Detailed explanation of sample code for Map in Java collection
interface , and the content stored in Map is a key-value pair (key-value)
(2) AbstractMap isInherited from Map's abstract class, which implements most of the API in Map.
(3) SortedMap is an interface inherited from Map. The content in SortedMap is sorted key-value pairs, and the sorting method is through a comparator. (4) NavigableMap inherits from SortedMap, which has a series of navigation methods, such as "get key-value pairs greater than or equal to a certainobject" etc.
(5 ) TreeMap inherits from the AbstractMap and NavigableMap interfaces, so the contents in TreeMap are ordered key-value pairs. (6) HashMap inherits from AbstractMap, and the content is also a key-value pair, but the order is not guaranteed. (7) WeakHashMap inherits from AbstractMap, and its key type is different from HashMap. WeakHashMap is a weak key. (8) HashTable inherits from Summary: HashMap is a hash table based on the "zipper method". It is generally used in single threads. The key values can be empty and supports Iterator (iterator) traversalHashtable is a hash table implemented based on the "zipper method". It is thread-safe and can be used in multi-threaded programs. Supports two traversal methods: Iterator traversal and Enumeration. WeakHashMap is also a hash table based on the "zipper method" and is also a weak key. TreeMap is an ordered hash table, implemented through a red-black tree, and the key values cannot be empty. .<p>public interface Map<K,V> {<br> int size();//数目<br> boolean isEmpty();//判断是否为空<br> boolean containsKey(<a href="http://www.php.cn/wiki/60.html" target="_blank">Object</a> key);//判断是否含有某个key<br> boolean containsValue(Object value);//判断是否含有某个值<br> V get(Object key);//通过key获得value<br> V put(K key, V value);//插入键值对<br> V remove(Object key);//通过key<a href="http://www.php.cn/php/php-tp-remove.html" target="_blank">删除</a><br> void put<a href="http://www.php.cn/wiki/1483.html" target="_blank">All</a>(Map<? <a href="http://www.php.cn/wiki/166.html" target="_blank">extends</a> K, ? extends V> m);//将一个Map插入<br> void <a href="http://www.php.cn/wiki/917.html" target="_blank">clear</a>();//清空<br> <a href="http://www.php.cn/code/8209.html" target="_blank">Set</a><K> keySet();//返回key集合<br> Collection<V> values();//返回value<br> Set<Map.Entry<K, V>> entrySet();//实体集合,Map的改变会影响到它<br> interface Entry<K,V> {<br> K getKey();//获得key<br> V getValue();//获得value<br> V setValue(V value);//设置值<br> boolean equals(Object o);//判断对象是否相等<br> int hashCode();//返回hashCode<br> //比较器,比较两个key<br> public <a href="http://www.php.cn/wiki/188.html" target="_blank">static</a> <K extends Comparable<? <a href="http://www.php.cn/code/8202.html" target="_blank">super</a> K>, V> Comparator<Map.Entry<K,V>> comparingByKey() {<br> <a href="http://www.php.cn/wiki/135.html" target="_blank">return</a> (Comparator<Map.Entry<K, V>> & Serializable)<br> (c1, c2) -> c1.getKey().compareTo(c2.getKey());<br> }<br> //比较两个值<br> public static <K, V extends Comparable<? super V>> Comparator<Map.Entry<K,V>> comparingByValue() {<br> return (Comparator<Map.Entry<K, V>> & Serializable)<br> (c1, c2) -> c1.getValue().compareTo(c2.getValue());<br> }<br> //比较两个key<br> public static <K, V> Comparator<Map.Entry<K, V>> comparingByKey(Comparator<? super K> cmp) {<br> Objects.<a href="http://www.php.cn/wiki/136.html" target="_blank">require</a>Non<a href="http://www.php.cn/wiki/62.html" target="_blank">Null</a>(cmp);<br> return (Comparator<Map.Entry<K, V>> & Serializable)<br> (c1, c2) -> cmp.compare(c1.getKey(), c2.getKey());<br> }<br> //比较两个值<br> public static <K, V> Comparator<Map.Entry<K, V>> comparingByValue(Comparator<? super V> cmp) {<br> Objects.requireNonNull(cmp);<br> return (Comparator<Map.Entry<K, V>> & Serializable)<br> (c1, c2) -> cmp.compare(c1.getValue(), c2.getValue());<br> }<br> }<br> //比较map是否相等<br> boolean equals(Object o);<br> int hashCode();//hashCode<br> default V get<a href="http://www.php.cn/wiki/1360.html" target="_blank">OrD</a>efault(Object key, V defaultValue) {<br> V v;<br> return (((v = get(key)) != null) || containsKey(key))<br> ? v<br> : defaultValue;<br> }<br> default void <a href="http://www.php.cn/wiki/127.html" target="_blank">forEach</a>(BiConsumer<? super K, ? super V> <a href="http://www.php.cn/java/java-Action.html" target="_blank">action</a>) {<br> Objects.requireNonNull(action);<br> for (Map.Entry<K, V> entry : entrySet()) {<br> K k;<br> V v;<br> try {<br> k = entry.getKey();<br> v = entry.getValue();<br> } catch(IllegalState<a href="http://www.php.cn/wiki/265.html" target="_blank">Exception</a> ise) {<br> // this usually means the entry is no longer in the map.<br> throw <a href="http://www.php.cn/wiki/165.html" target="_blank">new</a> Con<a href="http://www.php.cn/wiki/1046.html" target="_blank">current</a>Mod<a href="http://www.php.cn/wiki/109.html" target="_blank">if</a>icationException(ise);<br> }<br> action.accept(k, v);<br> }<br> }<br> default void replaceAll(BiFunction<? super K, ? super V, ? extends V> function) {<br> Objects.requireNonNull(function);<br> for (Map.Entry<K, V> entry : entrySet()) {<br> K k;<br> V v;<br> try {<br> k = entry.getKey();<br> v = entry.getValue();<br> } catch(IllegalStateException ise) {<br> // this usually means the entry is no longer in the map.<br> throw new ConcurrentModificationException(ise);<br> }<br><br> // ise thrown from function is not a cme.<br> v = function.apply(k, v);<br><br> try {<br> entry.setValue(v);<br> } catch(IllegalStateException ise) {<br> // this usually means the entry is no longer in the map.<br> throw new ConcurrentModificationException(ise);<br> }<br> }<br> }<br> default V putIfAbsent(K key, V value) {<br> V v = get(key);<br> if (v == null) {<br> v = put(key, value);<br> }<br><br> return v;<br> }<br> //删除某个key和value对应的对象<br> default boolean remove(Object key, Object value) {<br> Object curValue = get(key);<br> if (!Objects.equals(curValue, value) ||<br> (curValue == null && !containsKey(key))) {<br> return false;<br> }<br> remove(key);<br> return true;<br> }<br> //将某个key和oldValue对应的值替换为newValue<br> default boolean replace(K key, V oldValue, V newValue) {<br> Object curValue = get(key);<br> if (!Objects.equals(curValue, oldValue) ||<br> (curValue == null && !containsKey(key))) {<br> return false;<br> }<br> put(key, newValue);<br> return true;<br> }<br> //替换key的值<br> default V replace(K key, V value) {<br> V curValue;<br> if (((curValue = get(key)) != null) || containsKey(key)) {<br> curValue = put(key, value);<br> }<br> return curValue;<br> }<br> default V computeIfAbsent(K key,<br> Function<? super K, ? extends V> mappingFunction) {<br> Objects.requireNonNull(mappingFunction);<br> V v;<br> if ((v = get(key)) == null) {<br> V newValue;<br> if ((newValue = mappingFunction.apply(key)) != null) {<br> put(key, newValue);<br> return newValue;<br> }<br> }<br><br> return v;<br> }<br> default V computeIfPresent(K key,<br> BiFunction<? super K, ? super V, ? extends V> remappingFunction) {<br> Objects.requireNonNull(remappingFunction);<br> V oldValue;<br> if ((oldValue = get(key)) != null) {<br> V newValue = remappingFunction.apply(key, oldValue);<br> if (newValue != null) {<br> put(key, newValue);<br> return newValue;<br> } <a href="http://www.php.cn/wiki/111.html" target="_blank">else</a> {<br> remove(key);<br> return null;<br> }<br> } else {<br> return null;<br> }<br> }<br> default V compute(K key,<br> BiFunction<? super K, ? super V, ? extends V> remappingFunction) {<br> Objects.requireNonNull(remappingFunction);<br> V oldValue = get(key);<br><br> V newValue = remappingFunction.apply(key, oldValue);<br> if (newValue == null) {<br> // <a href="http://www.php.cn/wiki/1298.html" target="_blank">delete</a> mapping<br> if (oldValue != null || containsKey(key)) {<br> // something to remove<br> remove(key);<br> return null;<br> } else {<br> // nothing to do. Leave things as they were.<br> return null;<br> }<br> } else {<br> // add or replace old mapping<br> put(key, newValue);<br> return newValue;<br> }<br> }<br> default V merge(K key, V value,<br> BiFunction<? super V, ? super V, ? extends V> remappingFunction) {<br> Objects.requireNonNull(remappingFunction);<br> Objects.requireNonNull(value);<br> V oldValue = get(key);<br> V newValue = (oldValue == null) ? value :<br> remappingFunction.apply(oldValue, value);<br> if(newValue == null) {<br> remove(key);<br> } else {<br> put(key, newValue);<br> }<br> return newValue;<br> }<br>}<br></p>
The above is the detailed content of Detailed explanation of sample code for Map in Java collection. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










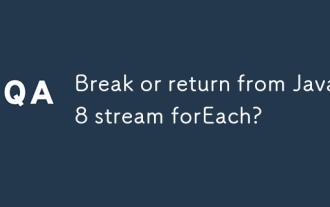
Java 8 introduces the Stream API, providing a powerful and expressive way to process data collections. However, a common question when using Stream is: How to break or return from a forEach operation? Traditional loops allow for early interruption or return, but Stream's forEach method does not directly support this method. This article will explain the reasons and explore alternative methods for implementing premature termination in Stream processing systems. Further reading: Java Stream API improvements Understand Stream forEach The forEach method is a terminal operation that performs one operation on each element in the Stream. Its design intention is
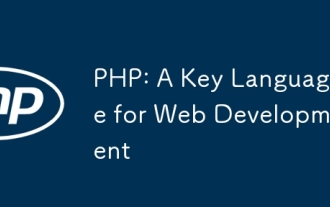
PHP is a scripting language widely used on the server side, especially suitable for web development. 1.PHP can embed HTML, process HTTP requests and responses, and supports a variety of databases. 2.PHP is used to generate dynamic web content, process form data, access databases, etc., with strong community support and open source resources. 3. PHP is an interpreted language, and the execution process includes lexical analysis, grammatical analysis, compilation and execution. 4.PHP can be combined with MySQL for advanced applications such as user registration systems. 5. When debugging PHP, you can use functions such as error_reporting() and var_dump(). 6. Optimize PHP code to use caching mechanisms, optimize database queries and use built-in functions. 7
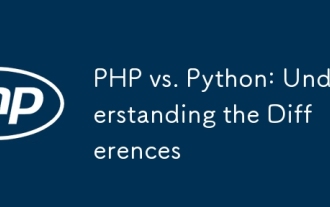
PHP and Python each have their own advantages, and the choice should be based on project requirements. 1.PHP is suitable for web development, with simple syntax and high execution efficiency. 2. Python is suitable for data science and machine learning, with concise syntax and rich libraries.
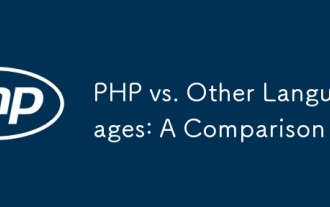
PHP is suitable for web development, especially in rapid development and processing dynamic content, but is not good at data science and enterprise-level applications. Compared with Python, PHP has more advantages in web development, but is not as good as Python in the field of data science; compared with Java, PHP performs worse in enterprise-level applications, but is more flexible in web development; compared with JavaScript, PHP is more concise in back-end development, but is not as good as JavaScript in front-end development.
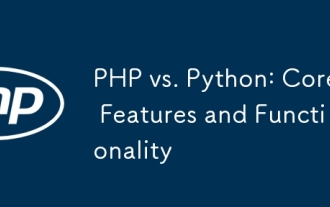
PHP and Python each have their own advantages and are suitable for different scenarios. 1.PHP is suitable for web development and provides built-in web servers and rich function libraries. 2. Python is suitable for data science and machine learning, with concise syntax and a powerful standard library. When choosing, it should be decided based on project requirements.
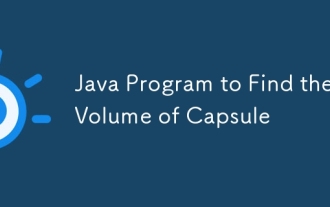
Capsules are three-dimensional geometric figures, composed of a cylinder and a hemisphere at both ends. The volume of the capsule can be calculated by adding the volume of the cylinder and the volume of the hemisphere at both ends. This tutorial will discuss how to calculate the volume of a given capsule in Java using different methods. Capsule volume formula The formula for capsule volume is as follows: Capsule volume = Cylindrical volume Volume Two hemisphere volume in, r: The radius of the hemisphere. h: The height of the cylinder (excluding the hemisphere). Example 1 enter Radius = 5 units Height = 10 units Output Volume = 1570.8 cubic units explain Calculate volume using formula: Volume = π × r2 × h (4
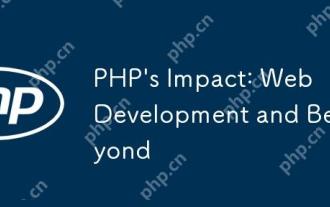
PHPhassignificantlyimpactedwebdevelopmentandextendsbeyondit.1)ItpowersmajorplatformslikeWordPressandexcelsindatabaseinteractions.2)PHP'sadaptabilityallowsittoscaleforlargeapplicationsusingframeworkslikeLaravel.3)Beyondweb,PHPisusedincommand-linescrip
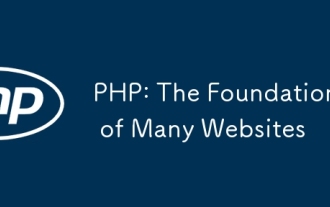
The reasons why PHP is the preferred technology stack for many websites include its ease of use, strong community support, and widespread use. 1) Easy to learn and use, suitable for beginners. 2) Have a huge developer community and rich resources. 3) Widely used in WordPress, Drupal and other platforms. 4) Integrate tightly with web servers to simplify development deployment.
