PHP WebDriver をフォームテストと入力テストに使用する方法
Jun 15, 2023 am 10:20 AMPHP 開発者にとって、テストは非常に重要なステップです。テストでは、フォーム テストと入力テストが非常に一般的なテスト タイプです。どちらのタイプのテストでも、PHP WebDriver は非常に適したテスト ツールです。この記事では、フォーム テストと入力テストに PHP WebDriver を使用する方法を詳しく説明します。
PHP WebDriver とは何ですか?
まず、PHP WebDriver とは何かを理解しましょう。 PHP WebDriver は、Selenium WebDriver に基づく PHP ラッパーです。 Firefox、Chrome などのさまざまなブラウザを駆動して、ユーザーの操作をシミュレートできます。 PHP WebDriver を使用して、フォーム テストや入力テストなどのさまざまなテストを実行できます。
フォーム テスト
フォーム テストは一般的なタイプのテストで、主に Web サイト内のさまざまなフォーム (登録フォーム、ログイン フォームなど) をテストするために使用されます。 PHP WebDriverを使ってフォームテストを行う方法を紹介します。
1. 環境をセットアップする
まず、PHP WebDriver をインストールする必要があります。 PHP WebDriver は Composer を介してインストールできます。具体的な手順は次のとおりです:
(1) 現在のプロジェクトのディレクトリに次の内容を含むcomposer.json ファイルを作成します:
{
"require": { "php-webdriver/webdriver": "dev-master" }
}
#(2) ターミナルでcomposer.jsonファイルが存在するディレクトリを入力し、次のコマンドを実行します: composer install2. テスト クラスの作成次に、テスト クラスを作成し、このクラスにテスト コードを記述する必要があります。テストコードはPHPUnitを使用して記述できます。以下はサンプル コードです: use PHPUnitFrameworkTestCase;use FacebookWebDriverRemoteRemoteWebDriver;
use FacebookWebDriverWebDriverBy;
use FacebookWebDriverWebDriverExpectedCondition;
{
/** * @var RemoteWebDriver */ protected $webDriver; public function setUp() { $capabilities = [ 'browserName' => 'chrome', ]; $this->webDriver = RemoteWebDriver::create('http://localhost:4444/wd/hub', $capabilities); $this->webDriver->manage()->window()->maximize(); } public function tearDown() { $this->webDriver->quit(); } public function testForm() { $this->webDriver->get('http://localhost/form/'); $this->webDriver->findElement(WebDriverBy::id('name'))->sendKeys('test'); $this->webDriver->findElement(WebDriverBy::id('email'))->sendKeys('test@example.com'); $this->webDriver->findElement(WebDriverBy::id('password'))->sendKeys('password'); $this->webDriver->findElement(WebDriverBy::id('confirm_password'))->sendKeys('password'); $this->webDriver->findElement(WebDriverBy::id('submit'))->click(); $this->webDriver->wait(10)->until( WebDriverExpectedCondition::urlContains('success.php') ); }
use FacebookWebDriverRemoteRemoteWebDriver;
use FacebookWebDriverWebDriverBy;
use FacebookWebDriverWebDriverExpectedCondition;
{
/** * @var RemoteWebDriver */ protected $webDriver; public function setUp() { $capabilities = [ 'browserName' => 'chrome', ]; $this->webDriver = RemoteWebDriver::create('http://localhost:4444/wd/hub', $capabilities); $this->webDriver->manage()->window()->maximize(); } public function tearDown() { $this->webDriver->quit(); } public function testInput() { $this->webDriver->get('http://localhost/input/'); $this->webDriver->findElement(WebDriverBy::id('input'))->sendKeys('test'); $this->webDriver->findElement(WebDriverBy::id('select'))->click(); $this->webDriver->findElement(WebDriverBy::xpath('//option[text()="option1"]'))->click(); $this->webDriver->findElement(WebDriverBy::id('checkbox1'))->click(); $this->webDriver->findElement(WebDriverBy::id('radio1'))->click(); }
以上がPHP WebDriver をフォームテストと入力テストに使用する方法の詳細内容です。詳細については、PHP 中国語 Web サイトの他の関連記事を参照してください。

人気の記事

人気の記事

ホットな記事タグ

メモ帳++7.3.1
使いやすく無料のコードエディター

SublimeText3 中国語版
中国語版、とても使いやすい

ゼンドスタジオ 13.0.1
強力な PHP 統合開発環境

ドリームウィーバー CS6
ビジュアル Web 開発ツール

SublimeText3 Mac版
神レベルのコード編集ソフト(SublimeText3)

ホットトピック










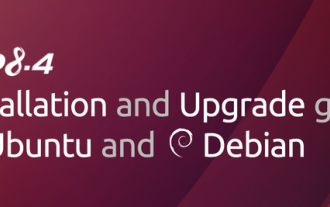
Ubuntu および Debian 用の PHP 8.4 インストールおよびアップグレード ガイド
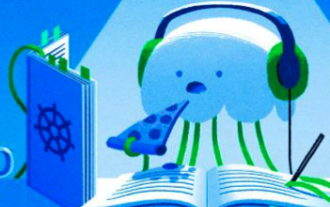
PHP 開発用に Visual Studio Code (VS Code) をセットアップする方法
