Go 言語の一般的なアプリケーション シナリオは何ですか?
Go 言語は、バックエンド開発、マイクロサービス アーキテクチャ、クラウド コンピューティング、ビッグ データ処理、機械学習、RESTful API の構築など、さまざまなシナリオに適しています。その中で、Go を使用して RESTful API を構築する簡単な手順には、ルーターのセットアップ、処理関数の定義、データの取得と JSON へのエンコード、応答の書き込みが含まれます。
Go 言語の一般的なアプリケーション シナリオ
Go 言語は、同時実行性と効率性を備えた多機能プログラミング言語です。プラットフォームの機能により、さまざまなアプリケーション シナリオを活用できるようになります。
#バックエンド開発
- RESTful API 開発
- マイクロサービス アーキテクチャ
- 分散システム
##クラウド コンピューティング
- クラウド サービス (AWS、GCP、Azure など) のためのツールとインフラストラクチャの開発
- サーバーレス アーキテクチャ
- 云機能
ビッグデータの処理と分析
- ストリーミング データ処理とパイプライン
- 機械学習および人工知能
コマンド ライン ツール
- システム管理および自動化スクリプト
- テストおよび品質保証ツール
Web アプリケーション ファイアウォール
- 侵入検知および防御システム
- 分散型サービス拒否 (DDoS) 保護
Go を使用して RESTful API を構築する方法は次のとおりです。簡単な実践例:
package main import ( "fmt" "log" "net/http" "github.com/gorilla/mux" ) func main() { router := mux.NewRouter() router.HandleFunc("/", HomeHandler).Methods("GET") router.HandleFunc("/users", UsersHandler).Methods("GET") router.HandleFunc("/users/{id}", UserHandler).Methods("GET") fmt.Println("Starting server on port 8080") log.Fatal(http.ListenAndServe(":8080", router)) } func HomeHandler(w http.ResponseWriter, r *http.Request) { fmt.Fprint(w, "Hello, world!") } func UsersHandler(w http.ResponseWriter, r *http.Request) { // Get all users from the database users := []User{ {ID: 1, Name: "Alice"}, {ID: 2, Name: "Bob"}, {ID: 3, Name: "Charlie"}, } // Encode the users into JSON and write it to the response if err := json.NewEncoder(w).Encode(users); err != nil { http.Error(w, "Error encoding users", http.StatusInternalServerError) } } func UserHandler(w http.ResponseWriter, r *http.Request) { // Get the user ID from the request id := mux.Vars(r)["id"] // Get the user from the database user, err := GetUserByID(id) if err != nil { http.Error(w, "No user found with that ID", http.StatusNotFound) return } // Encode the user into JSON and write it to the response if err := json.NewEncoder(w).Encode(user); err != nil { http.Error(w, "Error encoding user", http.StatusInternalServerError) } } type User struct { ID int `json:"id"` Name string `json:"name"` } func GetUserByID(id string) (*User, error) { // This function is a placeholder for a more complex implementation that // would retrieve a user by ID from a database. user := &User{ ID: 1, Name: "Alice", } return user, nil }
以上がGo 言語の一般的なアプリケーション シナリオは何ですか?の詳細内容です。詳細については、PHP 中国語 Web サイトの他の関連記事を参照してください。

ホットAIツール

Undresser.AI Undress
リアルなヌード写真を作成する AI 搭載アプリ

AI Clothes Remover
写真から衣服を削除するオンライン AI ツール。

Undress AI Tool
脱衣画像を無料で

Clothoff.io
AI衣類リムーバー

AI Hentai Generator
AIヘンタイを無料で生成します。

人気の記事

ホットツール

メモ帳++7.3.1
使いやすく無料のコードエディター

SublimeText3 中国語版
中国語版、とても使いやすい

ゼンドスタジオ 13.0.1
強力な PHP 統合開発環境

ドリームウィーバー CS6
ビジュアル Web 開発ツール

SublimeText3 Mac版
神レベルのコード編集ソフト(SublimeText3)

ホットトピック








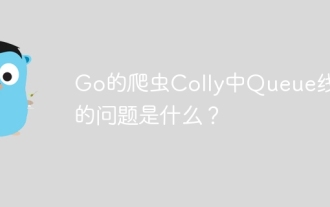
Go Crawler Collyのキュースレッドの問題は、Go言語でColly Crawler Libraryを使用する問題を調査します。 �...
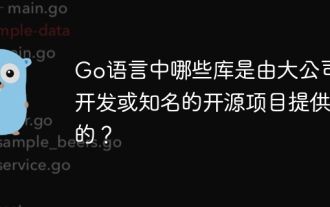
大企業または有名なオープンソースプロジェクトによって開発されたGOのどのライブラリが開発されていますか? GOでプログラミングするとき、開発者はしばしばいくつかの一般的なニーズに遭遇します...
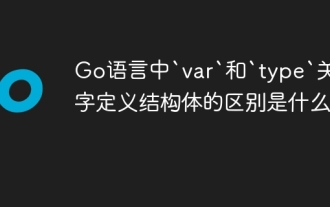
GO言語で構造を定義する2つの方法:VARとタイプのキーワードの違い。構造を定義するとき、GO言語はしばしば2つの異なる執筆方法を見ます:最初...
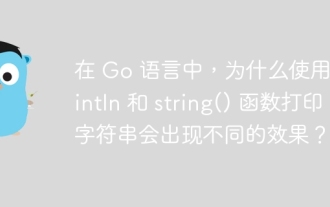
Go言語での文字列印刷の違い:printlnとstring()関数を使用する効果の違いはGOにあります...
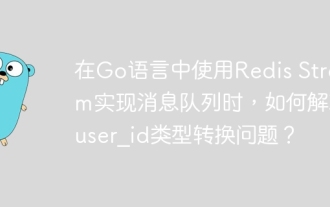
redisstreamを使用してGo言語でメッセージキューを実装する問題は、GO言語とRedisを使用することです...
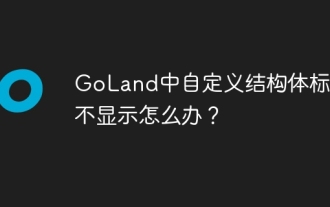
Golandのカスタム構造ラベルが表示されない場合はどうすればよいですか?ゴーランドを使用するためにGolandを使用する場合、多くの開発者はカスタム構造タグに遭遇します...
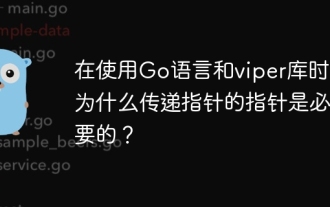
ポインター構文とviperライブラリの使用における問題への取り組みGO言語でプログラミングするとき、特にポインターの構文と使用を理解することが重要です...
