Four ways to parse XML
Four ways to parse XML
XML has now become a universal The data exchange format, with its platform independence, language independence and system independence, brings great convenience to data integration and interaction. For the grammatical knowledge and technical details of XML itself, you need to read relevant technical literature, which includes DOM (Document Object Model), DTD (Document Type Definition), SAX (Simple API for XML), XSD (Xml Schema Definition) ), XSLT (Extensible Stylesheet Language Transformations), please refer to the w3c official website documentation for more information.
XML is parsed in the same way in different languages, but the syntax implemented is different. There are two basic parsing methods, one is called SAX, and the other is called DOM. SAX is based on event stream parsing, and DOM is based on XML document tree structure parsing. Assume that the content and structure of our XML are as follows:
<?xml version="1.0" encoding="UTF-8"?><employees><employee> <name>ddviplinux</name> <sex>m</sex> <age>30</age></employee></employees>
This article uses JAVA language to generate and parse XML documents of DOM and SAX.
First define an interface for operating XML documents, XmlDocument. It defines the interface for creating and parsing XML documents.
package com.beyond.framework.bean; /** * @author zhengwei * 定义XML文档建立与解析的接口 */ public interface XmlDocument { /** * 建立XML文档 * @param fileName 文件全路径名称 */ public void createXml(String fileName); /** * 解析XML文档 * @param fileName 文件全路径名称 */ public void parserXml(String fileName); }
1. DOMGenerate and parse XML documents
## Defines a set of interfaces for the parsed version of an XML document. The parser reads in the entire document and builds a memory-resident tree structure that code can then manipulate using the DOM interface.
Advantages: The entire document tree is in memory, easy to operate; supports deletion, modification, rearrangement and other functions; Disadvantages: The entire document is transferred into memory (including useless nodes) , a waste of time and space; Usage occasions: Once the document is parsed, the data needs to be accessed multiple times; hardware resources are sufficient (memory, CPU).DomDemo ==.document = = .document.createElement("employees"= .document.createElement("employee"= .document.createElement("name".document.createTextNode("丁宏亮"= .document.createElement("sex".document.createTextNode("m"= .document.createElement("age".document.createTextNode("30"=== "gb2312""yes"= PrintWriter(= "生成XML文件成功!" ==== ( i = 0; i < employees.getLength(); i++== ( j = 0; j < employeeInfo.getLength(); j++== ( k = 0; k < employeeMeta.getLength(); k+++ ":" +"解析完毕"
2. SAXGenerate and parse XML documents
import java.io.FileInputStream;import java.io.FileNotFoundException;import java.io.IOException;import java.io.InputStream;import javax.xml.parsers.ParserConfigurationException;import javax.xml.parsers.SAXParser;import javax.xml.parsers.SAXParserFactory;import org.xml.sax.Attributes;import org.xml.sax.SAXException;import org.xml.sax.helpers.DefaultHandler;/*** @author zhengwei * SAX文档解析*/public class SaxDemo implements XmlDocument { public void createXml(String fileName) { System.out.println("<<"+filename+">>"); } public void parserXml(String fileName) { SAXParserFactory saxfac = SAXParserFactory.newInstance(); try { SAXParser saxparser = saxfac.newSAXParser(); InputStream is = new FileInputStream(fileName); saxparser.parse(is, new MySAXHandler()); } catch (ParserConfigurationException e) { e.printStackTrace(); } catch (SAXException e) { e.printStackTrace(); } catch (FileNotFoundException e) { e.printStackTrace(); } catch (IOException e) { e.printStackTrace(); } } } class MySAXHandler extends DefaultHandler { boolean hasAttribute = false; Attributes attributes = null; public void startDocument() throws SAXException { System.out.println("文档开始打印了"); } public void endDocument() throws SAXException { System.out.println("文档打印结束了"); } public void startElement(String uri, String localName, String qName, Attributes attributes) throws SAXException { if (qName.equals("employees")) { return; } if (qName.equals("employee")) { System.out.println(qName); } if (attributes.getLength() > 0) { this.attributes = attributes; this.hasAttribute = true; } } public void endElement(String uri, String localName, String qName) throws SAXException { if (hasAttribute && (attributes != null)) { for (int i = 0; i < attributes.getLength(); i++) { System.out.println(attributes.getQName(0) + attributes.getValue(0)); } } } public void characters(char[] ch, int start, int length) throws SAXException { System.out.println(new String(ch, start, length)); } }
3. DOM4JGenerate and parse XML documents
has excellent performance, powerful functions and extreme ease of useSpeciality, at the same time it is also an open source software. Nowadays you can see that more and more Java software is using DOM4J to read and write XML. It is particularly worth mentioning that even Sun's JAXM is also using DOM4J.
import java.io.File; import java.io.FileWriter; import java.io.IOException; import java.io.Writer; import java.util.Iterator; import org.dom4j.Document; import org.dom4j.DocumentException; import org.dom4j.DocumentHelper; import org.dom4j.Element; import org.dom4j.io.SAXReader; import org.dom4j.io.XMLWriter; /** * @author zhengwei * Dom4j 生成XML文档与解析XML文档 */ public class Dom4jDemo implements XmlDocument { public void createXml(String fileName) { Document document = DocumentHelper.createDocument(); Element employees=document.addElement("employees"); Element employee=employees.addElement("employee"); Element name= employee.addElement("name"); name.setText("ddvip"); Element sex=employee.addElement("sex"); sex.setText("m"); Element age=employee.addElement("age"); age.setText("29"); try { Writer fileWriter=new FileWriter(fileName); XMLWriter xmlWriter=new XMLWriter(fileWriter); xmlWriter.write(document); xmlWriter.close(); } catch (IOException e) { System.out.println(e.getMessage()); } } public void parserXml(String fileName) { File inputXml=new File(fileName); SAXReader saxReader = new SAXReader(); try { Document document = saxReader.read(inputXml); Element employees=document.getRootElement(); for(Iterator i = employees.elementIterator(); i.hasNext();){ Element employee = (Element) i.next();for(Iterator j = employee.elementIterator(); j.hasNext();){ Element node=(Element) j.next(); System.out.println(node.getName()+":"+node.getText()); } } } catch (DocumentException e) { System.out.println(e.getMessage()); } System.out.println("dom4j parserXml"); } }
4. JDOMGenerate and parse XML
import java.io.FileNotFoundException; import java.io.FileOutputStream; import java.io.IOException; import java.util.List; import org.jdom.Document; import org.jdom.Element; import org.jdom.JDOMException; import org.jdom.input.SAXBuilder; import org.jdom.output.XMLOutputter; /*** @author zhengwei * JDOM 生成与解析XML文档 */ public class JDomDemo implements XmlDocument { public void createXml(String fileName) { Document document; Element root; root=new Element("employees"); document=new Document(root); Element employee=new Element("employee"); root.addContent(employee); Element name=new Element("name"); name.setText("ddvip"); employee.addContent(name); Element sex=new Element("sex"); sex.setText("m"); employee.addContent(sex); Element age=new Element("age"); age.setText("23"); employee.addContent(age); XMLOutputter XMLOut = new XMLOutputter(); try { XMLOut.output(document, new FileOutputStream(fileName)); } catch (FileNotFoundException e) { e.printStackTrace(); } catch (IOException e) { e.printStackTrace(); } }public void parserXml(String fileName) { SAXBuilder builder=new SAXBuilder(false); try { Document document=builder.build(fileName); Element employees=document.getRootElement(); List employeeList=employees.getChildren("employee"); for(int i=0;i<EMPLOYEELIST.SIZE();I++){ iElement employee=(Element)employeeList.get(i); List employeeInfo=employee.getChildren(); for(int j=0;j<EMPLOYEEINFO.SIZE();J++){ System.out.println(((Element)employeeInfo.get(j)).getName()+":" +((Element)employeeInfo.get(j)).getValue()) } } } catch (JDOMException e) { e.printStackTrace(); } catch (IOException e) { e.printStackTrace(); } } }
5. Use dom4j to parse XML
Listing 1. Example XML Document (catalog.xml)
<?xml version="1.0" encoding="UTF-8"?> <catalog> <!--An XML Catalog--> <?target instruction?><journal title="XML Zone" publisher="IBM developerWorks"> <article level="Intermediate" date="December-2001"> <title>Java configuration with XML Schema</title> <author> <firstname>Marcello</firstname> <lastname>Vitaletti</lastname> </author> </article></journal> </catalog>
List 2. Modified XML Document (catalog-modified.xml)
<?xml version="1.0" encoding="UTF-8"?><catalog> <!--An XML catalog--><?target instruction?><journal title="XML Zone" publisher="IBM developerWorks"><article level="Introductory" date="October-2002"> <title>Create flexible and extensible XML schemas</title> <author><firstname>Ayesha</firstname> <lastname>Malik</lastname> </author> </article></journal></catalog>
Preset
Create document
- ##Modify document
Preset
This parser can be obtained from . Make dom4j-1.4/dom4j-full.jar accessible on the classpath, which includes the dom4j classes, the XPath engine, and the SAX and DOM interfaces. If you are already using the SAX and DOM interfaces included in the JAXP parser, add dom4j-1.4/dom4j.jar to the classpath. dom4j.jar includes the dom4j class and XPath engine, but does not include SAX and DOM interfaces.Creating a document
import org .dom4j.DocumentHelper; import org.dom4j.Element; |
# Use the DocumentHelper class to create a Document instance. DocumentHelper is a dom4j API factory class that generates XML document nodes.
Use the addElement() method to create the root element catalog. addElement() is used to add elements to an XML document.
|
##catalogElement.addProcessingInstruction("target","text");
Use the addElement() method in the catalog element to add the journal element. |
Use the addAttribute() method to add title and publisher attributes to the journal element. |
journalElement.addAttribute("publisher", " IBM developerWorks");
Add a journal element to the article element. |
##Element articleElement=journalElement.addElement("article");
Add level and date attributes to the article element. |
Add the title element to the article element. |
##Element lastNameElement=authorElement.addElement("lastname"); |
可以使用 addDocType()方法添加文档类型说明。
document.addDocType("catalog", null,"file://c:/Dtds/catalog.dtd"); |
这样就向 XML 文档中增加文档类型说明:
如果文档要使用文档类型定义(DTD)文档验证则必须有 Doctype。
XML 声明 自动添加到 XML 文档中。
清单 3 所示的例子程序 XmlDom4J.java 用于创建 XML 文档 catalog.xml。
清单 3. 生成 XML 文档 catalog.xml 的程序(XmlDom4J.java)
import org.dom4j.Document;import org.dom4j.DocumentHelper;import org.dom4j.Element;import org.dom4j.io.XMLWriter;import java.io.*;public class XmlDom4J{public void generateDocument(){ Document document = DocumentHelper.createDocument(); Element catalogElement = document.addElement("catalog"); catalogElement.addComment("An XML Catalog"); catalogElement.addProcessingInstruction("target","text"); Element journalElement = catalogElement.addElement("journal"); journalElement.addAttribute("title", "XML Zone"); journalElement.addAttribute("publisher", "IBM developerWorks"); Element articleElement=journalElement.addElement("article"); articleElement.addAttribute("level", "Intermediate"); articleElement.addAttribute("date", "December-2001"); Element titleElement=articleElement.addElement("title"); titleElement.setText("Java configuration with XML Schema"); Element authorElement=articleElement.addElement("author"); Element firstNameElement=authorElement.addElement("firstname"); firstNameElement.setText("Marcello"); Element lastNameElement=authorElement.addElement("lastname"); lastNameElement.setText("Vitaletti"); document.addDocType("catalog",null,"file://c:/Dtds/catalog.dtd");try{ XMLWriter output = new XMLWriter( new FileWriter(new File("c:/catalog/catalog.xml"))); output.write( document ); output.close(); } catch(IOException e){ System.out.println(e.getMessage()); } }public static void main(String[] argv){ XmlDom4J dom4j=new XmlDom4J(); dom4j.generateDocument(); } }
这一节讨论了创建 XML 文档的过程,下一节将介绍使用 dom4j API 修改这里创建的 XML 文档。
修改文档
这一节说明如何使用 dom4j API 修改示例 XML 文档 catalog.xml。
使用 SAXReader 解析 XML 文档 catalog.xml:
SAXReader saxReader = new SAXReader(); Document document = saxReader.read(inputXml);
SAXReader 包含在 org.dom4j.io 包中。
inputXml 是从 c:/catalog/catalog.xml 创建的 java.io.File。使用 XPath 表达式从 article 元素中获得 level 节点列表。如果 level 属性值是“Intermediate”则改为“Introductory”。
List list = document.selectNodes("//article/@level" ); Iterator iter=list.iterator(); while(iter.hasNext()){ Attribute attribute=(Attribute)iter.next(); if(attribute.getValue().equals("Intermediate")) attribute.setValue("Introductory"); }
获取 article 元素列表,从 article 元素中的 title 元素得到一个迭代器,并修改 title 元素的文本。
list = document.selectNodes("//article" ); iter=list.iterator(); while(iter.hasNext()){ Element element=(Element)iter.next(); Iterator iterator=element.elementIterator("title"); while(iterator.hasNext()){ Element titleElement=(Element)iterator.next(); if(titleElement.getText().equals("Java configuration with XML Schema")) titleElement.setText("Create flexible and extensible XML schema"); } }
通过和 title 元素类似的过程修改 author 元素。
清单 4 所示的示例程序 Dom4JParser.java 用于把 catalog.xml 文档修改成 catalog-modified.xml 文档。
清单 4. 用于修改 catalog.xml 的程序(Dom4Jparser.java)
import org.dom4j.Document;import org.dom4j.Element;import org.dom4j.Attribute;import java.util.List;import java.util.Iterator;import org.dom4j.io.XMLWriter;import java.io.*;import org.dom4j.DocumentException;import org.dom4j.io.SAXReader;public class Dom4JParser{public void modifyDocument(File inputXml){try{ SAXReader saxReader = new SAXReader(); Document document = saxReader.read(inputXml); List list = document.selectNodes("//article/@level" ); Iterator iter=list.iterator();while(iter.hasNext()){ Attribute attribute=(Attribute)iter.next();if(attribute.getValue().equals("Intermediate")) attribute.setValue("Introductory"); } list = document.selectNodes("//article/@date" ); iter=list.iterator();while(iter.hasNext()){ Attribute attribute=(Attribute)iter.next();if(attribute.getValue().equals("December-2001")) attribute.setValue("October-2002"); } list = document.selectNodes("//article" ); iter=list.iterator();while(iter.hasNext()){ Element element=(Element)iter.next(); Iterator iterator=element.elementIterator("title");while(iterator.hasNext()){ Element titleElement=(Element)iterator.next();if(titleElement.getText().equals("Java configuration with XMLSchema")) titleElement.setText("Create flexible and extensible XML schema"); } } list = document.selectNodes("//article/author" ); iter=list.iterator();while(iter.hasNext()){ Element element=(Element)iter.next(); Iterator iterator=element.elementIterator("firstname");while(iterator.hasNext()){ Element firstNameElement=(Element)iterator.next();if(firstNameElement.getText().equals("Marcello")) firstNameElement.setText("Ayesha"); } } list = document.selectNodes("//article/author" ); iter=list.iterator();while(iter.hasNext()){ Element element=(Element)iter.next(); Iterator iterator=element.elementIterator("lastname");while(iterator.hasNext()){ Element lastNameElement=(Element)iterator.next();if(lastNameElement.getText().equals("Vitaletti")) lastNameElement.setText("Malik"); } } XMLWriter output = new XMLWriter(new FileWriter( new File("c:/catalog/catalog-modified.xml") )); output.write( document ); output.close(); } catch(DocumentException e) { System.out.println(e.getMessage()); } catch(IOException e){ System.out.println(e.getMessage()); } }public static void main(String[] argv){ Dom4JParser dom4jParser=new Dom4JParser(); dom4jParser.modifyDocument(new File("c:/catalog/catalog.xml")); } }
结束语:包含在 dom4j 中的解析器是一种用于解析 XML 文档的非验证性工具,可以与JAXP、Crimson 或 Xerces 集成。本文说明了如何使用该解析器创建和修改 XML 文档。
The above is the detailed content of Four ways to parse XML. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










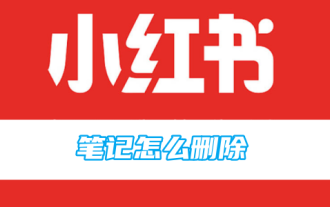
How to delete Xiaohongshu notes? Notes can be edited in the Xiaohongshu APP. Most users don’t know how to delete Xiaohongshu notes. Next, the editor brings users pictures and texts on how to delete Xiaohongshu notes. Tutorial, interested users come and take a look! Xiaohongshu usage tutorial How to delete Xiaohongshu notes 1. First open the Xiaohongshu APP and enter the main page, select [Me] in the lower right corner to enter the special area; 2. Then in the My area, click on the note page shown in the picture below , select the note you want to delete; 3. Enter the note page, click [three dots] in the upper right corner; 4. Finally, the function bar will expand at the bottom, click [Delete] to complete.
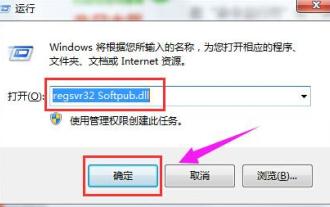
When deleting or decompressing a folder on your computer, sometimes a prompt dialog box "Error 0x80004005: Unspecified Error" will pop up. How should you solve this situation? There are actually many reasons why the error code 0x80004005 is prompted, but most of them are caused by viruses. We can re-register the dll to solve the problem. Below, the editor will explain to you the experience of handling the 0x80004005 error code. Some users are prompted with error code 0X80004005 when using their computers. The 0x80004005 error is mainly caused by the computer not correctly registering certain dynamic link library files, or by a firewall that does not allow HTTPS connections between the computer and the Internet. So how about
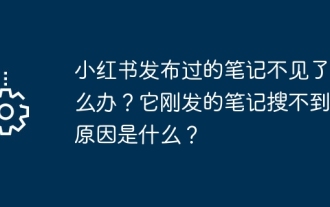
As a Xiaohongshu user, we have all encountered the situation where published notes suddenly disappeared, which is undoubtedly confusing and worrying. In this case, what should we do? This article will focus on the topic of "What to do if the notes published by Xiaohongshu are missing" and give you a detailed answer. 1. What should I do if the notes published by Xiaohongshu are missing? First, don't panic. If you find that your notes are missing, staying calm is key and don't panic. This may be caused by platform system failure or operational errors. Checking release records is easy. Just open the Xiaohongshu App and click "Me" → "Publish" → "All Publications" to view your own publishing records. Here you can easily find previously published notes. 3.Repost. If found
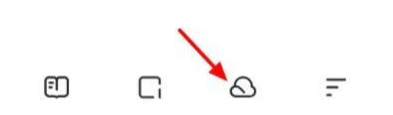
Quark Netdisk and Baidu Netdisk are currently the most commonly used Netdisk software for storing files. If you want to save the files in Quark Netdisk to Baidu Netdisk, how do you do it? In this issue, the editor has compiled the tutorial steps for transferring files from Quark Network Disk computer to Baidu Network Disk. Let’s take a look at how to operate it. How to save Quark network disk files to Baidu network disk? To transfer files from Quark Network Disk to Baidu Network Disk, you first need to download the required files from Quark Network Disk, then select the target folder in the Baidu Network Disk client and open it. Then, drag and drop the files downloaded from Quark Cloud Disk into the folder opened by the Baidu Cloud Disk client, or use the upload function to add the files to Baidu Cloud Disk. Make sure to check whether the file was successfully transferred in Baidu Cloud Disk after the upload is completed. That's it
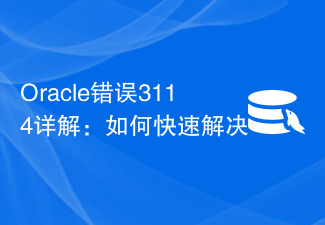
Detailed explanation of Oracle error 3114: How to solve it quickly, specific code examples are needed. During the development and management of Oracle database, we often encounter various errors, among which error 3114 is a relatively common problem. Error 3114 usually indicates a problem with the database connection, which may be caused by network failure, database service stop, or incorrect connection string settings. This article will explain in detail the cause of error 3114 and how to quickly solve this problem, and attach the specific code
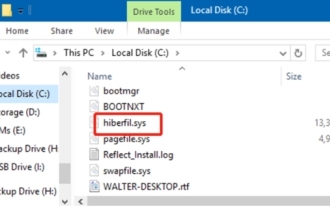
Recently, many netizens have asked the editor, what is the file hiberfil.sys? Can hiberfil.sys take up a lot of C drive space and be deleted? The editor can tell you that the hiberfil.sys file can be deleted. Let’s take a look at the details below. hiberfil.sys is a hidden file in the Windows system and also a system hibernation file. It is usually stored in the root directory of the C drive, and its size is equivalent to the size of the system's installed memory. This file is used when the computer is hibernated and contains the memory data of the current system so that it can be quickly restored to the previous state during recovery. Since its size is equal to the memory capacity, it may take up a larger amount of hard drive space. hiber
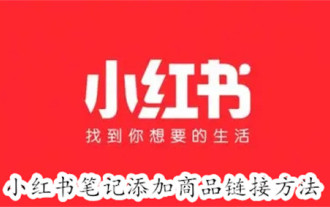
How to add product links in notes in Xiaohongshu? In the Xiaohongshu app, users can not only browse various contents but also shop, so there is a lot of content about shopping recommendations and good product sharing in this app. If If you are an expert on this app, you can also share some shopping experiences, find merchants for cooperation, add links in notes, etc. Many people are willing to use this app for shopping, because it is not only convenient, but also has many Experts will make some recommendations. You can browse interesting content and see if there are any clothing products that suit you. Let’s take a look at how to add product links to notes! How to add product links to Xiaohongshu Notes Open the app on the desktop of your mobile phone. Click on the app homepage
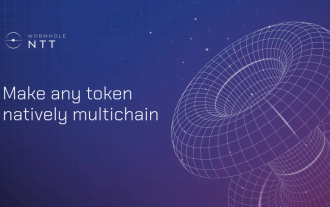
Wormhole is a leader in blockchain interoperability, focused on creating resilient, future-proof decentralized systems that prioritize ownership, control, and permissionless innovation. The foundation of this vision is a commitment to technical expertise, ethical principles, and community alignment to redefine the interoperability landscape with simplicity, clarity, and a broad suite of multi-chain solutions. With the rise of zero-knowledge proofs, scaling solutions, and feature-rich token standards, blockchains are becoming more powerful and interoperability is becoming increasingly important. In this innovative application environment, novel governance systems and practical capabilities bring unprecedented opportunities to assets across the network. Protocol builders are now grappling with how to operate in this emerging multi-chain
