


Summary of Kotlin syntax learning--variable definition, function expansion, Parcelable serialization
This article mainly introduces Kotlin grammar learning-variable definition, function expansion, Parcelable serialization and other briefly summarized related information. Friends in need can refer to
Kotlin grammar learning-variable definition , function extension, Parcelable serialization, etc. A brief summary
At this year’s Google I/O 2017 Developer Conference, Google announced that it would officially include Kotlin as the official first-level development language for Android programs. (First-class language), as an Android developer, of course, you must gradually become familiar with this language, and the first step is to learn the grammar.
Before this, we need to understand how to use Kotlin to write an Android application. For Android Studio 3.0 version, we can directly check the Include Kotlin support option when creating the project; for versions before 3.0, we need to install the Kotlin plug-in and manually configure gradle as follows.
Add the following code under the gradle of the app
apply plugin: 'kotlin-android' apply plugin: 'kotlin-android-extensions'
Add the following code under the gradle of the project
ext.kotlin_version = '1.1.2-3' classpath "org.jetbrains.kotlin:kotlin-gradle-plugin:$kotlin_version"
Kotlin defines variables
There are two types of variable definitions in kotlin, val and var, where val is equivalent to the final modified variable in Java (read-only), which is usually a constant, and var is usually a variable.
Kotlin's variable definition supports type inference during assignment, and all variables are modified to "not null" by default. You must explicitly add the ? modifier after the type before assigning it to null. .
When we write code, we should try our best to habitually design variables to be non-nullable, so that many problems will be reduced in subsequent operations on the variables.
Kotlin function extension
The specific syntax is fun + type.function (parameter)
fun Context.toast(message: String, length: Int = Toast.LENGTH_SHORT) { Toast.makeText(this, message, length).show() }
Kotlin Parcelable serialization
package com.john.kotlinstudy import android.os.Parcel import android.os.Parcelable /** * Java Bean 数据实体类 * Created by john on 17-5-24. */ data class UserBean(var name: String, var id: String) : Parcelable { constructor(source: Parcel) : this(source.readString(), source.readString()) override fun describeContents(): Int { return 0 } override fun writeToParcel(dest: Parcel, flags: Int) { dest.writeString(this.name) dest.writeString(this.id) } companion object { @JvmField val CREATOR: Parcelable.Creator<UserBean> = object : Parcelable.Creator<UserBean> { override fun createFromParcel(source: Parcel): UserBean { return UserBean(source) } override fun newArray(size: Int): Array<UserBean?> { return arrayOfNulls(size) } } } }
companion keyword interpretation
Unlike Java or C#, in Kotlin , Class does not have static methods. In most cases, it is recommended to use package-level functions instead of static methods.
If you need to write a function (such as a factory function) that can access the inside of Class without instantiating it, you can declare it as a real-name Object within Class.
In addition, if you declare a companion object in Class, all members in the object will be equivalent to using the static modifier in Java/C# syntax, externally These properties or functions can only be accessed through the class name.
@JvmField annotation function
Instructs the Kotlin compiler not to generate getters/setters for this property and replace it Exposed as a field.
If you need to expose a Kotlin property as a field in Java, you need to annotate it with the @JvmField annotation and the field will have the same visibility as the underlying property.
Writing tool classes in Kotlin
In Java, we will encapsulate some commonly used functions into tool classes. The tool class is actually Extensions to the functions of common classes such as String, Collection, IO, etc. The tool class methods and variables we write will be written static. Because we just want to call these methods without involving any attributes and variables in the tool class, so there is no need to instantiate (new). Since there is no need to instantiate, then just use static.
package com.john.kotlinstudy import android.content.Context import android.widget.Toast /** * Toast工具类 * Created by john on 17-5-24. */ object ToastUtils { fun toast(context: Context, message: String) { Toast.makeText(context, message, Toast.LENGTH_SHORT).show() } }
Kotlin Activity Jump
We set the click event in MainActivity, jump to another Activity, and pass the data at the same time
package com.john.kotlinstudy import android.content.Context import android.support.v7.app.AppCompatActivity import android.os.Bundle import android.widget.Toast import kotlinx.android.synthetic.main.activity_main.* class MainActivity : AppCompatActivity() { override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_main) test_tv.text = "hello kotlin" test_tv.setOnClickListener { ToastUtils.toast(this, "hello kotlin") val user = UserBean("zhang", "001") user.id = "100" SecondActivity.navigateTo(this, user) } } fun Context.toast(message: String, length: Int = Toast.LENGTH_SHORT) { Toast.makeText(this, message, length).show() } }
Then create a new A SecondActivity provides a static method for Activity jump. Everyone must know that the advantage of doing this is that the caller knows what parameters are required without looking at the source code. If you write according to java, you will find that there is no static keyword! Don't panic, you can use companion objects to achieve this. Companion objects are objects that accompany the declaration cycle of this class.
package com.john.kotlinstudy import android.content.Context import android.content.Intent import android.os.Bundle import android.support.v7.app.AppCompatActivity import kotlinx.android.synthetic.main.activity_second.* /** * 跳转Activity测试类 * Created by john on 17-5-24. */ class SecondActivity : AppCompatActivity() { override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_second) val user = intent.getParcelableExtra<UserBean>(EXTRA_KEY_USER) user_name_tv.text = user.name ToastUtils.toast(this, user.id) } //创建一个伴生对象 companion object { //extra的key val EXTRA_KEY_USER = "extra.user" fun navigateTo(context: Context, user: UserBean) { val intent = Intent(context, SecondActivity::class.java) intent.putExtra(EXTRA_KEY_USER, user) context.startActivity(intent) } } }
Summary
The above is just a brief introduction to some grammatical features of kotlin, which is considered an introduction. It can eliminate some unfamiliarity and fear of this new language. In fact, kotlin has many new features. , this still requires us to slowly digest and understand it during development.
【Related Recommendations】
1. JavaScript implementation method to determine variable type based on custom function
2. Details in Java How to create objects
3. A brief introduction to several java objects
The above is the detailed content of Summary of Kotlin syntax learning--variable definition, function expansion, Parcelable serialization. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










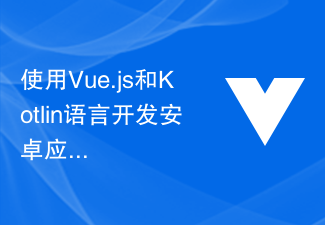
Some tips for developing Android applications using Vue.js and Kotlin language. With the popularity of mobile applications and the continuous growth of user needs, the development of Android applications has attracted more and more attention from developers. When developing Android apps, choosing the right technology stack is crucial. In recent years, Vue.js and Kotlin languages have gradually become popular choices for Android application development. This article will introduce some techniques for developing Android applications using Vue.js and Kotlin language, and give corresponding code examples. 1. Set up the development environment at the beginning
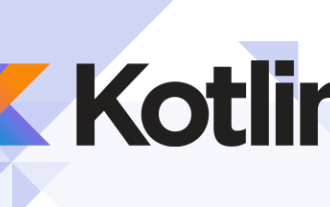
Kotlin is a statically typed programming language that has attracted huge attention in the field of software development. Its concise and easy-to-understand syntax, good compatibility with Java, and rich tool support provide developers with many advantages, so many developers choose Kotlin as their preferred language. Install Kotlin Programming Language 12Bookworm on Debian Step 1. Start by updating existing system packages. Open a terminal and enter the following commands: sudoaptupdatesudoaptupgrade These commands will get a list of available updates and upgrade current packages, ensuring your system is up to date. Step 2. Install Java. Kotlin in the Java Virtual Machine (J

The difference between Java and Kotlin functions: Syntax: Java functions need to specify parameter types and names, while Kotlin can omit the type and use lambda expressions; Parameters: Kotlin can omit parameter types using more concise syntax; Return value: Kotlin can omit the return value Type, the default is Unit; extension function: Kotlin can add new functions to existing classes, while Java needs to implement similar functions through inheritance; instance method call: Kotlin can omit the object name and use a more concise syntax.
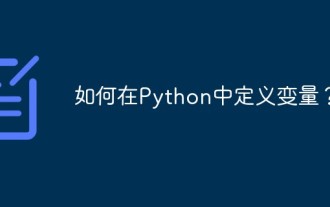
In Python, variables can be understood as containers for storing data. When we need to use or manipulate data, we can define variables to store the data so that we can easily call and process the data. The following will introduce how to define variables in Python. 1. Naming rules In Python, the naming rules for variables are very flexible and usually need to follow the following rules: variable names consist of letters, underscores and numbers, and the first part cannot be a number. Variable names can use uppercase and lowercase letters, but Python is case-sensitive. variable name
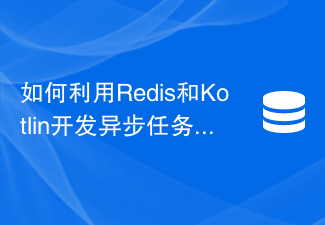
How to use Redis and Kotlin to develop asynchronous task queue functions Introduction: With the development of the Internet, the processing of asynchronous tasks has become more and more important. During the development process, we often encounter some time-consuming tasks, such as sending emails, processing big data, etc. In order to improve the performance and scalability of the system, we can use asynchronous task queues to process these tasks. This article will introduce how to use Redis and Kotlin to develop a simple asynchronous task queue, and provide specific code examples. 1. What is an asynchronous task?
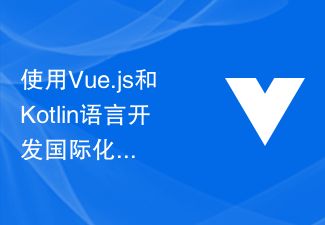
Use Vue.js and Kotlin language to develop mobile application solutions with international support. As the process of globalization accelerates, more and more mobile applications need to provide multi-language support to meet the needs of global users. During the development process, we can use Vue.js and Kotlin languages to implement internationalization functions so that the application can run normally in different language environments. 1. Vue.js international support Vue.js is a popular JavaScript framework that provides a wealth of tools and features.
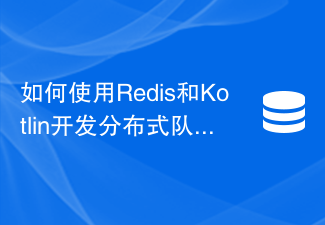
How to use Redis and Kotlin to develop distributed queue functions Introduction: With the rapid development of the Internet, distributed systems have attracted more and more attention. Distributed queue is one of the important components of distributed system, which can realize asynchronous processing and decoupling of messages. This article will introduce how to develop a simple distributed queue using Redis and Kotlin, and provide specific code examples. 1. Overview Distributed queues can publish and consume messages and ensure that messages are not lost. In a distributed system, the publishing and consumption of messages
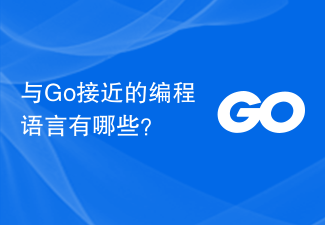
What programming languages are close to Go? In recent years, the Go language has gradually emerged in the field of software development and is favored by more and more developers. Although the Go language itself has the characteristics of simplicity, efficiency and strong concurrency, it sometimes encounters some limitations and shortcomings. Therefore, looking for a programming language that is close to the Go language has become a need. The following will introduce some programming languages close to the Go language and demonstrate their similarities through specific code examples. RustRust is a systems programming language with a focus on safety and concurrency
