Golang で色を比較する方法について話しましょう
Golang は、Google によって開発されたオープンソース プログラミング言語で、Web 開発、クラウド コンピューティング、ビッグ データ処理などの分野で広く使用されています。 Golang では画像の処理は非常に一般的な作業であり、画像内の色の処理も重要な作業です。この記事ではGolangで色を比較する方法を紹介します。
1. 色の表現
Golang では、一般的に色の表現方法として RGB 値と 16 進値が使用されます。 RGB (赤、緑、青) 値は 3 つの原色の値を指し、通常は 3 つの整数 (0 ~ 255) で表されます。
type RGB struct { R, G, B uint8 }
hex 値は 16 進数で表される色の値で、通常は表されます。 6 桁の文字列です (「#FFFFFF」は白を意味します):
type Hex struct { R, G, B uint8 }
また、色を相対的に表現する HSV (色相、彩度、明度) 値としての色表現方法もあります。直感的な色の表現方法ですが、この記事ではあまり紹介しません。
2. 色の比較
2 つの色の類似性の比較は、通常、それらの距離を計算することで実現できます。 Golang では、ユークリッド距離またはマンハッタン距離を使用して色間の距離を計算できます。
ユークリッド距離は 2 点間の直線距離を指します:
func euclideanDistance(c1, c2 RGB) float64 { r := float64(c1.R) - float64(c2.R) g := float64(c1.G) - float64(c2.G) b := float64(c1.B) - float64(c2.B) return math.Sqrt(r*r + g*g + b*b) }
マンハッタン距離は 2 点間の水平距離と垂直距離の合計を指します:
func manhattanDistance(c1, c2 RGB) float64 { r := math.Abs(float64(c1.R) - float64(c2.R)) g := math.Abs(float64(c1.G) - float64(c2.G)) b := math.Abs(float64(c1.B) - float64(c2.B)) return r + g + b }
Ofもちろん、上記の関数を 16 進値の色表現に適用することもできます:
func euclideanDistance(c1, c2 Hex) float64 { r1, g1, b1 := hexToRGB(c1) r2, g2, b2 := hexToRGB(c2) r := float64(r1) - float64(r2) g := float64(g1) - float64(g2) b := float64(b1) - float64(b2) return math.Sqrt(r*r + g*g + b*b) } func manhattanDistance(c1, c2 Hex) float64 { r1, g1, b1 := hexToRGB(c1) r2, g2, b2 := hexToRGB(c2) r := math.Abs(float64(r1) - float64(r2)) g := math.Abs(float64(g1) - float64(g2)) b := math.Abs(float64(b1) - float64(b2)) return r + g + b } func hexToRGB(c Hex) (uint8, uint8, uint8) { return c.R, c.G, c.B }
3. カラー コントラストのアプリケーション
カラー コントラストは、色の置換や色などの画像処理シナリオでよく使用されます。分析。たとえば、色置換関数を使用して、特定の色を別の色に置き換えることができます。
func replaceColor(img image.Image, oldColor, newColor RGB, threshold float64) image.Image { bounds := img.Bounds() out := image.NewRGBA(bounds) for x := bounds.Min.X; x < bounds.Max.X; x++ { for y := bounds.Min.Y; y < bounds.Max.Y; y++ { pixel := img.At(x, y) c := RGBModel.Convert(pixel).(RGB) distance := euclideanDistance(c, oldColor) if distance <= threshold { out.Set(x, y, newColor) } else { out.Set(x, y, pixel) } } } return out }
また、色分析関数を使用して、画像内の特定の色のピクセルを見つけ、その数を数えることができます :
func getColorCount(img image.Image, color RGB, threshold float64) int { bounds := img.Bounds() count := 0 for x := bounds.Min.X; x < bounds.Max.X; x++ { for y := bounds.Min.Y; y < bounds.Max.Y; y++ { pixel := img.At(x, y) c := RGBModel.Convert(pixel).(RGB) distance := euclideanDistance(c, color) if distance <= threshold { count++ } } } return count }
4. まとめ
この記事では、Golang で色を比較する方法と、画像処理にカラー コントラスト関数を適用する方法を紹介します。カラーコントラストは画像処理において重要な技術であり、これを習得することは画像処理の効率と精度を向上させる上で非常に重要です。
以上がGolang で色を比較する方法について話しましょうの詳細内容です。詳細については、PHP 中国語 Web サイトの他の関連記事を参照してください。

ホットAIツール

Undresser.AI Undress
リアルなヌード写真を作成する AI 搭載アプリ

AI Clothes Remover
写真から衣服を削除するオンライン AI ツール。

Undress AI Tool
脱衣画像を無料で

Clothoff.io
AI衣類リムーバー

AI Hentai Generator
AIヘンタイを無料で生成します。

人気の記事

ホットツール

メモ帳++7.3.1
使いやすく無料のコードエディター

SublimeText3 中国語版
中国語版、とても使いやすい

ゼンドスタジオ 13.0.1
強力な PHP 統合開発環境

ドリームウィーバー CS6
ビジュアル Web 開発ツール

SublimeText3 Mac版
神レベルのコード編集ソフト(SublimeText3)

ホットトピック








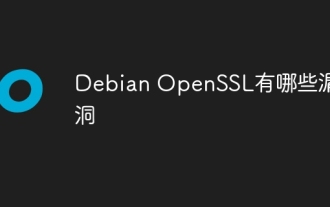
OpenSSLは、安全な通信で広く使用されているオープンソースライブラリとして、暗号化アルゴリズム、キー、証明書管理機能を提供します。ただし、その歴史的バージョンにはいくつかの既知のセキュリティの脆弱性があり、その一部は非常に有害です。この記事では、Debian SystemsのOpenSSLの共通の脆弱性と対応測定に焦点を当てます。 Debianopensslの既知の脆弱性:OpenSSLは、次のようないくつかの深刻な脆弱性を経験しています。攻撃者は、この脆弱性を、暗号化キーなどを含む、サーバー上の不正な読み取りの敏感な情報に使用できます。
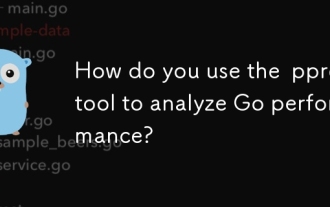
この記事では、プロファイリングの有効化、データの収集、CPUやメモリの問題などの一般的なボトルネックの識別など、GOパフォーマンスを分析するためにPPROFツールを使用する方法について説明します。
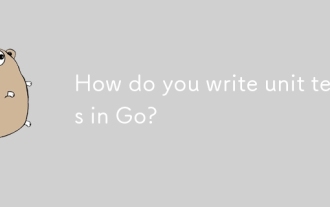
この記事では、GOでユニットテストを書くことで、ベストプラクティス、モッキングテクニック、効率的なテスト管理のためのツールについて説明します。
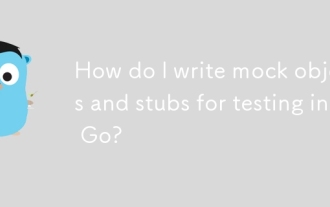
この記事では、ユニットテストのためにGOのモックとスタブを作成することを示しています。 インターフェイスの使用を強調し、模擬実装の例を提供し、模擬フォーカスを維持し、アサーションライブラリを使用するなどのベストプラクティスについて説明します。 articl
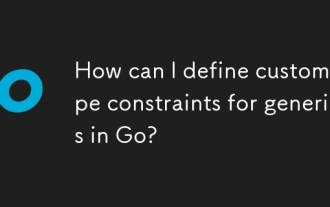
この記事では、GENICSのGOのカスタムタイプの制約について説明します。 インターフェイスがジェネリック関数の最小タイプ要件をどのように定義するかを詳しく説明し、タイプの安全性とコードの再利用性を改善します。 この記事では、制限とベストプラクティスについても説明しています
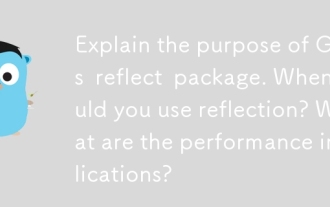
この記事では、コードのランタイム操作に使用されるGoの反射パッケージについて説明します。シリアル化、一般的なプログラミングなどに有益です。実行やメモリの使用量の増加、賢明な使用と最高のアドバイスなどのパフォーマンスコストについて警告します
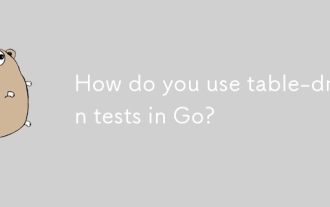
この記事では、GOでテーブル駆動型のテストを使用して説明します。これは、テストのテーブルを使用して複数の入力と結果を持つ関数をテストする方法です。読みやすさの向上、重複の減少、スケーラビリティ、一貫性、および
