ES6 アロー関数の実践コード例
この記事では、javascript に関する関連知識を提供します。主にアロー関数の適用に関する問題を紹介します。アロー関数式は、本来匿名関数が必要な場所に適しており、使用できません。皆さんの参考になれば幸いです。
[関連する推奨事項: JavaScript ビデオ チュートリアル 、Web フロントエンド ]
はじめに
アロー関数式の構文は関数式よりも簡潔であり、独自の this、引数、super または new.target を持ちません。アロー関数式は、匿名関数が必要であり、コンストラクターとして使用できない場合に適しています。 ---- MDN
基本的な使用法
パラメータ表現
(param1, param2, …, paramN) => { statements } (param1, param2, …, paramN) => expression //相当于:(param1, param2, …, paramN) =>{ return expression; } // 当只有一个参数时,圆括号是可选的: (singleParam) => { statements } singleParam => { statements } // 没有参数的函数应该写成一对圆括号。 () => { statements }
戻り値表現
let add1 = (num1, num2) => { num1 + num2 }; let add2 = (num1, num2) => { return num1 + num2 }; let add3 = (num1, num2) => (num1 + num2); let add4 = (num1, num2) => num1 + num2; console.log(add1(2, 3)); // undefined console.log(add2(2, 3)); // 5 console.log(add3(2, 3)); // 5 console.log(add4(2, 3)); // 5
高度な
//加括号的函数体返回对象字面量表达式: let func = () => ({foo: 'bar'}) console.log(func()); // {foo: 'bar'} //支持剩余参数和默认参数 (param1, param2, ...rest) => { statements } (param1 = defaultValue1, param2, …, paramN = defaultValueN) => { statements } //同样支持参数列表解构 let f = ([a, b] = [1, 2], {x: c} = {x: a + b}) => a + b + c; f(); // 6
this
ベスト プラクティス
- 匿名関数またはインライン コールバック関数を使用する必要がある場合は、アロー関数を使用してください。 eslint:prefer-arrow-callback, arrow-spacing
why?構文はより簡潔で、これはより期待に沿ったものです
関数のロジックが非常に複雑な場合は、名前付き関数を使用する必要があります。
// bad[1, 2, 3].map(function (x) { const y = x + 1; return x * y;});// good[1, 2, 3].map((x) => { const y = x + 1; return x * y;});
- 関数本体にステートメントが 1 つしかなく、そのステートメントに副作用がない場合。暗黙的に返すには短縮形式を使用し、明示的に返すには完全形式を使用します。
eslint: arrow-parens, arrow-body-style
// bad [1, 2, 3].map(number => { const nextNumber = number + 1; `A string containing the ${nextNumber}.`; }); // good [1, 2, 3].map(number => `A string containing the ${number}.`); // good [1, 2, 3].map((number) => { const nextNumber = number + 1; return `A string containing the ${nextNumber}.`; }); // good [1, 2, 3].map((number, index) => ({ [index]: number, })); // No implicit return with side effects function foo(callback) { const val = callback(); if (val === true) { // Do something if callback returns true } } let bool = false; // bad foo(() => bool = true); // good foo(() => { bool = true; });
- 式が複数行を占める場合は、可読性を高めるために括弧を使用してください
why? 関数の始まりと終わりはより明確です
// bad['get', 'post', 'put'].map(httpMethod => Object.prototype.hasOwnProperty.call( httpMagicObjectWithAVeryLongName, httpMethod, ));// good['get', 'post', 'put'].map(httpMethod => ( Object.prototype.hasOwnProperty.call( httpMagicObjectWithAVeryLongName, httpMethod, )));
- 関数にパラメーターが 1 つしかない場合は、括弧と中括弧を省略します。それ以外の場合は、一貫性を維持するために常に完全な書き込み方法を使用してください。 eslint: arrow-parens
// bad[1, 2, 3].map((x) => x * x);// good[1, 2, 3].map(x => x * x);// good[1, 2, 3].map(number => ( `A long string with the ${number}. It’s so long that we don’t want it to take up space on the .map line!`));// bad[1, 2, 3].map(x => { const y = x + 1; return x * y;});// good[1, 2, 3].map((x) => { const y = x + 1; return x * y;});
- 明確な => 構文を使用して、= と区別します。 eslint: no-confusing-arrow
// badconst itemHeight = item => item.height > 256 ? item.largeSize : item.smallSize;// badconst itemHeight = (item) => item.height > 256 ? item.largeSize : item.smallSize;// goodconst itemHeight = item => (item.height > 256 ? item.largeSize : item.smallSize);// goodconst itemHeight = (item) => { const { height, largeSize, smallSize } = item; return height > 256 ? largeSize : smallSize;
簡単な結論
アロー関数は new を使用してコンストラクターのインスタンスを作成できませんが、通常の関数は使用できます。 (アロー関数が作成されるとき、プログラムはそのための構築メソッドを作成しません。つまり、構築能力がなく、使用後に破棄されます。再利用される通常の関数とは異なり、コンストラクター プロトタイプ、つまりプロトタイプ属性は自動的に生成されません)
プログラムはアロー関数の引数オブジェクトを作成しません
これ通常の関数では動的ですが、アロー関数の this は、アロー関数をタイトにラップするオブジェクト (定義時に決定) を指します。
アロー関数は、 this の値を変更することはできません。 bind、call、apply は実行できますが、これらのメソッドを呼び出すことはできます (this の値がこれらのメソッドによって制御されないだけです)
[関連する推奨事項: javascript ビデオ チュートリアル、ウェブ フロントエンド]
以上がES6 アロー関数の実践コード例の詳細内容です。詳細については、PHP 中国語 Web サイトの他の関連記事を参照してください。

ホットAIツール

Undresser.AI Undress
リアルなヌード写真を作成する AI 搭載アプリ

AI Clothes Remover
写真から衣服を削除するオンライン AI ツール。

Undress AI Tool
脱衣画像を無料で

Clothoff.io
AI衣類リムーバー

AI Hentai Generator
AIヘンタイを無料で生成します。

人気の記事

ホットツール

メモ帳++7.3.1
使いやすく無料のコードエディター

SublimeText3 中国語版
中国語版、とても使いやすい

ゼンドスタジオ 13.0.1
強力な PHP 統合開発環境

ドリームウィーバー CS6
ビジュアル Web 開発ツール

SublimeText3 Mac版
神レベルのコード編集ソフト(SublimeText3)

ホットトピック










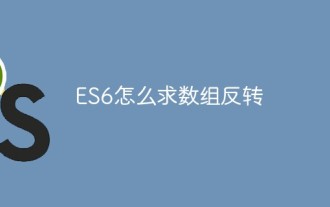
ES6 では、配列オブジェクトの reverse() メソッドを使用して、配列の反転を実現できます。このメソッドは、配列内の要素の順序を逆にして、最後の要素を最初に、最初の要素を最後に配置するために使用されます。構文「array」 。逆行する()"。 reverse() メソッドは元の配列を変更します。変更したくない場合は、拡張演算子 "..." とともに使用する必要があり、構文は "[...array].reverse() 」。
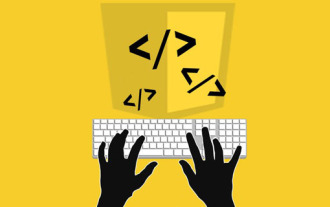
非同期はes7です。 async と await は ES7 に新しく追加されたもので、非同期操作のソリューションです。async/await は co モジュールとジェネレーター関数の糖衣構文と言え、より明確なセマンティクスで JS 非同期コードを解決します。名前が示すように、async は「非同期」を意味します。async は関数が非同期であることを宣言するために使用されます。async と await の間には厳密な規則があります。両方を互いに分離することはできず、await は async 関数内でのみ記述できます。

手順: 1. 構文 "newA=new Set(a); newB=new Set(b);" を使用して、2 つの配列をそれぞれセット型に変換します; 2. has() と filter() を使用して差分セットを検索します、構文 " new Set([...newA].filter(x =>!newB.has(x)))" では、差分セット要素がセット コレクションに含まれて返されます。 3. 配列を使用します。 from セットを配列に変換するタイプ、構文は「Array.from(collection)」です。
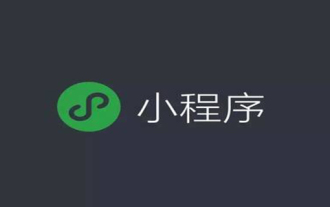
ブラウザの互換性のため。 ES6 は JS の新しい仕様として、多くの新しい構文と API を追加していますが、最新のブラウザーは ES6 の新機能を高度にサポートしていないため、ES6 コードを ES5 コードに変換する必要があります。 WeChat Web 開発者ツールでは、デフォルトで babel が使用され、開発者の ES6 構文コードを 3 つの端末すべてで適切にサポートされる ES5 コードに変換し、開発者がさまざまな環境によって引き起こされる開発上の問題を解決できるようにします。プロジェクト内でのみ設定して確認するだけです。 「ES6~ES5」オプション。
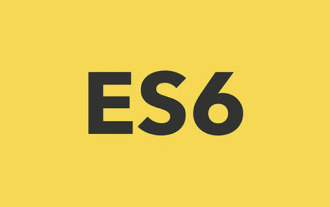
いいえ、require は CommonJS 仕様のモジュール構文であり、es6 仕様のモジュール構文は import です。 require は実行時にロードされ、import はコンパイル時にロードされます。require はコード内のどこにでも記述できます。import はファイルの先頭にのみ記述でき、条件文や関数スコープでは使用できません。モジュール属性は導入されるだけです。 require を実行した場合、そのためパフォーマンスは比較的低くなりますが、インポート コンパイル中に導入されたモジュールのプロパティのパフォーマンスはわずかに高くなります。
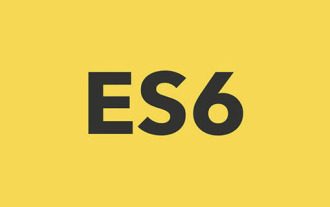
es6 では、一時的なデッド ゾーンは構文エラーであり、ブロックを閉じたスコープにする let および const コマンドを指します。コード ブロック内では、let/const コマンドを使用して変数が宣言される前に、変数は使用できず、変数が宣言される前は変数の「デッド ゾーン」に属します。これは構文上「一時デッド ゾーン」と呼ばれます。 ES6 では、一時的なデッド ゾーンや let ステートメントや const ステートメントでは変数のプロモーションが発生しないことを規定しています。これは主に実行時エラーを減らし、変数が宣言される前に使用されて予期しない動作が発生するのを防ぐためです。
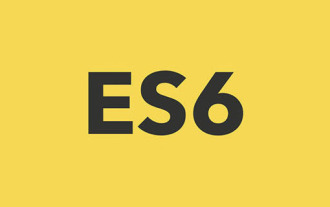
地図は注文済みです。 ES6 のマップ タイプは、多くのキーと値のペアを格納する順序付きリストです。キー名と対応する値はすべてのデータ型をサポートします。キー名の等価性は、「Objext.is()」メソッドを呼び出すことによって決定されます。 , したがって、数字の 5 と文字列「5」は 2 つのタイプとして判断され、プログラム内で 2 つの独立したキーとして現れることができます。
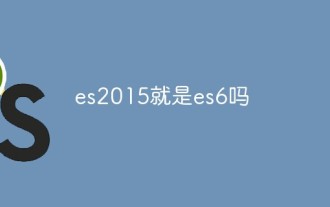
es2015はes6です。 es の正式名称は「ECMAScript」で、ECMA-262 標準に従って実装されたユニバーサルスクリプト言語です。2015 年 6 月に正式にリリースされたバージョンは、正式には ECMAScript2015 (ES2015) と呼ばれます。ECMAScript の 6 番目のバージョンであるため、 es6 と呼ばれます。
