mysql操作类库1.2
又经过了一次小的改动,现把最新版发出来: ?php/** +---------------------------------- * MySQL操作类库 +---------------------------------- * @author 马犇 +---------------------------------- * @version 1.2 (2013-5-31) +-----------------------
又经过了一次小的改动,现把最新版发出来:
<?php /** +---------------------------------- * MySQL操作类库 +---------------------------------- * @author 马犇 +---------------------------------- * @version 1.2 (2013-5-31) +---------------------------------- */ include (dirname(__FILE__) . DIRECTORY_SEPARATOR . 'config.php'); define('CLIENT_MULTI_RESULTS', 131072); class mysql { /* 主机地址 */ private $Host = '127.0.0.1'; /* 数据库名称 */ private $dbName = DB_NAME; /* 用户名 */ private $UserName = DB_USER; /* 连接密码 */ private $Password = DB_PWD; /* 数据库编码 */ private $dbCharSet = 'utf8'; /* 错误信息 */ private $errorMsg; /* 最后一次执行的SQL */ private $lastSql; /* 字段信息 */ private $fields = array(); /* 最后一次插入的ID */ public $lastInsID = null; /* 数据库连接ID */ private $linkID = 0; /* 当前查询ID */ private $queryID = null; /*主键*/ private $pk = null; public function __construct($DBName = '') { if ($DBName != '') $this->dbName = $DBName; $this->connect(); } /** +---------------------------------------------------------- * 连接数据库方法 +---------------------------------------------------------- * @access public +---------------------------------------------------------- */ public function connect() { if ($this->linkID == 0) { $this->linkID = mysql_connect($this->Host, $this->UserName, $this->Password, true, CLIENT_MULTI_RESULTS); if (!$this->linkID) { $this->errorMsg = '数据库连接错误\r\n' . mysql_error(); $this->halt(); } } if (!mysql_select_db($this->dbName, $this->linkID)) { $this->errorMsg = '打开数据库失败' . mysql_error($this->linkID); $this->halt('打开数据库失败'); } $dbVersion = mysql_get_server_info($this->linkID); if ($dbVersion >= "4.1") { //使用UTF8存取数据库 需要mysql 4.1.0以上支持 mysql_query("SET NAMES '" . $this->dbCharSet . "'", $this->linkID); } //设置CharSet mysql_query('set character set \'' . $this->dbCharSet . '\'', $this->linkID); //设置 sql_model if ($dbVersion > '5.0.1') { mysql_query("SET sql_mode=''", $this->linkID); } } /** +---------------------------------------------------------- * 释放查询结果 +---------------------------------------------------------- * @access public +---------------------------------------------------------- */ public function free() { if($this->queryID != null) mysql_free_result($this->queryID); $this->queryID = null; } /** +---------------------------------------------------------- * 执行语句 +---------------------------------------------------------- * @access public +---------------------------------------------------------- * @param string $sql sql指令 +---------------------------------------------------------- * @return bool or resource +---------------------------------------------------------- */ public function execute($sql) { if ($this->linkID == 0) $this->connect(); $this->lastSql = $sql; $this->queryID = mysql_query($sql); if (false == $this->queryID) { $this->errorMsg = 'SQL语句执行失败\r\n' . mysql_error($this->linkID); return false; } else { return $this->queryID; } } /** +---------------------------------------------------------- * 获取记录集的行数 +---------------------------------------------------------- * @access public +---------------------------------------------------------- * @param string $sql sql指令 可为空 * 如为空:返回上一结果集记录数 * 如不为空:返回当前sql语句的记录数 +---------------------------------------------------------- * @return integer +---------------------------------------------------------- */ public function getRowsNum($sql = '') { if ($this->linkID == 0) { $this->connect(); } if ($sql != '') { $this->execute($sql); } return mysql_num_rows($this->queryID); } /** +---------------------------------------------------------- * 表单数据直接插入到数据表中 +---------------------------------------------------------- * @access public +---------------------------------------------------------- * @param string $tableName 数据表名 +---------------------------------------------------------- * @return 执行成功返回插入记录的索引记录,失败返回false +---------------------------------------------------------- */ public function form2db($tableName) { $_POST["add_time"] = date('Y-m-d H:i:s'); $data = $_POST; $this->fields = $this->getFields($tableName); $data = $this->_facade($data); if ($this->insert($tableName, $data)) { return $this->lastInsID; } else { return false; } } /** +---------------------------------------------------------- * 数据直接插入到数据表中 +---------------------------------------------------------- * @access public +---------------------------------------------------------- * @param string $tableName 数据表名 +---------------------------------------------------------- * @param array $data 插入的数据 数据键名对应字段名,键值对应值 +---------------------------------------------------------- * @return boolean +---------------------------------------------------------- */ public function insert($tableName, $data) { $values = $fields = array(); foreach ($data as $key => $val) { $value = '\'' . addslashes($val) . '\''; if (is_scalar($value)) { // 过滤非标量数据 $values[] = $value; $fields[] = $key; } } $sql = 'INSERT INTO ' . trim($tableName) . '(' . implode(',', $fields) . ') VALUES(' . implode(',', $values) . ')'; if ($this->execute($sql)) { $this->errorMsg = '插入失败\r\n' . mysql_error($this->linkID); $this->lastInsID = mysql_insert_id($this->linkID); return true; } else { return false; } } /** +---------------------------------------------------------- * 更新操作 +---------------------------------------------------------- * @access public +---------------------------------------------------------- * @param string $tableName 数据表名 +---------------------------------------------------------- * @param array $data 插入的数据 数据键名对应字段名,键值对应值 +---------------------------------------------------------- * @param array $condition 更新条件,为安全起见,不能为空 +---------------------------------------------------------- * @param array $isForm 可为空,缺省为true * 如果为true,会当成表单更新数据表来处理,自动映射字段 * 如果为false,会当成普通的更新来处理,不会自动映射字段 +---------------------------------------------------------- * @return boolean +---------------------------------------------------------- */ public function update($tableName, $data, $condition, $isForm = true) { if (empty($condition)) { $this->errorMsg = '没有设置更新条件'; return false; } //处理分解condition if(is_array($condition)){ $condition = self::_parseCondition($condition); } if ($isForm) { $this->fields = $this->getFields($tableName); $data = $this->_facade($data); } $sql = 'UPDATE ' . trim($tableName) . ' SET '; foreach ($data as $key => $val) { $sql .= $key . '=\'' . $val . '\','; } $sql = substr($sql, 0, strlen($sql) - 1); $sql .= ' WHERE ' . $condition; if ($this->execute($sql)) { return true; } else { $this->errorMsg = '更新失败\r\n' . mysql_error($this->linkID); return false; } } /** +---------------------------------------------------------- * 删除操作 +---------------------------------------------------------- * @access public +---------------------------------------------------------- * @param string $tableName 数据表名 +---------------------------------------------------------- * @param array $condition 更新条件,为安全起见,不能为空 +---------------------------------------------------------- * @return boolean +---------------------------------------------------------- */ public function delete($tableName, $condition) { //处理分解condition if(is_array($condition)){ $condition = self::_parseCondition($condition); } $sql = 'delete from ' . $tableName . ' where 1=1 and ' . $condition; if (!$this->execute($sql)) return false; return true; } /** +---------------------------------------------------------- * 利用__call魔术方法实现一些特殊的Model方法 +---------------------------------------------------------- * @access public +---------------------------------------------------------- * @param string $method 方法名称 * @param array $args 调用参数 +---------------------------------------------------------- * @return mixed +---------------------------------------------------------- */ public function __call($method,$args){ /*根据某个字段获取记录字段的值 * 例1:getFieldByid(student_info,100,name)---获取学生表中id为100的学生姓名 * 例2:getFieldByxh(student_info,201215030223,address)---获取学生表中学号为201015030223的地址 * 注:"getFieldBy"不区分大小写,后面的字段名区分大小写 * 返回值:string */ if(strtolower(substr($method,0,10)) == 'getfieldby'){ $name = substr($method,10); $sql = 'select `'.$args[2].'` from '.$args[0].' where '.$name.'=\''.$args[1].'\''; if($this->execute($sql)){ $row = mysql_fetch_array($this->queryID); return $row[0]; }else{ return false; } } /*根据某个字段和值获取某条记录 * 例1:getByid(student_info,100)---获取学生表中id为100的学生信息 * 例2:getByxh(student_info,201215030223)---获取学生表中学号为201015030223的学生信息 * 注:"getBy"不区分大小写,后面的字段名区分大小写 * 返回值:array */ elseif(strtolower(substr($method,0,5)) == 'getby'){ $ret = array(); $name = substr($method,5); $sql = 'select * from '.$args[0].' where '.$name.'=\''.$args[1].'\''; if($this->execute($sql)){ $row = mysql_fetch_array($this->queryID); return $row; }else{ return false; } } } /** +---------------------------------------------------------- * 弹出错误提示,并终止运行 +---------------------------------------------------------- * @access public +---------------------------------------------------------- * @param string $msg 错误消息,可为空 +---------------------------------------------------------- */ public static function halt($msg = '') { if ($msg != '') { $msg .= '\r\n'; } $error = mysql_error(); die($msg); } /** +---------------------------------------------------------- * 获取最后一次查询ID +---------------------------------------------------------- * @access public +---------------------------------------------------------- */ public function getQueryId(){ return $this->queryID; } /** +---------------------------------------------------------- * 获取最后一次数据库操作错误信息 +---------------------------------------------------------- * @access public +---------------------------------------------------------- */ public function getLastError() { return $this->errorMsg; } /** +---------------------------------------------------------- * 获取最后一次执行的SQL语句 +---------------------------------------------------------- * @access public +---------------------------------------------------------- */ public function getLastSql() { return $this->lastSql; } /** +---------------------------------------------------------- * 获取最后一次插入数据库记录的索引ID号 +---------------------------------------------------------- * @access public +---------------------------------------------------------- */ public function getLastInsID() { return $this->lastInsID; } /** +---------------------------------------------------------- * 获取上一次操作影响的行数 +---------------------------------------------------------- * @access public +---------------------------------------------------------- */ public function getAffectedRows() { return mysql_affected_rows($this->linkID); } /** +---------------------------------------------------------- * 取得数据表的字段信息 +---------------------------------------------------------- * @access public +---------------------------------------------------------- */ public function getFields($tableName) { $result = array(); $this->execute('SHOW COLUMNS FROM ' . $this->parseKey($tableName)); while ($row = mysql_fetch_array($this->queryID)) { $result[] = $row; } $info = array(); if ($result) { foreach ($result as $key => $val) { $info[$val['Field']] = array( 'name' => $val['Field'], 'type' => $val['Type'], 'notnull' => (bool) ($val['Null'] === ''), // not null is empty, null is yes 'default' => $val['Default'], 'primary' => (strtolower($val['Key']) == 'pri'), 'autoinc' => (strtolower($val['Extra']) == 'auto_increment'), ); } } return $info; } /** +---------------------------------------------------------- * 字段和表名处理添加` +---------------------------------------------------------- * @access protected +---------------------------------------------------------- * @param string $key +---------------------------------------------------------- * @return string +---------------------------------------------------------- */ protected function parseKey(&$key) { $key = trim($key); if (false !== strpos($key, ' ') || false !== strpos($key, ',') || false !== strpos($key, '*') || false !== strpos($key, '(') || false !== strpos($key, '.') || false !== strpos($key, '`')) { //如果包含* 或者 使用了sql方法 则不作处理 } else { $key = '`' . $key . '`'; } return $key; } /** +---------------------------------------------------------- * 对保存到数据库的数据进行处理 +---------------------------------------------------------- * @access protected +---------------------------------------------------------- * @param mixed $data 要操作的数据 +---------------------------------------------------------- * @return boolean +---------------------------------------------------------- */ private function _facade($data) { // 检查非数据字段 if (!empty($this->fields)) { foreach ($data as $key => $val) { if (!array_key_exists($key, $this->fields)) { unset($data[$key]); } } } return $data; } public function close(){ mysql_close($this->linkID); } public function __destruct(){ $this->close(); } /* ** 2013.5.25新增 */ public function getPk($table){ //将pk置为空 $this->pk = null; $result = $this->getFields($table); foreach($result as $key => $val){ if($val['primary']){ $this->pk = $key; break; } } return $this->pk; } public function fetch(&$rst = null , $array_type = MYSQL_ASSOC){ if($rst == null){ $rst = $this->queryID; } if($this->queryID) return mysql_fetch_array($rst , $array_type); else return false; } //分解条件 private function _parseCondition($condition , $operator='AND'){ $return = ''; if (is_array($condition)) { $index = 0; foreach ($condition as $key => $value) { if ($index) { $return .= " ".$operator; } $return .= "`{$key}`='{$value}'"; $index++; } return $return; }else{ return false; } } /*事务处理开始*/ public function beginTransaction(){ $this->execute("START TRANSACTION"); } public function commit(){ $this->execute("COMMIT"); } public function rollback(){ $this->execute("ROLLBACK"); } /*事务处理结束*/ //根据条件查找一条记录 public function find($table,$condition = null,$field = null){ if(is_array($condition)){ $condition = self::_parseCondition($condition); } //处理condition和field $condition = $condition == null ? null : (is_array($condition) ? self::_parseCondition($condition) : $condition); $field = $field == null ? '*' : (is_array($field) ? implode(",",$field) : $field); $sql = 'SELECT ' . $field . ' FROM '.$table; if($condition != null){ $sql .= " WHERE " . $condition; } return $this->findOneBySql($sql); } //查找所有记录 public function findAll($table,$condition = null,$field = null){ if(is_array($condition)){ $condition = self::_parseCondition($condition); } //处理condition和field $condition = $condition == null ? null : (is_array($condition) ? self::_parseCondition($condition) : $condition); $field = $field == null ? '*' : (is_array($field) ? implode(",",$field) : $field); $sql = 'SELECT ' . $field . ' FROM '.$table; if($condition != null){ $sql .= " WHERE " . $condition; } return $this->findallBySql($sql); } public function findOneBySql($sql){ $sql .= " LIMIT 1"; $this->execute($sql); return $this->fetch(); } public function findAllBySql($sql){ $rows = array(); $this->execute($sql); while($row = $this->fetch()){ $rows[] = $row; } return $rows; } public function findByPk($table,$_pk){ $pk = $this->getPk($table); if($pk == null){ $this->errorMsg = "未找到该表的主键"; return false; }else{ return $this->find($table,array( $pk => $_pk )); } } public function deleteByPk($table,$_pk){ $pk = $this->getPk($table); if($pk == null){ $this->errorMsg = "未找到该表的主键"; return false; }else{ $sql = "DELETE FROM ".$table." WHERE `{$pk}`='{$_pk}'"; return $this->delete($table,array( $pk => $_pk )); } } } /* * 类库更新日志 2013.5.25 * 1、update delete操作中的条件可以设置为数组形式$key=>$value * 2、增加事务处理功能(只针对innodb引擎) * 3、增加根据条件查找一条记录 * 4、增加根据条件查找所有记录 * 5、增加根据主键查找记录 * 6、增加根据主键删除记录 */ ?>
原文地址:mysql操作类库1.2, 感谢原作者分享。

ホットAIツール

Undresser.AI Undress
リアルなヌード写真を作成する AI 搭載アプリ

AI Clothes Remover
写真から衣服を削除するオンライン AI ツール。

Undress AI Tool
脱衣画像を無料で

Clothoff.io
AI衣類リムーバー

AI Hentai Generator
AIヘンタイを無料で生成します。

人気の記事

ホットツール

メモ帳++7.3.1
使いやすく無料のコードエディター

SublimeText3 中国語版
中国語版、とても使いやすい

ゼンドスタジオ 13.0.1
強力な PHP 統合開発環境

ドリームウィーバー CS6
ビジュアル Web 開発ツール

SublimeText3 Mac版
神レベルのコード編集ソフト(SublimeText3)

ホットトピック










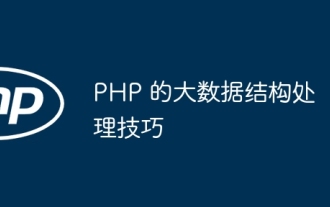
ビッグ データ構造の処理スキル: チャンキング: データ セットを分割してチャンクに処理し、メモリ消費を削減します。ジェネレーター: データ セット全体をロードせずにデータ項目を 1 つずつ生成します。無制限のデータ セットに適しています。ストリーミング: ファイルやクエリ結果を 1 行ずつ読み取ります。大きなファイルやリモート データに適しています。外部ストレージ: 非常に大規模なデータ セットの場合は、データをデータベースまたは NoSQL に保存します。
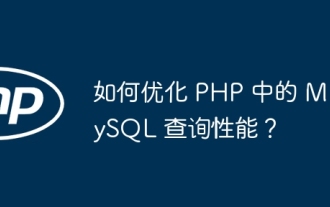
MySQL クエリのパフォーマンスは、検索時間を線形の複雑さから対数の複雑さまで短縮するインデックスを構築することで最適化できます。 PreparedStatement を使用して SQL インジェクションを防止し、クエリのパフォーマンスを向上させます。クエリ結果を制限し、サーバーによって処理されるデータ量を削減します。適切な結合タイプの使用、インデックスの作成、サブクエリの使用の検討など、結合クエリを最適化します。クエリを分析してボトルネックを特定し、キャッシュを使用してデータベースの負荷を軽減し、オーバーヘッドを最小限に抑えます。
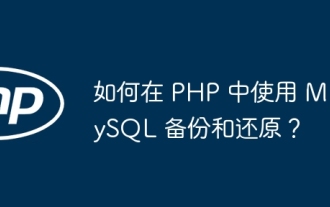
PHP で MySQL データベースをバックアップおよび復元するには、次の手順を実行します。 データベースをバックアップします。 mysqldump コマンドを使用して、データベースを SQL ファイルにダンプします。データベースの復元: mysql コマンドを使用して、SQL ファイルからデータベースを復元します。
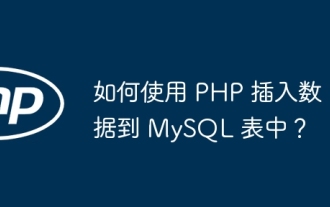
MySQLテーブルにデータを挿入するにはどうすればよいですか?データベースに接続する: mysqli を使用してデータベースへの接続を確立します。 SQL クエリを準備します。挿入する列と値を指定する INSERT ステートメントを作成します。クエリの実行: query() メソッドを使用して挿入クエリを実行します。成功すると、確認メッセージが出力されます。

MySQL 8.4 (2024 年時点の最新の LTS リリース) で導入された主な変更の 1 つは、「MySQL Native Password」プラグインがデフォルトで有効ではなくなったことです。さらに、MySQL 9.0 ではこのプラグインが完全に削除されています。 この変更は PHP および他のアプリに影響します
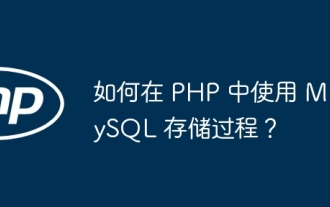
PHP で MySQL ストアド プロシージャを使用するには: PDO または MySQLi 拡張機能を使用して、MySQL データベースに接続します。ストアド プロシージャを呼び出すステートメントを準備します。ストアド プロシージャを実行します。結果セットを処理します (ストアド プロシージャが結果を返す場合)。データベース接続を閉じます。

PHP を使用して MySQL テーブルを作成するには、次の手順が必要です。 データベースに接続します。データベースが存在しない場合は作成します。データベースを選択します。テーブルを作成します。クエリを実行します。接続を閉じます。
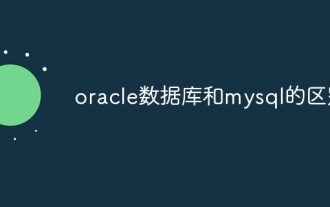
Oracle データベースと MySQL はどちらもリレーショナル モデルに基づいたデータベースですが、Oracle は互換性、スケーラビリティ、データ型、セキュリティの点で優れており、MySQL は速度と柔軟性に重点を置いており、小規模から中規模のデータ セットに適しています。 ① Oracle は幅広いデータ型を提供し、② 高度なセキュリティ機能を提供し、③ エンタープライズレベルのアプリケーションに適しています。① MySQL は NoSQL データ型をサポートし、② セキュリティ対策が少なく、③ 小規模から中規模のアプリケーションに適しています。
