Javaを使用してCMSシステムのサイトデータセキュリティバックアップ機能を実装する方法
Java を使用して CMS システムのサイト データ セキュリティ バックアップ機能を実装する方法
1. はじめに
インターネットの急速な発展に伴い、より多くの企業や個人がコンテンツ管理システム (CMS) を使用し始めています。 ) 独自の Web サイトを構築および管理します。サイト データの安全なバックアップは、Web サイトの正常な動作と回復を保証するための重要な手段です。この記事では、Java プログラミング言語を使用して CMS システムのサイト データ セキュリティ バックアップ機能を実装する方法を紹介し、関連するコード例を示します。
2. バックアップ方法の選択
サイトデータのバックアップ機能を実装する前に、まず適切なバックアップ方法を選択する必要があります。一般に、一般的なサイト データのバックアップ方法には、完全バックアップと増分バックアップが含まれます。
- 完全バックアップ
完全バックアップとは、Web ページ ファイル、データベース ファイルなどを含むサイト全体のデータの完全なバックアップを指します。通常、完全バックアップには時間がかかりますが、復元は比較的簡単で、バックアップ ファイルを元の場所に復元するだけで済みます。 - 増分バックアップ
増分バックアップとは、サイト データの新しい部分と変更された部分をバックアップすることを指します。完全バックアップと比較して、増分バックアップは時間とスペースのオーバーヘッドが少なくなります。ただし、復元する場合は、最初に完全バックアップを復元し、次に増分バックアップを完全バックアップに適用する必要があります。
バックアップ方法を選択するときは、特定のニーズとリソースの状態に基づいて比較検討する必要があります。大規模な CMS システムの場合、データのセキュリティとバックアップ効率を最大化するために、完全バックアップと増分バックアップを組み合わせて使用することを一般的に推奨します。
3. Java によるバックアップ機能の実装
Java では、ファイル操作やデータベース操作に関するクラス ライブラリを使用して、CMS システムのサイト データのバックアップ機能を実装できます。
- 完全バックアップの実装例
次は、Java を使用して完全バックアップを実装するコード例です。
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import java.nio.channels.FileChannel;
public class BackupUtils {
public static void backup(String sourcePath, String targetPath) throws IOException { File sourceFile = new File(sourcePath); if (!sourceFile.exists()) { throw new IOException("Source file does not exist."); } File targetFile = new File(targetPath); if (!targetFile.exists()) { targetFile.mkdirs(); } FileChannel sourceChannel = null; FileChannel targetChannel = null; try { sourceChannel = new FileInputStream(sourceFile).getChannel(); targetChannel = new FileOutputStream(targetFile).getChannel(); targetChannel.transferFrom(sourceChannel, 0, sourceChannel.size()); } finally { if (sourceChannel != null) { sourceChannel.close(); } if (targetChannel != null) { targetChannel.close(); } } }
}
このツール クラスを使用して、指定されたパスにあるすべてのソース ファイルをターゲット パスにバックアップします。
- 増分バックアップの実装例
次は、Java を使用して増分バックアップを実装するコード例です。
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
public class IncrementalBackupUtils {
public static void backup(String sourceFilePath, String targetFolderPath) throws IOException { File sourceFile = new File(sourceFilePath); if (!sourceFile.exists()) { throw new IOException("Source file does not exist."); } File targetFolder = new File(targetFolderPath); if (!targetFolder.exists()) { targetFolder.mkdirs(); } File targetFile = new File(targetFolder, sourceFile.getName()); byte[] buffer = new byte[1024]; int length; try (FileOutputStream output = new FileOutputStream(targetFile)) { try (FileInputStream input = new FileInputStream(sourceFile)) { while ((length = input.read(buffer)) > 0) { output.write(buffer, 0, length); } } } }
}
このツール クラスを使用して、ファイルをソース ファイルは、ソース ファイルと同じファイル名を維持したまま、ターゲット フォルダーに増分バックアップされます。
4. 概要
サイト データの安全なバックアップを確保することは、CMS システムの正常な動作と回復を保証するための重要な手段です。 Java は広く使用されているプログラミング言語として、サイト データの安全なバックアップ機能を簡単に実装できる豊富なクラス ライブラリとツールを提供します。
この記事では、完全バックアップと増分バックアップの概念を紹介し、対応する Java コード例を提供します。これにより、読者が CMS システムのサイト データ セキュリティ バックアップ機能の実装をよりよく理解し、実践できるようになります。
以上がJavaを使用してCMSシステムのサイトデータセキュリティバックアップ機能を実装する方法の詳細内容です。詳細については、PHP 中国語 Web サイトの他の関連記事を参照してください。

ホットAIツール

Undresser.AI Undress
リアルなヌード写真を作成する AI 搭載アプリ

AI Clothes Remover
写真から衣服を削除するオンライン AI ツール。

Undress AI Tool
脱衣画像を無料で

Clothoff.io
AI衣類リムーバー

AI Hentai Generator
AIヘンタイを無料で生成します。

人気の記事

ホットツール

メモ帳++7.3.1
使いやすく無料のコードエディター

SublimeText3 中国語版
中国語版、とても使いやすい

ゼンドスタジオ 13.0.1
強力な PHP 統合開発環境

ドリームウィーバー CS6
ビジュアル Web 開発ツール

SublimeText3 Mac版
神レベルのコード編集ソフト(SublimeText3)

ホットトピック








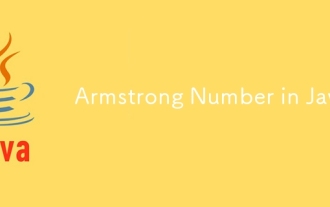
Java のアームストロング番号に関するガイド。ここでは、Java でのアームストロング数の概要とコードの一部について説明します。
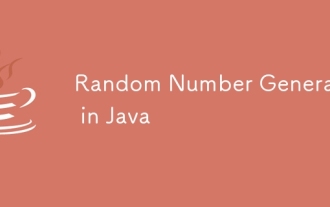
Java の乱数ジェネレーターのガイド。ここでは、Java の関数について例を挙げて説明し、2 つの異なるジェネレーターについて例を挙げて説明します。
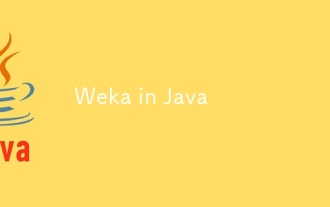
Java の Weka へのガイド。ここでは、weka java の概要、使い方、プラットフォームの種類、利点について例を交えて説明します。

この記事では、Java Spring の面接で最もよく聞かれる質問とその詳細な回答をまとめました。面接を突破できるように。
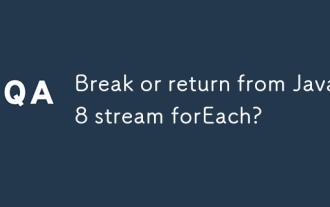
Java 8は、Stream APIを導入し、データ収集を処理する強力で表現力のある方法を提供します。ただし、ストリームを使用する際の一般的な質問は次のとおりです。 従来のループにより、早期の中断やリターンが可能になりますが、StreamのForeachメソッドはこの方法を直接サポートしていません。この記事では、理由を説明し、ストリーム処理システムに早期終了を実装するための代替方法を調査します。 さらに読み取り:JavaストリームAPIの改善 ストリームを理解してください Foreachメソッドは、ストリーム内の各要素で1つの操作を実行する端末操作です。その設計意図はです
