Java マルチスレッド プログラミングの面接における重要な知識ポイント
Java マルチスレッド プログラミングには、同時実行を可能にするスレッドの作成と管理が含まれます。スレッド、同期、スレッド プールの基本概念、および実際の例について説明します。スレッドは、メモリ空間を共有し、同時実行を可能にする軽量のプロセスです。同期は、ロックまたはアトミック操作を通じて共有リソースへのアクセスを保護します。スレッド プールはスレッドを管理し、パフォーマンスを向上させ、作成と破棄のオーバーヘッドを削減します。実際の例では、マルチスレッドを使用してディレクトリ内のファイルを並行してスキャンします。
#Java マルチスレッド プログラミングのインタビューにおける重要な知識ポイント
1. スレッドの基本概念
- スレッドはオペレーティング システムの軽量プロセスであり、プロセスと同じメモリ空間を共有します。
- スレッドを使用すると、複数の独立したタスクを同じプログラム内で同時に実行できます。
コード例:
class MyThread extends Thread { public void run() { System.out.println("This is a thread"); } } public class Main { public static void main(String[] args) { MyThread thread = new MyThread(); thread.start(); } }
2. スレッド同期
- スレッド同期により、アクセス時に次のことが保証されます。リソースを共有するときにデータ競合を回避します。 同期は、ロック メカニズムまたはアトミック操作を使用して実現できます。
コード例 (同期を使用):
class Counter { private int count; public synchronized void increment() { count++; } public synchronized int getCount() { return count; } } public class Main { public static void main(String[] args) { Counter counter = new Counter(); Thread thread1 = new Thread(() -> { for (int i = 0; i < 10000; i++) { counter.increment(); } }); Thread thread2 = new Thread(() -> { for (int i = 0; i < 10000; i++) { counter.increment(); } }); thread1.start(); thread2.start(); thread1.join(); thread2.join(); System.out.println(counter.getCount()); // 输出:20000 } }
3. スレッド プール
- スレッド プールスレッドを管理するリソースのセットです。 これにより、パフォーマンスが向上し、スレッドの作成と破棄のオーバーヘッドが軽減されます。
ExecutorService executor = Executors.newFixedThreadPool(5);
for (int i = 0; i < 10; i++) {
executor.submit(() -> {
System.out.println("This is a thread from the pool");
});
}
executor.shutdown();
複数のスレッドを使用して、大きなディレクトリ内のファイルを並行してスキャンします。
import java.io.File;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
public class FileScanner {
private static void scan(File dir) {
File[] files = dir.listFiles();
if (files == null)
return;
ExecutorService executor = Executors.newFixedThreadPool(Runtime.getRuntime().availableProcessors());
for (File f : files) {
executor.submit(() -> {
if (f.isDirectory())
scan(f);
else
System.out.println(f.getAbsolutePath());
});
}
executor.shutdown();
}
public static void main(String[] args) {
File root = new File("..."); // 替换为要扫描的目录
scan(root);
}
}
以上がJava マルチスレッド プログラミングの面接における重要な知識ポイントの詳細内容です。詳細については、PHP 中国語 Web サイトの他の関連記事を参照してください。

ホットAIツール

Undresser.AI Undress
リアルなヌード写真を作成する AI 搭載アプリ

AI Clothes Remover
写真から衣服を削除するオンライン AI ツール。

Undress AI Tool
脱衣画像を無料で

Clothoff.io
AI衣類リムーバー

Video Face Swap
完全無料の AI 顔交換ツールを使用して、あらゆるビデオの顔を簡単に交換できます。

人気の記事

ホットツール

メモ帳++7.3.1
使いやすく無料のコードエディター

SublimeText3 中国語版
中国語版、とても使いやすい

ゼンドスタジオ 13.0.1
強力な PHP 統合開発環境

ドリームウィーバー CS6
ビジュアル Web 開発ツール

SublimeText3 Mac版
神レベルのコード編集ソフト(SublimeText3)

ホットトピック








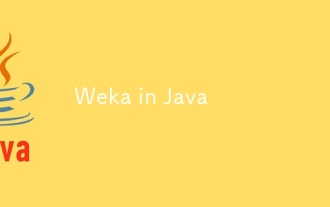
Java の Weka へのガイド。ここでは、weka java の概要、使い方、プラットフォームの種類、利点について例を交えて説明します。

この記事では、Java Spring の面接で最もよく聞かれる質問とその詳細な回答をまとめました。面接を突破できるように。
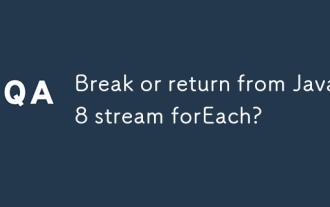
Java 8は、Stream APIを導入し、データ収集を処理する強力で表現力のある方法を提供します。ただし、ストリームを使用する際の一般的な質問は次のとおりです。 従来のループにより、早期の中断やリターンが可能になりますが、StreamのForeachメソッドはこの方法を直接サポートしていません。この記事では、理由を説明し、ストリーム処理システムに早期終了を実装するための代替方法を調査します。 さらに読み取り:JavaストリームAPIの改善 ストリームを理解してください Foreachメソッドは、ストリーム内の各要素で1つの操作を実行する端末操作です。その設計意図はです
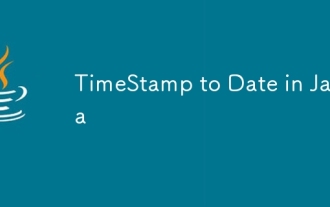
Java での日付までのタイムスタンプに関するガイド。ここでは、Java でタイムスタンプを日付に変換する方法とその概要について、例とともに説明します。
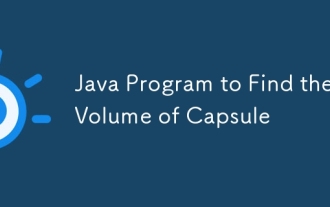
カプセルは3次元の幾何学的図形で、両端にシリンダーと半球で構成されています。カプセルの体積は、シリンダーの体積と両端に半球の体積を追加することで計算できます。このチュートリアルでは、さまざまな方法を使用して、Javaの特定のカプセルの体積を計算する方法について説明します。 カプセルボリュームフォーミュラ カプセルボリュームの式は次のとおりです。 カプセル体積=円筒形の体積2つの半球体積 で、 R:半球の半径。 H:シリンダーの高さ(半球を除く)。 例1 入力 RADIUS = 5ユニット 高さ= 10単位 出力 ボリューム= 1570.8立方ユニット 説明する 式を使用してボリュームを計算します。 ボリューム=π×R2×H(4
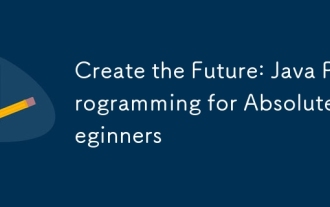
Java は、初心者と経験豊富な開発者の両方が学習できる人気のあるプログラミング言語です。このチュートリアルは基本的な概念から始まり、高度なトピックに進みます。 Java Development Kit をインストールしたら、簡単な「Hello, World!」プログラムを作成してプログラミングを練習できます。コードを理解したら、コマンド プロンプトを使用してプログラムをコンパイルして実行すると、コンソールに「Hello, World!」と出力されます。 Java の学習はプログラミングの旅の始まりであり、習熟が深まるにつれて、より複雑なアプリケーションを作成できるようになります。
