LaravelでPHPのデコレータパターンを使用する方法について話しましょう
Laravel で PHP のデコレータ パターンを使用するにはどうすればよいですか? LaravelでのPHPデコレータモードの使い方については以下の記事で紹介していますので、ご参考になれば幸いです。
デコレータ パターン定義:
同じクラス内の他のオブジェクトに影響を与えることなく、オブジェクトに特別な動作を追加するのに役立ちます。Wikipedia:
デコレータ パターンは、単一のオブジェクトに動作を動的に追加できるようにするデザイン パターンです。同じクラス内の他のオブジェクトの動作
質問
Post モデルがあるとします。class Post extends Model { public function scopePublished($query) { return $query->where('published_at', '<=', 'NOW()'); } }
class PostsController extends Controller { public function index() { $posts = Post::published()->get(); return $posts; } }
class PostsController extends Controller { public function index() { $minutes = 1440; # 1 day $posts = Cache::remember('posts', $minutes, function () { return Post::published()->get(); }); return $posts; } }
ウェアハウス パターン
ほとんどの場合、ウェアハウス パターンはデコレータ パターンに接続されています。 まず、repository パターンを使用して投稿の取得方法を分離し、次のような app/Repositories/Posts/PostsRepositoryInterface.php
namespace App\Repositories\Posts; interface PostsRepositoryInterface { public function get(); public function find(int $id); }
<div class="code" style="position:relative; padding:0px; margin:0px;"><pre class="brush:php;toolbar:false">namespace App\Repositories\Posts;
use App\Post;
class PostsRepository implements PostsRepositoryInterface
{
protected $model;
public function __construct(Post $model) {
$this->model = $model;
}
public function get() {
return $this->model->published()->get();
}
public function find(int $id) {
return $this->model->published()->find($id);
}
}</pre><div class="contentsignin">ログイン後にコピー</div></div>
PostsController に戻り、変更を
namespace App\Http\Controllers; use App\Repositories\Posts\PostsRepositoryInterface; use Illuminate\Http\Request; class PostsController extends Controller { public function index(PostsRepositoryInterface $postsRepo) { return $postsRepo->get(); } }
に適用します。コントローラーは正常になり、詳細を十分に知っているので、仕事をやり遂げることができます。
ここでは、Laravel の IOC を利用して、Posts インターフェースの特定のオブジェクトを挿入して投稿を取得します。
必要なのは、インターフェースを使用するときに作成するクラスを Laravel の IOC に指示することだけです。
あなたの
app/Providers/AppServiceProvider.php にバインディング メソッドを追加します <div class="code" style="position:relative; padding:0px; margin:0px;"><pre class="brush:php;toolbar:false">namespace App\Providers;
use App\Repositories\Posts\PostsRepositoryInterface;
use App\Repositories\Posts\PostsRepository;
use Illuminate\Support\ServiceProvider;
class AppServiceProvider extends ServiceProvider
{
public function register()
{
$this->app->bind(PostsRepositoryInterface::class,PostsRepository::class);
}
}</pre><div class="contentsignin">ログイン後にコピー</div></div>
Now を注入するたびに
Laravel は # のインスタンスを作成します#PostsRepository を返し、それを返します。
デコレータによるキャッシュ冒頭で、デコレータ パターンを使用すると、オブジェクト内の他のオブジェクトに影響を与えることなく、単一のオブジェクトに動作を追加できると述べました。同じクラス。
ここではキャッシュが動作であり、オブジェクト/クラスはPostsRepository
です。 次の内容を含む
PostsCacheRepository を
に作成しましょう このクラスでは、Caching オブジェクトと PostsRepository オブジェクトを受け入れ、クラス (デコレータ) を使用して、PostsRepository インスタンスにキャッシュ動作を追加します。 同じ例を使用して、HTTP リクエストを何らかのサービスに送信し、失敗した場合にはモデルを返すことができます。このパターンと、動作の追加がいかに簡単であるかがメリットになると思います。
最後に、AppServiceProvider インターフェイス バインディングを変更して、PostsRepository の代わりに PostsCacheRepository インスタンスを作成します。
namespace App\Repositories\Posts; use App\Post; use Illuminate\Cache\CacheManager; class PostsCacheRepository implements PostsRepositoryInterface { protected $repo; protected $cache; const TTL = 1440; # 1 day public function __construct(CacheManager $cache, PostsRepository $repo) { $this->repo = $repo; $this->cache = $cache; } public function get() { return $this->cache->remember('posts', self::TTL, function () { return $this->repo->get(); }); } public function find(int $id) { return $this->cache->remember('posts.'.$id, self::TTL, function () { return $this->repo->find($id); }); } }
ここでファイルをもう一度確認すると、読み取りと保守が非常に簡単であることがわかります。 。同様に、ある時点でキャッシュ層を削除することにした場合もテスト可能です。
AppServiceProviderのバインディングを変更するだけです。追加の変更は必要ありません。
#結論
デコレータ パターンを使用してモデルをキャッシュする方法を学びましたウェアハウスがどのように機能するかを示しましたパターン デコレータ パターンへの接続依存性注入と Laravel IOC で私たちの生活が楽になる方法
- Laravel コンポーネントは強力です
- この記事を楽しんで読んでいただければ幸いです。強力なデザイン パターンと、プロジェクトの保守と管理を容易にする方法を示します。
- 元のアドレス: https://dev.to/ahmedash95/design-patterns-in-php-decorator-with -laravel-5hk6
laravel ビデオ チュートリアル
]以上がLaravelでPHPのデコレータパターンを使用する方法について話しましょうの詳細内容です。詳細については、PHP 中国語 Web サイトの他の関連記事を参照してください。

ホットAIツール

Undresser.AI Undress
リアルなヌード写真を作成する AI 搭載アプリ

AI Clothes Remover
写真から衣服を削除するオンライン AI ツール。

Undress AI Tool
脱衣画像を無料で

Clothoff.io
AI衣類リムーバー

AI Hentai Generator
AIヘンタイを無料で生成します。

人気の記事

ホットツール

メモ帳++7.3.1
使いやすく無料のコードエディター

SublimeText3 中国語版
中国語版、とても使いやすい

ゼンドスタジオ 13.0.1
強力な PHP 統合開発環境

ドリームウィーバー CS6
ビジュアル Web 開発ツール

SublimeText3 Mac版
神レベルのコード編集ソフト(SublimeText3)

ホットトピック








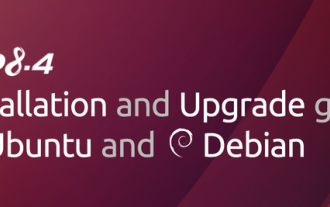
PHP 8.4 では、いくつかの新機能、セキュリティの改善、パフォーマンスの改善が行われ、かなりの量の機能の非推奨と削除が行われています。 このガイドでは、Ubuntu、Debian、またはその派生版に PHP 8.4 をインストールする方法、または PHP 8.4 にアップグレードする方法について説明します。
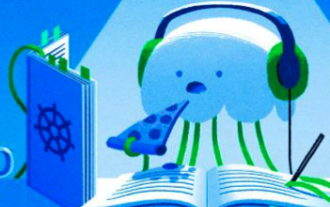
Visual Studio Code (VS Code とも呼ばれる) は、すべての主要なオペレーティング システムで利用できる無料のソース コード エディター (統合開発環境 (IDE)) です。 多くのプログラミング言語の拡張機能の大規模なコレクションを備えた VS Code は、
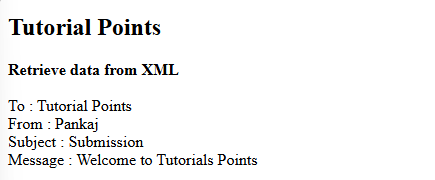
このチュートリアルでは、PHPを使用してXMLドキュメントを効率的に処理する方法を示しています。 XML(拡張可能なマークアップ言語)は、人間の読みやすさとマシン解析の両方に合わせて設計された多用途のテキストベースのマークアップ言語です。一般的にデータストレージに使用されます
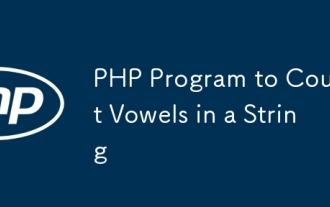
文字列は、文字、数字、シンボルを含む一連の文字です。このチュートリアルでは、さまざまな方法を使用してPHPの特定の文字列内の母音の数を計算する方法を学びます。英語の母音は、a、e、i、o、u、そしてそれらは大文字または小文字である可能性があります。 母音とは何ですか? 母音は、特定の発音を表すアルファベットのある文字です。大文字と小文字など、英語には5つの母音があります。 a、e、i、o、u 例1 入力:string = "tutorialspoint" 出力:6 説明する 文字列「TutorialSpoint」の母音は、u、o、i、a、o、iです。合計で6元があります
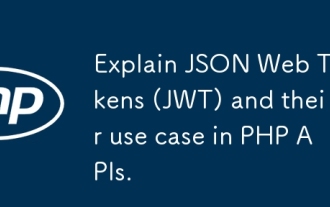
JWTは、JSONに基づくオープン標準であり、主にアイデンティティ認証と情報交換のために、当事者間で情報を安全に送信するために使用されます。 1。JWTは、ヘッダー、ペイロード、署名の3つの部分で構成されています。 2。JWTの実用的な原則には、JWTの生成、JWTの検証、ペイロードの解析という3つのステップが含まれます。 3. PHPでの認証にJWTを使用する場合、JWTを生成および検証でき、ユーザーの役割と許可情報を高度な使用に含めることができます。 4.一般的なエラーには、署名検証障害、トークンの有効期限、およびペイロードが大きくなります。デバッグスキルには、デバッグツールの使用とロギングが含まれます。 5.パフォーマンスの最適化とベストプラクティスには、適切な署名アルゴリズムの使用、有効期間を合理的に設定することが含まれます。
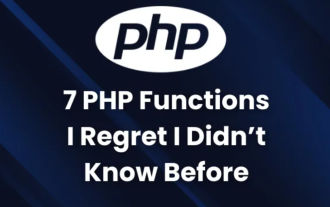
あなたが経験豊富な PHP 開発者であれば、すでにそこにいて、すでにそれを行っていると感じているかもしれません。あなたは、運用を達成するために、かなりの数のアプリケーションを開発し、数百万行のコードをデバッグし、大量のスクリプトを微調整してきました。
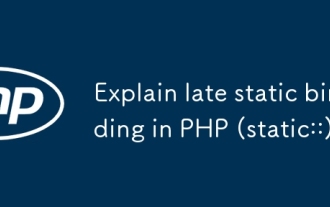
静的結合(静的::) PHPで後期静的結合(LSB)を実装し、クラスを定義するのではなく、静的コンテキストで呼び出しクラスを参照できるようにします。 1)解析プロセスは実行時に実行されます。2)継承関係のコールクラスを検索します。3)パフォーマンスオーバーヘッドをもたらす可能性があります。
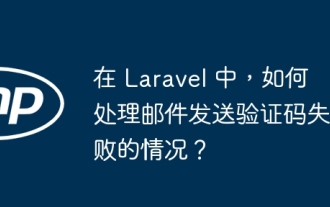
Laravelの電子メールの検証コードの送信の障害を処理する方法は、Laravelを使用することです...
