PHP および WebRTC プロトコルを使用してリアルタイムのオーディオおよびビデオ通信を行う方法
PHP および WebRTC プロトコルを使用してリアルタイムのオーディオおよびビデオ通信を行う方法
今日のインターネット時代において、リアルタイムのオーディオおよびビデオ通信は人々の日常生活に不可欠な部分となっています。 WebRTC (Web Real-Time Communication) テクノロジは、オープンなリアルタイム通信標準として、Web アプリケーションにリアルタイムのオーディオおよびビデオ通信を組み込むための強力なサポートを提供します。この記事では、リアルタイムのオーディオおよびビデオ通信に PHP および WebRTC プロトコルを使用する方法を紹介し、対応するコード例を示します。
- WebRTC の概要
WebRTC は、Google が開発、推進しているリアルタイム通信標準であり、Web ブラウザ上で音声、ビデオ、データのリアルタイム伝送を実現できます。これは標準ネットワーク プロトコル (HTTP や WebSocket など) と JavaScript API に基づいており、追加のプラグインや拡張機能を必要とせずに、P2P テクノロジを介したリアルタイム データ送信を可能にします。 - 準備作業
PHP と WebRTC を使用してリアルタイムの音声およびビデオ通信を開始する前に、いくつかの準備作業を行う必要があります。まず、最新バージョンの PHP と Web サーバー (Apache や Nginx など) がインストールされていることを確認します。次に、Google Chrome や Mozilla Firefox など、WebRTC をサポートするブラウザも必要になります。 - サーバーのセットアップ
リアルタイムの音声およびビデオ通信を実現するには、2 者間のシグナリングを調整して送信するシグナリング サーバーを構築する必要があります。 PHP では、WebSocket テクノロジーを使用してシグナリング サーバーを実装できます。
以下は、Ratchet WebSocket ライブラリを使用して実装された単純なシグナリング サーバーの例です:
<?php use RatchetMessageComponentInterface; use RatchetConnectionInterface; require 'vendor/autoload.php'; class SignalingServer implements MessageComponentInterface { protected $clients; public function __construct() { $this->clients = new SplObjectStorage; } public function onOpen(ConnectionInterface $conn) { $this->clients->attach($conn); } public function onMessage(ConnectionInterface $from, $msg) { foreach ($this->clients as $client) { if ($client !== $from) { $client->send($msg); } } } public function onClose(ConnectionInterface $conn) { $this->clients->detach($conn); } public function onError(ConnectionInterface $conn, Exception $e) { $conn->close(); } } $server = RatchetServerIoServer::factory( new RatchetHttpHttpServer( new RatchetWebSocketWsServer( new SignalingServer() ) ), 8080 ); $server->run();
Ratchet WebSocket ライブラリは、WebSocket を実装するために上記のコードで使用されていることに注意してください。サーバ。 Composer を使用してライブラリをインストールできます。
- WebRTC アプリケーションの作成
クライアント側では、WebRTC テクノロジを使用して、リアルタイムのオーディオおよびビデオ通信アプリケーションを作成します。 HTML5 と JavaScript を通じて実現できます。
以下は、単純な WebRTC アプリケーションのコード例です。
<!DOCTYPE html> <html> <head> <title>WebRTC Video Chat</title> </head> <body> <video id="localVideo" autoplay></video> <video id="remoteVideo" autoplay></video> <button id="startButton">Start Call</button> <button id="hangupButton">Hang Up</button> <script src="https://webrtc.github.io/adapter/adapter-latest.js"></script> <script> const startButton = document.getElementById('startButton'); const hangupButton = document.getElementById('hangupButton'); const localVideo = document.getElementById('localVideo'); const remoteVideo = document.getElementById('remoteVideo'); let localStream; let peerConnection; startButton.addEventListener('click', startCall); hangupButton.addEventListener('click', hangup); async function startCall() { localStream = await navigator.mediaDevices.getUserMedia({audio: true, video: true}); localVideo.srcObject = localStream; const configuration = {iceServers: [{urls: 'stun:stun.l.google.com:19302'}]}; peerConnection = new RTCPeerConnection(configuration); peerConnection.addEventListener('icecandidate', handleIceCandidate); peerConnection.addEventListener('track', handleRemoteStreamAdded); localStream.getTracks().forEach(track => { peerConnection.addTrack(track, localStream); }); const offer = await peerConnection.createOffer(); await peerConnection.setLocalDescription(offer); // 将信令通过WebSocket发送给信令服务器 sendSignaling(JSON.stringify(offer)); } async function handleIceCandidate(event) { if (event.candidate) { sendSignaling(JSON.stringify({ice: event.candidate})); } } async function handleRemoteStreamAdded(event) { remoteVideo.srcObject = event.streams[0]; } async function hangup() { localStream.getTracks().forEach(track => { track.stop(); }); peerConnection.close(); // 发送挂断信令给信令服务器 sendSignaling(JSON.stringify({hangup: true})); } function sendSignaling(message) { const ws = new WebSocket('ws://localhost:8080'); ws.addEventListener('open', () => { ws.send(message); ws.close(); }); } </script> </body> </html>
上記のコードでは、まず getUserMedia API を通じてローカルのオーディオおよびビデオ ストリームを取得し、それを表示します。ページ プレゼンテーションを作成します。次に、RTCPeerConnection オブジェクトを作成し、icecandidate とそのオブジェクトの追跡イベントをリッスンしました。 createOffer メソッドを通じて、デバイス間で交換するための SDP (セッション記述プロトコル) を生成し、setLocalDescription メソッドを通じてローカルの説明を設定します。最後に、この SDP シグナリングをシグナリング サーバーに送信します。
- オーディオおよびビデオ通信の実装
2 つのデバイス間でオーディオおよびビデオ通信を実現するには、シグナリング サーバーと WebRTC アプリケーションにコードを追加する必要があります。以下は簡単な実装例です:
シグナリング サーバー:
<?php // ... public function onMessage(ConnectionInterface $from, $msg) { $data = json_decode($msg); if (isset($data->sdp)) { // 处理SDP信令(包括offer和answer) foreach ($this->clients as $client) { if ($client !== $from) { $client->send($msg); } } } elseif (isset($data->ice)) { // 处理ICE候选信令 foreach ($this->clients as $client) { if ($client !== $from) { $client->send($msg); } } } elseif (isset($data->hangup)) { // 处理挂断信令 foreach ($this->clients as $client) { if ($client !== $from) { $client->send($msg); $this->onClose($client); } } } } // ...
WebRTC アプリケーション:
// ... async function handleSignalingMessage(message) { const data = JSON.parse(message); if (data.sdp) { await peerConnection.setRemoteDescription(new RTCSessionDescription(data.sdp)); if (data.sdp.type === 'offer') { const answer = await peerConnection.createAnswer(); await peerConnection.setLocalDescription(answer); // 发送回答信令给信令服务器 sendSignaling(JSON.stringify(answer)); } } else if (data.ice) { await peerConnection.addIceCandidate(new RTCIceCandidate(data.ice)); } else if (data.hangup) { // 处理挂断信令 hangup(); } } // ...
デバイス A がシグナリング サーバー経由でデバイス B への通話を開始するときデバイス B がオファー シグナリングを含む WebSocket メッセージを受信するとき。デバイス B は、リモート記述を設定することによって通話要求を受け入れ、独自の応答シグナリングを生成し、それをデバイス A に送り返します。
デバイス A がデバイス B から応答シグナリングを受信すると、リモート記述を設定し、デバイス B との接続の確立を開始します。 ICE 候補シグナリングを交換することにより、デバイス A とデバイス B は最適な通信パスを見つけます。
デバイス A またはデバイス B が通話を終了すると、シグナリング サーバーにハングアップ シグナリングが送信され、相手との接続が切断されます。
概要
PHP および WebRTC プロトコルを使用すると、リアルタイムの音声およびビデオ通信を簡単に実現できます。この記事では、WebRTC の基本原理と使用法について学び、対応するコード例を提供しました。この記事の紹介が、リアルタイムのオーディオおよびビデオ通信に PHP および WebRTC プロトコルを使用する方法を読者が理解するのに役立つことを願っています。
以上がPHP および WebRTC プロトコルを使用してリアルタイムのオーディオおよびビデオ通信を行う方法の詳細内容です。詳細については、PHP 中国語 Web サイトの他の関連記事を参照してください。

ホットAIツール

Undresser.AI Undress
リアルなヌード写真を作成する AI 搭載アプリ

AI Clothes Remover
写真から衣服を削除するオンライン AI ツール。

Undress AI Tool
脱衣画像を無料で

Clothoff.io
AI衣類リムーバー

Video Face Swap
完全無料の AI 顔交換ツールを使用して、あらゆるビデオの顔を簡単に交換できます。

人気の記事

ホットツール

メモ帳++7.3.1
使いやすく無料のコードエディター

SublimeText3 中国語版
中国語版、とても使いやすい

ゼンドスタジオ 13.0.1
強力な PHP 統合開発環境

ドリームウィーバー CS6
ビジュアル Web 開発ツール

SublimeText3 Mac版
神レベルのコード編集ソフト(SublimeText3)

ホットトピック










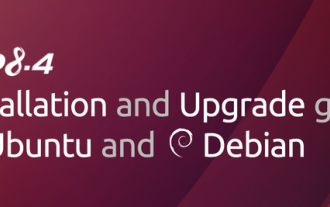
PHP 8.4 では、いくつかの新機能、セキュリティの改善、パフォーマンスの改善が行われ、かなりの量の機能の非推奨と削除が行われています。 このガイドでは、Ubuntu、Debian、またはその派生版に PHP 8.4 をインストールする方法、または PHP 8.4 にアップグレードする方法について説明します。
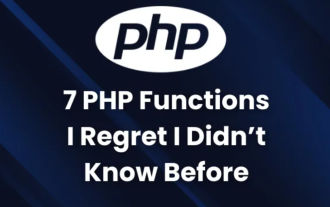
あなたが経験豊富な PHP 開発者であれば、すでにそこにいて、すでにそれを行っていると感じているかもしれません。あなたは、運用を達成するために、かなりの数のアプリケーションを開発し、数百万行のコードをデバッグし、大量のスクリプトを微調整してきました。
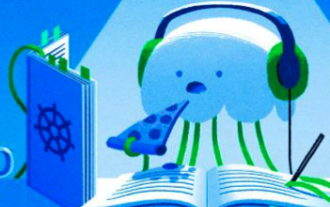
Visual Studio Code (VS Code とも呼ばれる) は、すべての主要なオペレーティング システムで利用できる無料のソース コード エディター (統合開発環境 (IDE)) です。 多くのプログラミング言語の拡張機能の大規模なコレクションを備えた VS Code は、
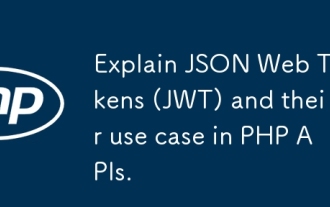
JWTは、JSONに基づくオープン標準であり、主にアイデンティティ認証と情報交換のために、当事者間で情報を安全に送信するために使用されます。 1。JWTは、ヘッダー、ペイロード、署名の3つの部分で構成されています。 2。JWTの実用的な原則には、JWTの生成、JWTの検証、ペイロードの解析という3つのステップが含まれます。 3. PHPでの認証にJWTを使用する場合、JWTを生成および検証でき、ユーザーの役割と許可情報を高度な使用に含めることができます。 4.一般的なエラーには、署名検証障害、トークンの有効期限、およびペイロードが大きくなります。デバッグスキルには、デバッグツールの使用とロギングが含まれます。 5.パフォーマンスの最適化とベストプラクティスには、適切な署名アルゴリズムの使用、有効期間を合理的に設定することが含まれます。
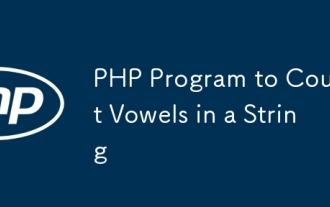
文字列は、文字、数字、シンボルを含む一連の文字です。このチュートリアルでは、さまざまな方法を使用してPHPの特定の文字列内の母音の数を計算する方法を学びます。英語の母音は、a、e、i、o、u、そしてそれらは大文字または小文字である可能性があります。 母音とは何ですか? 母音は、特定の発音を表すアルファベットのある文字です。大文字と小文字など、英語には5つの母音があります。 a、e、i、o、u 例1 入力:string = "tutorialspoint" 出力:6 説明する 文字列「TutorialSpoint」の母音は、u、o、i、a、o、iです。合計で6元があります
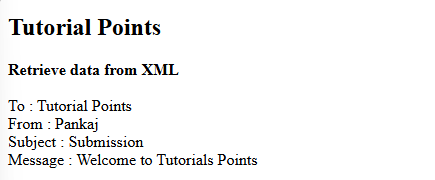
このチュートリアルでは、PHPを使用してXMLドキュメントを効率的に処理する方法を示しています。 XML(拡張可能なマークアップ言語)は、人間の読みやすさとマシン解析の両方に合わせて設計された多用途のテキストベースのマークアップ言語です。一般的にデータストレージに使用されます
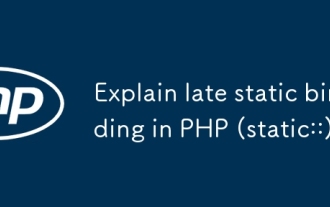
静的結合(静的::) PHPで後期静的結合(LSB)を実装し、クラスを定義するのではなく、静的コンテキストで呼び出しクラスを参照できるようにします。 1)解析プロセスは実行時に実行されます。2)継承関係のコールクラスを検索します。3)パフォーマンスオーバーヘッドをもたらす可能性があります。

PHPの魔法の方法は何ですか? PHPの魔法の方法には次のものが含まれます。1。\ _ \ _コンストラクト、オブジェクトの初期化に使用されます。 2。\ _ \ _リソースのクリーンアップに使用される破壊。 3。\ _ \ _呼び出し、存在しないメソッド呼び出しを処理します。 4。\ _ \ _ get、dynamic属性アクセスを実装します。 5。\ _ \ _セット、動的属性設定を実装します。これらの方法は、特定の状況で自動的に呼び出され、コードの柔軟性と効率を向上させます。
