C# 2 での GDI+ プログラミングのための 10 の基本スキル
5. グラデーションカラー塗りつぶし
次の 2 つのブラシを使用する必要があります:
LinearGradientBrush(LinearGradientBrush)
PathGuadientBrush(PathGuadientBrush)
private void button4_Click(object sender,System.EventArgs e)
{
/ /描画面Graphics g = this.pictureboxii1.creategraphics();
new Point(0, 10),new Point(150, 10),Color.FromArgb(255, 0, 0),Color.FromArgb(0, 255, 0));ペンペン= new Pen(lgbrush);
//直線セグメントを描画し、線形ブラシ グラデーション効果を備えたペンで四角形を塗りつぶします
g.DrawLine(pen, 10, 130, 500, 130);
g.FillRectangle (lgbrush, 10, 150, 370, 30);
//パスを定義し、楕円を追加しますGraphicsPath gp = new GraphicsPath();
gp.AddEllipse(10, 10, 200, 100) ;
//このパスを使用してパス グラデーション ブラシを定義します
PathGradientBrush Brush =
new PathGradientBrush(gp);
//楕円に追加されたパスで 2 番目のパス グラデーション ブラシを定義します
GraphicsPath gp2 = new GraphicsPath();
gp2.AddEllipse(300, 0, 200, 100);
PathGradientBrush Brush2 = new PathGradientBrush(gp2);
//中心点の位置と色を設定します
brush2 . CenterPoint = new PointF(450, 50);
6、GDI+ 座標系
ユニバーサル座標系 - ユーザー定義の座標系。 ページ座標系 - 仮想座標系。
デバイス座標系 - 画面座標系。
ページ座標系とデバイス座標系の単位が両方ともピクセルの場合、それらは同じです。
private void button10_Click(object sender, System.EventArgse)
{
g.Clear(Color.White);
this.Draw(g);
private void Draw(Graphics g)
{
g.DrawLine(Pens.Black, 10, 10, 100, 100);
g.DrawEllipse(Pens.Black, 50, 50, 200, 100);
g.DrawArc(Pens.Black, 100, 10, 100, 100, 20, 160);
g.DrawRectangle(Pens.Green, 50, 200, 150, 100);
private void button5_Click(object sender, System.EventArgs e)
{
//Shift left
Graphics g = this.pictureBoxII1.CreateGraphics();
g.Clear(Color.White);
g。 TranslateTransform(-50, 0);
private void button6_Click(object sender, System.EventArgs e)
{
//右にシフト
Graphics g = this .pictureBoxII1.CreateGraphics();
g.Clear(Color.White);
g.TranslateTransform(50, 0);
private void button7_Click(オブジェクト送信者, System.EventArgs e)
{
//
グラフィックスを回転 g = this.pictureBoxII1.CreateGraphics();
g.Clear(Color.White);
g.RotateTransform(-30);
private void button8_Click(object sender, System.EventArgs e)
{
//拡大
グラフィックス g = this.pictureBoxII1.CreateGraphics();
g。 Clear(Color.White);
g.ScaleTransform(1.2f, 1.2f);
private void button9_Click(object sender, System.EventArgs e)
{
//Reduce
Graphics g = this.pictureBoxII1.CreateGraphics();
g.Clear(Color.White);
g.ScaleTransform(0.8f, 0.8f);
7. グローバル座標 - 変換は描画面上のすべてのプリミティブに影響を与えます。通常、ユニバーサル座標系を設定するために使用されます。
次のプログラムは、元の固定点をコントロールの中心に移動し、Y 軸が上を向くようにします。
//まず円を描きます
Graphics g = e.Graphics;
g.FillRectangle(Brushes.White, this.ClientRectangle);
g.DrawEllipse(Pens.Black, -100, -100, 200, 200);
//y 軸を前方に向けるには、x 軸に対してミラーリングする必要があります
//変換行列は [1,0,0,-1,0,0 ]
Matrix mat = new Matrix(1, 0, 0, -1, 0, 0);
g.Transform = mat;
Rectangle rect = this.ClientRectangle;
int w = rect.Width;
//原点を中心に半径100の円を作る
g.DrawEllipse( Pens.Red, -100, -100, 200, 200);
g.TranslateTransform(100, 100);g.DrawEllipse(Pens.Green, -100, -100, 200, 200);
g.ScaleTransform(2, 2);
g.DrawEllipse(Pens.Blue, -100, -100, 200, 200) );
8. ローカル座標系 - 特定のグラフィックのみが変換され、他のグラフィック要素は変更されません。
protected override void OnPaint(PaintEventArgs e)
{
Graphics g = e.Graphics;
//クライアント領域は白に設定されます
g.FillRectangle(Brushes.White, this.ClientRectangle);
/ /Y軸が上を向いています
Matrix mat = new Matrix(1, 0, 0, -1, 0, 0);
g.Transform = mat;
//座標原点を中心に移動しますフォーム
Rectangle rect = this.ClientRectangle;
int w = rect.Width;
int h = rect.Height;
g.TranslateTransform(w/2, -h/2);
//Drawグローバル座標の楕円
g.DrawEllipse(Pens.Red, -100, -100, 200, 200);
g.FillRectangle(Brushes.Black, -108, 0, 8, 8);
g。 FillRectangle(Brushes.Black, 100, 0, 8, 8);
g.FillRectangle(Brushes.Black, 0, 100, 8, 8);
g.FillRectangle(Brushes.Black, 0, -108, 8 , 8);
//楕円を作成し、ローカル座標系で変換します
GraphicsPath gp = new GraphicsPath();
gp.AddEllipse(-100, -100, 200, 200);
Matrix mat2 = new Matrix() ;
//Translation
mat2.Translate(150, 150);
//Rotation
mat2.Rotate(30);
gp.Transform(mat2);
g。 DrawPath(Pens.Blue, gp);
PointF[] p = gp.PathPoints;
g.FillRectangle(Brushes.Black, p[0].X-2, p[0].Y+2, 4 , 4);
g.FillRectangle(Brushes.Black, p[3].X-2, p[3].Y+2, 4, 4);
g.FillRectangle(Brushes.Black, p[6 ].X-4, p[6].Y-4, 4, 4);
g.FillRectangle(Brushes.Black, p[9].X-4, p[9].Y-4, 4, 4);
gp.Dispose();
//base.OnPaint (e);
}
9. アルファブレンディング
Color.FromArgb() の A は Alpha です。アルファ値の範囲は 0 ~ 255 です。0 は完全に透明を意味し、255 は完全に不透明を意味します。
現在の色 = 前景色×アルファ/255+背景色×(255-アルファ)/255
protected override void OnPaint(PaintEventArgs e)
{
Graphics g = e.Graphics;
//塗りつぶされた長方形を作成します
SolidBrush Brush = new SolidBrush(Color.BlueViolet);
g.FillRectangle(brush, 180, 70, 200, 150);
// 2 つのビットマップの間にビットマップを作成します 透明効果があります
ビットマップbm1 = new Bitmap(200, 100);
Graphics bg1 = Graphics.FromImage(bm1);
SolidBrush redBrush =
new SolidBrush(Color.FromArgb(210, 255, 0, 0) );
SolidBrush greenBrush =
new SolidBrush(Color.FromArgb(210, 0, 255, 0));
bg1.FillRectangle(redBrush, 0, 0, 150, 70);
bg1.FillRectangle(greenBrush , 30, 30, 150 , 70);
g.DrawImage(bm1, 100, 100);
//2 つのビットマップ間に透明効果のないビットマップを作成します
Bitmap bm2 = new Bitmap( 200, 100); aGraphics BG2 = Graphics。 fromimage (BM2); gBg2.fillrectangle ( greenBrush, 30, 30, 150, 70);
g.CompositingQuality = CompositingQuality.GammaCorrected;
g.DrawImage(bm2, 300, 200);
/ /base.OnPaint (e);}10. アンチエイリアス
g.ScaleTransform(8, 8);
// グラフィックスとテキストのアンチエイリアスなし
Draw(g);
// アンチエイリアスを設定するg.SmoothingMode = SmoothingMode.AntiAlias;//右に40シフト
g.TranslateTransform(40, 0) ;
//base.OnPaint (e);
private void Draw(Graphics g)
{
//グラフィックとテキストを描画
g.DrawEllipse(Pens.Gray, 20, 20, 30, 10);
string s = "アンチエイリアシング テスト" ;
Font font = new Font("宋体", 5);
SolidBrush ブラシ = new SolidBrush(Color.Gray);
g。 DrawString(s, font, Brush, 10, 40);}
C# 2 での GDI+ プログラミングの 10 の基本スキルについては、関連記事については、PHP 中国語 Web サイトに注目してください。

ホットAIツール

Undresser.AI Undress
リアルなヌード写真を作成する AI 搭載アプリ

AI Clothes Remover
写真から衣服を削除するオンライン AI ツール。

Undress AI Tool
脱衣画像を無料で

Clothoff.io
AI衣類リムーバー

AI Hentai Generator
AIヘンタイを無料で生成します。

人気の記事

ホットツール

メモ帳++7.3.1
使いやすく無料のコードエディター

SublimeText3 中国語版
中国語版、とても使いやすい

ゼンドスタジオ 13.0.1
強力な PHP 統合開発環境

ドリームウィーバー CS6
ビジュアル Web 開発ツール

SublimeText3 Mac版
神レベルのコード編集ソフト(SublimeText3)

ホットトピック








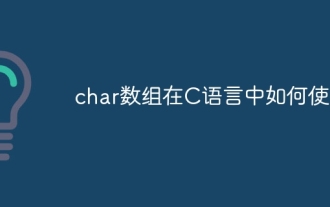
Char Arrayは文字シーケンスをC言語で保存し、char array_name [size]として宣言されます。アクセス要素はサブスクリプト演算子に渡され、要素は文字列のエンドポイントを表すnullターミネーター「\ 0」で終了します。 C言語は、strlen()、strcpy()、strcat()、strcmp()など、さまざまな文字列操作関数を提供します。
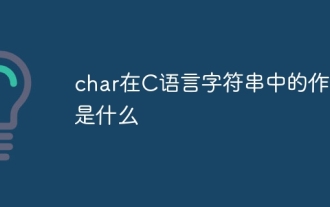
Cでは、文字列でCharタイプが使用されます。1。単一の文字を保存します。 2。配列を使用して文字列を表し、ヌルターミネーターで終了します。 3。文字列操作関数を介して動作します。 4.キーボードから文字列を読み取りまたは出力します。
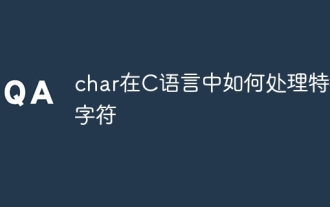
C言語では、以下などのエスケープシーケンスを通じて特殊文字が処理されます。\ nはラインブレークを表します。 \ tはタブ文字を意味します。 ESACEシーケンスまたは文字定数を使用して、Char C = '\ n'などの特殊文字を表します。バックスラッシュは2回逃げる必要があることに注意してください。さまざまなプラットフォームとコンパイラが異なるエスケープシーケンスを持っている場合があります。ドキュメントを参照してください。
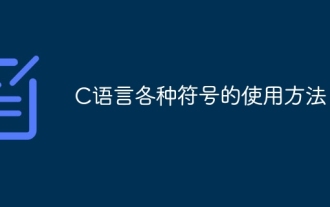
c言語のシンボルの使用方法は、算術、割り当て、条件、ロジック、ビット演算子などをカバーします。算術演算子は基本的な数学的操作に使用されます。割り当てと追加、下位、乗算、除算の割り当てには、条件操作に使用されます。ポインター、ファイル終了マーカー、および非数値値。
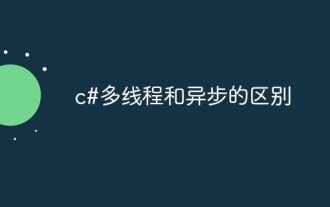
マルチスレッドと非同期の違いは、マルチスレッドが複数のスレッドを同時に実行し、現在のスレッドをブロックせずに非同期に操作を実行することです。マルチスレッドは計算集約型タスクに使用されますが、非同期はユーザーインタラクションに使用されます。マルチスレッドの利点は、コンピューティングのパフォーマンスを改善することですが、非同期の利点はUIスレッドをブロックしないことです。マルチスレッドまたは非同期を選択することは、タスクの性質に依存します。計算集約型タスクマルチスレッド、外部リソースと相互作用し、UIの応答性を非同期に使用する必要があるタスクを使用します。
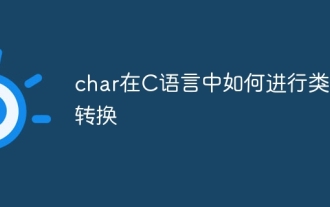
C言語では、charタイプの変換は、キャスト:キャスト文字を使用することにより、別のタイプに直接変換できます。自動タイプ変換:あるタイプのデータが別のタイプの値に対応できる場合、コンパイラは自動的に変換します。
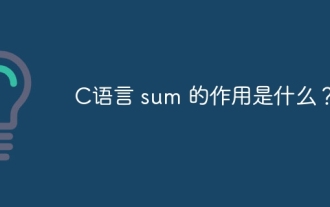
C言語に組み込みの合計機能はないため、自分で書く必要があります。合計は、配列を通過して要素を蓄積することで達成できます。ループバージョン:合計は、ループとアレイの長さを使用して計算されます。ポインターバージョン:ポインターを使用してアレイ要素を指し示し、効率的な合計が自己概要ポインターを通じて達成されます。アレイバージョンを動的に割り当てます:[アレイ]を動的に割り当ててメモリを自分で管理し、メモリの漏れを防ぐために割り当てられたメモリが解放されます。
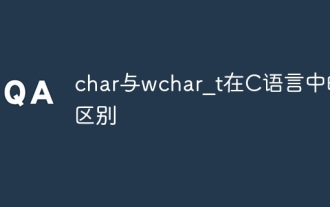
C言語では、charとwchar_tの主な違いは文字エンコードです。CharはASCIIを使用するか、ASCIIを拡張し、WCHAR_TはUnicodeを使用します。 Charは1〜2バイトを占め、WCHAR_Tは2〜4バイトを占有します。 charは英語のテキストに適しており、wchar_tは多言語テキストに適しています。 CHARは広くサポートされており、WCHAR_TはコンパイラとオペレーティングシステムがUnicodeをサポートするかどうかに依存します。 CHARの文字範囲は限られており、WCHAR_Tの文字範囲が大きく、特別な機能が算術演算に使用されます。
