python3+PyQt5はヒストグラムを実装します
この記事では主に python3+PyQt5 でヒストグラムを実装する方法を詳しく紹介します。興味のある方は参考にしてください。この記事は Python3+pyqt5 の例を抜粋して Python Qt GUI を実装します。
#!/usr/bin/env python3 import random import sys from PyQt5.QtCore import (QAbstractListModel, QAbstractTableModel, QModelIndex, QSize, QTimer, QVariant, Qt,pyqtSignal) from PyQt5.QtWidgets import (QApplication, QDialog, QHBoxLayout, QListView, QSpinBox, QStyledItemDelegate,QStyleOptionViewItem, QWidget) from PyQt5.QtGui import QColor,QPainter,QPixmap class BarGraphModel(QAbstractListModel): dataChanged=pyqtSignal(QModelIndex,QModelIndex) def __init__(self): super(BarGraphModel, self).__init__() self.__data = [] self.__colors = {} self.minValue = 0 self.maxValue = 0 def rowCount(self, index=QModelIndex()): return len(self.__data) def insertRows(self, row, count): extra = row + count if extra >= len(self.__data): self.beginInsertRows(QModelIndex(), row, row + count - 1) self.__data.extend([0] * (extra - len(self.__data) + 1)) self.endInsertRows() return True return False def flags(self, index): #return (QAbstractTableModel.flags(self, index)|Qt.ItemIsEditable) return (QAbstractListModel.flags(self, index)|Qt.ItemIsEditable) def setData(self, index, value, role=Qt.DisplayRole): row = index.row() if not index.isValid() or 0 > row >= len(self.__data): return False changed = False if role == Qt.DisplayRole: value = value self.__data[row] = value if self.minValue > value: self.minValue = value if self.maxValue < value: self.maxValue = value changed = True elif role == Qt.UserRole: self.__colors[row] = value #self.emit(SIGNAL("dataChanged(QModelIndex,QModelIndex)"), # index, index) self.dataChanged[QModelIndex,QModelIndex].emit(index, index) changed = True if changed: #self.emit(SIGNAL("dataChanged(QModelIndex,QModelIndex)"), # index, index) self.dataChanged[QModelIndex,QModelIndex].emit(index, index) return changed def data(self, index, role=Qt.DisplayRole): row = index.row() if not index.isValid() or 0 > row >= len(self.__data): return QVariant() if role == Qt.DisplayRole: return self.__data[row] if role == Qt.UserRole: return QVariant(self.__colors.get(row, QColor(Qt.red))) if role == Qt.DecorationRole: color = QColor(self.__colors.get(row, QColor(Qt.red))) pixmap = QPixmap(20, 20) pixmap.fill(color) return QVariant(pixmap) return QVariant() class BarGraphDelegate(QStyledItemDelegate): def __init__(self, minimum=0, maximum=100, parent=None): super(BarGraphDelegate, self).__init__(parent) self.minimum = minimum self.maximum = maximum def paint(self, painter, option, index): myoption = QStyleOptionViewItem(option) myoption.displayAlignment |= (Qt.AlignRight|Qt.AlignVCenter) QStyledItemDelegate.paint(self, painter, myoption, index) def createEditor(self, parent, option, index): spinbox = QSpinBox(parent) spinbox.setRange(self.minimum, self.maximum) spinbox.setAlignment(Qt.AlignRight|Qt.AlignVCenter) return spinbox def setEditorData(self, editor, index): value = index.model().data(index, Qt.DisplayRole) editor.setValue(value) def setModelData(self, editor, model, index): editor.interpretText() model.setData(index, editor.value()) class BarGraphView(QWidget): WIDTH = 20 def __init__(self, parent=None): super(BarGraphView, self).__init__(parent) self.model = None def setModel(self, model): self.model = model #self.connect(self.model, # SIGNAL("dataChanged(QModelIndex,QModelIndex)"), # self.update) self.model.dataChanged[QModelIndex,QModelIndex].connect(self.update) #self.connect(self.model, SIGNAL("modelReset()"), self.update) self.model.modelReset.connect(self.update) def sizeHint(self): return self.minimumSizeHint() def minimumSizeHint(self): if self.model is None: return QSize(BarGraphView.WIDTH * 10, 100) return QSize(BarGraphView.WIDTH * self.model.rowCount(), 100) def paintEvent(self, event): if self.model is None: return painter = QPainter(self) painter.setRenderHint(QPainter.Antialiasing) span = self.model.maxValue - self.model.minValue painter.setWindow(0, 0, BarGraphView.WIDTH * self.model.rowCount(), span) for row in range(self.model.rowCount()): x = row * BarGraphView.WIDTH index = self.model.index(row) color = QColor(self.model.data(index, Qt.UserRole)) y = self.model.data(index) painter.fillRect(x, span - y, BarGraphView.WIDTH, y, color) class MainForm(QDialog): def __init__(self, parent=None): super(MainForm, self).__init__(parent) self.model = BarGraphModel() self.barGraphView = BarGraphView() self.barGraphView.setModel(self.model) self.listView = QListView() self.listView.setModel(self.model) self.listView.setItemDelegate(BarGraphDelegate(0, 1000, self)) self.listView.setMaximumWidth(100) self.listView.setEditTriggers(QListView.DoubleClicked| QListView.EditKeyPressed) layout = QHBoxLayout() layout.addWidget(self.listView) layout.addWidget(self.barGraphView, 1) self.setLayout(layout) self.setWindowTitle("Bar Grapher") QTimer.singleShot(0, self.initialLoad) def initialLoad(self): # Generate fake data count = 20 self.model.insertRows(0, count - 1) for row in range(count): value = random.randint(1, 150) color = QColor(random.randint(0, 255), random.randint(0, 255), random.randint(0, 255)) index = self.model.index(row) self.model.setData(index, value) self.model.setData(index, QVariant(color), Qt.UserRole) app = QApplication(sys.argv) form = MainForm() form.resize(600, 400) form.show() app.exec_()
実行結果:
関連する推奨事項:
以上がpython3+PyQt5はヒストグラムを実装しますの詳細内容です。詳細については、PHP 中国語 Web サイトの他の関連記事を参照してください。

ホットAIツール

Undresser.AI Undress
リアルなヌード写真を作成する AI 搭載アプリ

AI Clothes Remover
写真から衣服を削除するオンライン AI ツール。

Undress AI Tool
脱衣画像を無料で

Clothoff.io
AI衣類リムーバー

AI Hentai Generator
AIヘンタイを無料で生成します。

人気の記事

ホットツール

メモ帳++7.3.1
使いやすく無料のコードエディター

SublimeText3 中国語版
中国語版、とても使いやすい

ゼンドスタジオ 13.0.1
強力な PHP 統合開発環境

ドリームウィーバー CS6
ビジュアル Web 開発ツール

SublimeText3 Mac版
神レベルのコード編集ソフト(SublimeText3)

ホットトピック








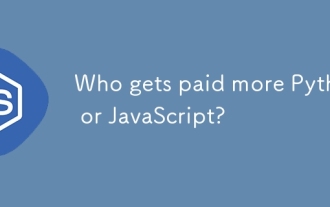
スキルや業界のニーズに応じて、PythonおよびJavaScript開発者には絶対的な給与はありません。 1. Pythonは、データサイエンスと機械学習でさらに支払われる場合があります。 2。JavaScriptは、フロントエンドとフルスタックの開発に大きな需要があり、その給与もかなりです。 3。影響要因には、経験、地理的位置、会社の規模、特定のスキルが含まれます。
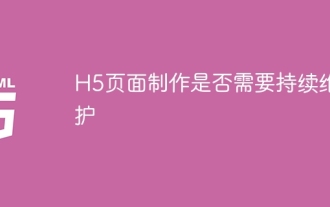
H5ページは、コードの脆弱性、ブラウザー互換性、パフォーマンスの最適化、セキュリティの更新、ユーザーエクスペリエンスの改善などの要因のため、継続的に維持する必要があります。効果的なメンテナンス方法には、完全なテストシステムの確立、バージョン制御ツールの使用、定期的にページのパフォーマンスの監視、ユーザーフィードバックの収集、メンテナンス計画の策定が含まれます。
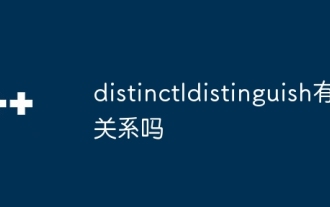
明確で明確なものは区別に関連していますが、それらは異なる方法で使用されます。明確な(形容詞)は、物事自体の独自性を説明し、物事の違いを強調するために使用されます。明確な(動詞)は、区別の動作または能力を表し、差別プロセスを説明するために使用されます。プログラミングでは、個別は、重複排除操作などのコレクション内の要素の独自性を表すためによく使用されます。明確なは、奇数や偶数の偶数を区別するなど、アルゴリズムまたは関数の設計に反映されます。最適化する場合、異なる操作は適切なアルゴリズムとデータ構造を選択する必要がありますが、異なる操作は、論理効率の区別を最適化し、明確で読み取り可能なコードの書き込みに注意を払う必要があります。
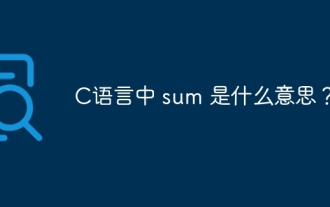
Cには組み込みの合計関数はありませんが、次のように実装できます。ループを使用して要素を1つずつ蓄積します。ポインターを使用して、要素に1つずつアクセスして蓄積します。大量のデータ量については、並列計算を検討してください。
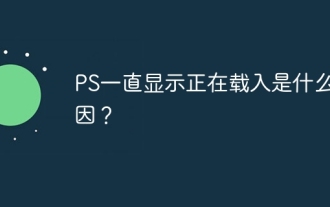
PSの「読み込み」の問題は、リソースアクセスまたは処理の問題によって引き起こされます。ハードディスクの読み取り速度は遅いか悪いです。CrystaldiskInfoを使用して、ハードディスクの健康を確認し、問題のあるハードディスクを置き換えます。不十分なメモリ:高解像度の画像と複雑な層処理に対するPSのニーズを満たすためのメモリをアップグレードします。グラフィックカードドライバーは時代遅れまたは破損しています:ドライバーを更新して、PSとグラフィックスカードの間の通信を最適化します。ファイルパスが長すぎるか、ファイル名に特殊文字があります。短いパスを使用して特殊文字を避けます。 PS独自の問題:PSインストーラーを再インストールまたは修理します。
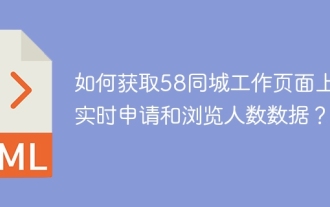
クロール中に58.com作業ページの動的データを取得するにはどうすればよいですか? Crawlerツールを使用して58.comの作業ページをrawったら、これに遭遇する可能性があります...
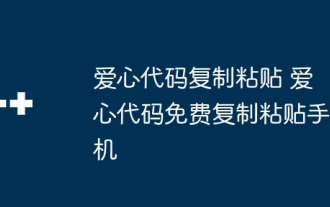
コードのコピーと貼り付けは不可能ではありませんが、注意して扱う必要があります。コード内の環境、ライブラリ、バージョンなどの依存関係は、現在のプロジェクトと一致しないため、エラーや予測不可能な結果が得られます。ファイルパス、従属ライブラリ、Pythonバージョンなど、コンテキストが一貫していることを確認してください。さらに、特定のライブラリのコードをコピーして貼り付けるときは、ライブラリとその依存関係をインストールする必要がある場合があります。一般的なエラーには、パスエラー、バージョンの競合、一貫性のないコードスタイルが含まれます。パフォーマンスの最適化は、コードの元の目的と制約に従って再設計またはリファクタリングする必要があります。コピーされたコードを理解してデバッグすることが重要であり、盲目的にコピーして貼り付けないでください。
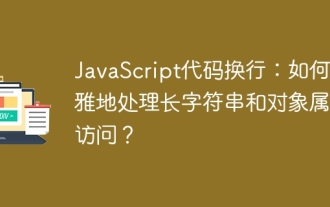
JavaScriptコードの詳細な説明JavaScriptコードを書くとき、私たちはしばしば長すぎるコードの行に遭遇します。
