AngularJS_AngularJS の依存性注入メカニズムの詳細な説明
依存関係の注入は、コンポーネント内の依存関係のハードコーディングをコンポーネント内に与えることで置き換えるソフトウェア設計パターンです。これにより、1 つのコンポーネントが依存関係の検索から依存関係の構成までの作業が軽減されます。これにより、コンポーネントが再利用可能、保守可能、テスト可能になります。
AngularJS は、最高の依存関係注入メカニズムを提供します。相互に依存関係を注入できる次のコア コンポーネントを提供します。
- 値
- 工場
- サービス
- プロバイダー
- 定数値
値
値は、構成フェーズのコントローラーで値を渡すために使用される単純な JavaScript オブジェクトです。
//define a module var mainApp = angular.module("mainApp", []); //create a value object as "defaultInput" and pass it a data. mainApp.value("defaultInput", 5); ... //inject the value in the controller using its name "defaultInput" mainApp.controller('CalcController', function($scope, CalcService, defaultInput) { $scope.number = defaultInput; $scope.result = CalcService.square($scope.number); $scope.square = function() { $scope.result = CalcService.square($scope.number); } });
工場
Factory は関数の値を返すために使用されます。サービスやコントローラーが必要とするときはいつでも、オンデマンドで価値を生み出します。通常、ファクトリ関数を使用して、対応する値を計算して返します
//define a module var mainApp = angular.module("mainApp", []); //create a factory "MathService" which provides a method multiply to return multiplication of two numbers mainApp.factory('MathService', function() { var factory = {}; factory.multiply = function(a, b) { return a * b } return factory; }); //inject the factory "MathService" in a service to utilize the multiply method of factory. mainApp.service('CalcService', function(MathService){ this.square = function(a) { return MathService.multiply(a,a); } }); ...
サービス
サービスは、特定のタスクを実行する一連の関数を含む単一の JavaScript オブジェクトです。サービスは、service() 関数を使用して定義され、その後コントローラーに挿入されます。
//define a module var mainApp = angular.module("mainApp", []); ... //create a service which defines a method square to return square of a number. mainApp.service('CalcService', function(MathService){ this.square = function(a) { return MathService.multiply(a,a); } }); //inject the service "CalcService" into the controller mainApp.controller('CalcController', function($scope, CalcService, defaultInput) { $scope.number = defaultInput; $scope.result = CalcService.square($scope.number); $scope.square = function() { $scope.result = CalcService.square($scope.number); } });
プロバイダ
AngularJS 内部作成プロセスの構成フェーズ中に使用されるプロバイダー サービス、ファクトリーなど (AngularJS 自体がブートストラップするときに対応)。以下で説明するスクリプトを使用して、以前に作成した MathService を作成できます。プロバイダーは、値/サービス/ファクトリーを返す特別なファクトリ メソッドと get() メソッドです。
//define a module var mainApp = angular.module("mainApp", []); ... //create a service using provider which defines a method square to return square of a number. mainApp.config(function($provide) { $provide.provider('MathService', function() { this.$get = function() { var factory = {}; factory.multiply = function(a, b) { return a * b; } return factory; }; }); });
定数
定数は、定数を構成することで構成フェーズ中に値を渡すことができないこと、および構成フェーズ中に値を渡すことができないという事実を説明するために使用されます。
mainApp.constant("configParam", "constant value");
例
次の例は、上記のすべてのコマンドを示しています。
testAngularJS.html
<html> <head> <title>AngularJS Dependency Injection</title> </head> <body> <h2>AngularJS Sample Application</h2> <div ng-app="mainApp" ng-controller="CalcController"> <p>Enter a number: <input type="number" ng-model="number" /> <button ng-click="square()">X<sup>2</sup></button> <p>Result: {{result}}</p> </div> <script src="http://ajax.googleapis.com/ajax/libs/angularjs/1.2.15/angular.min.js"></script> <script> var mainApp = angular.module("mainApp", []); mainApp.config(function($provide) { $provide.provider('MathService', function() { this.$get = function() { var factory = {}; factory.multiply = function(a, b) { return a * b; } return factory; }; }); }); mainApp.value("defaultInput", 5); mainApp.factory('MathService', function() { var factory = {}; factory.multiply = function(a, b) { return a * b; } return factory; }); mainApp.service('CalcService', function(MathService){ this.square = function(a) { return MathService.multiply(a,a); } }); mainApp.controller('CalcController', function($scope, CalcService, defaultInput) { $scope.number = defaultInput; $scope.result = CalcService.square($scope.number); $scope.square = function() { $scope.result = CalcService.square($scope.number); } }); </script> </body> </html>
結果
Web ブラウザで textAngularJS.html を開きます。以下の結果をご覧ください。

ホットAIツール

Undresser.AI Undress
リアルなヌード写真を作成する AI 搭載アプリ

AI Clothes Remover
写真から衣服を削除するオンライン AI ツール。

Undress AI Tool
脱衣画像を無料で

Clothoff.io
AI衣類リムーバー

AI Hentai Generator
AIヘンタイを無料で生成します。

人気の記事

ホットツール

メモ帳++7.3.1
使いやすく無料のコードエディター

SublimeText3 中国語版
中国語版、とても使いやすい

ゼンドスタジオ 13.0.1
強力な PHP 統合開発環境

ドリームウィーバー CS6
ビジュアル Web 開発ツール

SublimeText3 Mac版
神レベルのコード編集ソフト(SublimeText3)

ホットトピック








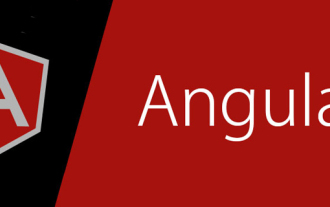
この記事では、依存性注入について説明し、依存性注入によって解決される問題とそのネイティブの記述方法を紹介し、Angular の依存性注入フレームワークについて説明します。
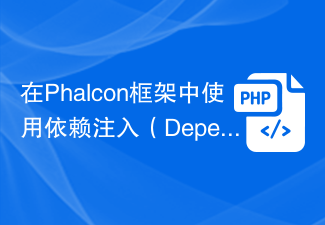
Phalcon フレームワークで依存関係注入 (DependencyInjection) を使用する方法の紹介: 現代のソフトウェア開発では、依存関係注入 (DependencyInjection) は、コードの保守性とテスト容易性を向上させることを目的とした一般的な設計パターンです。高速かつ低コストの PHP フレームワークである Phalcon フレームワークは、アプリケーションの依存関係を管理および整理するための依存関係注入の使用もサポートしています。この記事では、Phalcon フレームワークの使用方法を紹介します。

Javascript は、コードの構成、コードのプログラミング パラダイム、およびオブジェクト指向理論の点で非常にユニークな言語です。しかし、JavaScript が 20 年間主流であったとはいえ、jQuery、Angularjs、さらには React などの人気のあるフレームワークを理解したい場合は、「Black Horse Cloud Classroom JavaScript Advanced Framework」を見てください。デザインビデオチュートリアル」。
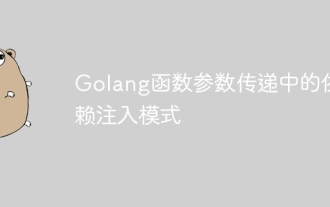
Go では、依存関係注入 (DI) モードは、値の受け渡しやポインターの受け渡しなど、関数パラメーターの受け渡しを通じて実装されます。 DI パターンでは、依存関係は通常、分離を改善し、ロック競合を軽減し、テスト容易性をサポートするためにポインターとして渡されます。ポインターを使用すると、関数はインターフェイスの種類にのみ依存するため、具体的な実装から切り離されます。また、ポインターの受け渡しにより、大きなオブジェクトを渡す際のオーバーヘッドが削減されるため、ロックの競合が軽減されます。さらに、DI パターンでは依存関係を簡単にモックできるため、DI パターンを使用した関数の単体テストを簡単に作成できます。
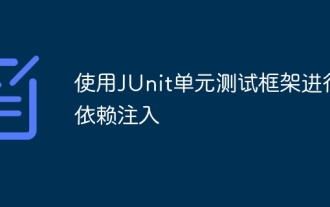
JUnit を使用した依存関係注入のテストの概要は次のとおりです。 モック オブジェクトを使用して依存関係を作成します。 @Mock アノテーションを使用して、依存関係のモック オブジェクトを作成できます。テスト データの設定: @Before メソッドは各テスト メソッドの前に実行され、テスト データの設定に使用されます。モックの動作を構成する: Mockito.when() メソッドは、モック オブジェクトの予期される動作を構成します。結果の検証:assertEquals() は、実際の結果が期待値と一致するかどうかを確認するためにアサートします。実際の応用: 依存関係注入フレームワーク (Spring Framework など) を使用して依存関係を注入し、JUnit 単体テストを通じて注入の正確さとコードの正常な動作を検証できます。
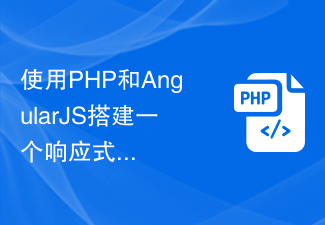
今日の情報化時代において、ウェブサイトは人々が情報を入手し、コミュニケーションを図るための重要なツールとなっています。レスポンシブな Web サイトはさまざまなデバイスに適応し、ユーザーに高品質のエクスペリエンスを提供できます。これは、現代の Web サイト開発のホットスポットとなっています。この記事では、PHP と AngularJS を使用して応答性の高い Web サイトを構築し、高品質のユーザー エクスペリエンスを提供する方法を紹介します。 PHP の概要 PHP は、Web 開発に最適なオープンソースのサーバー側プログラミング言語です。 PHP には、学びやすさ、クロスプラットフォーム、豊富なツール ライブラリ、開発効率など、多くの利点があります。
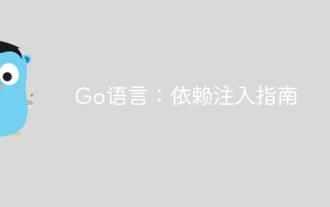
回答: Go 言語では、インターフェイスと構造を通じて依存関係の注入を実装できます。依存関係の動作を記述するインターフェイスを定義します。このインターフェースを実装する構造体を作成します。インターフェイスを介して関数のパラメーターとして依存関係を注入します。テストやさまざまなシナリオで依存関係を簡単に置き換えることができます。
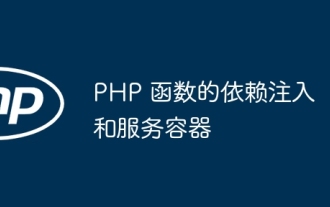
回答: PHP の依存関係インジェクションとサービス コンテナーは、依存関係を柔軟に管理し、コードのテスト容易性を向上させるのに役立ちます。依存関係の注入: 依存関係をコンテナー経由で渡し、関数内での直接作成を回避し、柔軟性を向上させます。サービスコンテナ: プログラム内で簡単にアクセスできるように依存関係インスタンスを保存し、疎結合をさらに強化します。実際のケース: サンプル アプリケーションは、依存関係の注入とサービス コンテナーの実際のアプリケーションを示し、疎結合の利点を反映してコントローラーに依存関係を注入します。
