "find"、 "置換"); この方法は、最初の一致のみを置き換えます。すべての一致を置き換えるには、正規表現を使用して、グローバルフラグGを追加します。 str = str.replace(/fi
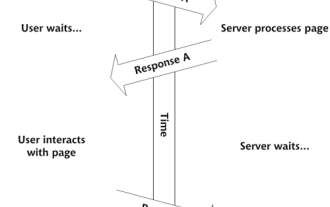
それで、あなたはここで、Ajaxと呼ばれるこのことについてすべてを学ぶ準備ができています。しかし、それは正確には何ですか? Ajaxという用語は、動的でインタラクティブなWebコンテンツを作成するために使用されるテクノロジーのゆるいグループ化を指します。 Ajaxという用語は、もともとJesse Jによって造られました
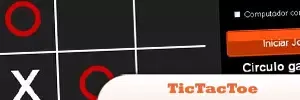
10の楽しいjQueryゲームプラグインして、あなたのウェブサイトをより魅力的にし、ユーザーの粘着性を高めます! Flashは依然としてカジュアルなWebゲームを開発するのに最適なソフトウェアですが、jQueryは驚くべき効果を生み出すこともできます。また、純粋なアクションフラッシュゲームに匹敵するものではありませんが、場合によってはブラウザで予期せぬ楽しみもできます。 jquery tic toeゲーム ゲームプログラミングの「Hello World」には、JQueryバージョンがあります。 ソースコード jQueryクレイジーワードコンポジションゲーム これは空白のゲームであり、単語の文脈を知らないために奇妙な結果を生み出すことができます。 ソースコード jquery鉱山の掃引ゲーム
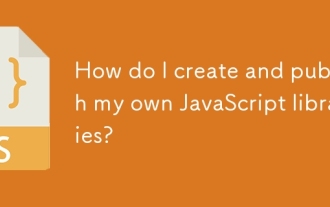
記事では、JavaScriptライブラリの作成、公開、および維持について説明し、計画、開発、テスト、ドキュメント、およびプロモーション戦略に焦点を当てています。
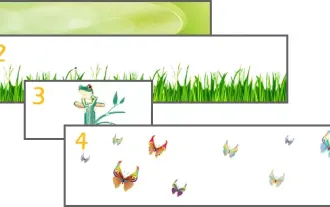
このチュートリアルでは、jQueryを使用して魅惑的な視差の背景効果を作成する方法を示しています。 見事な視覚的な深さを作成するレイヤー画像を備えたヘッダーバナーを構築します。 更新されたプラグインは、jQuery 1.6.4以降で動作します。 ダウンロードしてください
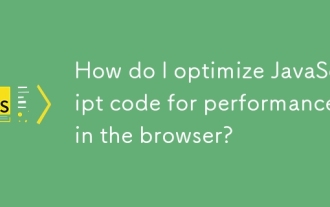
この記事では、ブラウザでJavaScriptのパフォーマンスを最適化するための戦略について説明し、実行時間の短縮、ページの負荷速度への影響を最小限に抑えることに焦点を当てています。
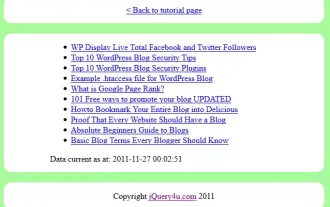
この記事では、JQueryとAjaxを使用して5秒ごとにDivのコンテンツを自動的に更新する方法を示しています。 この例は、RSSフィードからの最新のブログ投稿と、最後の更新タイムスタンプを取得して表示します。 読み込み画像はオプションです
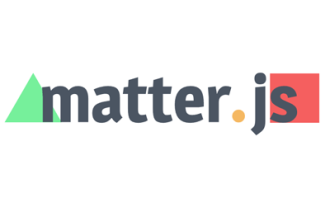
Matter.jsは、JavaScriptで書かれた2D Rigid Body Physics Engineです。このライブラリは、ブラウザで2D物理学を簡単にシミュレートするのに役立ちます。剛体を作成し、質量、面積、密度などの物理的特性を割り当てる機能など、多くの機能を提供します。また、重力摩擦など、さまざまな種類の衝突や力をシミュレートすることもできます。 Matter.jsは、すべての主流ブラウザをサポートしています。さらに、タッチを検出し、応答性が高いため、モバイルデバイスに適しています。これらの機能はすべて、物理ベースの2Dゲームまたはシミュレーションを簡単に作成できるため、エンジンの使用方法を学ぶために時間をかける価値があります。このチュートリアルでは、このライブラリのインストールや使用法を含むこのライブラリの基本を取り上げ、
