JavaScriptのエラーオブジェクト
JavaScript で実行時エラーが発生すると、Error
オブジェクトが発生します。多くの場合、これらの標準 Error オブジェクトを拡張して、独自のカスタム Error
オブジェクトを作成することもできます。 Properties
オブジェクトには 2 つのプロパティがあります
- エラー名を設定または返します。具体的には、エラーが属するコンストラクターの名前を返します。 これには 6 つの異なる値があります -
EvalError、RangeError
、ReferenceError
、TypeError
、SyntaxError
、URIエラー
。この記事の後半で説明するように、すべてのエラー タイプは Object-> Error-> RangeError
を継承します。
-エラー メッセージを設定または返します
例
Error
オブジェクトを使用して新しい Error
を作成し、throw
キーワードを使用して明示的にエラーをスローできます。
try{
throw new Error('Some Error Occurred!')
}
catch(e){
console.error('Error Occurred. ' + e.name + ': ' + e.message)
}
次の
instanceof を使用することもできます。特定のエラー タイプを処理するためのキーワード。
try{
someFunction()
}
catch(e){
if(e instanceof EvalError) {
console.error(e.name + ': ' + e.message)
}
else if(e instanceof RangeError) {
console.error(e.name + ': ' + e.message)
}
// ... something else
}
を継承するクラスを作成して、独自のエラー タイプを定義することもできます。オブジェクトエラータイプ。
#
class CustomError extends Error { constructor(description, ...params) { super(...params) if(Error.captureStackTrace){ Error.captureStackTrace(this, CustomError) } this.name = 'CustomError_MyError' this.description = description this.date = new Date() } } try{ throw new CustomError('Custom Error', 'Some Error Occurred') } catch(e){ console.error(e.name) //CustomError_MyError console.error(e.description) //Custom Error console.error(e.message) //Some Error Occurred console.error(e.stack) //stacktrace }
エラーのオブジェクト タイプ
eval()
に関連するエラーの原因を示すerror インスタンスを作成します。
ここで注意すべき点は、現在の ECMAScript 仕様ではサポートされておらず、ランタイムによってスローされないことです。代わりに、
SyntaxError
#文法
new EvalError([message[, fileName[, lineNumber]]])
例
##
try{ throw new EvalError('Eval Error Occurred'); } catch(e){ console.log(e instanceof EvalError); // true console.log(e.message); // "Eval Error Occurred" console.log(e.name); // "EvalError" console.log(e.stack); // "EvalError: Eval Error Occurred..." }
ブラウザの互換性
2. RangeError
error
new RangeError([message[, fileName[, lineNumber]]])
下面的情况会触发该错误:
1)根据String.prototype.normalize()
,我们传递了一个不允许的字符串值。
// Uncaught RangeError: The normalization form should be one of NFC, NFD, NFKC, NFKD String.prototype.normalize(“-1”)
2)使用Array
构造函数创建非法长度的数组
// RangeError: Invalid array length var arr = new Array(-1);
3)诸如 Number.prototype.toExponential()
,Number.prototype.toFixed()
或Number.prototype.toPrecision()
之类的数字方法会接收无效值。
// Uncaught RangeError: toExponential() argument must be between 0 and 100 Number.prototype.toExponential(101) // Uncaught RangeError: toFixed() digits argument must be between 0 and 100 Number.prototype.toFixed(-1) // Uncaught RangeError: toPrecision() argument must be between 1 and 100 Number.prototype.toPrecision(101)
事例
对于数值
function checkRange(n) { if( !(n >= 0 && n <= 100) ) { throw new RangeError("The argument must be between 0 and 100."); } }; try { checkRange(101); } catch(error) { if (error instanceof RangeError) { console.log(error.name); console.log(error.message); } }
对于非数值
function checkJusticeLeaque(value) { if(["batman", "superman", "flash"].includes(value) === false) { throw new RangeError('The hero must be in Justice Leaque...'); } } try { checkJusticeLeaque("wolverine"); } catch(error) { if(error instanceof RangeError) { console.log(error.name); console.log(error.message); } }
浏览器兼容性
3. ReferenceError
创建一个error
实例,表示错误的原因:无效引用。
new ReferenceError([message[, fileName[, lineNumber]]])
事例
ReferenceError
被自动触发。
try { callJusticeLeaque(); } catch(e){ console.log(e instanceof ReferenceError) // true console.log(e.message) // callJusticeLeaque is not defined console.log(e.name) // "ReferenceError" console.log(e.stack) // ReferenceError: callJusticeLeaque is not defined.. } or as simple as a/10;
显式抛出ReferenceError
try { throw new ReferenceError('Reference Error Occurred') } catch(e){ console.log(e instanceof ReferenceError) // true console.log(e.message) // Reference Error Occurred console.log(e.name) // "ReferenceError" console.log(e.stack) // ReferenceError: Reference Error Occurred. }
浏览器兼容性
4. SyntaxError
创建一个error实例,表示错误的原因:eval()在解析代码的过程中发生的语法错误。
换句话说,当 JS 引擎在解析代码时遇到不符合语言语法的令牌或令牌顺序时,将抛出SyntaxError
。
捕获语法错误
try { eval('Justice Leaque'); } catch(e){ console.error(e instanceof SyntaxError); // true console.error(e.message); // Unexpected identifier console.error(e.name); // SyntaxError console.error(e.stack); // SyntaxError: Unexpected identifier } let a = 100/; // Uncaught SyntaxError: Unexpected token ';' // Uncaught SyntaxError: Unexpected token ] in JSON JSON.parse('[1, 2, 3, 4,]'); // Uncaught SyntaxError: Unexpected token } in JSON JSON.parse('{"aa": 11,}');
创建一个SyntaxError
try { throw new SyntaxError('Syntax Error Occurred'); } catch(e){ console.error(e instanceof SyntaxError); // true console.error(e.message); // Syntax Error Occurred console.error(e.name); // SyntaxError console.error(e.stack); // SyntaxError: Syntax Error Occurred }
浏览器兼容性
5. TypeError
创建一个error实例,表示错误的原因:变量或参数不属于有效类型。
new TypeError([message[, fileName[, lineNumber]]])
下面情况会引发 TypeError
:
- 在传递和预期的函数的参数或操作数之间存在类型不兼容。
- 试图更新无法更改的值。
- 值使用不当。
例如:
const a = 10; a = "string"; // Uncaught TypeError: Assignment to constant variable null.name // Uncaught TypeError: Cannot read property 'name' of null
捕获TypeError
try { var num = 1; num.toUpperCase(); } catch(e){ console.log(e instanceof TypeError) // true console.log(e.message) // num.toUpperCase is not a function console.log(e.name) // "TypeError" console.log(e.stack) // TypeError: num.toUpperCase is not a function }
创建 TypeError
try { throw new TypeError('TypeError Occurred') } catch(e){ console.log(e instanceof TypeError) // true console.log(e.message) // TypeError Occurred console.log(e.name) // TypeError console.log(e.stack) // TypeError: TypeError Occurred }
浏览器兼容性
6. URIError
创建一个error实例,表示错误的原因:给 encodeURI(
)或 decodeURl()
传递的参数无效。
如果未正确使用全局URI处理功能,则会发生这种情况。
简单来说,当我们将不正确的参数传递给encodeURIComponent()或
decodeURIComponent()函数时,就会引发这种情况。
new URIError([message[, fileName[, lineNumber]]])
encodeURIComponent()
通过用表示字符的UTF-8编码的一个,两个,三个或四个转义序列替换某些字符的每个实例来对URI进行编码。
// "https%3A%2F%2Fmedium.com%2F" encodeURIComponent('https://medium.com/');
decodeURIComponent()
——对之前由encodeURIComponent
创建的统一资源标识符(Uniform Resource Identifier, URI)组件进行解码。
// https://medium.com/ decodeURIComponent("https%3A%2F%2Fmedium.com%2F")
捕捉URIError
try { decodeURIComponent('%') } catch (e) { console.log(e instanceof URIError) // true console.log(e.message) // URI malformed console.log(e.name) // URIError console.log(e.stack) // URIError: URI malformed... }
显式抛出URIError
try { throw new URIError('URIError Occurred') } catch (e) { console.log(e instanceof URIError) // true console.log(e.message) // URIError Occurred console.log(e.name) // "URIError" console.log(e.stack) // URIError: URIError Occurred.... }
浏览器兼容性
英文原文地址:http://help.dottoro.com/ljfhismo.php
作者:Isha Jauhari
更多编程相关知识,请访问:编程视频!!
以上がJavaScriptのエラーオブジェクトの詳細内容です。詳細については、PHP 中国語 Web サイトの他の関連記事を参照してください。

ホットAIツール

Undresser.AI Undress
リアルなヌード写真を作成する AI 搭載アプリ

AI Clothes Remover
写真から衣服を削除するオンライン AI ツール。

Undress AI Tool
脱衣画像を無料で

Clothoff.io
AI衣類リムーバー

AI Hentai Generator
AIヘンタイを無料で生成します。

人気の記事

ホットツール

メモ帳++7.3.1
使いやすく無料のコードエディター

SublimeText3 中国語版
中国語版、とても使いやすい

ゼンドスタジオ 13.0.1
強力な PHP 統合開発環境

ドリームウィーバー CS6
ビジュアル Web 開発ツール

SublimeText3 Mac版
神レベルのコード編集ソフト(SublimeText3)

ホットトピック








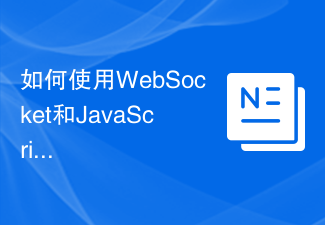
WebSocket と JavaScript を使用してオンライン音声認識システムを実装する方法 はじめに: 技術の継続的な発展により、音声認識技術は人工知能の分野の重要な部分になりました。 WebSocket と JavaScript をベースとしたオンライン音声認識システムは、低遅延、リアルタイム、クロスプラットフォームという特徴があり、広く使用されるソリューションとなっています。この記事では、WebSocket と JavaScript を使用してオンライン音声認識システムを実装する方法を紹介します。
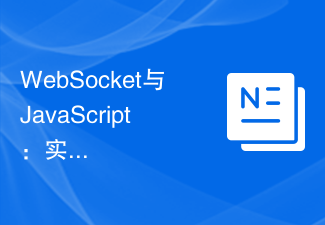
WebSocketとJavaScript:リアルタイム監視システムを実現するためのキーテクノロジー はじめに: インターネット技術の急速な発展に伴い、リアルタイム監視システムは様々な分野で広く利用されています。リアルタイム監視を実現するための重要なテクノロジーの 1 つは、WebSocket と JavaScript の組み合わせです。この記事では、リアルタイム監視システムにおける WebSocket と JavaScript のアプリケーションを紹介し、コード例を示し、その実装原理を詳しく説明します。 1.WebSocketテクノロジー
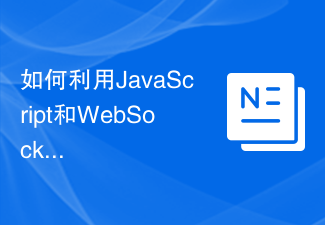
JavaScript と WebSocket を使用してリアルタイム オンライン注文システムを実装する方法の紹介: インターネットの普及とテクノロジーの進歩に伴い、ますます多くのレストランがオンライン注文サービスを提供し始めています。リアルタイムのオンライン注文システムを実装するには、JavaScript と WebSocket テクノロジを使用できます。 WebSocket は、TCP プロトコルをベースとした全二重通信プロトコルで、クライアントとサーバー間のリアルタイム双方向通信を実現します。リアルタイムオンラインオーダーシステムにおいて、ユーザーが料理を選択して注文するとき
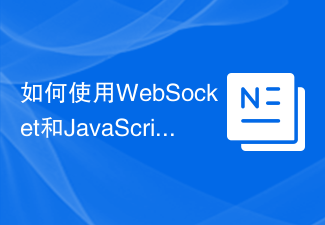
WebSocket と JavaScript を使用してオンライン予約システムを実装する方法 今日のデジタル時代では、ますます多くの企業やサービスがオンライン予約機能を提供する必要があります。効率的かつリアルタイムのオンライン予約システムを実装することが重要です。この記事では、WebSocket と JavaScript を使用してオンライン予約システムを実装する方法と、具体的なコード例を紹介します。 1. WebSocket とは何ですか? WebSocket は、単一の TCP 接続における全二重方式です。
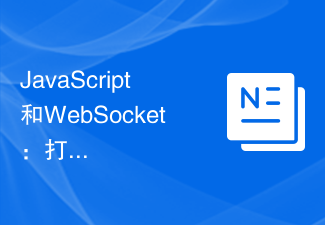
JavaScript と WebSocket: 効率的なリアルタイム天気予報システムの構築 はじめに: 今日、天気予報の精度は日常生活と意思決定にとって非常に重要です。テクノロジーの発展に伴い、リアルタイムで気象データを取得することで、より正確で信頼性の高い天気予報を提供できるようになりました。この記事では、JavaScript と WebSocket テクノロジを使用して効率的なリアルタイム天気予報システムを構築する方法を学びます。この記事では、具体的なコード例を通じて実装プロセスを説明します。私たちは
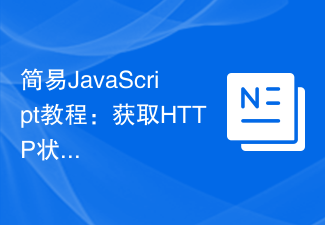
JavaScript チュートリアル: HTTP ステータス コードを取得する方法、特定のコード例が必要です 序文: Web 開発では、サーバーとのデータ対話が頻繁に発生します。サーバーと通信するとき、多くの場合、返された HTTP ステータス コードを取得して操作が成功したかどうかを判断し、さまざまなステータス コードに基づいて対応する処理を実行する必要があります。この記事では、JavaScript を使用して HTTP ステータス コードを取得する方法を説明し、いくつかの実用的なコード例を示します。 XMLHttpRequestの使用

使用法: JavaScript では、insertBefore() メソッドを使用して、DOM ツリーに新しいノードを挿入します。このメソッドには、挿入される新しいノードと参照ノード (つまり、新しいノードが挿入されるノード) の 2 つのパラメータが必要です。
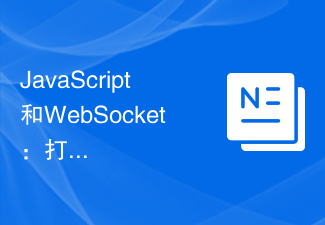
JavaScript は Web 開発で広く使用されているプログラミング言語であり、WebSocket はリアルタイム通信に使用されるネットワーク プロトコルです。 2 つの強力な機能を組み合わせることで、効率的なリアルタイム画像処理システムを構築できます。この記事では、JavaScript と WebSocket を使用してこのシステムを実装する方法と、具体的なコード例を紹介します。まず、リアルタイム画像処理システムの要件と目標を明確にする必要があります。リアルタイムの画像データを収集できるカメラ デバイスがあるとします。
