C# シャロー コピーとディープ コピーの例の分析
場合によっては、データベースからデータを読み取ってオブジェクトを埋めたり、ハードディスクからファイルを読み取ってオブジェクトを埋めたりする必要がありますが、これには比較的時間がかかります。今回はオブジェクトをコピーすることを考えます。この記事では、C# のシャロー コピーとディープ コピーの使用法を例の形で分析します。詳細は以下のとおりです:
1. 浅いコピー
1. 「浅いコピー」とは:
オブジェクトをコピーするとき、オブジェクトの値型メンバーはそれ自身をコピーし、オブジェクトの参照型メンバーの場合はオブジェクトのみをコピーします。マネージド ヒープ上のオブジェクト インスタンスを指す参照がコピーされます。
2. 参照型のクラスメンバーと値型の構造体メンバーを含むオブジェクトがあります。
Cinema には参照型メンバー Room と値型メンバー Film が含まれます。
public class Room { public int _maxSeat; public Room(int maxSeat) { this._maxSeat = maxSeat; } } public struct Film { public string _name; public Film(string name) { this._name = name; } } public class Cinema { public Room _room; public Film _film; public Cinema(Room room, Film film) { this._room = room; this._film = film; } public object Clone() { return MemberwiseClone(); //对引用类型实施浅复制 } }
3. コピー後の効果をテストします
①コピー前に元のオブジェクトの値型と参照型のメンバーの値を出力します
②元のオブジェクトをコピーし、値型の値を出力しますコピーしたオブジェクトの値型と参照型のメンバーを再度出力します
③ 元のオブジェクトの値を変更し、元のオブジェクトの値型と参照型のメンバーを再度出力します
④ コピーしたオブジェクトの値型と参照型のメンバーを再度出力します
static void Main(string[] args) { Room room1 = new Room(60); Film film1 = new Film("家园防线"); Cinema cinema1 = new Cinema(room1, film1); Cinema cinema2 = (Cinema)cinema1.Clone(); Console.WriteLine("拷贝之前,结构成员的字段值为{0},引用类型成员的字段值为{1}", cinema1._film._name,cinema1._room._maxSeat); Console.WriteLine("拷贝之后,新的结构成员的字段值为{0},引用类型成员的字段值为{1}", cinema2._film._name, cinema2._room._maxSeat); //修改拷贝之前引用类型的字段值 cinema1._film._name = "极品飞车"; cinema1._room._maxSeat = 80; Console.WriteLine("修改之后,结构成员的字段值为{0},引用类型成员的字段值为{1}", cinema1._film._name, cinema1._room._maxSeat); Console.WriteLine("修改之后,新的结构成员的字段值为{0},引用类型成员的字段值为{1}", cinema2._film._name, cinema2._room._maxSeat); Console.ReadKey(); }
分析:
浅いコピーの重要なポイントは参照型です。コピーされるのはオブジェクト参照であり、マネージド ヒープ上のオブジェクト インスタンスを指します。元の対応する参照型の値を変更すると、コピーされたオブジェクトに影響します。
2.ディープコピー
1.「ディープコピー」とは
参照メンバーが指すオブジェクトをコピーし、元のオブジェクトインスタンスに含まれるデータをマネージドヒープ上に割り当てます。マネージド ヒープの例。
2. 各オブジェクトのメンバーをコピーすることによるディープコピー
public object Clone() { Room room = new Room(); room._maxSeat = this._room._maxSeat;//复制当前引用类型成员的值到新对象 Film film = this._film; //值类型直接赋值 Cinema cinema = new Cinema(room, film); return cinema; }
3. シリアル化とデシリアライズによってディープコピーを行うこともできます
public object Clone1() { BinaryFormatter bf = new BinaryFormatter(); MemoryStream ms = new MemoryStream(); bf.Serialize(ms, this); //复制到流中 ms.Position = 0; return (bf.Deserialize(ms)); }
[Serializable] public class Room { public int _maxSeat; public Room() {} public Room(int maxSeat) { this._maxSeat = maxSeat; } } [Serializable] public struct Film { public string _name; public Film(string name) { this._name = name; } } [Serializable] public class Cinema { public Room _room; public Film _film; public Cinema(Room room, Film film) { this._room = room; this._film = film; } //浅拷贝 //public object Clone() //{ // return MemberwiseClone(); //对引用类型实施浅复制 //} //深拷贝 对每个对象成员进行复制 public object Clone() { Room room = new Room(); room._maxSeat = this._room._maxSeat;//复制当前引用类型成员的值到新对象 Film film = this._film; //值类型直接赋值 Cinema cinema = new Cinema(room, film); return cinema; } //使用序列化和反序列化进行复制 public object Clone1() { BinaryFormatter bf = new BinaryFormatter(); MemoryStream ms = new MemoryStream(); bf.Serialize(ms, this); //复制到流中 ms.Position = 0; return (bf.Deserialize(ms)); } }
②元のオブジェクトにコピーしてコピーしたオブジェクトの値型と参照型のメンバーの値を出力します
③ 元のオブジェクトの値を変更し、再度元のオブジェクトの値型と参照型のメンバーの値を出力します
④コピーされたオブジェクトの値型と参照型のメンバーの値を再度
static void Main(string[] args) { Room room1 = new Room(60); Film film1 = new Film("家园防线"); Cinema cinema1 = new Cinema(room1, film1); Cinema cinema2 = (Cinema)cinema1.Clone1(); Console.WriteLine("拷贝之前,结构成员的字段值为{0},引用类型成员的字段值为{1}", cinema1._film._name,cinema1._room._maxSeat); Console.WriteLine("拷贝之后,新的结构成员的字段值为{0},引用类型成员的字段值为{1}", cinema2._film._name, cinema2._room._maxSeat); //修改拷贝之前引用类型的字段值 cinema1._film._name = "极品飞车"; cinema1._room._maxSeat = 80; Console.WriteLine("修改之后,结构成员的字段值为{0},引用类型成员的字段值为{1}", cinema1._film._name, cinema1._room._maxSeat); Console.WriteLine("修改之后,新的结构成员的字段值为{0},引用类型成员的字段值为{1}", cinema2._film._name, cinema2._room._maxSeat); Console.ReadKey(); }

ホットAIツール

Undresser.AI Undress
リアルなヌード写真を作成する AI 搭載アプリ

AI Clothes Remover
写真から衣服を削除するオンライン AI ツール。

Undress AI Tool
脱衣画像を無料で

Clothoff.io
AI衣類リムーバー

AI Hentai Generator
AIヘンタイを無料で生成します。

人気の記事

ホットツール

メモ帳++7.3.1
使いやすく無料のコードエディター

SublimeText3 中国語版
中国語版、とても使いやすい

ゼンドスタジオ 13.0.1
強力な PHP 統合開発環境

ドリームウィーバー CS6
ビジュアル Web 開発ツール

SublimeText3 Mac版
神レベルのコード編集ソフト(SublimeText3)

ホットトピック








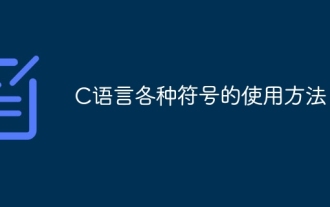
c言語のシンボルの使用方法は、算術、割り当て、条件、ロジック、ビット演算子などをカバーします。算術演算子は基本的な数学的操作に使用されます。割り当てと追加、下位、乗算、除算の割り当てには、条件操作に使用されます。ポインター、ファイル終了マーカー、および非数値値。
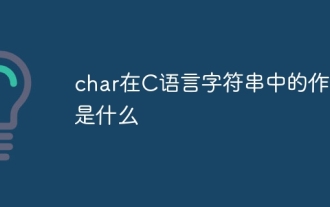
Cでは、文字列でCharタイプが使用されます。1。単一の文字を保存します。 2。配列を使用して文字列を表し、ヌルターミネーターで終了します。 3。文字列操作関数を介して動作します。 4.キーボードから文字列を読み取りまたは出力します。
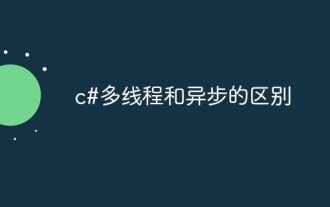
マルチスレッドと非同期の違いは、マルチスレッドが複数のスレッドを同時に実行し、現在のスレッドをブロックせずに非同期に操作を実行することです。マルチスレッドは計算集約型タスクに使用されますが、非同期はユーザーインタラクションに使用されます。マルチスレッドの利点は、コンピューティングのパフォーマンスを改善することですが、非同期の利点はUIスレッドをブロックしないことです。マルチスレッドまたは非同期を選択することは、タスクの性質に依存します。計算集約型タスクマルチスレッド、外部リソースと相互作用し、UIの応答性を非同期に使用する必要があるタスクを使用します。
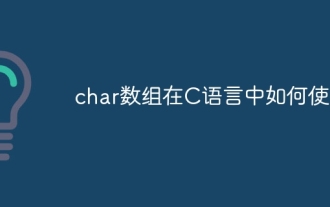
Char Arrayは文字シーケンスをC言語で保存し、char array_name [size]として宣言されます。アクセス要素はサブスクリプト演算子に渡され、要素は文字列のエンドポイントを表すnullターミネーター「\ 0」で終了します。 C言語は、strlen()、strcpy()、strcat()、strcmp()など、さまざまな文字列操作関数を提供します。
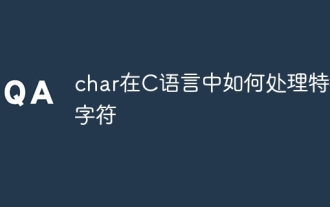
C言語では、以下などのエスケープシーケンスを通じて特殊文字が処理されます。\ nはラインブレークを表します。 \ tはタブ文字を意味します。 ESACEシーケンスまたは文字定数を使用して、Char C = '\ n'などの特殊文字を表します。バックスラッシュは2回逃げる必要があることに注意してください。さまざまなプラットフォームとコンパイラが異なるエスケープシーケンスを持っている場合があります。ドキュメントを参照してください。
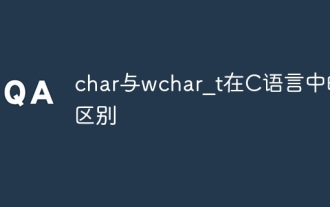
C言語では、charとwchar_tの主な違いは文字エンコードです。CharはASCIIを使用するか、ASCIIを拡張し、WCHAR_TはUnicodeを使用します。 Charは1〜2バイトを占め、WCHAR_Tは2〜4バイトを占有します。 charは英語のテキストに適しており、wchar_tは多言語テキストに適しています。 CHARは広くサポートされており、WCHAR_TはコンパイラとオペレーティングシステムがUnicodeをサポートするかどうかに依存します。 CHARの文字範囲は限られており、WCHAR_Tの文字範囲が大きく、特別な機能が算術演算に使用されます。
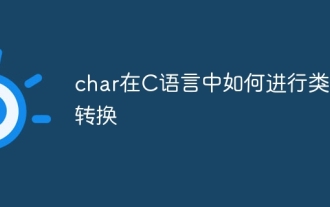
C言語では、charタイプの変換は、キャスト:キャスト文字を使用することにより、別のタイプに直接変換できます。自動タイプ変換:あるタイプのデータが別のタイプの値に対応できる場合、コンパイラは自動的に変換します。
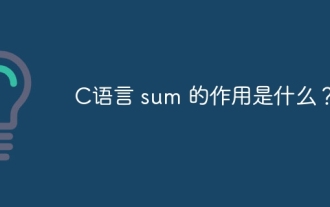
C言語に組み込みの合計機能はないため、自分で書く必要があります。合計は、配列を通過して要素を蓄積することで達成できます。ループバージョン:合計は、ループとアレイの長さを使用して計算されます。ポインターバージョン:ポインターを使用してアレイ要素を指し示し、効率的な合計が自己概要ポインターを通じて達成されます。アレイバージョンを動的に割り当てます:[アレイ]を動的に割り当ててメモリを自分で管理し、メモリの漏れを防ぐために割り当てられたメモリが解放されます。
